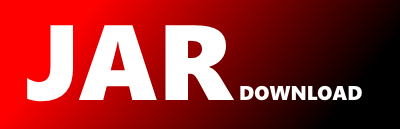
odata.msgraph.client.entity.ServicePrincipalRiskDetection Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.complex.SignInLocation;
import odata.msgraph.client.enums.ActivityType;
import odata.msgraph.client.enums.RiskDetail;
import odata.msgraph.client.enums.RiskDetectionTimingType;
import odata.msgraph.client.enums.RiskLevel;
import odata.msgraph.client.enums.RiskState;
import odata.msgraph.client.enums.TokenIssuerType;
/**
*
* Org.OData.Capabilities.V1.CountRestrictions
*
* Countable = false
*
* Org.OData.Capabilities.V1.FilterRestrictions
*
* Filterable = true
*
* Org.OData.Capabilities.V1.SelectRestrictions
*
* Selectable = true
*
* Org.OData.Capabilities.V1.SkipSupported
*
* false
*
* Org.OData.Capabilities.V1.TopSupported
*
* true
*/@JsonPropertyOrder({
"@odata.type",
"activity",
"activityDateTime",
"additionalInfo",
"appId",
"correlationId",
"detectedDateTime",
"detectionTimingType",
"ipAddress",
"keyIds",
"lastUpdatedDateTime",
"location",
"requestId",
"riskDetail",
"riskEventType",
"riskLevel",
"riskState",
"servicePrincipalDisplayName",
"servicePrincipalId",
"source",
"tokenIssuerType"})
@JsonInclude(Include.NON_NULL)
public class ServicePrincipalRiskDetection extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.servicePrincipalRiskDetection";
}
@JsonProperty("activity")
protected ActivityType activity;
@JsonProperty("activityDateTime")
protected OffsetDateTime activityDateTime;
@JsonProperty("additionalInfo")
protected String additionalInfo;
@JsonProperty("appId")
protected String appId;
@JsonProperty("correlationId")
protected String correlationId;
@JsonProperty("detectedDateTime")
protected OffsetDateTime detectedDateTime;
@JsonProperty("detectionTimingType")
protected RiskDetectionTimingType detectionTimingType;
@JsonProperty("ipAddress")
protected String ipAddress;
@JsonProperty("keyIds")
protected List keyIds;
@JsonProperty("keyIds@nextLink")
protected String keyIdsNextLink;
@JsonProperty("lastUpdatedDateTime")
protected OffsetDateTime lastUpdatedDateTime;
@JsonProperty("location")
protected SignInLocation location;
@JsonProperty("requestId")
protected String requestId;
@JsonProperty("riskDetail")
protected RiskDetail riskDetail;
@JsonProperty("riskEventType")
protected String riskEventType;
@JsonProperty("riskLevel")
protected RiskLevel riskLevel;
@JsonProperty("riskState")
protected RiskState riskState;
@JsonProperty("servicePrincipalDisplayName")
protected String servicePrincipalDisplayName;
@JsonProperty("servicePrincipalId")
protected String servicePrincipalId;
@JsonProperty("source")
protected String source;
@JsonProperty("tokenIssuerType")
protected TokenIssuerType tokenIssuerType;
protected ServicePrincipalRiskDetection() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderServicePrincipalRiskDetection() {
return new Builder();
}
public static final class Builder {
private String id;
private ActivityType activity;
private OffsetDateTime activityDateTime;
private String additionalInfo;
private String appId;
private String correlationId;
private OffsetDateTime detectedDateTime;
private RiskDetectionTimingType detectionTimingType;
private String ipAddress;
private List keyIds;
private String keyIdsNextLink;
private OffsetDateTime lastUpdatedDateTime;
private SignInLocation location;
private String requestId;
private RiskDetail riskDetail;
private String riskEventType;
private RiskLevel riskLevel;
private RiskState riskState;
private String servicePrincipalDisplayName;
private String servicePrincipalId;
private String source;
private TokenIssuerType tokenIssuerType;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder activity(ActivityType activity) {
this.activity = activity;
this.changedFields = changedFields.add("activity");
return this;
}
public Builder activityDateTime(OffsetDateTime activityDateTime) {
this.activityDateTime = activityDateTime;
this.changedFields = changedFields.add("activityDateTime");
return this;
}
public Builder additionalInfo(String additionalInfo) {
this.additionalInfo = additionalInfo;
this.changedFields = changedFields.add("additionalInfo");
return this;
}
public Builder appId(String appId) {
this.appId = appId;
this.changedFields = changedFields.add("appId");
return this;
}
public Builder correlationId(String correlationId) {
this.correlationId = correlationId;
this.changedFields = changedFields.add("correlationId");
return this;
}
public Builder detectedDateTime(OffsetDateTime detectedDateTime) {
this.detectedDateTime = detectedDateTime;
this.changedFields = changedFields.add("detectedDateTime");
return this;
}
public Builder detectionTimingType(RiskDetectionTimingType detectionTimingType) {
