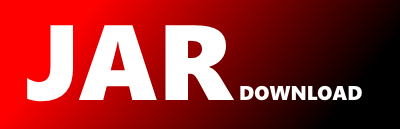
odata.msgraph.client.entity.Windows10TeamGeneralConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.LocalTime;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.enums.MiracastChannel;
import odata.msgraph.client.enums.WelcomeScreenMeetingInformation;
/**
* “This topic provides descriptions of the declared methods, properties and
* relationships exposed by the windows10TeamGeneralConfiguration resource.”
*/@JsonPropertyOrder({
"@odata.type",
"azureOperationalInsightsBlockTelemetry",
"azureOperationalInsightsWorkspaceId",
"azureOperationalInsightsWorkspaceKey",
"connectAppBlockAutoLaunch",
"maintenanceWindowBlocked",
"maintenanceWindowDurationInHours",
"maintenanceWindowStartTime",
"miracastBlocked",
"miracastChannel",
"miracastRequirePin",
"settingsBlockMyMeetingsAndFiles",
"settingsBlockSessionResume",
"settingsBlockSigninSuggestions",
"settingsDefaultVolume",
"settingsScreenTimeoutInMinutes",
"settingsSessionTimeoutInMinutes",
"settingsSleepTimeoutInMinutes",
"welcomeScreenBackgroundImageUrl",
"welcomeScreenBlockAutomaticWakeUp",
"welcomeScreenMeetingInformation"})
@JsonInclude(Include.NON_NULL)
public class Windows10TeamGeneralConfiguration extends DeviceConfiguration implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.windows10TeamGeneralConfiguration";
}
@JsonProperty("azureOperationalInsightsBlockTelemetry")
protected Boolean azureOperationalInsightsBlockTelemetry;
@JsonProperty("azureOperationalInsightsWorkspaceId")
protected String azureOperationalInsightsWorkspaceId;
@JsonProperty("azureOperationalInsightsWorkspaceKey")
protected String azureOperationalInsightsWorkspaceKey;
@JsonProperty("connectAppBlockAutoLaunch")
protected Boolean connectAppBlockAutoLaunch;
@JsonProperty("maintenanceWindowBlocked")
protected Boolean maintenanceWindowBlocked;
@JsonProperty("maintenanceWindowDurationInHours")
protected Integer maintenanceWindowDurationInHours;
@JsonProperty("maintenanceWindowStartTime")
protected LocalTime maintenanceWindowStartTime;
@JsonProperty("miracastBlocked")
protected Boolean miracastBlocked;
@JsonProperty("miracastChannel")
protected MiracastChannel miracastChannel;
@JsonProperty("miracastRequirePin")
protected Boolean miracastRequirePin;
@JsonProperty("settingsBlockMyMeetingsAndFiles")
protected Boolean settingsBlockMyMeetingsAndFiles;
@JsonProperty("settingsBlockSessionResume")
protected Boolean settingsBlockSessionResume;
@JsonProperty("settingsBlockSigninSuggestions")
protected Boolean settingsBlockSigninSuggestions;
@JsonProperty("settingsDefaultVolume")
protected Integer settingsDefaultVolume;
@JsonProperty("settingsScreenTimeoutInMinutes")
protected Integer settingsScreenTimeoutInMinutes;
@JsonProperty("settingsSessionTimeoutInMinutes")
protected Integer settingsSessionTimeoutInMinutes;
@JsonProperty("settingsSleepTimeoutInMinutes")
protected Integer settingsSleepTimeoutInMinutes;
@JsonProperty("welcomeScreenBackgroundImageUrl")
protected String welcomeScreenBackgroundImageUrl;
@JsonProperty("welcomeScreenBlockAutomaticWakeUp")
protected Boolean welcomeScreenBlockAutomaticWakeUp;
@JsonProperty("welcomeScreenMeetingInformation")
protected WelcomeScreenMeetingInformation welcomeScreenMeetingInformation;
protected Windows10TeamGeneralConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderWindows10TeamGeneralConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private Integer version;
private List assignments;
private List deviceSettingStateSummaries;
private List deviceStatuses;
private DeviceConfigurationDeviceOverview deviceStatusOverview;
private List userStatuses;
private DeviceConfigurationUserOverview userStatusOverview;
private Boolean azureOperationalInsightsBlockTelemetry;
private String azureOperationalInsightsWorkspaceId;
private String azureOperationalInsightsWorkspaceKey;
private Boolean connectAppBlockAutoLaunch;
private Boolean maintenanceWindowBlocked;
private Integer maintenanceWindowDurationInHours;
private LocalTime maintenanceWindowStartTime;
private Boolean miracastBlocked;
private MiracastChannel miracastChannel;
private Boolean miracastRequirePin;
private Boolean settingsBlockMyMeetingsAndFiles;
private Boolean settingsBlockSessionResume;
private Boolean settingsBlockSigninSuggestions;
private Integer settingsDefaultVolume;
private Integer settingsScreenTimeoutInMinutes;
private Integer settingsSessionTimeoutInMinutes;
private Integer settingsSleepTimeoutInMinutes;
private String welcomeScreenBackgroundImageUrl;
private Boolean welcomeScreenBlockAutomaticWakeUp;
private WelcomeScreenMeetingInformation welcomeScreenMeetingInformation;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
public Builder assignments(List assignments) {
this.assignments = assignments;
this.changedFields = changedFields.add("assignments");
return this;
