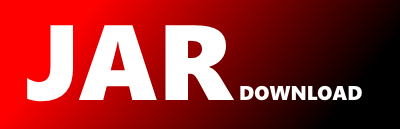
odata.msgraph.client.entity.Security Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.entity.collection.request.SecureScoreCollectionRequest;
import odata.msgraph.client.entity.collection.request.SecureScoreControlProfileCollectionRequest;
import odata.msgraph.client.entity.collection.request.SubjectRightsRequestCollectionRequest;
import odata.msgraph.client.entity.request.AttackSimulationRootRequest;
import odata.msgraph.client.security.entity.Alert;
import odata.msgraph.client.security.entity.CasesRoot;
import odata.msgraph.client.security.entity.Incident;
import odata.msgraph.client.security.entity.ThreatIntelligence;
import odata.msgraph.client.security.entity.TriggerTypesRoot;
import odata.msgraph.client.security.entity.TriggersRoot;
import odata.msgraph.client.security.entity.collection.request.AlertCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.IncidentCollectionRequest;
import odata.msgraph.client.security.entity.request.CasesRootRequest;
import odata.msgraph.client.security.entity.request.ThreatIntelligenceRequest;
import odata.msgraph.client.security.entity.request.TriggerTypesRootRequest;
import odata.msgraph.client.security.entity.request.TriggersRootRequest;
/**
*
* Org.OData.Capabilities.V1.CountRestrictions
*
* Countable = false
*
* Org.OData.Capabilities.V1.ExpandRestrictions
*
* Expandable = true
*
* Org.OData.Capabilities.V1.FilterRestrictions
*
* Filterable = false
*
* Org.OData.Capabilities.V1.SearchRestrictions
*
* Searchable = false
*
* Org.OData.Capabilities.V1.SelectRestrictions
*
* Selectable = false
*
* Org.OData.Capabilities.V1.SkipSupported
*
* true
*
* Org.OData.Capabilities.V1.SortRestrictions
*
* Sortable = true
*
* Org.OData.Capabilities.V1.TopSupported
*
* false
*/@JsonPropertyOrder({
"@odata.type",
"subjectRightsRequests",
"cases",
"alerts_v2",
"incidents",
"attackSimulation",
"triggers",
"triggerTypes",
"alerts",
"secureScoreControlProfiles",
"secureScores",
"threatIntelligence"})
@JsonInclude(Include.NON_NULL)
public class Security extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.security";
}
@JsonProperty("subjectRightsRequests")
protected List subjectRightsRequests;
@JsonProperty("cases")
protected CasesRoot cases;
@JsonProperty("alerts_v2")
protected List alerts_v2;
@JsonProperty("incidents")
protected List incidents;
@JsonProperty("attackSimulation")
protected AttackSimulationRoot attackSimulation;
@JsonProperty("triggers")
protected TriggersRoot triggers;
@JsonProperty("triggerTypes")
protected TriggerTypesRoot triggerTypes;
@JsonProperty("alerts")
protected List alerts;
@JsonProperty("secureScoreControlProfiles")
protected List secureScoreControlProfiles;
@JsonProperty("secureScores")
protected List secureScores;
@JsonProperty("threatIntelligence")
protected ThreatIntelligence threatIntelligence;
protected Security() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderSecurity() {
return new Builder();
}
public static final class Builder {
private String id;
private List subjectRightsRequests;
private CasesRoot cases;
private List alerts_v2;
private List incidents;
private AttackSimulationRoot attackSimulation;
private TriggersRoot triggers;
private TriggerTypesRoot triggerTypes;
private List alerts;
private List secureScoreControlProfiles;
private List secureScores;
private ThreatIntelligence threatIntelligence;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder subjectRightsRequests(List subjectRightsRequests) {
this.subjectRightsRequests = subjectRightsRequests;
this.changedFields = changedFields.add("subjectRightsRequests");
return this;
}
public Builder subjectRightsRequests(SubjectRightsRequest... subjectRightsRequests) {
return subjectRightsRequests(Arrays.asList(subjectRightsRequests));
}
public Builder cases(CasesRoot cases) {
this.cases = cases;
this.changedFields = changedFields.add("cases");
return this;
}
public Builder alerts_v2(List alerts_v2) {
this.alerts_v2 = alerts_v2;
this.changedFields = changedFields.add("alerts_v2");
return this;
}
public Builder alerts_v2(Alert... alerts_v2) {
return alerts_v2(Arrays.asList(alerts_v2));
}
public Builder incidents(List incidents) {
this.incidents = incidents;
this.changedFields = changedFields.add("incidents");
return this;
}
public Builder incidents(Incident... incidents) {
return incidents(Arrays.asList(incidents));
}
public Builder attackSimulation(AttackSimulationRoot attackSimulation) {
this.attackSimulation = attackSimulation;
this.changedFields = changedFields.add("attackSimulation");
return this;
}
