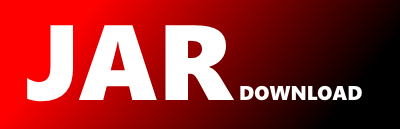
odata.msgraph.client.entity.UserRegistrationDetails Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.enums.SignInUserType;
import odata.msgraph.client.enums.UserDefaultAuthenticationMethod;
/**
*
* Org.OData.Capabilities.V1.FilterRestrictions
*
* Filterable = true
*
* Org.OData.Capabilities.V1.SkipSupported
*
* false
*
* Org.OData.Capabilities.V1.TopSupported
*
* true
*/@JsonPropertyOrder({
"@odata.type",
"isAdmin",
"isMfaCapable",
"isMfaRegistered",
"isPasswordlessCapable",
"isSsprCapable",
"isSsprEnabled",
"isSsprRegistered",
"isSystemPreferredAuthenticationMethodEnabled",
"lastUpdatedDateTime",
"methodsRegistered",
"systemPreferredAuthenticationMethods",
"userDisplayName",
"userPreferredMethodForSecondaryAuthentication",
"userPrincipalName",
"userType"})
@JsonInclude(Include.NON_NULL)
public class UserRegistrationDetails extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.userRegistrationDetails";
}
@JsonProperty("isAdmin")
protected Boolean isAdmin;
@JsonProperty("isMfaCapable")
protected Boolean isMfaCapable;
@JsonProperty("isMfaRegistered")
protected Boolean isMfaRegistered;
@JsonProperty("isPasswordlessCapable")
protected Boolean isPasswordlessCapable;
@JsonProperty("isSsprCapable")
protected Boolean isSsprCapable;
@JsonProperty("isSsprEnabled")
protected Boolean isSsprEnabled;
@JsonProperty("isSsprRegistered")
protected Boolean isSsprRegistered;
@JsonProperty("isSystemPreferredAuthenticationMethodEnabled")
protected Boolean isSystemPreferredAuthenticationMethodEnabled;
@JsonProperty("lastUpdatedDateTime")
protected OffsetDateTime lastUpdatedDateTime;
@JsonProperty("methodsRegistered")
protected List methodsRegistered;
@JsonProperty("methodsRegistered@nextLink")
protected String methodsRegisteredNextLink;
@JsonProperty("systemPreferredAuthenticationMethods")
protected List systemPreferredAuthenticationMethods;
@JsonProperty("systemPreferredAuthenticationMethods@nextLink")
protected String systemPreferredAuthenticationMethodsNextLink;
@JsonProperty("userDisplayName")
protected String userDisplayName;
@JsonProperty("userPreferredMethodForSecondaryAuthentication")
protected UserDefaultAuthenticationMethod userPreferredMethodForSecondaryAuthentication;
@JsonProperty("userPrincipalName")
protected String userPrincipalName;
@JsonProperty("userType")
protected SignInUserType userType;
protected UserRegistrationDetails() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderUserRegistrationDetails() {
return new Builder();
}
public static final class Builder {
private String id;
private Boolean isAdmin;
private Boolean isMfaCapable;
private Boolean isMfaRegistered;
private Boolean isPasswordlessCapable;
private Boolean isSsprCapable;
private Boolean isSsprEnabled;
private Boolean isSsprRegistered;
private Boolean isSystemPreferredAuthenticationMethodEnabled;
private OffsetDateTime lastUpdatedDateTime;
private List methodsRegistered;
private String methodsRegisteredNextLink;
private List systemPreferredAuthenticationMethods;
private String systemPreferredAuthenticationMethodsNextLink;
private String userDisplayName;
private UserDefaultAuthenticationMethod userPreferredMethodForSecondaryAuthentication;
private String userPrincipalName;
private SignInUserType userType;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder isAdmin(Boolean isAdmin) {
this.isAdmin = isAdmin;
this.changedFields = changedFields.add("isAdmin");
return this;
}
public Builder isMfaCapable(Boolean isMfaCapable) {
this.isMfaCapable = isMfaCapable;
this.changedFields = changedFields.add("isMfaCapable");
return this;
}
public Builder isMfaRegistered(Boolean isMfaRegistered) {
this.isMfaRegistered = isMfaRegistered;
this.changedFields = changedFields.add("isMfaRegistered");
return this;
}
public Builder isPasswordlessCapable(Boolean isPasswordlessCapable) {
this.isPasswordlessCapable = isPasswordlessCapable;
this.changedFields = changedFields.add("isPasswordlessCapable");
return this;
}
public Builder isSsprCapable(Boolean isSsprCapable) {
this.isSsprCapable = isSsprCapable;
this.changedFields = changedFields.add("isSsprCapable");
return this;
}
public Builder isSsprEnabled(Boolean isSsprEnabled) {
this.isSsprEnabled = isSsprEnabled;
this.changedFields = changedFields.add("isSsprEnabled");
return this;
}
public Builder isSsprRegistered(Boolean isSsprRegistered) {
this.isSsprRegistered = isSsprRegistered;
this.changedFields = changedFields.add("isSsprRegistered");
return this;
}
public Builder isSystemPreferredAuthenticationMethodEnabled(Boolean isSystemPreferredAuthenticationMethodEnabled) {