this.detectionTimingType = detectionTimingType;
this.changedFields = changedFields.add("detectionTimingType");
return this;
}
public Builder ipAddress(String ipAddress) {
this.ipAddress = ipAddress;
this.changedFields = changedFields.add("ipAddress");
return this;
}
public Builder keyIds(List keyIds) {
this.keyIds = keyIds;
this.changedFields = changedFields.add("keyIds");
return this;
}
public Builder keyIds(String... keyIds) {
return keyIds(Arrays.asList(keyIds));
}
public Builder keyIdsNextLink(String keyIdsNextLink) {
this.keyIdsNextLink = keyIdsNextLink;
this.changedFields = changedFields.add("keyIds");
return this;
}
public Builder lastUpdatedDateTime(OffsetDateTime lastUpdatedDateTime) {
this.lastUpdatedDateTime = lastUpdatedDateTime;
this.changedFields = changedFields.add("lastUpdatedDateTime");
return this;
}
public Builder location(SignInLocation location) {
this.location = location;
this.changedFields = changedFields.add("location");
return this;
}
public Builder requestId(String requestId) {
this.requestId = requestId;
this.changedFields = changedFields.add("requestId");
return this;
}
public Builder riskDetail(RiskDetail riskDetail) {
this.riskDetail = riskDetail;
this.changedFields = changedFields.add("riskDetail");
return this;
}
public Builder riskEventType(String riskEventType) {
this.riskEventType = riskEventType;
this.changedFields = changedFields.add("riskEventType");
return this;
}
public Builder riskLevel(RiskLevel riskLevel) {
this.riskLevel = riskLevel;
this.changedFields = changedFields.add("riskLevel");
return this;
}
public Builder riskState(RiskState riskState) {
this.riskState = riskState;
this.changedFields = changedFields.add("riskState");
return this;
}
public Builder servicePrincipalDisplayName(String servicePrincipalDisplayName) {
this.servicePrincipalDisplayName = servicePrincipalDisplayName;
this.changedFields = changedFields.add("servicePrincipalDisplayName");
return this;
}
public Builder servicePrincipalId(String servicePrincipalId) {
this.servicePrincipalId = servicePrincipalId;
this.changedFields = changedFields.add("servicePrincipalId");
return this;
}
public Builder source(String source) {
this.source = source;
this.changedFields = changedFields.add("source");
return this;
}
public Builder tokenIssuerType(TokenIssuerType tokenIssuerType) {
this.tokenIssuerType = tokenIssuerType;
this.changedFields = changedFields.add("tokenIssuerType");
return this;
}
public ServicePrincipalRiskDetection build() {
ServicePrincipalRiskDetection _x = new ServicePrincipalRiskDetection();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.servicePrincipalRiskDetection";
_x.id = id;
_x.activity = activity;
_x.activityDateTime = activityDateTime;
_x.additionalInfo = additionalInfo;
_x.appId = appId;
_x.correlationId = correlationId;
_x.detectedDateTime = detectedDateTime;
_x.detectionTimingType = detectionTimingType;
_x.ipAddress = ipAddress;
_x.keyIds = keyIds;
_x.keyIdsNextLink = keyIdsNextLink;
_x.lastUpdatedDateTime = lastUpdatedDateTime;
_x.location = location;
_x.requestId = requestId;
_x.riskDetail = riskDetail;
_x.riskEventType = riskEventType;
_x.riskLevel = riskLevel;
_x.riskState = riskState;
_x.servicePrincipalDisplayName = servicePrincipalDisplayName;
_x.servicePrincipalId = servicePrincipalId;
_x.source = source;
_x.tokenIssuerType = tokenIssuerType;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
@Property(name="activity")
@JsonIgnore
public Optional getActivity() {
return Optional.ofNullable(activity);
}
public ServicePrincipalRiskDetection withActivity(ActivityType activity) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("activity");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.activity = activity;
return _x;
}
@Property(name="activityDateTime")
@JsonIgnore
public Optional getActivityDateTime() {
return Optional.ofNullable(activityDateTime);
}
public ServicePrincipalRiskDetection withActivityDateTime(OffsetDateTime activityDateTime) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("activityDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.activityDateTime = activityDateTime;
return _x;
}
@Property(name="additionalInfo")
@JsonIgnore
public Optional getAdditionalInfo() {
return Optional.ofNullable(additionalInfo);
}
public ServicePrincipalRiskDetection withAdditionalInfo(String additionalInfo) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("additionalInfo");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.additionalInfo = additionalInfo;
return _x;
}
@Property(name="appId")
@JsonIgnore
public Optional getAppId() {
return Optional.ofNullable(appId);
}
