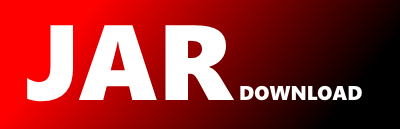
odata.msgraph.client.entity.WindowsAppX Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.complex.MimeContent;
import odata.msgraph.client.complex.WindowsMinimumOperatingSystem;
import odata.msgraph.client.enums.MobileAppPublishingState;
import odata.msgraph.client.enums.WindowsArchitecture;
/**
* “Contains properties and inherited properties for Windows AppX Line Of Business
* apps.”
*/@JsonPropertyOrder({
"@odata.type",
"applicableArchitectures",
"identityName",
"identityPublisherHash",
"identityResourceIdentifier",
"identityVersion",
"isBundle",
"minimumSupportedOperatingSystem"})
@JsonInclude(Include.NON_NULL)
public class WindowsAppX extends MobileLobApp implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.windowsAppX";
}
@JsonProperty("applicableArchitectures")
protected WindowsArchitecture applicableArchitectures;
@JsonProperty("identityName")
protected String identityName;
@JsonProperty("identityPublisherHash")
protected String identityPublisherHash;
@JsonProperty("identityResourceIdentifier")
protected String identityResourceIdentifier;
@JsonProperty("identityVersion")
protected String identityVersion;
@JsonProperty("isBundle")
protected Boolean isBundle;
@JsonProperty("minimumSupportedOperatingSystem")
protected WindowsMinimumOperatingSystem minimumSupportedOperatingSystem;
protected WindowsAppX() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderWindowsAppX() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private String developer;
private String displayName;
private String informationUrl;
private Boolean isFeatured;
private MimeContent largeIcon;
private OffsetDateTime lastModifiedDateTime;
private String notes;
private String owner;
private String privacyInformationUrl;
private String publisher;
private MobileAppPublishingState publishingState;
private List assignments;
private String committedContentVersion;
private String fileName;
private Long size;
private List contentVersions;
private WindowsArchitecture applicableArchitectures;
private String identityName;
private String identityPublisherHash;
private String identityResourceIdentifier;
private String identityVersion;
private Boolean isBundle;
private WindowsMinimumOperatingSystem minimumSupportedOperatingSystem;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder developer(String developer) {
this.developer = developer;
this.changedFields = changedFields.add("developer");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder informationUrl(String informationUrl) {
this.informationUrl = informationUrl;
this.changedFields = changedFields.add("informationUrl");
return this;
}
public Builder isFeatured(Boolean isFeatured) {
this.isFeatured = isFeatured;
this.changedFields = changedFields.add("isFeatured");
return this;
}
public Builder largeIcon(MimeContent largeIcon) {
this.largeIcon = largeIcon;
this.changedFields = changedFields.add("largeIcon");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder notes(String notes) {
this.notes = notes;
this.changedFields = changedFields.add("notes");
return this;
}
public Builder owner(String owner) {
this.owner = owner;
this.changedFields = changedFields.add("owner");
return this;
}
public Builder privacyInformationUrl(String privacyInformationUrl) {
this.privacyInformationUrl = privacyInformationUrl;
this.changedFields = changedFields.add("privacyInformationUrl");
return this;
}
public Builder publisher(String publisher) {
this.publisher = publisher;
this.changedFields = changedFields.add("publisher");
return this;
}
public Builder publishingState(MobileAppPublishingState publishingState) {
this.publishingState = publishingState;
this.changedFields = changedFields.add("publishingState");
return this;
}
public Builder assignments(List assignments) {
this.assignments = assignments;
this.changedFields = changedFields.add("assignments");
return this;
}
public Builder assignments(MobileAppAssignment... assignments) {
return assignments(Arrays.asList(assignments));
}
public Builder committedContentVersion(String committedContentVersion) {
this.committedContentVersion = committedContentVersion;
this.changedFields = changedFields.add("committedContentVersion");
return this;
}
public Builder fileName(String fileName) {
this.fileName = fileName;
this.changedFields = changedFields.add("fileName");
return this;
}
public Builder size(Long size) {
this.size = size;
this.changedFields = changedFields.add("size");
return this;
}
public Builder contentVersions(List contentVersions) {
this.contentVersions = contentVersions;
this.changedFields = changedFields.add("contentVersions");
return this;
}
public Builder contentVersions(MobileAppContent... contentVersions) {
return contentVersions(Arrays.asList(contentVersions));
}
/**
* “The Windows architecture(s) on which this app can run. Possible values are: `
* none`, `x86`, `x64`, `arm`, `neutral`; default value is `none`.”