public Builder triggers(TriggersRoot triggers) {
this.triggers = triggers;
this.changedFields = changedFields.add("triggers");
return this;
}
public Builder triggerTypes(TriggerTypesRoot triggerTypes) {
this.triggerTypes = triggerTypes;
this.changedFields = changedFields.add("triggerTypes");
return this;
}
public Builder alerts(List alerts) {
this.alerts = alerts;
this.changedFields = changedFields.add("alerts");
return this;
}
public Builder alerts(odata.msgraph.client.entity.Alert... alerts) {
return alerts(Arrays.asList(alerts));
}
public Builder secureScoreControlProfiles(List secureScoreControlProfiles) {
this.secureScoreControlProfiles = secureScoreControlProfiles;
this.changedFields = changedFields.add("secureScoreControlProfiles");
return this;
}
public Builder secureScoreControlProfiles(SecureScoreControlProfile... secureScoreControlProfiles) {
return secureScoreControlProfiles(Arrays.asList(secureScoreControlProfiles));
}
public Builder secureScores(List secureScores) {
this.secureScores = secureScores;
this.changedFields = changedFields.add("secureScores");
return this;
}
public Builder secureScores(SecureScore... secureScores) {
return secureScores(Arrays.asList(secureScores));
}
public Builder threatIntelligence(ThreatIntelligence threatIntelligence) {
this.threatIntelligence = threatIntelligence;
this.changedFields = changedFields.add("threatIntelligence");
return this;
}
public Security build() {
Security _x = new Security();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.security";
_x.id = id;
_x.subjectRightsRequests = subjectRightsRequests;
_x.cases = cases;
_x.alerts_v2 = alerts_v2;
_x.incidents = incidents;
_x.attackSimulation = attackSimulation;
_x.triggers = triggers;
_x.triggerTypes = triggerTypes;
_x.alerts = alerts;
_x.secureScoreControlProfiles = secureScoreControlProfiles;
_x.secureScores = secureScores;
_x.threatIntelligence = threatIntelligence;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
public Security withUnmappedField(String name, Object value) {
Security _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="subjectRightsRequests")
@JsonIgnore
public SubjectRightsRequestCollectionRequest getSubjectRightsRequests() {
return new SubjectRightsRequestCollectionRequest(
contextPath.addSegment("subjectRightsRequests"), Optional.ofNullable(subjectRightsRequests));
}
@NavigationProperty(name="cases")
@JsonIgnore
public CasesRootRequest getCases() {
return new CasesRootRequest(contextPath.addSegment("cases"), Optional.ofNullable(cases));
}
@NavigationProperty(name="alerts_v2")
@JsonIgnore
public AlertCollectionRequest getAlerts_v2() {
return new AlertCollectionRequest(
contextPath.addSegment("alerts_v2"), Optional.ofNullable(alerts_v2));
}
@NavigationProperty(name="incidents")
@JsonIgnore
public IncidentCollectionRequest getIncidents() {
return new IncidentCollectionRequest(
contextPath.addSegment("incidents"), Optional.ofNullable(incidents));
}
@NavigationProperty(name="attackSimulation")
@JsonIgnore
public AttackSimulationRootRequest getAttackSimulation() {
return new AttackSimulationRootRequest(contextPath.addSegment("attackSimulation"), Optional.ofNullable(attackSimulation));
}
@NavigationProperty(name="triggers")
@JsonIgnore
public TriggersRootRequest getTriggers() {
return new TriggersRootRequest(contextPath.addSegment("triggers"), Optional.ofNullable(triggers));
}
@NavigationProperty(name="triggerTypes")
@JsonIgnore
public TriggerTypesRootRequest getTriggerTypes() {
return new TriggerTypesRootRequest(contextPath.addSegment("triggerTypes"), Optional.ofNullable(triggerTypes));
}
@NavigationProperty(name="alerts")
@JsonIgnore
public odata.msgraph.client.entity.collection.request.AlertCollectionRequest getAlerts() {
return new odata.msgraph.client.entity.collection.request.AlertCollectionRequest(
contextPath.addSegment("alerts"), Optional.ofNullable(alerts));
}
@NavigationProperty(name="secureScoreControlProfiles")
@JsonIgnore
public SecureScoreControlProfileCollectionRequest getSecureScoreControlProfiles() {
return new SecureScoreControlProfileCollectionRequest(
contextPath.addSegment("secureScoreControlProfiles"), Optional.ofNullable(secureScoreControlProfiles));
}
@NavigationProperty(name="secureScores")
@JsonIgnore
public SecureScoreCollectionRequest getSecureScores() {
return new SecureScoreCollectionRequest(
contextPath.addSegment("secureScores"), Optional.ofNullable(secureScores));
}
@NavigationProperty(name="threatIntelligence")
@JsonIgnore
public ThreatIntelligenceRequest getThreatIntelligence() {
