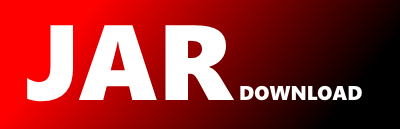
odata.msgraph.client.entity.WindowsInformationProtection Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.UUID;
import odata.msgraph.client.complex.WindowsInformationProtectionApp;
import odata.msgraph.client.complex.WindowsInformationProtectionDataRecoveryCertificate;
import odata.msgraph.client.complex.WindowsInformationProtectionIPRangeCollection;
import odata.msgraph.client.complex.WindowsInformationProtectionProxiedDomainCollection;
import odata.msgraph.client.complex.WindowsInformationProtectionResourceCollection;
import odata.msgraph.client.entity.collection.request.TargetedManagedAppPolicyAssignmentCollectionRequest;
import odata.msgraph.client.entity.collection.request.WindowsInformationProtectionAppLockerFileCollectionRequest;
import odata.msgraph.client.enums.WindowsInformationProtectionEnforcementLevel;
/**
* “Policy for Windows information protection to configure detailed management
* settings”
*/@JsonPropertyOrder({
"@odata.type",
"azureRightsManagementServicesAllowed",
"dataRecoveryCertificate",
"enforcementLevel",
"enterpriseDomain",
"enterpriseInternalProxyServers",
"enterpriseIPRanges",
"enterpriseIPRangesAreAuthoritative",
"enterpriseNetworkDomainNames",
"enterpriseProtectedDomainNames",
"enterpriseProxiedDomains",
"enterpriseProxyServers",
"enterpriseProxyServersAreAuthoritative",
"exemptApps",
"iconsVisible",
"indexingEncryptedStoresOrItemsBlocked",
"isAssigned",
"neutralDomainResources",
"protectedApps",
"protectionUnderLockConfigRequired",
"revokeOnUnenrollDisabled",
"rightsManagementServicesTemplateId",
"smbAutoEncryptedFileExtensions",
"assignments",
"exemptAppLockerFiles",
"protectedAppLockerFiles"})
@JsonInclude(Include.NON_NULL)
public class WindowsInformationProtection extends ManagedAppPolicy implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.windowsInformationProtection";
}
@JsonProperty("azureRightsManagementServicesAllowed")
protected Boolean azureRightsManagementServicesAllowed;
@JsonProperty("dataRecoveryCertificate")
protected WindowsInformationProtectionDataRecoveryCertificate dataRecoveryCertificate;
@JsonProperty("enforcementLevel")
protected WindowsInformationProtectionEnforcementLevel enforcementLevel;
@JsonProperty("enterpriseDomain")
protected String enterpriseDomain;
@JsonProperty("enterpriseInternalProxyServers")
protected List enterpriseInternalProxyServers;
@JsonProperty("enterpriseInternalProxyServers@nextLink")
protected String enterpriseInternalProxyServersNextLink;
@JsonProperty("enterpriseIPRanges")
protected List enterpriseIPRanges;
@JsonProperty("enterpriseIPRanges@nextLink")
protected String enterpriseIPRangesNextLink;
@JsonProperty("enterpriseIPRangesAreAuthoritative")
protected Boolean enterpriseIPRangesAreAuthoritative;
@JsonProperty("enterpriseNetworkDomainNames")
protected List enterpriseNetworkDomainNames;
@JsonProperty("enterpriseNetworkDomainNames@nextLink")
protected String enterpriseNetworkDomainNamesNextLink;
@JsonProperty("enterpriseProtectedDomainNames")
protected List enterpriseProtectedDomainNames;
@JsonProperty("enterpriseProtectedDomainNames@nextLink")
protected String enterpriseProtectedDomainNamesNextLink;
@JsonProperty("enterpriseProxiedDomains")
protected List enterpriseProxiedDomains;
@JsonProperty("enterpriseProxiedDomains@nextLink")
protected String enterpriseProxiedDomainsNextLink;
@JsonProperty("enterpriseProxyServers")
protected List enterpriseProxyServers;
@JsonProperty("enterpriseProxyServers@nextLink")
protected String enterpriseProxyServersNextLink;
@JsonProperty("enterpriseProxyServersAreAuthoritative")
protected Boolean enterpriseProxyServersAreAuthoritative;
@JsonProperty("exemptApps")
protected List exemptApps;
@JsonProperty("exemptApps@nextLink")
protected String exemptAppsNextLink;
@JsonProperty("iconsVisible")
protected Boolean iconsVisible;
@JsonProperty("indexingEncryptedStoresOrItemsBlocked")
protected Boolean indexingEncryptedStoresOrItemsBlocked;
@JsonProperty("isAssigned")
protected Boolean isAssigned;
@JsonProperty("neutralDomainResources")
protected List neutralDomainResources;
@JsonProperty("neutralDomainResources@nextLink")
protected String neutralDomainResourcesNextLink;
@JsonProperty("protectedApps")
