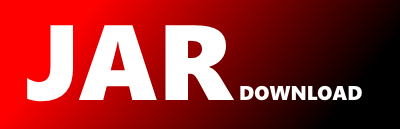
odata.msgraph.client.entity.UserExperienceAnalyticsDevicePerformance Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Double;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
import odata.msgraph.client.enums.DiskType;
import odata.msgraph.client.enums.UserExperienceAnalyticsHealthState;
/**
* “The user experience analytics device performance entity contains device boot
* performance details.”
*/@JsonPropertyOrder({
"@odata.type",
"averageBlueScreens",
"averageRestarts",
"blueScreenCount",
"bootScore",
"coreBootTimeInMs",
"coreLoginTimeInMs",
"deviceCount",
"deviceName",
"diskType",
"groupPolicyBootTimeInMs",
"groupPolicyLoginTimeInMs",
"healthStatus",
"loginScore",
"manufacturer",
"model",
"modelStartupPerformanceScore",
"operatingSystemVersion",
"responsiveDesktopTimeInMs",
"restartCount",
"startupPerformanceScore"})
@JsonInclude(Include.NON_NULL)
public class UserExperienceAnalyticsDevicePerformance extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.userExperienceAnalyticsDevicePerformance";
}
@JsonProperty("averageBlueScreens")
protected Double averageBlueScreens;
@JsonProperty("averageRestarts")
protected Double averageRestarts;
@JsonProperty("blueScreenCount")
protected Integer blueScreenCount;
@JsonProperty("bootScore")
protected Integer bootScore;
@JsonProperty("coreBootTimeInMs")
protected Integer coreBootTimeInMs;
@JsonProperty("coreLoginTimeInMs")
protected Integer coreLoginTimeInMs;
@JsonProperty("deviceCount")
protected Long deviceCount;
@JsonProperty("deviceName")
protected String deviceName;
@JsonProperty("diskType")
protected DiskType diskType;
@JsonProperty("groupPolicyBootTimeInMs")
protected Integer groupPolicyBootTimeInMs;
@JsonProperty("groupPolicyLoginTimeInMs")
protected Integer groupPolicyLoginTimeInMs;
@JsonProperty("healthStatus")
protected UserExperienceAnalyticsHealthState healthStatus;
@JsonProperty("loginScore")
protected Integer loginScore;
@JsonProperty("manufacturer")
protected String manufacturer;
@JsonProperty("model")
protected String model;
@JsonProperty("modelStartupPerformanceScore")
protected Double modelStartupPerformanceScore;
@JsonProperty("operatingSystemVersion")
protected String operatingSystemVersion;
@JsonProperty("responsiveDesktopTimeInMs")
protected Integer responsiveDesktopTimeInMs;
@JsonProperty("restartCount")
protected Integer restartCount;
@JsonProperty("startupPerformanceScore")
protected Double startupPerformanceScore;
protected UserExperienceAnalyticsDevicePerformance() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderUserExperienceAnalyticsDevicePerformance() {
return new Builder();
}
public static final class Builder {
private String id;
private Double averageBlueScreens;
private Double averageRestarts;
private Integer blueScreenCount;
private Integer bootScore;
private Integer coreBootTimeInMs;
private Integer coreLoginTimeInMs;
private Long deviceCount;
private String deviceName;
private DiskType diskType;
private Integer groupPolicyBootTimeInMs;
private Integer groupPolicyLoginTimeInMs;
private UserExperienceAnalyticsHealthState healthStatus;
private Integer loginScore;
private String manufacturer;
private String model;
private Double modelStartupPerformanceScore;
private String operatingSystemVersion;
private Integer responsiveDesktopTimeInMs;
private Integer restartCount;
private Double startupPerformanceScore;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
/**
* “Average (mean) number of Blue Screens per device in the last 30 days. Valid
* values 0 to 9999999”
*
* @param averageBlueScreens
* value of {@code averageBlueScreens} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder averageBlueScreens(Double averageBlueScreens) {
this.averageBlueScreens = averageBlueScreens;
this.changedFields = changedFields.add("averageBlueScreens");
return this;
}
/**
* “Average (mean) number of Restarts per device in the last 30 days. Valid values 0
* to 9999999”
*
* @param averageRestarts
* value of {@code averageRestarts} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder averageRestarts(Double averageRestarts) {
this.averageRestarts = averageRestarts;
this.changedFields = changedFields.add("averageRestarts");
return this;
}
/**
* “Number of Blue Screens in the last 30 days. Valid values 0 to 9999999”
*
* @param blueScreenCount
* value of {@code blueScreenCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder blueScreenCount(Integer blueScreenCount) {
this.blueScreenCount = blueScreenCount;
this.changedFields = changedFields.add("blueScreenCount");
return this;
}
/**
* “The user experience analytics device boot score.”