}
public Builder assignments(DeviceConfigurationAssignment... assignments) {
return assignments(Arrays.asList(assignments));
}
public Builder deviceSettingStateSummaries(List deviceSettingStateSummaries) {
this.deviceSettingStateSummaries = deviceSettingStateSummaries;
this.changedFields = changedFields.add("deviceSettingStateSummaries");
return this;
}
public Builder deviceSettingStateSummaries(SettingStateDeviceSummary... deviceSettingStateSummaries) {
return deviceSettingStateSummaries(Arrays.asList(deviceSettingStateSummaries));
}
public Builder deviceStatuses(List deviceStatuses) {
this.deviceStatuses = deviceStatuses;
this.changedFields = changedFields.add("deviceStatuses");
return this;
}
public Builder deviceStatuses(DeviceConfigurationDeviceStatus... deviceStatuses) {
return deviceStatuses(Arrays.asList(deviceStatuses));
}
public Builder deviceStatusOverview(DeviceConfigurationDeviceOverview deviceStatusOverview) {
this.deviceStatusOverview = deviceStatusOverview;
this.changedFields = changedFields.add("deviceStatusOverview");
return this;
}
public Builder userStatuses(List userStatuses) {
this.userStatuses = userStatuses;
this.changedFields = changedFields.add("userStatuses");
return this;
}
public Builder userStatuses(DeviceConfigurationUserStatus... userStatuses) {
return userStatuses(Arrays.asList(userStatuses));
}
public Builder userStatusOverview(DeviceConfigurationUserOverview userStatusOverview) {
this.userStatusOverview = userStatusOverview;
this.changedFields = changedFields.add("userStatusOverview");
return this;
}
/**
* “Indicates whether or not to Block Azure Operational Insights.”
*
* @param azureOperationalInsightsBlockTelemetry
* value of {@code azureOperationalInsightsBlockTelemetry} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder azureOperationalInsightsBlockTelemetry(Boolean azureOperationalInsightsBlockTelemetry) {
this.azureOperationalInsightsBlockTelemetry = azureOperationalInsightsBlockTelemetry;
this.changedFields = changedFields.add("azureOperationalInsightsBlockTelemetry");
return this;
}
/**
* “The Azure Operational Insights workspace id.”
*
* @param azureOperationalInsightsWorkspaceId
* value of {@code azureOperationalInsightsWorkspaceId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder azureOperationalInsightsWorkspaceId(String azureOperationalInsightsWorkspaceId) {
this.azureOperationalInsightsWorkspaceId = azureOperationalInsightsWorkspaceId;
this.changedFields = changedFields.add("azureOperationalInsightsWorkspaceId");
return this;
}
/**
* “The Azure Operational Insights Workspace key.”
*
* @param azureOperationalInsightsWorkspaceKey
* value of {@code azureOperationalInsightsWorkspaceKey} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder azureOperationalInsightsWorkspaceKey(String azureOperationalInsightsWorkspaceKey) {
this.azureOperationalInsightsWorkspaceKey = azureOperationalInsightsWorkspaceKey;
this.changedFields = changedFields.add("azureOperationalInsightsWorkspaceKey");
return this;
}
/**
* “Specifies whether to automatically launch the Connect app whenever a projection
* is initiated.”
*
* @param connectAppBlockAutoLaunch
* value of {@code connectAppBlockAutoLaunch} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder connectAppBlockAutoLaunch(Boolean connectAppBlockAutoLaunch) {
this.connectAppBlockAutoLaunch = connectAppBlockAutoLaunch;
this.changedFields = changedFields.add("connectAppBlockAutoLaunch");
return this;
}
/**
* “Indicates whether or not to Block setting a maintenance window for device
* updates.”
*
* @param maintenanceWindowBlocked
* value of {@code maintenanceWindowBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder maintenanceWindowBlocked(Boolean maintenanceWindowBlocked) {
this.maintenanceWindowBlocked = maintenanceWindowBlocked;
this.changedFields = changedFields.add("maintenanceWindowBlocked");
return this;
}
/**
* “Maintenance window duration for device updates. Valid values 0 to 5”
*
* @param maintenanceWindowDurationInHours
* value of {@code maintenanceWindowDurationInHours} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder maintenanceWindowDurationInHours(Integer maintenanceWindowDurationInHours) {
this.maintenanceWindowDurationInHours = maintenanceWindowDurationInHours;
this.changedFields = changedFields.add("maintenanceWindowDurationInHours");
return this;
}
/**
* “Maintenance window start time for device updates.”
*
* @param maintenanceWindowStartTime
* value of {@code maintenanceWindowStartTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder maintenanceWindowStartTime(LocalTime maintenanceWindowStartTime) {
this.maintenanceWindowStartTime = maintenanceWindowStartTime;
this.changedFields = changedFields.add("maintenanceWindowStartTime");
return this;
}
/**
* “Indicates whether or not to Block wireless projection.”