protected List protectedApps;
@JsonProperty("protectedApps@nextLink")
protected String protectedAppsNextLink;
@JsonProperty("protectionUnderLockConfigRequired")
protected Boolean protectionUnderLockConfigRequired;
@JsonProperty("revokeOnUnenrollDisabled")
protected Boolean revokeOnUnenrollDisabled;
@JsonProperty("rightsManagementServicesTemplateId")
protected UUID rightsManagementServicesTemplateId;
@JsonProperty("smbAutoEncryptedFileExtensions")
protected List smbAutoEncryptedFileExtensions;
@JsonProperty("smbAutoEncryptedFileExtensions@nextLink")
protected String smbAutoEncryptedFileExtensionsNextLink;
@JsonProperty("assignments")
protected List assignments;
@JsonProperty("exemptAppLockerFiles")
protected List exemptAppLockerFiles;
@JsonProperty("protectedAppLockerFiles")
protected List protectedAppLockerFiles;
protected WindowsInformationProtection() {
super();
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “Specifies whether to allow Azure RMS encryption for WIP”
*
* @return property azureRightsManagementServicesAllowed
*/
@Property(name="azureRightsManagementServicesAllowed")
@JsonIgnore
public Optional getAzureRightsManagementServicesAllowed() {
return Optional.ofNullable(azureRightsManagementServicesAllowed);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* azureRightsManagementServicesAllowed} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Specifies whether to allow Azure RMS encryption for WIP”
*
* @param azureRightsManagementServicesAllowed
* new value of {@code azureRightsManagementServicesAllowed} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code azureRightsManagementServicesAllowed} field changed
*/
public WindowsInformationProtection withAzureRightsManagementServicesAllowed(Boolean azureRightsManagementServicesAllowed) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("azureRightsManagementServicesAllowed");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.azureRightsManagementServicesAllowed = azureRightsManagementServicesAllowed;
return _x;
}
/**
* “Specifies a recovery certificate that can be used for data recovery of encrypted
* files. This is the same as the data recovery agent(DRA) certificate for
* encrypting file system(EFS)”
*
* @return property dataRecoveryCertificate
*/
@Property(name="dataRecoveryCertificate")
@JsonIgnore
public Optional getDataRecoveryCertificate() {
return Optional.ofNullable(dataRecoveryCertificate);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* dataRecoveryCertificate} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Specifies a recovery certificate that can be used for data recovery of encrypted
* files. This is the same as the data recovery agent(DRA) certificate for
* encrypting file system(EFS)”
*
* @param dataRecoveryCertificate
* new value of {@code dataRecoveryCertificate} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code dataRecoveryCertificate} field changed
*/
public WindowsInformationProtection withDataRecoveryCertificate(WindowsInformationProtectionDataRecoveryCertificate dataRecoveryCertificate) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("dataRecoveryCertificate");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.dataRecoveryCertificate = dataRecoveryCertificate;
return _x;
}
/**
* “WIP enforcement level.See the Enum definition for supported values”
*
* @return property enforcementLevel
*/
@Property(name="enforcementLevel")
@JsonIgnore
public Optional getEnforcementLevel() {
return Optional.ofNullable(enforcementLevel);
}
/**
* Returns an immutable copy of {@code this} with just the {@code enforcementLevel}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “WIP enforcement level.See the Enum definition for supported values”
*
* @param enforcementLevel
* new value of {@code enforcementLevel} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enforcementLevel} field changed
*/
public WindowsInformationProtection withEnforcementLevel(WindowsInformationProtectionEnforcementLevel enforcementLevel) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("enforcementLevel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.enforcementLevel = enforcementLevel;
return _x;
}
/**
* “Primary enterprise domain”
*
* @return property enterpriseDomain
*/
@Property(name="enterpriseDomain")