*
* @param bootScore
* value of {@code bootScore} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder bootScore(Integer bootScore) {
this.bootScore = bootScore;
this.changedFields = changedFields.add("bootScore");
return this;
}
/**
* “The user experience analytics device core boot time in milliseconds.”
*
* @param coreBootTimeInMs
* value of {@code coreBootTimeInMs} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder coreBootTimeInMs(Integer coreBootTimeInMs) {
this.coreBootTimeInMs = coreBootTimeInMs;
this.changedFields = changedFields.add("coreBootTimeInMs");
return this;
}
/**
* “The user experience analytics device core login time in milliseconds.”
*
* @param coreLoginTimeInMs
* value of {@code coreLoginTimeInMs} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder coreLoginTimeInMs(Integer coreLoginTimeInMs) {
this.coreLoginTimeInMs = coreLoginTimeInMs;
this.changedFields = changedFields.add("coreLoginTimeInMs");
return this;
}
/**
* “User experience analytics summarized device count.”
*
* @param deviceCount
* value of {@code deviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceCount(Long deviceCount) {
this.deviceCount = deviceCount;
this.changedFields = changedFields.add("deviceCount");
return this;
}
/**
* “The user experience analytics device name.”
*
* @param deviceName
* value of {@code deviceName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceName(String deviceName) {
this.deviceName = deviceName;
this.changedFields = changedFields.add("deviceName");
return this;
}
/**
* “The user experience analytics device disk type.”
*
* @param diskType
* value of {@code diskType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder diskType(DiskType diskType) {
this.diskType = diskType;
this.changedFields = changedFields.add("diskType");
return this;
}
/**
* “The user experience analytics device group policy boot time in milliseconds.”
*
* @param groupPolicyBootTimeInMs
* value of {@code groupPolicyBootTimeInMs} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder groupPolicyBootTimeInMs(Integer groupPolicyBootTimeInMs) {
this.groupPolicyBootTimeInMs = groupPolicyBootTimeInMs;
this.changedFields = changedFields.add("groupPolicyBootTimeInMs");
return this;
}
/**
* “The user experience analytics device group policy login time in milliseconds.”
*
* @param groupPolicyLoginTimeInMs
* value of {@code groupPolicyLoginTimeInMs} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder groupPolicyLoginTimeInMs(Integer groupPolicyLoginTimeInMs) {
this.groupPolicyLoginTimeInMs = groupPolicyLoginTimeInMs;
this.changedFields = changedFields.add("groupPolicyLoginTimeInMs");
return this;
}
/**
* “The health state of the user experience analytics device.”
*
* @param healthStatus
* value of {@code healthStatus} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder healthStatus(UserExperienceAnalyticsHealthState healthStatus) {
this.healthStatus = healthStatus;
this.changedFields = changedFields.add("healthStatus");
return this;
}
/**
* “The user experience analytics device login score.”
*
* @param loginScore
* value of {@code loginScore} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder loginScore(Integer loginScore) {
this.loginScore = loginScore;
this.changedFields = changedFields.add("loginScore");
return this;
}
/**
* “The user experience analytics device manufacturer.”
*
* @param manufacturer
* value of {@code manufacturer} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder manufacturer(String manufacturer) {
this.manufacturer = manufacturer;
this.changedFields = changedFields.add("manufacturer");
return this;
}
/**
* “The user experience analytics device model.”
