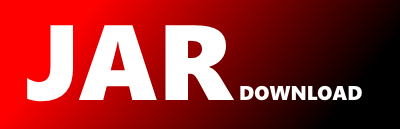
odata.msgraph.client.entity.UserExperienceAnalyticsModelScores Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Double;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
import odata.msgraph.client.enums.UserExperienceAnalyticsHealthState;
/**
* “The user experience analytics model scores entity consolidates the various
* Endpoint Analytics scores.”
*/@JsonPropertyOrder({
"@odata.type",
"appReliabilityScore",
"batteryHealthScore",
"endpointAnalyticsScore",
"healthStatus",
"manufacturer",
"model",
"modelDeviceCount",
"startupPerformanceScore",
"workFromAnywhereScore"})
@JsonInclude(Include.NON_NULL)
public class UserExperienceAnalyticsModelScores extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.userExperienceAnalyticsModelScores";
}
@JsonProperty("appReliabilityScore")
protected Double appReliabilityScore;
@JsonProperty("batteryHealthScore")
protected Double batteryHealthScore;
@JsonProperty("endpointAnalyticsScore")
protected Double endpointAnalyticsScore;
@JsonProperty("healthStatus")
protected UserExperienceAnalyticsHealthState healthStatus;
@JsonProperty("manufacturer")
protected String manufacturer;
@JsonProperty("model")
protected String model;
@JsonProperty("modelDeviceCount")
protected Long modelDeviceCount;
@JsonProperty("startupPerformanceScore")
protected Double startupPerformanceScore;
@JsonProperty("workFromAnywhereScore")
protected Double workFromAnywhereScore;
protected UserExperienceAnalyticsModelScores() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderUserExperienceAnalyticsModelScores() {
return new Builder();
}
public static final class Builder {
private String id;
private Double appReliabilityScore;
private Double batteryHealthScore;
private Double endpointAnalyticsScore;
private UserExperienceAnalyticsHealthState healthStatus;
private String manufacturer;
private String model;
private Long modelDeviceCount;
private Double startupPerformanceScore;
private Double workFromAnywhereScore;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
/**
* “Indicates a score calculated from application health data to indicate when a
* device is having problems running one or more applications. Valid values range
* from 0-100. Value -1 means associated score is unavailable. A higher score
* indicates a healthier device. Read-only. Valid values -1.79769313486232E+308 to
* 1.79769313486232E+308”
*
* @param appReliabilityScore
* value of {@code appReliabilityScore} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder appReliabilityScore(Double appReliabilityScore) {
this.appReliabilityScore = appReliabilityScore;
this.changedFields = changedFields.add("appReliabilityScore");
return this;
}
/**
* “Indicates a calulated score indicating the health of the device's battery. Valid
* values range from 0-100. Value -1 means associated score is unavailable. A
* higher score indicates a healthier device. Read-only. Valid values -1.
* 79769313486232E+308 to 1.79769313486232E+308”
*
* @param batteryHealthScore
* value of {@code batteryHealthScore} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder batteryHealthScore(Double batteryHealthScore) {
this.batteryHealthScore = batteryHealthScore;
this.changedFields = changedFields.add("batteryHealthScore");
return this;
}
/**
* “Indicates a weighted average of the various scores. Valid values range from 0-
* 100. Value -1 means associated score is unavailable. A higher score indicates a
* healthier device. Read-only. Valid values -1.79769313486232E+308 to 1.
* 79769313486232E+308”
*
* @param endpointAnalyticsScore
* value of {@code endpointAnalyticsScore} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder endpointAnalyticsScore(Double endpointAnalyticsScore) {
this.endpointAnalyticsScore = endpointAnalyticsScore;
this.changedFields = changedFields.add("endpointAnalyticsScore");
return this;
}
/**
* “The health status of the device. Possible values are: unknown, insufficientData,
* needsAttention, meetingGoals. Unknown by default. Supports: $filter, $select, $
* OrderBy. Read-only.”
*
* @param healthStatus
* value of {@code healthStatus} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder healthStatus(UserExperienceAnalyticsHealthState healthStatus) {
this.healthStatus = healthStatus;
this.changedFields = changedFields.add("healthStatus");
return this;
}
/**
* “The manufacturer name of the device. Examples: Microsoft Corporation, HP, Lenovo
* . Supports: $select, $OrderBy. Read-only.”
*
* @param manufacturer
* value of {@code manufacturer} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder manufacturer(String manufacturer) {
this.manufacturer = manufacturer;
this.changedFields = changedFields.add("manufacturer");
return this;
}
/**
* “The model name of the device. Supports: $select, $OrderBy. Read-only.”