*
* @param miracastBlocked
* value of {@code miracastBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder miracastBlocked(Boolean miracastBlocked) {
this.miracastBlocked = miracastBlocked;
this.changedFields = changedFields.add("miracastBlocked");
return this;
}
/**
* “The channel.”
*
* @param miracastChannel
* value of {@code miracastChannel} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder miracastChannel(MiracastChannel miracastChannel) {
this.miracastChannel = miracastChannel;
this.changedFields = changedFields.add("miracastChannel");
return this;
}
/**
* “Indicates whether or not to require a pin for wireless projection.”
*
* @param miracastRequirePin
* value of {@code miracastRequirePin} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder miracastRequirePin(Boolean miracastRequirePin) {
this.miracastRequirePin = miracastRequirePin;
this.changedFields = changedFields.add("miracastRequirePin");
return this;
}
/**
* “Specifies whether to disable the "My meetings and files" feature in the Start
* menu, which shows the signed-in user's meetings and files from Office 365.”
*
* @param settingsBlockMyMeetingsAndFiles
* value of {@code settingsBlockMyMeetingsAndFiles} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder settingsBlockMyMeetingsAndFiles(Boolean settingsBlockMyMeetingsAndFiles) {
this.settingsBlockMyMeetingsAndFiles = settingsBlockMyMeetingsAndFiles;
this.changedFields = changedFields.add("settingsBlockMyMeetingsAndFiles");
return this;
}
/**
* “Specifies whether to allow the ability to resume a session when the session
* times out.”
*
* @param settingsBlockSessionResume
* value of {@code settingsBlockSessionResume} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder settingsBlockSessionResume(Boolean settingsBlockSessionResume) {
this.settingsBlockSessionResume = settingsBlockSessionResume;
this.changedFields = changedFields.add("settingsBlockSessionResume");
return this;
}
/**
* “Specifies whether to disable auto-populating of the sign-in dialog with invitees
* from scheduled meetings.”
*
* @param settingsBlockSigninSuggestions
* value of {@code settingsBlockSigninSuggestions} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder settingsBlockSigninSuggestions(Boolean settingsBlockSigninSuggestions) {
this.settingsBlockSigninSuggestions = settingsBlockSigninSuggestions;
this.changedFields = changedFields.add("settingsBlockSigninSuggestions");
return this;
}
/**
* “Specifies the default volume value for a new session. Permitted values are 0-100
* . The default is 45. Valid values 0 to 100”
*
* @param settingsDefaultVolume
* value of {@code settingsDefaultVolume} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder settingsDefaultVolume(Integer settingsDefaultVolume) {
this.settingsDefaultVolume = settingsDefaultVolume;
this.changedFields = changedFields.add("settingsDefaultVolume");
return this;
}
/**
* “Specifies the number of minutes until the Hub screen turns off.”
*
* @param settingsScreenTimeoutInMinutes
* value of {@code settingsScreenTimeoutInMinutes} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder settingsScreenTimeoutInMinutes(Integer settingsScreenTimeoutInMinutes) {
this.settingsScreenTimeoutInMinutes = settingsScreenTimeoutInMinutes;
this.changedFields = changedFields.add("settingsScreenTimeoutInMinutes");
return this;
}
/**
* “Specifies the number of minutes until the session times out.”
*
* @param settingsSessionTimeoutInMinutes
* value of {@code settingsSessionTimeoutInMinutes} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder settingsSessionTimeoutInMinutes(Integer settingsSessionTimeoutInMinutes) {
this.settingsSessionTimeoutInMinutes = settingsSessionTimeoutInMinutes;
this.changedFields = changedFields.add("settingsSessionTimeoutInMinutes");
return this;
}
/**
* “Specifies the number of minutes until the Hub enters sleep mode.”
*
* @param settingsSleepTimeoutInMinutes
* value of {@code settingsSleepTimeoutInMinutes} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder settingsSleepTimeoutInMinutes(Integer settingsSleepTimeoutInMinutes) {
this.settingsSleepTimeoutInMinutes = settingsSleepTimeoutInMinutes;
this.changedFields = changedFields.add("settingsSleepTimeoutInMinutes");
return this;
}
/**
* “The welcome screen background image URL. The URL must use the HTTPS protocol and
* return a PNG image.”
*
* @param welcomeScreenBackgroundImageUrl
* value of {@code welcomeScreenBackgroundImageUrl} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder welcomeScreenBackgroundImageUrl(String welcomeScreenBackgroundImageUrl) {
this.welcomeScreenBackgroundImageUrl = welcomeScreenBackgroundImageUrl;
this.changedFields = changedFields.add("welcomeScreenBackgroundImageUrl");
return this;
}
/**
* “Indicates whether or not to Block the welcome screen from waking up
* automatically when someone enters the room.”
*
* @param welcomeScreenBlockAutomaticWakeUp
* value of {@code welcomeScreenBlockAutomaticWakeUp} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder welcomeScreenBlockAutomaticWakeUp(Boolean welcomeScreenBlockAutomaticWakeUp) {
this.welcomeScreenBlockAutomaticWakeUp = welcomeScreenBlockAutomaticWakeUp;
this.changedFields = changedFields.add("welcomeScreenBlockAutomaticWakeUp");
return this;
}
/**
* “The welcome screen meeting information shown.”