*
* @param model
* value of {@code model} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder model(String model) {
this.model = model;
this.changedFields = changedFields.add("model");
return this;
}
/**
* “The user experience analytics model level startup performance score. Valid
* values -1.79769313486232E+308 to 1.79769313486232E+308”
*
* @param modelStartupPerformanceScore
* value of {@code modelStartupPerformanceScore} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder modelStartupPerformanceScore(Double modelStartupPerformanceScore) {
this.modelStartupPerformanceScore = modelStartupPerformanceScore;
this.changedFields = changedFields.add("modelStartupPerformanceScore");
return this;
}
/**
* “The user experience analytics device Operating System version.”
*
* @param operatingSystemVersion
* value of {@code operatingSystemVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder operatingSystemVersion(String operatingSystemVersion) {
this.operatingSystemVersion = operatingSystemVersion;
this.changedFields = changedFields.add("operatingSystemVersion");
return this;
}
/**
* “The user experience analytics responsive desktop time in milliseconds.”
*
* @param responsiveDesktopTimeInMs
* value of {@code responsiveDesktopTimeInMs} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder responsiveDesktopTimeInMs(Integer responsiveDesktopTimeInMs) {
this.responsiveDesktopTimeInMs = responsiveDesktopTimeInMs;
this.changedFields = changedFields.add("responsiveDesktopTimeInMs");
return this;
}
/**
* “Number of Restarts in the last 30 days. Valid values 0 to 9999999”
*
* @param restartCount
* value of {@code restartCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder restartCount(Integer restartCount) {
this.restartCount = restartCount;
this.changedFields = changedFields.add("restartCount");
return this;
}
/**
* “The user experience analytics device startup performance score. Valid values -1.
* 79769313486232E+308 to 1.79769313486232E+308”
*
* @param startupPerformanceScore
* value of {@code startupPerformanceScore} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder startupPerformanceScore(Double startupPerformanceScore) {
this.startupPerformanceScore = startupPerformanceScore;
this.changedFields = changedFields.add("startupPerformanceScore");
return this;
}
public UserExperienceAnalyticsDevicePerformance build() {
UserExperienceAnalyticsDevicePerformance _x = new UserExperienceAnalyticsDevicePerformance();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.userExperienceAnalyticsDevicePerformance";
_x.id = id;
_x.averageBlueScreens = averageBlueScreens;
_x.averageRestarts = averageRestarts;
_x.blueScreenCount = blueScreenCount;
_x.bootScore = bootScore;
_x.coreBootTimeInMs = coreBootTimeInMs;
_x.coreLoginTimeInMs = coreLoginTimeInMs;
_x.deviceCount = deviceCount;
_x.deviceName = deviceName;
_x.diskType = diskType;
_x.groupPolicyBootTimeInMs = groupPolicyBootTimeInMs;
_x.groupPolicyLoginTimeInMs = groupPolicyLoginTimeInMs;
_x.healthStatus = healthStatus;
_x.loginScore = loginScore;
_x.manufacturer = manufacturer;
_x.model = model;
_x.modelStartupPerformanceScore = modelStartupPerformanceScore;
_x.operatingSystemVersion = operatingSystemVersion;
_x.responsiveDesktopTimeInMs = responsiveDesktopTimeInMs;
_x.restartCount = restartCount;
_x.startupPerformanceScore = startupPerformanceScore;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “Average (mean) number of Blue Screens per device in the last 30 days. Valid
* values 0 to 9999999”
*
* @return property averageBlueScreens
*/
@Property(name="averageBlueScreens")
@JsonIgnore
public Optional getAverageBlueScreens() {
return Optional.ofNullable(averageBlueScreens);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* averageBlueScreens} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Average (mean) number of Blue Screens per device in the last 30 days. Valid
* values 0 to 9999999”
*
* @param averageBlueScreens
* new value of {@code averageBlueScreens} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code averageBlueScreens} field changed
*/
public UserExperienceAnalyticsDevicePerformance withAverageBlueScreens(Double averageBlueScreens) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("averageBlueScreens");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.averageBlueScreens = averageBlueScreens;
return _x;
}
/**
* “Average (mean) number of Restarts per device in the last 30 days. Valid values 0
* to 9999999”
*
* @return property averageRestarts
*/
@Property(name="averageRestarts")
@JsonIgnore
public Optional getAverageRestarts() {
return Optional.ofNullable(averageRestarts);
}
/**