this.isSystemPreferredAuthenticationMethodEnabled = isSystemPreferredAuthenticationMethodEnabled;
this.changedFields = changedFields.add("isSystemPreferredAuthenticationMethodEnabled");
return this;
}
public Builder lastUpdatedDateTime(OffsetDateTime lastUpdatedDateTime) {
this.lastUpdatedDateTime = lastUpdatedDateTime;
this.changedFields = changedFields.add("lastUpdatedDateTime");
return this;
}
public Builder methodsRegistered(List methodsRegistered) {
this.methodsRegistered = methodsRegistered;
this.changedFields = changedFields.add("methodsRegistered");
return this;
}
public Builder methodsRegistered(String... methodsRegistered) {
return methodsRegistered(Arrays.asList(methodsRegistered));
}
public Builder methodsRegisteredNextLink(String methodsRegisteredNextLink) {
this.methodsRegisteredNextLink = methodsRegisteredNextLink;
this.changedFields = changedFields.add("methodsRegistered");
return this;
}
public Builder systemPreferredAuthenticationMethods(List systemPreferredAuthenticationMethods) {
this.systemPreferredAuthenticationMethods = systemPreferredAuthenticationMethods;
this.changedFields = changedFields.add("systemPreferredAuthenticationMethods");
return this;
}
public Builder systemPreferredAuthenticationMethods(String... systemPreferredAuthenticationMethods) {
return systemPreferredAuthenticationMethods(Arrays.asList(systemPreferredAuthenticationMethods));
}
public Builder systemPreferredAuthenticationMethodsNextLink(String systemPreferredAuthenticationMethodsNextLink) {
this.systemPreferredAuthenticationMethodsNextLink = systemPreferredAuthenticationMethodsNextLink;
this.changedFields = changedFields.add("systemPreferredAuthenticationMethods");
return this;
}
public Builder userDisplayName(String userDisplayName) {
this.userDisplayName = userDisplayName;
this.changedFields = changedFields.add("userDisplayName");
return this;
}
public Builder userPreferredMethodForSecondaryAuthentication(UserDefaultAuthenticationMethod userPreferredMethodForSecondaryAuthentication) {
this.userPreferredMethodForSecondaryAuthentication = userPreferredMethodForSecondaryAuthentication;
this.changedFields = changedFields.add("userPreferredMethodForSecondaryAuthentication");
return this;
}
public Builder userPrincipalName(String userPrincipalName) {
this.userPrincipalName = userPrincipalName;
this.changedFields = changedFields.add("userPrincipalName");
return this;
}
public Builder userType(SignInUserType userType) {
this.userType = userType;
this.changedFields = changedFields.add("userType");
return this;
}
public UserRegistrationDetails build() {
UserRegistrationDetails _x = new UserRegistrationDetails();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.userRegistrationDetails";
_x.id = id;
_x.isAdmin = isAdmin;
_x.isMfaCapable = isMfaCapable;
_x.isMfaRegistered = isMfaRegistered;
_x.isPasswordlessCapable = isPasswordlessCapable;
_x.isSsprCapable = isSsprCapable;
_x.isSsprEnabled = isSsprEnabled;
_x.isSsprRegistered = isSsprRegistered;
_x.isSystemPreferredAuthenticationMethodEnabled = isSystemPreferredAuthenticationMethodEnabled;
_x.lastUpdatedDateTime = lastUpdatedDateTime;
_x.methodsRegistered = methodsRegistered;
_x.methodsRegisteredNextLink = methodsRegisteredNextLink;
_x.systemPreferredAuthenticationMethods = systemPreferredAuthenticationMethods;
_x.systemPreferredAuthenticationMethodsNextLink = systemPreferredAuthenticationMethodsNextLink;
_x.userDisplayName = userDisplayName;
_x.userPreferredMethodForSecondaryAuthentication = userPreferredMethodForSecondaryAuthentication;
_x.userPrincipalName = userPrincipalName;
_x.userType = userType;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
@Property(name="isAdmin")
@JsonIgnore
public Optional getIsAdmin() {
return Optional.ofNullable(isAdmin);
}
public UserRegistrationDetails withIsAdmin(Boolean isAdmin) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("isAdmin");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.isAdmin = isAdmin;
return _x;
}
@Property(name="isMfaCapable")
@JsonIgnore
public Optional getIsMfaCapable() {
return Optional.ofNullable(isMfaCapable);
}
public UserRegistrationDetails withIsMfaCapable(Boolean isMfaCapable) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("isMfaCapable");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.isMfaCapable = isMfaCapable;
return _x;
}
@Property(name="isMfaRegistered")
@JsonIgnore
public Optional getIsMfaRegistered() {
return Optional.ofNullable(isMfaRegistered);
}