*
* @param welcomeScreenMeetingInformation
* value of {@code welcomeScreenMeetingInformation} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder welcomeScreenMeetingInformation(WelcomeScreenMeetingInformation welcomeScreenMeetingInformation) {
this.welcomeScreenMeetingInformation = welcomeScreenMeetingInformation;
this.changedFields = changedFields.add("welcomeScreenMeetingInformation");
return this;
}
public Windows10TeamGeneralConfiguration build() {
Windows10TeamGeneralConfiguration _x = new Windows10TeamGeneralConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.windows10TeamGeneralConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.version = version;
_x.assignments = assignments;
_x.deviceSettingStateSummaries = deviceSettingStateSummaries;
_x.deviceStatuses = deviceStatuses;
_x.deviceStatusOverview = deviceStatusOverview;
_x.userStatuses = userStatuses;
_x.userStatusOverview = userStatusOverview;
_x.azureOperationalInsightsBlockTelemetry = azureOperationalInsightsBlockTelemetry;
_x.azureOperationalInsightsWorkspaceId = azureOperationalInsightsWorkspaceId;
_x.azureOperationalInsightsWorkspaceKey = azureOperationalInsightsWorkspaceKey;
_x.connectAppBlockAutoLaunch = connectAppBlockAutoLaunch;
_x.maintenanceWindowBlocked = maintenanceWindowBlocked;
_x.maintenanceWindowDurationInHours = maintenanceWindowDurationInHours;
_x.maintenanceWindowStartTime = maintenanceWindowStartTime;
_x.miracastBlocked = miracastBlocked;
_x.miracastChannel = miracastChannel;
_x.miracastRequirePin = miracastRequirePin;
_x.settingsBlockMyMeetingsAndFiles = settingsBlockMyMeetingsAndFiles;
_x.settingsBlockSessionResume = settingsBlockSessionResume;
_x.settingsBlockSigninSuggestions = settingsBlockSigninSuggestions;
_x.settingsDefaultVolume = settingsDefaultVolume;
_x.settingsScreenTimeoutInMinutes = settingsScreenTimeoutInMinutes;
_x.settingsSessionTimeoutInMinutes = settingsSessionTimeoutInMinutes;
_x.settingsSleepTimeoutInMinutes = settingsSleepTimeoutInMinutes;
_x.welcomeScreenBackgroundImageUrl = welcomeScreenBackgroundImageUrl;
_x.welcomeScreenBlockAutomaticWakeUp = welcomeScreenBlockAutomaticWakeUp;
_x.welcomeScreenMeetingInformation = welcomeScreenMeetingInformation;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “Indicates whether or not to Block Azure Operational Insights.”
*
* @return property azureOperationalInsightsBlockTelemetry
*/
@Property(name="azureOperationalInsightsBlockTelemetry")
@JsonIgnore
public Optional getAzureOperationalInsightsBlockTelemetry() {
return Optional.ofNullable(azureOperationalInsightsBlockTelemetry);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* azureOperationalInsightsBlockTelemetry} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Indicates whether or not to Block Azure Operational Insights.”
*
* @param azureOperationalInsightsBlockTelemetry
* new value of {@code azureOperationalInsightsBlockTelemetry} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code azureOperationalInsightsBlockTelemetry} field changed
*/
public Windows10TeamGeneralConfiguration withAzureOperationalInsightsBlockTelemetry(Boolean azureOperationalInsightsBlockTelemetry) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("azureOperationalInsightsBlockTelemetry");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.azureOperationalInsightsBlockTelemetry = azureOperationalInsightsBlockTelemetry;
return _x;
}
/**
* “The Azure Operational Insights workspace id.”
*
* @return property azureOperationalInsightsWorkspaceId
*/
@Property(name="azureOperationalInsightsWorkspaceId")
@JsonIgnore
public Optional getAzureOperationalInsightsWorkspaceId() {
return Optional.ofNullable(azureOperationalInsightsWorkspaceId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* azureOperationalInsightsWorkspaceId} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The Azure Operational Insights workspace id.”
*
* @param azureOperationalInsightsWorkspaceId
* new value of {@code azureOperationalInsightsWorkspaceId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code azureOperationalInsightsWorkspaceId} field changed
*/
public Windows10TeamGeneralConfiguration withAzureOperationalInsightsWorkspaceId(String azureOperationalInsightsWorkspaceId) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("azureOperationalInsightsWorkspaceId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.azureOperationalInsightsWorkspaceId = azureOperationalInsightsWorkspaceId;
return _x;
}
/**
* “The Azure Operational Insights Workspace key.”
*
* @return property azureOperationalInsightsWorkspaceKey
*/
@Property(name="azureOperationalInsightsWorkspaceKey")
@JsonIgnore
public Optional getAzureOperationalInsightsWorkspaceKey() {
return Optional.ofNullable(azureOperationalInsightsWorkspaceKey);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* azureOperationalInsightsWorkspaceKey} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “The Azure Operational Insights Workspace key.”