* Returns an immutable copy of {@code this} with just the {@code averageRestarts}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Average (mean) number of Restarts per device in the last 30 days. Valid values 0
* to 9999999”
*
* @param averageRestarts
* new value of {@code averageRestarts} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code averageRestarts} field changed
*/
public UserExperienceAnalyticsDevicePerformance withAverageRestarts(Double averageRestarts) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("averageRestarts");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.averageRestarts = averageRestarts;
return _x;
}
/**
* “Number of Blue Screens in the last 30 days. Valid values 0 to 9999999”
*
* @return property blueScreenCount
*/
@Property(name="blueScreenCount")
@JsonIgnore
public Optional getBlueScreenCount() {
return Optional.ofNullable(blueScreenCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code blueScreenCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Number of Blue Screens in the last 30 days. Valid values 0 to 9999999”
*
* @param blueScreenCount
* new value of {@code blueScreenCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code blueScreenCount} field changed
*/
public UserExperienceAnalyticsDevicePerformance withBlueScreenCount(Integer blueScreenCount) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("blueScreenCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.blueScreenCount = blueScreenCount;
return _x;
}
/**
* “The user experience analytics device boot score.”
*
* @return property bootScore
*/
@Property(name="bootScore")
@JsonIgnore
public Optional getBootScore() {
return Optional.ofNullable(bootScore);
}
/**
* Returns an immutable copy of {@code this} with just the {@code bootScore} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The user experience analytics device boot score.”
*
* @param bootScore
* new value of {@code bootScore} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code bootScore} field changed
*/
public UserExperienceAnalyticsDevicePerformance withBootScore(Integer bootScore) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("bootScore");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.bootScore = bootScore;
return _x;
}
/**
* “The user experience analytics device core boot time in milliseconds.”
*
* @return property coreBootTimeInMs
*/
@Property(name="coreBootTimeInMs")
@JsonIgnore
public Optional getCoreBootTimeInMs() {
return Optional.ofNullable(coreBootTimeInMs);
}
/**
* Returns an immutable copy of {@code this} with just the {@code coreBootTimeInMs}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The user experience analytics device core boot time in milliseconds.”
*
* @param coreBootTimeInMs
* new value of {@code coreBootTimeInMs} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code coreBootTimeInMs} field changed
*/
public UserExperienceAnalyticsDevicePerformance withCoreBootTimeInMs(Integer coreBootTimeInMs) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("coreBootTimeInMs");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.coreBootTimeInMs = coreBootTimeInMs;
return _x;
}
/**
* “The user experience analytics device core login time in milliseconds.”
*
* @return property coreLoginTimeInMs
*/
@Property(name="coreLoginTimeInMs")
@JsonIgnore
public Optional getCoreLoginTimeInMs() {
return Optional.ofNullable(coreLoginTimeInMs);
}
/**
* Returns an immutable copy of {@code this} with just the {@code coreLoginTimeInMs
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The user experience analytics device core login time in milliseconds.”
*
* @param coreLoginTimeInMs
* new value of {@code coreLoginTimeInMs} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code coreLoginTimeInMs} field changed
*/
public UserExperienceAnalyticsDevicePerformance withCoreLoginTimeInMs(Integer coreLoginTimeInMs) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("coreLoginTimeInMs");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.coreLoginTimeInMs = coreLoginTimeInMs;
return _x;
}
/**
* “User experience analytics summarized device count.”
*
* @return property deviceCount
*/
@Property(name="deviceCount")
@JsonIgnore
public Optional getDeviceCount() {
return Optional.ofNullable(deviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “User experience analytics summarized device count.”
*
* @param deviceCount
* new value of {@code deviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceCount} field changed
*/
public UserExperienceAnalyticsDevicePerformance withDeviceCount(Long deviceCount) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("deviceCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.deviceCount = deviceCount;
return _x;
}
/**
* “The user experience analytics device name.”