*
* @param applicableArchitectures
* value of {@code applicableArchitectures} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder applicableArchitectures(WindowsArchitecture applicableArchitectures) {
this.applicableArchitectures = applicableArchitectures;
this.changedFields = changedFields.add("applicableArchitectures");
return this;
}
/**
* “The identity name of the uploaded app package. For example: "Contoso.DemoApp".”
*
* @param identityName
* value of {@code identityName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder identityName(String identityName) {
this.identityName = identityName;
this.changedFields = changedFields.add("identityName");
return this;
}
/**
* “The identity publisher hash of the uploaded app package. This is the hash of the
* publisher from the manifest. For example: "AB82CD0XYZ".”
*
* @param identityPublisherHash
* value of {@code identityPublisherHash} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder identityPublisherHash(String identityPublisherHash) {
this.identityPublisherHash = identityPublisherHash;
this.changedFields = changedFields.add("identityPublisherHash");
return this;
}
/**
* “The identity resource identifier of the uploaded app package. For example: "
* TestResourceId".”
*
* @param identityResourceIdentifier
* value of {@code identityResourceIdentifier} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder identityResourceIdentifier(String identityResourceIdentifier) {
this.identityResourceIdentifier = identityResourceIdentifier;
this.changedFields = changedFields.add("identityResourceIdentifier");
return this;
}
/**
* “The identity version of the uploaded app package. For example: "1.0.0.0".”
*
* @param identityVersion
* value of {@code identityVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder identityVersion(String identityVersion) {
this.identityVersion = identityVersion;
this.changedFields = changedFields.add("identityVersion");
return this;
}
/**
* “When TRUE, indicates that the app is a bundle. When FALSE, indicates that the
* app is not a bundle. By default, property is set to FALSE.”
*
* @param isBundle
* value of {@code isBundle} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder isBundle(Boolean isBundle) {
this.isBundle = isBundle;
this.changedFields = changedFields.add("isBundle");
return this;
}
/**
* “The value for the minimum applicable operating system. Valid values for a
* WindowsAppX app include `v8_0`, `v8_1` and `v10_0`. If the app is a bundle, the
* minimum supported OS has to be at least `v8_1`.”
*
* @param minimumSupportedOperatingSystem
* value of {@code minimumSupportedOperatingSystem} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder minimumSupportedOperatingSystem(WindowsMinimumOperatingSystem minimumSupportedOperatingSystem) {
this.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
this.changedFields = changedFields.add("minimumSupportedOperatingSystem");
return this;
}
public WindowsAppX build() {
WindowsAppX _x = new WindowsAppX();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.windowsAppX";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.developer = developer;
_x.displayName = displayName;
_x.informationUrl = informationUrl;
_x.isFeatured = isFeatured;
_x.largeIcon = largeIcon;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.notes = notes;
_x.owner = owner;
_x.privacyInformationUrl = privacyInformationUrl;
_x.publisher = publisher;
_x.publishingState = publishingState;
_x.assignments = assignments;
_x.committedContentVersion = committedContentVersion;
_x.fileName = fileName;
_x.size = size;
_x.contentVersions = contentVersions;
_x.applicableArchitectures = applicableArchitectures;
_x.identityName = identityName;
_x.identityPublisherHash = identityPublisherHash;
_x.identityResourceIdentifier = identityResourceIdentifier;
_x.identityVersion = identityVersion;
_x.isBundle = isBundle;
_x.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “The Windows architecture(s) on which this app can run. Possible values are: `
* none`, `x86`, `x64`, `arm`, `neutral`; default value is `none`.”
*
* @return property applicableArchitectures
*/
@Property(name="applicableArchitectures")
@JsonIgnore
public Optional getApplicableArchitectures() {
return Optional.ofNullable(applicableArchitectures);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* applicableArchitectures} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The Windows architecture(s) on which this app can run. Possible values are: `
* none`, `x86`, `x64`, `arm`, `neutral`; default value is `none`.”