public UserRegistrationDetails withIsMfaRegistered(Boolean isMfaRegistered) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("isMfaRegistered");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.isMfaRegistered = isMfaRegistered;
return _x;
}
@Property(name="isPasswordlessCapable")
@JsonIgnore
public Optional getIsPasswordlessCapable() {
return Optional.ofNullable(isPasswordlessCapable);
}
public UserRegistrationDetails withIsPasswordlessCapable(Boolean isPasswordlessCapable) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("isPasswordlessCapable");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.isPasswordlessCapable = isPasswordlessCapable;
return _x;
}
@Property(name="isSsprCapable")
@JsonIgnore
public Optional getIsSsprCapable() {
return Optional.ofNullable(isSsprCapable);
}
public UserRegistrationDetails withIsSsprCapable(Boolean isSsprCapable) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("isSsprCapable");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.isSsprCapable = isSsprCapable;
return _x;
}
@Property(name="isSsprEnabled")
@JsonIgnore
public Optional getIsSsprEnabled() {
return Optional.ofNullable(isSsprEnabled);
}
public UserRegistrationDetails withIsSsprEnabled(Boolean isSsprEnabled) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("isSsprEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.isSsprEnabled = isSsprEnabled;
return _x;
}
@Property(name="isSsprRegistered")
@JsonIgnore
public Optional getIsSsprRegistered() {
return Optional.ofNullable(isSsprRegistered);
}
public UserRegistrationDetails withIsSsprRegistered(Boolean isSsprRegistered) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("isSsprRegistered");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.isSsprRegistered = isSsprRegistered;
return _x;
}
@Property(name="isSystemPreferredAuthenticationMethodEnabled")
@JsonIgnore
public Optional getIsSystemPreferredAuthenticationMethodEnabled() {
return Optional.ofNullable(isSystemPreferredAuthenticationMethodEnabled);
}
public UserRegistrationDetails withIsSystemPreferredAuthenticationMethodEnabled(Boolean isSystemPreferredAuthenticationMethodEnabled) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("isSystemPreferredAuthenticationMethodEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.isSystemPreferredAuthenticationMethodEnabled = isSystemPreferredAuthenticationMethodEnabled;
return _x;
}
@Property(name="lastUpdatedDateTime")
@JsonIgnore
public Optional getLastUpdatedDateTime() {
return Optional.ofNullable(lastUpdatedDateTime);
}
public UserRegistrationDetails withLastUpdatedDateTime(OffsetDateTime lastUpdatedDateTime) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("lastUpdatedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.lastUpdatedDateTime = lastUpdatedDateTime;
return _x;
}
@Property(name="methodsRegistered")
@JsonIgnore
public CollectionPage getMethodsRegistered() {
return new CollectionPage(contextPath, String.class, this.methodsRegistered, Optional.ofNullable(methodsRegisteredNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public UserRegistrationDetails withMethodsRegistered(List methodsRegistered) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("methodsRegistered");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.methodsRegistered = methodsRegistered;
return _x;
}
@Property(name="methodsRegistered")
@JsonIgnore
public CollectionPage getMethodsRegistered(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.methodsRegistered, Optional.ofNullable(methodsRegisteredNextLink), Collections.emptyList(), options);
}
@Property(name="systemPreferredAuthenticationMethods")
@JsonIgnore
public CollectionPage getSystemPreferredAuthenticationMethods() {
return new CollectionPage(contextPath, String.class, this.systemPreferredAuthenticationMethods, Optional.ofNullable(systemPreferredAuthenticationMethodsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public UserRegistrationDetails withSystemPreferredAuthenticationMethods(List systemPreferredAuthenticationMethods) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("systemPreferredAuthenticationMethods");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.systemPreferredAuthenticationMethods = systemPreferredAuthenticationMethods;
return _x;
}
@Property(name="systemPreferredAuthenticationMethods")
@JsonIgnore
public CollectionPage getSystemPreferredAuthenticationMethods(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.systemPreferredAuthenticationMethods, Optional.ofNullable(systemPreferredAuthenticationMethodsNextLink), Collections.emptyList(), options);
}
@Property(name="userDisplayName")
@JsonIgnore