*
* @return property deviceName
*/
@Property(name="deviceName")
@JsonIgnore
public Optional getDeviceName() {
return Optional.ofNullable(deviceName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceName} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The user experience analytics device name.”
*
* @param deviceName
* new value of {@code deviceName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceName} field changed
*/
public UserExperienceAnalyticsDevicePerformance withDeviceName(String deviceName) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("deviceName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.deviceName = deviceName;
return _x;
}
/**
* “The user experience analytics device disk type.”
*
* @return property diskType
*/
@Property(name="diskType")
@JsonIgnore
public Optional getDiskType() {
return Optional.ofNullable(diskType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code diskType} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The user experience analytics device disk type.”
*
* @param diskType
* new value of {@code diskType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code diskType} field changed
*/
public UserExperienceAnalyticsDevicePerformance withDiskType(DiskType diskType) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("diskType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.diskType = diskType;
return _x;
}
/**
* “The user experience analytics device group policy boot time in milliseconds.”
*
* @return property groupPolicyBootTimeInMs
*/
@Property(name="groupPolicyBootTimeInMs")
@JsonIgnore
public Optional getGroupPolicyBootTimeInMs() {
return Optional.ofNullable(groupPolicyBootTimeInMs);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* groupPolicyBootTimeInMs} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The user experience analytics device group policy boot time in milliseconds.”
*
* @param groupPolicyBootTimeInMs
* new value of {@code groupPolicyBootTimeInMs} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code groupPolicyBootTimeInMs} field changed
*/
public UserExperienceAnalyticsDevicePerformance withGroupPolicyBootTimeInMs(Integer groupPolicyBootTimeInMs) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("groupPolicyBootTimeInMs");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.groupPolicyBootTimeInMs = groupPolicyBootTimeInMs;
return _x;
}
/**
* “The user experience analytics device group policy login time in milliseconds.”
*
* @return property groupPolicyLoginTimeInMs
*/
@Property(name="groupPolicyLoginTimeInMs")
@JsonIgnore
public Optional getGroupPolicyLoginTimeInMs() {
return Optional.ofNullable(groupPolicyLoginTimeInMs);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* groupPolicyLoginTimeInMs} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The user experience analytics device group policy login time in milliseconds.”
*
* @param groupPolicyLoginTimeInMs
* new value of {@code groupPolicyLoginTimeInMs} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code groupPolicyLoginTimeInMs} field changed
*/
public UserExperienceAnalyticsDevicePerformance withGroupPolicyLoginTimeInMs(Integer groupPolicyLoginTimeInMs) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("groupPolicyLoginTimeInMs");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.groupPolicyLoginTimeInMs = groupPolicyLoginTimeInMs;
return _x;
}
/**
* “The health state of the user experience analytics device.”
*
* @return property healthStatus
*/
@Property(name="healthStatus")
@JsonIgnore
public Optional getHealthStatus() {
return Optional.ofNullable(healthStatus);
}
/**
* Returns an immutable copy of {@code this} with just the {@code healthStatus}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The health state of the user experience analytics device.”
*
* @param healthStatus
* new value of {@code healthStatus} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code healthStatus} field changed
*/
public UserExperienceAnalyticsDevicePerformance withHealthStatus(UserExperienceAnalyticsHealthState healthStatus) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("healthStatus");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.healthStatus = healthStatus;
return _x;
}
/**
* “The user experience analytics device login score.”
*
* @return property loginScore
*/
@Property(name="loginScore")
@JsonIgnore
public Optional getLoginScore() {
return Optional.ofNullable(loginScore);
}
/**
* Returns an immutable copy of {@code this} with just the {@code loginScore} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The user experience analytics device login score.”
*
* @param loginScore
* new value of {@code loginScore} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code loginScore} field changed
*/
public UserExperienceAnalyticsDevicePerformance withLoginScore(Integer loginScore) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("loginScore");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.loginScore = loginScore;
return _x;
}
/**
* “The user experience analytics device manufacturer.”
*
* @return property manufacturer
*/
@Property(name="manufacturer")
@JsonIgnore
public Optional getManufacturer() {
return Optional.ofNullable(manufacturer);
}
/**
* Returns an immutable copy of {@code this} with just the {@code manufacturer}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The user experience analytics device manufacturer.”