*
* @param azureOperationalInsightsWorkspaceKey
* new value of {@code azureOperationalInsightsWorkspaceKey} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code azureOperationalInsightsWorkspaceKey} field changed
*/
public Windows10TeamGeneralConfiguration withAzureOperationalInsightsWorkspaceKey(String azureOperationalInsightsWorkspaceKey) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("azureOperationalInsightsWorkspaceKey");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.azureOperationalInsightsWorkspaceKey = azureOperationalInsightsWorkspaceKey;
return _x;
}
/**
* “Specifies whether to automatically launch the Connect app whenever a projection
* is initiated.”
*
* @return property connectAppBlockAutoLaunch
*/
@Property(name="connectAppBlockAutoLaunch")
@JsonIgnore
public Optional getConnectAppBlockAutoLaunch() {
return Optional.ofNullable(connectAppBlockAutoLaunch);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* connectAppBlockAutoLaunch} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Specifies whether to automatically launch the Connect app whenever a projection
* is initiated.”
*
* @param connectAppBlockAutoLaunch
* new value of {@code connectAppBlockAutoLaunch} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code connectAppBlockAutoLaunch} field changed
*/
public Windows10TeamGeneralConfiguration withConnectAppBlockAutoLaunch(Boolean connectAppBlockAutoLaunch) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("connectAppBlockAutoLaunch");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.connectAppBlockAutoLaunch = connectAppBlockAutoLaunch;
return _x;
}
/**
* “Indicates whether or not to Block setting a maintenance window for device
* updates.”
*
* @return property maintenanceWindowBlocked
*/
@Property(name="maintenanceWindowBlocked")
@JsonIgnore
public Optional getMaintenanceWindowBlocked() {
return Optional.ofNullable(maintenanceWindowBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* maintenanceWindowBlocked} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to Block setting a maintenance window for device
* updates.”
*
* @param maintenanceWindowBlocked
* new value of {@code maintenanceWindowBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code maintenanceWindowBlocked} field changed
*/
public Windows10TeamGeneralConfiguration withMaintenanceWindowBlocked(Boolean maintenanceWindowBlocked) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("maintenanceWindowBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.maintenanceWindowBlocked = maintenanceWindowBlocked;
return _x;
}
/**
* “Maintenance window duration for device updates. Valid values 0 to 5”
*
* @return property maintenanceWindowDurationInHours
*/
@Property(name="maintenanceWindowDurationInHours")
@JsonIgnore
public Optional getMaintenanceWindowDurationInHours() {
return Optional.ofNullable(maintenanceWindowDurationInHours);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* maintenanceWindowDurationInHours} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Maintenance window duration for device updates. Valid values 0 to 5”
*
* @param maintenanceWindowDurationInHours
* new value of {@code maintenanceWindowDurationInHours} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code maintenanceWindowDurationInHours} field changed
*/
public Windows10TeamGeneralConfiguration withMaintenanceWindowDurationInHours(Integer maintenanceWindowDurationInHours) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("maintenanceWindowDurationInHours");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.maintenanceWindowDurationInHours = maintenanceWindowDurationInHours;
return _x;
}
/**
* “Maintenance window start time for device updates.”
*
* @return property maintenanceWindowStartTime
*/
@Property(name="maintenanceWindowStartTime")
@JsonIgnore
public Optional getMaintenanceWindowStartTime() {
return Optional.ofNullable(maintenanceWindowStartTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* maintenanceWindowStartTime} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Maintenance window start time for device updates.”
*
* @param maintenanceWindowStartTime
* new value of {@code maintenanceWindowStartTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code maintenanceWindowStartTime} field changed
*/
public Windows10TeamGeneralConfiguration withMaintenanceWindowStartTime(LocalTime maintenanceWindowStartTime) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("maintenanceWindowStartTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.maintenanceWindowStartTime = maintenanceWindowStartTime;
return _x;
}
/**
* “Indicates whether or not to Block wireless projection.”
*
* @return property miracastBlocked
*/
@Property(name="miracastBlocked")
@JsonIgnore
public Optional getMiracastBlocked() {
return Optional.ofNullable(miracastBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code miracastBlocked}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether or not to Block wireless projection.”
*
* @param miracastBlocked
* new value of {@code miracastBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code miracastBlocked} field changed
*/
public Windows10TeamGeneralConfiguration withMiracastBlocked(Boolean miracastBlocked) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("miracastBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.miracastBlocked = miracastBlocked;
return _x;
}
/**
* “The channel.”
*
* @return property miracastChannel
*/
@Property(name="miracastChannel")
@JsonIgnore
public Optional getMiracastChannel() {
return Optional.ofNullable(miracastChannel);
}
/**
* Returns an immutable copy of {@code this} with just the {@code miracastChannel}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The channel.”
*
* @param miracastChannel
* new value of {@code miracastChannel} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code miracastChannel} field changed
*/
public Windows10TeamGeneralConfiguration withMiracastChannel(MiracastChannel miracastChannel) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("miracastChannel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.miracastChannel = miracastChannel;
return _x;
}
/**
* “Indicates whether or not to require a pin for wireless projection.”
*
* @return property miracastRequirePin
*/
@Property(name="miracastRequirePin")
@JsonIgnore
public Optional getMiracastRequirePin() {
return Optional.ofNullable(miracastRequirePin);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* miracastRequirePin} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Indicates whether or not to require a pin for wireless projection.”