*
* @param applicableArchitectures
* new value of {@code applicableArchitectures} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code applicableArchitectures} field changed
*/
public WindowsAppX withApplicableArchitectures(WindowsArchitecture applicableArchitectures) {
WindowsAppX _x = _copy();
_x.changedFields = changedFields.add("applicableArchitectures");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsAppX");
_x.applicableArchitectures = applicableArchitectures;
return _x;
}
/**
* “The identity name of the uploaded app package. For example: "Contoso.DemoApp".”
*
* @return property identityName
*/
@Property(name="identityName")
@JsonIgnore
public Optional getIdentityName() {
return Optional.ofNullable(identityName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code identityName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The identity name of the uploaded app package. For example: "Contoso.DemoApp".”
*
* @param identityName
* new value of {@code identityName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code identityName} field changed
*/
public WindowsAppX withIdentityName(String identityName) {
WindowsAppX _x = _copy();
_x.changedFields = changedFields.add("identityName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsAppX");
_x.identityName = identityName;
return _x;
}
/**
* “The identity publisher hash of the uploaded app package. This is the hash of the
* publisher from the manifest. For example: "AB82CD0XYZ".”
*
* @return property identityPublisherHash
*/
@Property(name="identityPublisherHash")
@JsonIgnore
public Optional getIdentityPublisherHash() {
return Optional.ofNullable(identityPublisherHash);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* identityPublisherHash} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The identity publisher hash of the uploaded app package. This is the hash of the
* publisher from the manifest. For example: "AB82CD0XYZ".”
*
* @param identityPublisherHash
* new value of {@code identityPublisherHash} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code identityPublisherHash} field changed
*/
public WindowsAppX withIdentityPublisherHash(String identityPublisherHash) {
WindowsAppX _x = _copy();
_x.changedFields = changedFields.add("identityPublisherHash");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsAppX");
_x.identityPublisherHash = identityPublisherHash;
return _x;
}
/**
* “The identity resource identifier of the uploaded app package. For example: "
* TestResourceId".”
*
* @return property identityResourceIdentifier
*/
@Property(name="identityResourceIdentifier")
@JsonIgnore
public Optional getIdentityResourceIdentifier() {
return Optional.ofNullable(identityResourceIdentifier);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* identityResourceIdentifier} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The identity resource identifier of the uploaded app package. For example: "
* TestResourceId".”
*
* @param identityResourceIdentifier
* new value of {@code identityResourceIdentifier} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code identityResourceIdentifier} field changed
*/
public WindowsAppX withIdentityResourceIdentifier(String identityResourceIdentifier) {
WindowsAppX _x = _copy();
_x.changedFields = changedFields.add("identityResourceIdentifier");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsAppX");
_x.identityResourceIdentifier = identityResourceIdentifier;
return _x;
}
/**
* “The identity version of the uploaded app package. For example: "1.0.0.0".”
*
* @return property identityVersion
*/
@Property(name="identityVersion")
@JsonIgnore
public Optional getIdentityVersion() {
return Optional.ofNullable(identityVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code identityVersion}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The identity version of the uploaded app package. For example: "1.0.0.0".”
*
* @param identityVersion
* new value of {@code identityVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code identityVersion} field changed
*/
public WindowsAppX withIdentityVersion(String identityVersion) {
WindowsAppX _x = _copy();
_x.changedFields = changedFields.add("identityVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsAppX");
_x.identityVersion = identityVersion;
return _x;
}
/**
* “When TRUE, indicates that the app is a bundle. When FALSE, indicates that the
* app is not a bundle. By default, property is set to FALSE.”
*
* @return property isBundle
*/
@Property(name="isBundle")
@JsonIgnore
public Optional getIsBundle() {
return Optional.ofNullable(isBundle);
}
/**
* Returns an immutable copy of {@code this} with just the {@code isBundle} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates that the app is a bundle. When FALSE, indicates that the
* app is not a bundle. By default, property is set to FALSE.”