*
* @param manufacturer
* new value of {@code manufacturer} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code manufacturer} field changed
*/
public UserExperienceAnalyticsDevicePerformance withManufacturer(String manufacturer) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("manufacturer");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.manufacturer = manufacturer;
return _x;
}
/**
* “The user experience analytics device model.”
*
* @return property model
*/
@Property(name="model")
@JsonIgnore
public Optional getModel() {
return Optional.ofNullable(model);
}
/**
* Returns an immutable copy of {@code this} with just the {@code model} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The user experience analytics device model.”
*
* @param model
* new value of {@code model} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code model} field changed
*/
public UserExperienceAnalyticsDevicePerformance withModel(String model) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("model");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.model = model;
return _x;
}
/**
* “The user experience analytics model level startup performance score. Valid
* values -1.79769313486232E+308 to 1.79769313486232E+308”
*
* @return property modelStartupPerformanceScore
*/
@Property(name="modelStartupPerformanceScore")
@JsonIgnore
public Optional getModelStartupPerformanceScore() {
return Optional.ofNullable(modelStartupPerformanceScore);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* modelStartupPerformanceScore} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The user experience analytics model level startup performance score. Valid
* values -1.79769313486232E+308 to 1.79769313486232E+308”
*
* @param modelStartupPerformanceScore
* new value of {@code modelStartupPerformanceScore} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code modelStartupPerformanceScore} field changed
*/
public UserExperienceAnalyticsDevicePerformance withModelStartupPerformanceScore(Double modelStartupPerformanceScore) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("modelStartupPerformanceScore");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.modelStartupPerformanceScore = modelStartupPerformanceScore;
return _x;
}
/**
* “The user experience analytics device Operating System version.”
*
* @return property operatingSystemVersion
*/
@Property(name="operatingSystemVersion")
@JsonIgnore
public Optional getOperatingSystemVersion() {
return Optional.ofNullable(operatingSystemVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* operatingSystemVersion} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The user experience analytics device Operating System version.”
*
* @param operatingSystemVersion
* new value of {@code operatingSystemVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code operatingSystemVersion} field changed
*/
public UserExperienceAnalyticsDevicePerformance withOperatingSystemVersion(String operatingSystemVersion) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("operatingSystemVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.operatingSystemVersion = operatingSystemVersion;
return _x;
}
/**
* “The user experience analytics responsive desktop time in milliseconds.”
*
* @return property responsiveDesktopTimeInMs
*/
@Property(name="responsiveDesktopTimeInMs")
@JsonIgnore
public Optional getResponsiveDesktopTimeInMs() {
return Optional.ofNullable(responsiveDesktopTimeInMs);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* responsiveDesktopTimeInMs} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The user experience analytics responsive desktop time in milliseconds.”
*
* @param responsiveDesktopTimeInMs
* new value of {@code responsiveDesktopTimeInMs} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code responsiveDesktopTimeInMs} field changed
*/
public UserExperienceAnalyticsDevicePerformance withResponsiveDesktopTimeInMs(Integer responsiveDesktopTimeInMs) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("responsiveDesktopTimeInMs");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.responsiveDesktopTimeInMs = responsiveDesktopTimeInMs;
return _x;
}
/**
* “Number of Restarts in the last 30 days. Valid values 0 to 9999999”
*
* @return property restartCount
*/
@Property(name="restartCount")
@JsonIgnore
public Optional getRestartCount() {
return Optional.ofNullable(restartCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code restartCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Number of Restarts in the last 30 days. Valid values 0 to 9999999”
*
* @param restartCount
* new value of {@code restartCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code restartCount} field changed
*/
public UserExperienceAnalyticsDevicePerformance withRestartCount(Integer restartCount) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("restartCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.restartCount = restartCount;
return _x;
}
/**
* “The user experience analytics device startup performance score. Valid values -1.
* 79769313486232E+308 to 1.79769313486232E+308”
*
* @return property startupPerformanceScore
*/
@Property(name="startupPerformanceScore")
@JsonIgnore
public Optional getStartupPerformanceScore() {
return Optional.ofNullable(startupPerformanceScore);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* startupPerformanceScore} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The user experience analytics device startup performance score. Valid values -1.