public ServicePrincipalRiskDetection withAppId(String appId) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("appId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.appId = appId;
return _x;
}
@Property(name="correlationId")
@JsonIgnore
public Optional getCorrelationId() {
return Optional.ofNullable(correlationId);
}
public ServicePrincipalRiskDetection withCorrelationId(String correlationId) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("correlationId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.correlationId = correlationId;
return _x;
}
@Property(name="detectedDateTime")
@JsonIgnore
public Optional getDetectedDateTime() {
return Optional.ofNullable(detectedDateTime);
}
public ServicePrincipalRiskDetection withDetectedDateTime(OffsetDateTime detectedDateTime) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("detectedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.detectedDateTime = detectedDateTime;
return _x;
}
@Property(name="detectionTimingType")
@JsonIgnore
public Optional getDetectionTimingType() {
return Optional.ofNullable(detectionTimingType);
}
public ServicePrincipalRiskDetection withDetectionTimingType(RiskDetectionTimingType detectionTimingType) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("detectionTimingType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.detectionTimingType = detectionTimingType;
return _x;
}
@Property(name="ipAddress")
@JsonIgnore
public Optional getIpAddress() {
return Optional.ofNullable(ipAddress);
}
public ServicePrincipalRiskDetection withIpAddress(String ipAddress) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("ipAddress");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.ipAddress = ipAddress;
return _x;
}
@Property(name="keyIds")
@JsonIgnore
public CollectionPage getKeyIds() {
return new CollectionPage(contextPath, String.class, this.keyIds, Optional.ofNullable(keyIdsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public ServicePrincipalRiskDetection withKeyIds(List keyIds) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("keyIds");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.keyIds = keyIds;
return _x;
}
@Property(name="keyIds")
@JsonIgnore
public CollectionPage getKeyIds(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.keyIds, Optional.ofNullable(keyIdsNextLink), Collections.emptyList(), options);
}
@Property(name="lastUpdatedDateTime")
@JsonIgnore
public Optional getLastUpdatedDateTime() {
return Optional.ofNullable(lastUpdatedDateTime);
}
public ServicePrincipalRiskDetection withLastUpdatedDateTime(OffsetDateTime lastUpdatedDateTime) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("lastUpdatedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.lastUpdatedDateTime = lastUpdatedDateTime;
return _x;
}
@Property(name="location")
@JsonIgnore
public Optional getLocation() {
return Optional.ofNullable(location);
}
public ServicePrincipalRiskDetection withLocation(SignInLocation location) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("location");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.location = location;
return _x;
}
@Property(name="requestId")
@JsonIgnore
public Optional getRequestId() {
return Optional.ofNullable(requestId);
}
public ServicePrincipalRiskDetection withRequestId(String requestId) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("requestId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.requestId = requestId;
return _x;
}
@Property(name="riskDetail")
@JsonIgnore
public Optional getRiskDetail() {
return Optional.ofNullable(riskDetail);
}
public ServicePrincipalRiskDetection withRiskDetail(RiskDetail riskDetail) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("riskDetail");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.riskDetail = riskDetail;
return _x;
}
@Property(name="riskEventType")
@JsonIgnore
public Optional getRiskEventType() {
return Optional.ofNullable(riskEventType);
}
public ServicePrincipalRiskDetection withRiskEventType(String riskEventType) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("riskEventType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.riskEventType = riskEventType;
return _x;
}
@Property(name="riskLevel")
@JsonIgnore
public Optional getRiskLevel() {
return Optional.ofNullable(riskLevel);
}
public ServicePrincipalRiskDetection withRiskLevel(RiskLevel riskLevel) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("riskLevel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.riskLevel = riskLevel;
return _x;
}
@Property(name="riskState")
@JsonIgnore
public Optional getRiskState() {
return Optional.ofNullable(riskState);
}