*
* @param miracastRequirePin
* new value of {@code miracastRequirePin} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code miracastRequirePin} field changed
*/
public Windows10TeamGeneralConfiguration withMiracastRequirePin(Boolean miracastRequirePin) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("miracastRequirePin");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.miracastRequirePin = miracastRequirePin;
return _x;
}
/**
* “Specifies whether to disable the "My meetings and files" feature in the Start
* menu, which shows the signed-in user's meetings and files from Office 365.”
*
* @return property settingsBlockMyMeetingsAndFiles
*/
@Property(name="settingsBlockMyMeetingsAndFiles")
@JsonIgnore
public Optional getSettingsBlockMyMeetingsAndFiles() {
return Optional.ofNullable(settingsBlockMyMeetingsAndFiles);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* settingsBlockMyMeetingsAndFiles} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Specifies whether to disable the "My meetings and files" feature in the Start
* menu, which shows the signed-in user's meetings and files from Office 365.”
*
* @param settingsBlockMyMeetingsAndFiles
* new value of {@code settingsBlockMyMeetingsAndFiles} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code settingsBlockMyMeetingsAndFiles} field changed
*/
public Windows10TeamGeneralConfiguration withSettingsBlockMyMeetingsAndFiles(Boolean settingsBlockMyMeetingsAndFiles) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("settingsBlockMyMeetingsAndFiles");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.settingsBlockMyMeetingsAndFiles = settingsBlockMyMeetingsAndFiles;
return _x;
}
/**
* “Specifies whether to allow the ability to resume a session when the session
* times out.”
*
* @return property settingsBlockSessionResume
*/
@Property(name="settingsBlockSessionResume")
@JsonIgnore
public Optional getSettingsBlockSessionResume() {
return Optional.ofNullable(settingsBlockSessionResume);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* settingsBlockSessionResume} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Specifies whether to allow the ability to resume a session when the session
* times out.”
*
* @param settingsBlockSessionResume
* new value of {@code settingsBlockSessionResume} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code settingsBlockSessionResume} field changed
*/
public Windows10TeamGeneralConfiguration withSettingsBlockSessionResume(Boolean settingsBlockSessionResume) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("settingsBlockSessionResume");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.settingsBlockSessionResume = settingsBlockSessionResume;
return _x;
}
/**
* “Specifies whether to disable auto-populating of the sign-in dialog with invitees
* from scheduled meetings.”
*
* @return property settingsBlockSigninSuggestions
*/
@Property(name="settingsBlockSigninSuggestions")
@JsonIgnore
public Optional getSettingsBlockSigninSuggestions() {
return Optional.ofNullable(settingsBlockSigninSuggestions);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* settingsBlockSigninSuggestions} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Specifies whether to disable auto-populating of the sign-in dialog with invitees
* from scheduled meetings.”
*
* @param settingsBlockSigninSuggestions
* new value of {@code settingsBlockSigninSuggestions} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code settingsBlockSigninSuggestions} field changed
*/
public Windows10TeamGeneralConfiguration withSettingsBlockSigninSuggestions(Boolean settingsBlockSigninSuggestions) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("settingsBlockSigninSuggestions");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.settingsBlockSigninSuggestions = settingsBlockSigninSuggestions;
return _x;
}
/**
* “Specifies the default volume value for a new session. Permitted values are 0-100
* . The default is 45. Valid values 0 to 100”
*
* @return property settingsDefaultVolume
*/
@Property(name="settingsDefaultVolume")
@JsonIgnore
public Optional getSettingsDefaultVolume() {
return Optional.ofNullable(settingsDefaultVolume);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* settingsDefaultVolume} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Specifies the default volume value for a new session. Permitted values are 0-100
* . The default is 45. Valid values 0 to 100”
*
* @param settingsDefaultVolume
* new value of {@code settingsDefaultVolume} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code settingsDefaultVolume} field changed
*/
public Windows10TeamGeneralConfiguration withSettingsDefaultVolume(Integer settingsDefaultVolume) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("settingsDefaultVolume");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.settingsDefaultVolume = settingsDefaultVolume;
return _x;
}
/**
* “Specifies the number of minutes until the Hub screen turns off.”
*
* @return property settingsScreenTimeoutInMinutes
*/
@Property(name="settingsScreenTimeoutInMinutes")
@JsonIgnore
public Optional getSettingsScreenTimeoutInMinutes() {
return Optional.ofNullable(settingsScreenTimeoutInMinutes);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* settingsScreenTimeoutInMinutes} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Specifies the number of minutes until the Hub screen turns off.”
*
* @param settingsScreenTimeoutInMinutes
* new value of {@code settingsScreenTimeoutInMinutes} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code settingsScreenTimeoutInMinutes} field changed
*/
public Windows10TeamGeneralConfiguration withSettingsScreenTimeoutInMinutes(Integer settingsScreenTimeoutInMinutes) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("settingsScreenTimeoutInMinutes");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.settingsScreenTimeoutInMinutes = settingsScreenTimeoutInMinutes;
return _x;
}
/**
* “Specifies the number of minutes until the session times out.”