return new ThreatIntelligenceRequest(contextPath.addSegment("threatIntelligence"), Optional.ofNullable(threatIntelligence));
}
public Security withSubjectRightsRequests(List subjectRightsRequests) {
Security _x = _copy();
_x.changedFields = changedFields.add("subjectRightsRequests");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security");
_x.subjectRightsRequests = subjectRightsRequests;
return _x;
}
public Security withCases(CasesRoot cases) {
Security _x = _copy();
_x.changedFields = changedFields.add("cases");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security");
_x.cases = cases;
return _x;
}
public Security withAlerts_v2(List alerts_v2) {
Security _x = _copy();
_x.changedFields = changedFields.add("alerts_v2");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security");
_x.alerts_v2 = alerts_v2;
return _x;
}
public Security withIncidents(List incidents) {
Security _x = _copy();
_x.changedFields = changedFields.add("incidents");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security");
_x.incidents = incidents;
return _x;
}
public Security withAttackSimulation(AttackSimulationRoot attackSimulation) {
Security _x = _copy();
_x.changedFields = changedFields.add("attackSimulation");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security");
_x.attackSimulation = attackSimulation;
return _x;
}
public Security withTriggers(TriggersRoot triggers) {
Security _x = _copy();
_x.changedFields = changedFields.add("triggers");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security");
_x.triggers = triggers;
return _x;
}
public Security withTriggerTypes(TriggerTypesRoot triggerTypes) {
Security _x = _copy();
_x.changedFields = changedFields.add("triggerTypes");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security");
_x.triggerTypes = triggerTypes;
return _x;
}
public Security withAlerts(List alerts) {
Security _x = _copy();
_x.changedFields = changedFields.add("alerts");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security");
_x.alerts = alerts;
return _x;
}
public Security withSecureScoreControlProfiles(List secureScoreControlProfiles) {
Security _x = _copy();
_x.changedFields = changedFields.add("secureScoreControlProfiles");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security");
_x.secureScoreControlProfiles = secureScoreControlProfiles;
return _x;
}
public Security withSecureScores(List secureScores) {
Security _x = _copy();
_x.changedFields = changedFields.add("secureScores");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security");
_x.secureScores = secureScores;
return _x;
}
public Security withThreatIntelligence(ThreatIntelligence threatIntelligence) {
Security _x = _copy();
_x.changedFields = changedFields.add("threatIntelligence");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security");
_x.threatIntelligence = threatIntelligence;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Security patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Security _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Security put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Security _x = _copy();
_x.changedFields = null;
return _x;
}
private Security _copy() {
Security _x = new Security();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.subjectRightsRequests = subjectRightsRequests;
_x.cases = cases;
_x.alerts_v2 = alerts_v2;
_x.incidents = incidents;
_x.attackSimulation = attackSimulation;
_x.triggers = triggers;
_x.triggerTypes = triggerTypes;
_x.alerts = alerts;
_x.secureScoreControlProfiles = secureScoreControlProfiles;
_x.secureScores = secureScores;
_x.threatIntelligence = threatIntelligence;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Security[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("subjectRightsRequests=");
b.append(this.subjectRightsRequests);
b.append(", ");
b.append("cases=");
b.append(this.cases);
b.append(", ");
b.append("alerts_v2=");
b.append(this.alerts_v2);
b.append(", ");
b.append("incidents=");
b.append(this.incidents);
b.append(", ");
b.append("attackSimulation=");
b.append(this.attackSimulation);
b.append(", ");
b.append("triggers=");
b.append(this.triggers);
b.append(", ");
b.append("triggerTypes=");
b.append(this.triggerTypes);
b.append(", ");
b.append("alerts=");
b.append(this.alerts);
b.append(", ");
b.append("secureScoreControlProfiles=");
b.append(this.secureScoreControlProfiles);
b.append(", ");
b.append("secureScores=");
b.append(this.secureScores);
b.append(", ");
b.append("threatIntelligence=");
b.append(this.threatIntelligence);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}