* 79769313486232E+308 to 1.79769313486232E+308”
*
* @param startupPerformanceScore
* new value of {@code startupPerformanceScore} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code startupPerformanceScore} field changed
*/
public UserExperienceAnalyticsDevicePerformance withStartupPerformanceScore(Double startupPerformanceScore) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = changedFields.add("startupPerformanceScore");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsDevicePerformance");
_x.startupPerformanceScore = startupPerformanceScore;
return _x;
}
public UserExperienceAnalyticsDevicePerformance withUnmappedField(String name, Object value) {
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public UserExperienceAnalyticsDevicePerformance patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public UserExperienceAnalyticsDevicePerformance put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
UserExperienceAnalyticsDevicePerformance _x = _copy();
_x.changedFields = null;
return _x;
}
private UserExperienceAnalyticsDevicePerformance _copy() {
UserExperienceAnalyticsDevicePerformance _x = new UserExperienceAnalyticsDevicePerformance();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.averageBlueScreens = averageBlueScreens;
_x.averageRestarts = averageRestarts;
_x.blueScreenCount = blueScreenCount;
_x.bootScore = bootScore;
_x.coreBootTimeInMs = coreBootTimeInMs;
_x.coreLoginTimeInMs = coreLoginTimeInMs;
_x.deviceCount = deviceCount;
_x.deviceName = deviceName;
_x.diskType = diskType;
_x.groupPolicyBootTimeInMs = groupPolicyBootTimeInMs;
_x.groupPolicyLoginTimeInMs = groupPolicyLoginTimeInMs;
_x.healthStatus = healthStatus;
_x.loginScore = loginScore;
_x.manufacturer = manufacturer;
_x.model = model;
_x.modelStartupPerformanceScore = modelStartupPerformanceScore;
_x.operatingSystemVersion = operatingSystemVersion;
_x.responsiveDesktopTimeInMs = responsiveDesktopTimeInMs;
_x.restartCount = restartCount;
_x.startupPerformanceScore = startupPerformanceScore;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("UserExperienceAnalyticsDevicePerformance[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("averageBlueScreens=");
b.append(this.averageBlueScreens);
b.append(", ");
b.append("averageRestarts=");
b.append(this.averageRestarts);
b.append(", ");
b.append("blueScreenCount=");
b.append(this.blueScreenCount);
b.append(", ");
b.append("bootScore=");
b.append(this.bootScore);
b.append(", ");
b.append("coreBootTimeInMs=");
b.append(this.coreBootTimeInMs);
b.append(", ");
b.append("coreLoginTimeInMs=");
b.append(this.coreLoginTimeInMs);
b.append(", ");
b.append("deviceCount=");
b.append(this.deviceCount);
b.append(", ");
b.append("deviceName=");
b.append(this.deviceName);
b.append(", ");
b.append("diskType=");
b.append(this.diskType);
b.append(", ");
b.append("groupPolicyBootTimeInMs=");
b.append(this.groupPolicyBootTimeInMs);
b.append(", ");
b.append("groupPolicyLoginTimeInMs=");
b.append(this.groupPolicyLoginTimeInMs);
b.append(", ");
b.append("healthStatus=");
b.append(this.healthStatus);
b.append(", ");
b.append("loginScore=");
b.append(this.loginScore);
b.append(", ");
b.append("manufacturer=");
b.append(this.manufacturer);
b.append(", ");
b.append("model=");
b.append(this.model);
b.append(", ");
b.append("modelStartupPerformanceScore=");
b.append(this.modelStartupPerformanceScore);
b.append(", ");
b.append("operatingSystemVersion=");
b.append(this.operatingSystemVersion);
b.append(", ");
b.append("responsiveDesktopTimeInMs=");
b.append(this.responsiveDesktopTimeInMs);
b.append(", ");
b.append("restartCount=");
b.append(this.restartCount);
b.append(", ");
b.append("startupPerformanceScore=");
b.append(this.startupPerformanceScore);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}