*
* @param model
* value of {@code model} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder model(String model) {
this.model = model;
this.changedFields = changedFields.add("model");
return this;
}
/**
* “Indicates unique devices count of given model in a consolidated report. Supports
* : $select, $OrderBy. Read-only. Valid values -9.22337203685478E+18 to 9.
* 22337203685478E+18”
*
* @param modelDeviceCount
* value of {@code modelDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder modelDeviceCount(Long modelDeviceCount) {
this.modelDeviceCount = modelDeviceCount;
this.changedFields = changedFields.add("modelDeviceCount");
return this;
}
/**
* “Indicates a weighted average of boot score and logon score used for measuring
* startup performance. Valid values range from 0-100. Value -1 means associated
* score is unavailable. A higher score indicates a healthier device. Read-only.
* Valid values -1.79769313486232E+308 to 1.79769313486232E+308”
*
* @param startupPerformanceScore
* value of {@code startupPerformanceScore} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder startupPerformanceScore(Double startupPerformanceScore) {
this.startupPerformanceScore = startupPerformanceScore;
this.changedFields = changedFields.add("startupPerformanceScore");
return this;
}
/**
* “Indicates a weighted score of the work from anywhere on a device level. Valid
* values range from 0-100. Value -1 means associated score is unavailable. A
* higher score indicates a healthier device. Read-only. Valid values -1.
* 79769313486232E+308 to 1.79769313486232E+308”
*
* @param workFromAnywhereScore
* value of {@code workFromAnywhereScore} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workFromAnywhereScore(Double workFromAnywhereScore) {
this.workFromAnywhereScore = workFromAnywhereScore;
this.changedFields = changedFields.add("workFromAnywhereScore");
return this;
}
public UserExperienceAnalyticsModelScores build() {
UserExperienceAnalyticsModelScores _x = new UserExperienceAnalyticsModelScores();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.userExperienceAnalyticsModelScores";
_x.id = id;
_x.appReliabilityScore = appReliabilityScore;
_x.batteryHealthScore = batteryHealthScore;
_x.endpointAnalyticsScore = endpointAnalyticsScore;
_x.healthStatus = healthStatus;
_x.manufacturer = manufacturer;
_x.model = model;
_x.modelDeviceCount = modelDeviceCount;
_x.startupPerformanceScore = startupPerformanceScore;
_x.workFromAnywhereScore = workFromAnywhereScore;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “Indicates a score calculated from application health data to indicate when a
* device is having problems running one or more applications. Valid values range
* from 0-100. Value -1 means associated score is unavailable. A higher score
* indicates a healthier device. Read-only. Valid values -1.79769313486232E+308 to
* 1.79769313486232E+308”
*
* @return property appReliabilityScore
*/
@Property(name="appReliabilityScore")
@JsonIgnore
public Optional getAppReliabilityScore() {
return Optional.ofNullable(appReliabilityScore);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* appReliabilityScore} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Indicates a score calculated from application health data to indicate when a
* device is having problems running one or more applications. Valid values range
* from 0-100. Value -1 means associated score is unavailable. A higher score
* indicates a healthier device. Read-only. Valid values -1.79769313486232E+308 to
* 1.79769313486232E+308”
*
* @param appReliabilityScore
* new value of {@code appReliabilityScore} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code appReliabilityScore} field changed
*/
public UserExperienceAnalyticsModelScores withAppReliabilityScore(Double appReliabilityScore) {
UserExperienceAnalyticsModelScores _x = _copy();
_x.changedFields = changedFields.add("appReliabilityScore");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsModelScores");
_x.appReliabilityScore = appReliabilityScore;
return _x;
}
/**
* “Indicates a calulated score indicating the health of the device's battery. Valid
* values range from 0-100. Value -1 means associated score is unavailable. A
* higher score indicates a healthier device. Read-only. Valid values -1.
* 79769313486232E+308 to 1.79769313486232E+308”
*
* @return property batteryHealthScore
*/
@Property(name="batteryHealthScore")
@JsonIgnore
public Optional getBatteryHealthScore() {
return Optional.ofNullable(batteryHealthScore);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* batteryHealthScore} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Indicates a calulated score indicating the health of the device's battery. Valid
* values range from 0-100. Value -1 means associated score is unavailable. A
* higher score indicates a healthier device. Read-only. Valid values -1.
* 79769313486232E+308 to 1.79769313486232E+308”
*
* @param batteryHealthScore
* new value of {@code batteryHealthScore} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code batteryHealthScore} field changed
*/
public UserExperienceAnalyticsModelScores withBatteryHealthScore(Double batteryHealthScore) {
UserExperienceAnalyticsModelScores _x = _copy();
_x.changedFields = changedFields.add("batteryHealthScore");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsModelScores");
_x.batteryHealthScore = batteryHealthScore;
return _x;
}
/**
* “Indicates a weighted average of the various scores. Valid values range from 0-
* 100. Value -1 means associated score is unavailable. A higher score indicates a
* healthier device. Read-only. Valid values -1.79769313486232E+308 to 1.