public ServicePrincipalRiskDetection withRiskState(RiskState riskState) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("riskState");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.riskState = riskState;
return _x;
}
@Property(name="servicePrincipalDisplayName")
@JsonIgnore
public Optional getServicePrincipalDisplayName() {
return Optional.ofNullable(servicePrincipalDisplayName);
}
public ServicePrincipalRiskDetection withServicePrincipalDisplayName(String servicePrincipalDisplayName) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("servicePrincipalDisplayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.servicePrincipalDisplayName = servicePrincipalDisplayName;
return _x;
}
@Property(name="servicePrincipalId")
@JsonIgnore
public Optional getServicePrincipalId() {
return Optional.ofNullable(servicePrincipalId);
}
public ServicePrincipalRiskDetection withServicePrincipalId(String servicePrincipalId) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("servicePrincipalId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.servicePrincipalId = servicePrincipalId;
return _x;
}
@Property(name="source")
@JsonIgnore
public Optional getSource() {
return Optional.ofNullable(source);
}
public ServicePrincipalRiskDetection withSource(String source) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("source");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.source = source;
return _x;
}
@Property(name="tokenIssuerType")
@JsonIgnore
public Optional getTokenIssuerType() {
return Optional.ofNullable(tokenIssuerType);
}
public ServicePrincipalRiskDetection withTokenIssuerType(TokenIssuerType tokenIssuerType) {
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = changedFields.add("tokenIssuerType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.servicePrincipalRiskDetection");
_x.tokenIssuerType = tokenIssuerType;
return _x;
}
public ServicePrincipalRiskDetection withUnmappedField(String name, Object value) {
ServicePrincipalRiskDetection _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ServicePrincipalRiskDetection patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ServicePrincipalRiskDetection put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
ServicePrincipalRiskDetection _x = _copy();
_x.changedFields = null;
return _x;
}
private ServicePrincipalRiskDetection _copy() {
ServicePrincipalRiskDetection _x = new ServicePrincipalRiskDetection();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.activity = activity;
_x.activityDateTime = activityDateTime;
_x.additionalInfo = additionalInfo;
_x.appId = appId;
_x.correlationId = correlationId;
_x.detectedDateTime = detectedDateTime;
_x.detectionTimingType = detectionTimingType;
_x.ipAddress = ipAddress;
_x.keyIds = keyIds;
_x.lastUpdatedDateTime = lastUpdatedDateTime;
_x.location = location;
_x.requestId = requestId;
_x.riskDetail = riskDetail;
_x.riskEventType = riskEventType;
_x.riskLevel = riskLevel;
_x.riskState = riskState;
_x.servicePrincipalDisplayName = servicePrincipalDisplayName;
_x.servicePrincipalId = servicePrincipalId;
_x.source = source;
_x.tokenIssuerType = tokenIssuerType;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ServicePrincipalRiskDetection[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("activity=");
b.append(this.activity);
b.append(", ");
b.append("activityDateTime=");
b.append(this.activityDateTime);
b.append(", ");
b.append("additionalInfo=");
b.append(this.additionalInfo);
b.append(", ");
b.append("appId=");
b.append(this.appId);
b.append(", ");
b.append("correlationId=");
b.append(this.correlationId);
b.append(", ");
b.append("detectedDateTime=");
b.append(this.detectedDateTime);
b.append(", ");
b.append("detectionTimingType=");
b.append(this.detectionTimingType);
b.append(", ");
b.append("ipAddress=");
b.append(this.ipAddress);
b.append(", ");
b.append("keyIds=");
b.append(this.keyIds);
b.append(", ");
b.append("lastUpdatedDateTime=");
b.append(this.lastUpdatedDateTime);
b.append(", ");
b.append("location=");
b.append(this.location);
b.append(", ");
b.append("requestId=");
b.append(this.requestId);
b.append(", ");
b.append("riskDetail=");
b.append(this.riskDetail);
b.append(", ");
b.append("riskEventType=");
b.append(this.riskEventType);
b.append(", ");
b.append("riskLevel=");
b.append(this.riskLevel);
b.append(", ");
b.append("riskState=");
b.append(this.riskState);
b.append(", ");
b.append("servicePrincipalDisplayName=");
b.append(this.servicePrincipalDisplayName);
b.append(", ");
b.append("servicePrincipalId=");
b.append(this.servicePrincipalId);
b.append(", ");
b.append("source=");
b.append(this.source);
b.append(", ");
b.append("tokenIssuerType=");
b.append(this.tokenIssuerType);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}