*
* @return property settingsSessionTimeoutInMinutes
*/
@Property(name="settingsSessionTimeoutInMinutes")
@JsonIgnore
public Optional getSettingsSessionTimeoutInMinutes() {
return Optional.ofNullable(settingsSessionTimeoutInMinutes);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* settingsSessionTimeoutInMinutes} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Specifies the number of minutes until the session times out.”
*
* @param settingsSessionTimeoutInMinutes
* new value of {@code settingsSessionTimeoutInMinutes} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code settingsSessionTimeoutInMinutes} field changed
*/
public Windows10TeamGeneralConfiguration withSettingsSessionTimeoutInMinutes(Integer settingsSessionTimeoutInMinutes) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("settingsSessionTimeoutInMinutes");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.settingsSessionTimeoutInMinutes = settingsSessionTimeoutInMinutes;
return _x;
}
/**
* “Specifies the number of minutes until the Hub enters sleep mode.”
*
* @return property settingsSleepTimeoutInMinutes
*/
@Property(name="settingsSleepTimeoutInMinutes")
@JsonIgnore
public Optional getSettingsSleepTimeoutInMinutes() {
return Optional.ofNullable(settingsSleepTimeoutInMinutes);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* settingsSleepTimeoutInMinutes} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Specifies the number of minutes until the Hub enters sleep mode.”
*
* @param settingsSleepTimeoutInMinutes
* new value of {@code settingsSleepTimeoutInMinutes} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code settingsSleepTimeoutInMinutes} field changed
*/
public Windows10TeamGeneralConfiguration withSettingsSleepTimeoutInMinutes(Integer settingsSleepTimeoutInMinutes) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("settingsSleepTimeoutInMinutes");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.settingsSleepTimeoutInMinutes = settingsSleepTimeoutInMinutes;
return _x;
}
/**
* “The welcome screen background image URL. The URL must use the HTTPS protocol and
* return a PNG image.”
*
* @return property welcomeScreenBackgroundImageUrl
*/
@Property(name="welcomeScreenBackgroundImageUrl")
@JsonIgnore
public Optional getWelcomeScreenBackgroundImageUrl() {
return Optional.ofNullable(welcomeScreenBackgroundImageUrl);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* welcomeScreenBackgroundImageUrl} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The welcome screen background image URL. The URL must use the HTTPS protocol and
* return a PNG image.”
*
* @param welcomeScreenBackgroundImageUrl
* new value of {@code welcomeScreenBackgroundImageUrl} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code welcomeScreenBackgroundImageUrl} field changed
*/
public Windows10TeamGeneralConfiguration withWelcomeScreenBackgroundImageUrl(String welcomeScreenBackgroundImageUrl) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("welcomeScreenBackgroundImageUrl");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.welcomeScreenBackgroundImageUrl = welcomeScreenBackgroundImageUrl;
return _x;
}
/**
* “Indicates whether or not to Block the welcome screen from waking up
* automatically when someone enters the room.”
*
* @return property welcomeScreenBlockAutomaticWakeUp
*/
@Property(name="welcomeScreenBlockAutomaticWakeUp")
@JsonIgnore
public Optional getWelcomeScreenBlockAutomaticWakeUp() {
return Optional.ofNullable(welcomeScreenBlockAutomaticWakeUp);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* welcomeScreenBlockAutomaticWakeUp} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates whether or not to Block the welcome screen from waking up
* automatically when someone enters the room.”
*
* @param welcomeScreenBlockAutomaticWakeUp
* new value of {@code welcomeScreenBlockAutomaticWakeUp} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code welcomeScreenBlockAutomaticWakeUp} field changed
*/
public Windows10TeamGeneralConfiguration withWelcomeScreenBlockAutomaticWakeUp(Boolean welcomeScreenBlockAutomaticWakeUp) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("welcomeScreenBlockAutomaticWakeUp");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.welcomeScreenBlockAutomaticWakeUp = welcomeScreenBlockAutomaticWakeUp;
return _x;
}
/**
* “The welcome screen meeting information shown.”
*
* @return property welcomeScreenMeetingInformation
*/
@Property(name="welcomeScreenMeetingInformation")
@JsonIgnore
public Optional getWelcomeScreenMeetingInformation() {
return Optional.ofNullable(welcomeScreenMeetingInformation);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* welcomeScreenMeetingInformation} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The welcome screen meeting information shown.”