* 79769313486232E+308”
*
* @return property endpointAnalyticsScore
*/
@Property(name="endpointAnalyticsScore")
@JsonIgnore
public Optional getEndpointAnalyticsScore() {
return Optional.ofNullable(endpointAnalyticsScore);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* endpointAnalyticsScore} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates a weighted average of the various scores. Valid values range from 0-
* 100. Value -1 means associated score is unavailable. A higher score indicates a
* healthier device. Read-only. Valid values -1.79769313486232E+308 to 1.
* 79769313486232E+308”
*
* @param endpointAnalyticsScore
* new value of {@code endpointAnalyticsScore} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code endpointAnalyticsScore} field changed
*/
public UserExperienceAnalyticsModelScores withEndpointAnalyticsScore(Double endpointAnalyticsScore) {
UserExperienceAnalyticsModelScores _x = _copy();
_x.changedFields = changedFields.add("endpointAnalyticsScore");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsModelScores");
_x.endpointAnalyticsScore = endpointAnalyticsScore;
return _x;
}
/**
* “The health status of the device. Possible values are: unknown, insufficientData,
* needsAttention, meetingGoals. Unknown by default. Supports: $filter, $select, $
* OrderBy. Read-only.”
*
* @return property healthStatus
*/
@Property(name="healthStatus")
@JsonIgnore
public Optional getHealthStatus() {
return Optional.ofNullable(healthStatus);
}
/**
* Returns an immutable copy of {@code this} with just the {@code healthStatus}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The health status of the device. Possible values are: unknown, insufficientData,
* needsAttention, meetingGoals. Unknown by default. Supports: $filter, $select, $
* OrderBy. Read-only.”
*
* @param healthStatus
* new value of {@code healthStatus} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code healthStatus} field changed
*/
public UserExperienceAnalyticsModelScores withHealthStatus(UserExperienceAnalyticsHealthState healthStatus) {
UserExperienceAnalyticsModelScores _x = _copy();
_x.changedFields = changedFields.add("healthStatus");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsModelScores");
_x.healthStatus = healthStatus;
return _x;
}
/**
* “The manufacturer name of the device. Examples: Microsoft Corporation, HP, Lenovo
* . Supports: $select, $OrderBy. Read-only.”
*
* @return property manufacturer
*/
@Property(name="manufacturer")
@JsonIgnore
public Optional getManufacturer() {
return Optional.ofNullable(manufacturer);
}
/**
* Returns an immutable copy of {@code this} with just the {@code manufacturer}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The manufacturer name of the device. Examples: Microsoft Corporation, HP, Lenovo
* . Supports: $select, $OrderBy. Read-only.”
*
* @param manufacturer
* new value of {@code manufacturer} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code manufacturer} field changed
*/
public UserExperienceAnalyticsModelScores withManufacturer(String manufacturer) {
UserExperienceAnalyticsModelScores _x = _copy();
_x.changedFields = changedFields.add("manufacturer");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsModelScores");
_x.manufacturer = manufacturer;
return _x;
}
/**
* “The model name of the device. Supports: $select, $OrderBy. Read-only.”
*
* @return property model
*/
@Property(name="model")
@JsonIgnore
public Optional getModel() {
return Optional.ofNullable(model);
}
/**
* Returns an immutable copy of {@code this} with just the {@code model} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The model name of the device. Supports: $select, $OrderBy. Read-only.”
*
* @param model
* new value of {@code model} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code model} field changed
*/
public UserExperienceAnalyticsModelScores withModel(String model) {
UserExperienceAnalyticsModelScores _x = _copy();
_x.changedFields = changedFields.add("model");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsModelScores");
_x.model = model;
return _x;
}
/**
* “Indicates unique devices count of given model in a consolidated report. Supports
* : $select, $OrderBy. Read-only. Valid values -9.22337203685478E+18 to 9.
* 22337203685478E+18”
*
* @return property modelDeviceCount
*/
@Property(name="modelDeviceCount")
@JsonIgnore
public Optional getModelDeviceCount() {
return Optional.ofNullable(modelDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code modelDeviceCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates unique devices count of given model in a consolidated report. Supports
* : $select, $OrderBy. Read-only. Valid values -9.22337203685478E+18 to 9.