public Optional getUserDisplayName() {
return Optional.ofNullable(userDisplayName);
}
public UserRegistrationDetails withUserDisplayName(String userDisplayName) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("userDisplayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.userDisplayName = userDisplayName;
return _x;
}
@Property(name="userPreferredMethodForSecondaryAuthentication")
@JsonIgnore
public Optional getUserPreferredMethodForSecondaryAuthentication() {
return Optional.ofNullable(userPreferredMethodForSecondaryAuthentication);
}
public UserRegistrationDetails withUserPreferredMethodForSecondaryAuthentication(UserDefaultAuthenticationMethod userPreferredMethodForSecondaryAuthentication) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("userPreferredMethodForSecondaryAuthentication");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.userPreferredMethodForSecondaryAuthentication = userPreferredMethodForSecondaryAuthentication;
return _x;
}
@Property(name="userPrincipalName")
@JsonIgnore
public Optional getUserPrincipalName() {
return Optional.ofNullable(userPrincipalName);
}
public UserRegistrationDetails withUserPrincipalName(String userPrincipalName) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("userPrincipalName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.userPrincipalName = userPrincipalName;
return _x;
}
@Property(name="userType")
@JsonIgnore
public Optional getUserType() {
return Optional.ofNullable(userType);
}
public UserRegistrationDetails withUserType(SignInUserType userType) {
UserRegistrationDetails _x = _copy();
_x.changedFields = changedFields.add("userType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userRegistrationDetails");
_x.userType = userType;
return _x;
}
public UserRegistrationDetails withUnmappedField(String name, Object value) {
UserRegistrationDetails _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public UserRegistrationDetails patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
UserRegistrationDetails _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public UserRegistrationDetails put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
UserRegistrationDetails _x = _copy();
_x.changedFields = null;
return _x;
}
private UserRegistrationDetails _copy() {
UserRegistrationDetails _x = new UserRegistrationDetails();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.isAdmin = isAdmin;
_x.isMfaCapable = isMfaCapable;
_x.isMfaRegistered = isMfaRegistered;
_x.isPasswordlessCapable = isPasswordlessCapable;
_x.isSsprCapable = isSsprCapable;
_x.isSsprEnabled = isSsprEnabled;
_x.isSsprRegistered = isSsprRegistered;
_x.isSystemPreferredAuthenticationMethodEnabled = isSystemPreferredAuthenticationMethodEnabled;
_x.lastUpdatedDateTime = lastUpdatedDateTime;
_x.methodsRegistered = methodsRegistered;
_x.systemPreferredAuthenticationMethods = systemPreferredAuthenticationMethods;
_x.userDisplayName = userDisplayName;
_x.userPreferredMethodForSecondaryAuthentication = userPreferredMethodForSecondaryAuthentication;
_x.userPrincipalName = userPrincipalName;
_x.userType = userType;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("UserRegistrationDetails[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("isAdmin=");
b.append(this.isAdmin);
b.append(", ");
b.append("isMfaCapable=");
b.append(this.isMfaCapable);
b.append(", ");
b.append("isMfaRegistered=");
b.append(this.isMfaRegistered);
b.append(", ");
b.append("isPasswordlessCapable=");
b.append(this.isPasswordlessCapable);
b.append(", ");
b.append("isSsprCapable=");
b.append(this.isSsprCapable);
b.append(", ");
b.append("isSsprEnabled=");
b.append(this.isSsprEnabled);
b.append(", ");
b.append("isSsprRegistered=");
b.append(this.isSsprRegistered);
b.append(", ");
b.append("isSystemPreferredAuthenticationMethodEnabled=");
b.append(this.isSystemPreferredAuthenticationMethodEnabled);
b.append(", ");
b.append("lastUpdatedDateTime=");
b.append(this.lastUpdatedDateTime);
b.append(", ");
b.append("methodsRegistered=");
b.append(this.methodsRegistered);
b.append(", ");
b.append("systemPreferredAuthenticationMethods=");
b.append(this.systemPreferredAuthenticationMethods);
b.append(", ");
b.append("userDisplayName=");
b.append(this.userDisplayName);
b.append(", ");
b.append("userPreferredMethodForSecondaryAuthentication=");
b.append(this.userPreferredMethodForSecondaryAuthentication);
b.append(", ");
b.append("userPrincipalName=");
b.append(this.userPrincipalName);
b.append(", ");
b.append("userType=");
b.append(this.userType);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}