*
* @param isBundle
* new value of {@code isBundle} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code isBundle} field changed
*/
public WindowsAppX withIsBundle(Boolean isBundle) {
WindowsAppX _x = _copy();
_x.changedFields = changedFields.add("isBundle");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsAppX");
_x.isBundle = isBundle;
return _x;
}
/**
* “The value for the minimum applicable operating system. Valid values for a
* WindowsAppX app include `v8_0`, `v8_1` and `v10_0`. If the app is a bundle, the
* minimum supported OS has to be at least `v8_1`.”
*
* @return property minimumSupportedOperatingSystem
*/
@Property(name="minimumSupportedOperatingSystem")
@JsonIgnore
public Optional getMinimumSupportedOperatingSystem() {
return Optional.ofNullable(minimumSupportedOperatingSystem);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* minimumSupportedOperatingSystem} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The value for the minimum applicable operating system. Valid values for a
* WindowsAppX app include `v8_0`, `v8_1` and `v10_0`. If the app is a bundle, the
* minimum supported OS has to be at least `v8_1`.”
*
* @param minimumSupportedOperatingSystem
* new value of {@code minimumSupportedOperatingSystem} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code minimumSupportedOperatingSystem} field changed
*/
public WindowsAppX withMinimumSupportedOperatingSystem(WindowsMinimumOperatingSystem minimumSupportedOperatingSystem) {
WindowsAppX _x = _copy();
_x.changedFields = changedFields.add("minimumSupportedOperatingSystem");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsAppX");
_x.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
return _x;
}
public WindowsAppX withUnmappedField(String name, Object value) {
WindowsAppX _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsAppX patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
WindowsAppX _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsAppX put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
WindowsAppX _x = _copy();
_x.changedFields = null;
return _x;
}
private WindowsAppX _copy() {
WindowsAppX _x = new WindowsAppX();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.developer = developer;
_x.displayName = displayName;
_x.informationUrl = informationUrl;
_x.isFeatured = isFeatured;
_x.largeIcon = largeIcon;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.notes = notes;
_x.owner = owner;
_x.privacyInformationUrl = privacyInformationUrl;
_x.publisher = publisher;
_x.publishingState = publishingState;
_x.assignments = assignments;
_x.committedContentVersion = committedContentVersion;
_x.fileName = fileName;
_x.size = size;
_x.contentVersions = contentVersions;
_x.applicableArchitectures = applicableArchitectures;
_x.identityName = identityName;
_x.identityPublisherHash = identityPublisherHash;
_x.identityResourceIdentifier = identityResourceIdentifier;
_x.identityVersion = identityVersion;
_x.isBundle = isBundle;
_x.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("WindowsAppX[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("developer=");
b.append(this.developer);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("informationUrl=");
b.append(this.informationUrl);
b.append(", ");
b.append("isFeatured=");
b.append(this.isFeatured);
b.append(", ");
b.append("largeIcon=");
b.append(this.largeIcon);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("notes=");
b.append(this.notes);
b.append(", ");
b.append("owner=");
b.append(this.owner);
b.append(", ");
b.append("privacyInformationUrl=");
b.append(this.privacyInformationUrl);
b.append(", ");
b.append("publisher=");
b.append(this.publisher);
b.append(", ");
b.append("publishingState=");
b.append(this.publishingState);
b.append(", ");
b.append("assignments=");
b.append(this.assignments);
b.append(", ");
b.append("committedContentVersion=");
b.append(this.committedContentVersion);
b.append(", ");
b.append("fileName=");
b.append(this.fileName);
b.append(", ");
b.append("size=");
b.append(this.size);
b.append(", ");
b.append("contentVersions=");
b.append(this.contentVersions);
b.append(", ");
b.append("applicableArchitectures=");
b.append(this.applicableArchitectures);
b.append(", ");
b.append("identityName=");
b.append(this.identityName);
b.append(", ");
b.append("identityPublisherHash=");
b.append(this.identityPublisherHash);
b.append(", ");
b.append("identityResourceIdentifier=");
b.append(this.identityResourceIdentifier);
b.append(", ");
b.append("identityVersion=");
b.append(this.identityVersion);
b.append(", ");
b.append("isBundle=");
b.append(this.isBundle);
b.append(", ");
b.append("minimumSupportedOperatingSystem=");
b.append(this.minimumSupportedOperatingSystem);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}