* 22337203685478E+18”
*
* @param modelDeviceCount
* new value of {@code modelDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code modelDeviceCount} field changed
*/
public UserExperienceAnalyticsModelScores withModelDeviceCount(Long modelDeviceCount) {
UserExperienceAnalyticsModelScores _x = _copy();
_x.changedFields = changedFields.add("modelDeviceCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsModelScores");
_x.modelDeviceCount = modelDeviceCount;
return _x;
}
/**
* “Indicates a weighted average of boot score and logon score used for measuring
* startup performance. Valid values range from 0-100. Value -1 means associated
* score is unavailable. A higher score indicates a healthier device. Read-only.
* Valid values -1.79769313486232E+308 to 1.79769313486232E+308”
*
* @return property startupPerformanceScore
*/
@Property(name="startupPerformanceScore")
@JsonIgnore
public Optional getStartupPerformanceScore() {
return Optional.ofNullable(startupPerformanceScore);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* startupPerformanceScore} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates a weighted average of boot score and logon score used for measuring
* startup performance. Valid values range from 0-100. Value -1 means associated
* score is unavailable. A higher score indicates a healthier device. Read-only.
* Valid values -1.79769313486232E+308 to 1.79769313486232E+308”
*
* @param startupPerformanceScore
* new value of {@code startupPerformanceScore} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code startupPerformanceScore} field changed
*/
public UserExperienceAnalyticsModelScores withStartupPerformanceScore(Double startupPerformanceScore) {
UserExperienceAnalyticsModelScores _x = _copy();
_x.changedFields = changedFields.add("startupPerformanceScore");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsModelScores");
_x.startupPerformanceScore = startupPerformanceScore;
return _x;
}
/**
* “Indicates a weighted score of the work from anywhere on a device level. Valid
* values range from 0-100. Value -1 means associated score is unavailable. A
* higher score indicates a healthier device. Read-only. Valid values -1.
* 79769313486232E+308 to 1.79769313486232E+308”
*
* @return property workFromAnywhereScore
*/
@Property(name="workFromAnywhereScore")
@JsonIgnore
public Optional getWorkFromAnywhereScore() {
return Optional.ofNullable(workFromAnywhereScore);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workFromAnywhereScore} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Indicates a weighted score of the work from anywhere on a device level. Valid
* values range from 0-100. Value -1 means associated score is unavailable. A
* higher score indicates a healthier device. Read-only. Valid values -1.
* 79769313486232E+308 to 1.79769313486232E+308”
*
* @param workFromAnywhereScore
* new value of {@code workFromAnywhereScore} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workFromAnywhereScore} field changed
*/
public UserExperienceAnalyticsModelScores withWorkFromAnywhereScore(Double workFromAnywhereScore) {
UserExperienceAnalyticsModelScores _x = _copy();
_x.changedFields = changedFields.add("workFromAnywhereScore");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsModelScores");
_x.workFromAnywhereScore = workFromAnywhereScore;
return _x;
}
public UserExperienceAnalyticsModelScores withUnmappedField(String name, Object value) {
UserExperienceAnalyticsModelScores _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public UserExperienceAnalyticsModelScores patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
UserExperienceAnalyticsModelScores _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public UserExperienceAnalyticsModelScores put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
UserExperienceAnalyticsModelScores _x = _copy();
_x.changedFields = null;
return _x;
}
private UserExperienceAnalyticsModelScores _copy() {
UserExperienceAnalyticsModelScores _x = new UserExperienceAnalyticsModelScores();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.appReliabilityScore = appReliabilityScore;
_x.batteryHealthScore = batteryHealthScore;
_x.endpointAnalyticsScore = endpointAnalyticsScore;
_x.healthStatus = healthStatus;
_x.manufacturer = manufacturer;
_x.model = model;
_x.modelDeviceCount = modelDeviceCount;
_x.startupPerformanceScore = startupPerformanceScore;
_x.workFromAnywhereScore = workFromAnywhereScore;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("UserExperienceAnalyticsModelScores[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("appReliabilityScore=");
b.append(this.appReliabilityScore);
b.append(", ");
b.append("batteryHealthScore=");
b.append(this.batteryHealthScore);
b.append(", ");
b.append("endpointAnalyticsScore=");
b.append(this.endpointAnalyticsScore);
b.append(", ");
b.append("healthStatus=");
b.append(this.healthStatus);
b.append(", ");
b.append("manufacturer=");
b.append(this.manufacturer);
b.append(", ");
b.append("model=");
b.append(this.model);
b.append(", ");
b.append("modelDeviceCount=");
b.append(this.modelDeviceCount);
b.append(", ");
b.append("startupPerformanceScore=");
b.append(this.startupPerformanceScore);
b.append(", ");
b.append("workFromAnywhereScore=");
b.append(this.workFromAnywhereScore);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}