*
* @param welcomeScreenMeetingInformation
* new value of {@code welcomeScreenMeetingInformation} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code welcomeScreenMeetingInformation} field changed
*/
public Windows10TeamGeneralConfiguration withWelcomeScreenMeetingInformation(WelcomeScreenMeetingInformation welcomeScreenMeetingInformation) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("welcomeScreenMeetingInformation");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windows10TeamGeneralConfiguration");
_x.welcomeScreenMeetingInformation = welcomeScreenMeetingInformation;
return _x;
}
public Windows10TeamGeneralConfiguration withUnmappedField(String name, Object value) {
Windows10TeamGeneralConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Windows10TeamGeneralConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Windows10TeamGeneralConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Windows10TeamGeneralConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private Windows10TeamGeneralConfiguration _copy() {
Windows10TeamGeneralConfiguration _x = new Windows10TeamGeneralConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.version = version;
_x.assignments = assignments;
_x.deviceSettingStateSummaries = deviceSettingStateSummaries;
_x.deviceStatuses = deviceStatuses;
_x.deviceStatusOverview = deviceStatusOverview;
_x.userStatuses = userStatuses;
_x.userStatusOverview = userStatusOverview;
_x.azureOperationalInsightsBlockTelemetry = azureOperationalInsightsBlockTelemetry;
_x.azureOperationalInsightsWorkspaceId = azureOperationalInsightsWorkspaceId;
_x.azureOperationalInsightsWorkspaceKey = azureOperationalInsightsWorkspaceKey;
_x.connectAppBlockAutoLaunch = connectAppBlockAutoLaunch;
_x.maintenanceWindowBlocked = maintenanceWindowBlocked;
_x.maintenanceWindowDurationInHours = maintenanceWindowDurationInHours;
_x.maintenanceWindowStartTime = maintenanceWindowStartTime;
_x.miracastBlocked = miracastBlocked;
_x.miracastChannel = miracastChannel;
_x.miracastRequirePin = miracastRequirePin;
_x.settingsBlockMyMeetingsAndFiles = settingsBlockMyMeetingsAndFiles;
_x.settingsBlockSessionResume = settingsBlockSessionResume;
_x.settingsBlockSigninSuggestions = settingsBlockSigninSuggestions;
_x.settingsDefaultVolume = settingsDefaultVolume;
_x.settingsScreenTimeoutInMinutes = settingsScreenTimeoutInMinutes;
_x.settingsSessionTimeoutInMinutes = settingsSessionTimeoutInMinutes;
_x.settingsSleepTimeoutInMinutes = settingsSleepTimeoutInMinutes;
_x.welcomeScreenBackgroundImageUrl = welcomeScreenBackgroundImageUrl;
_x.welcomeScreenBlockAutomaticWakeUp = welcomeScreenBlockAutomaticWakeUp;
_x.welcomeScreenMeetingInformation = welcomeScreenMeetingInformation;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Windows10TeamGeneralConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("assignments=");
b.append(this.assignments);
b.append(", ");
b.append("deviceSettingStateSummaries=");
b.append(this.deviceSettingStateSummaries);
b.append(", ");
b.append("deviceStatuses=");
b.append(this.deviceStatuses);
b.append(", ");
b.append("deviceStatusOverview=");
b.append(this.deviceStatusOverview);
b.append(", ");
b.append("userStatuses=");
b.append(this.userStatuses);
b.append(", ");
b.append("userStatusOverview=");
b.append(this.userStatusOverview);
b.append(", ");
b.append("azureOperationalInsightsBlockTelemetry=");
b.append(this.azureOperationalInsightsBlockTelemetry);
b.append(", ");
b.append("azureOperationalInsightsWorkspaceId=");
b.append(this.azureOperationalInsightsWorkspaceId);
b.append(", ");
b.append("azureOperationalInsightsWorkspaceKey=");
b.append(this.azureOperationalInsightsWorkspaceKey);
b.append(", ");
b.append("connectAppBlockAutoLaunch=");
b.append(this.connectAppBlockAutoLaunch);
b.append(", ");
b.append("maintenanceWindowBlocked=");
b.append(this.maintenanceWindowBlocked);
b.append(", ");
b.append("maintenanceWindowDurationInHours=");
b.append(this.maintenanceWindowDurationInHours);
b.append(", ");
b.append("maintenanceWindowStartTime=");
b.append(this.maintenanceWindowStartTime);
b.append(", ");
b.append("miracastBlocked=");
b.append(this.miracastBlocked);
b.append(", ");
b.append("miracastChannel=");
b.append(this.miracastChannel);
b.append(", ");
b.append("miracastRequirePin=");
b.append(this.miracastRequirePin);
b.append(", ");
b.append("settingsBlockMyMeetingsAndFiles=");
b.append(this.settingsBlockMyMeetingsAndFiles);
b.append(", ");
b.append("settingsBlockSessionResume=");
b.append(this.settingsBlockSessionResume);
b.append(", ");
b.append("settingsBlockSigninSuggestions=");
b.append(this.settingsBlockSigninSuggestions);
b.append(", ");
b.append("settingsDefaultVolume=");
b.append(this.settingsDefaultVolume);
b.append(", ");
b.append("settingsScreenTimeoutInMinutes=");
b.append(this.settingsScreenTimeoutInMinutes);
b.append(", ");
b.append("settingsSessionTimeoutInMinutes=");
b.append(this.settingsSessionTimeoutInMinutes);
b.append(", ");
b.append("settingsSleepTimeoutInMinutes=");
b.append(this.settingsSleepTimeoutInMinutes);
b.append(", ");
b.append("welcomeScreenBackgroundImageUrl=");
b.append(this.welcomeScreenBackgroundImageUrl);
b.append(", ");
b.append("welcomeScreenBlockAutomaticWakeUp=");
b.append(this.welcomeScreenBlockAutomaticWakeUp);
b.append(", ");
b.append("welcomeScreenMeetingInformation=");
b.append(this.welcomeScreenMeetingInformation);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}