@JsonIgnore
public Optional getEnterpriseDomain() {
return Optional.ofNullable(enterpriseDomain);
}
/**
* Returns an immutable copy of {@code this} with just the {@code enterpriseDomain}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Primary enterprise domain”
*
* @param enterpriseDomain
* new value of {@code enterpriseDomain} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enterpriseDomain} field changed
*/
public WindowsInformationProtection withEnterpriseDomain(String enterpriseDomain) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("enterpriseDomain");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.enterpriseDomain = enterpriseDomain;
return _x;
}
/**
* “This is the comma-separated list of internal proxy servers. For example, "157.54
* .14.28, 157.54.11.118, 10.202.14.167, 157.53.14.163, 157.69.210.59". These
* proxies have been configured by the admin to connect to specific resources on
* the Internet. They are considered to be enterprise network locations. The
* proxies are only leveraged in configuring the EnterpriseProxiedDomains policy to
* force traffic to the matched domains through these proxies”
*
* @return property enterpriseInternalProxyServers
*/
@Property(name="enterpriseInternalProxyServers")
@JsonIgnore
public CollectionPage getEnterpriseInternalProxyServers() {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.enterpriseInternalProxyServers, Optional.ofNullable(enterpriseInternalProxyServersNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* enterpriseInternalProxyServers} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “This is the comma-separated list of internal proxy servers. For example, "157.54
* .14.28, 157.54.11.118, 10.202.14.167, 157.53.14.163, 157.69.210.59". These
* proxies have been configured by the admin to connect to specific resources on
* the Internet. They are considered to be enterprise network locations. The
* proxies are only leveraged in configuring the EnterpriseProxiedDomains policy to
* force traffic to the matched domains through these proxies”
*
* @param enterpriseInternalProxyServers
* new value of {@code enterpriseInternalProxyServers} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enterpriseInternalProxyServers} field changed
*/
public WindowsInformationProtection withEnterpriseInternalProxyServers(List enterpriseInternalProxyServers) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("enterpriseInternalProxyServers");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.enterpriseInternalProxyServers = enterpriseInternalProxyServers;
return _x;
}
/**
* “This is the comma-separated list of internal proxy servers. For example, "157.54
* .14.28, 157.54.11.118, 10.202.14.167, 157.53.14.163, 157.69.210.59". These
* proxies have been configured by the admin to connect to specific resources on
* the Internet. They are considered to be enterprise network locations. The
* proxies are only leveraged in configuring the EnterpriseProxiedDomains policy to
* force traffic to the matched domains through these proxies”
*
* @param options
* specify connect and read timeouts
* @return property enterpriseInternalProxyServers
*/
@Property(name="enterpriseInternalProxyServers")
@JsonIgnore
public CollectionPage getEnterpriseInternalProxyServers(HttpRequestOptions options) {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.enterpriseInternalProxyServers, Optional.ofNullable(enterpriseInternalProxyServersNextLink), Collections.emptyList(), options);
}
/**
* “Sets the enterprise IP ranges that define the computers in the enterprise
* network. Data that comes from those computers will be considered part of the
* enterprise and protected. These locations will be considered a safe destination
* for enterprise data to be shared to”
*
* @return property enterpriseIPRanges
*/
@Property(name="enterpriseIPRanges")
@JsonIgnore
public CollectionPage getEnterpriseIPRanges() {
return new CollectionPage(contextPath, WindowsInformationProtectionIPRangeCollection.class, this.enterpriseIPRanges, Optional.ofNullable(enterpriseIPRangesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* enterpriseIPRanges} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Sets the enterprise IP ranges that define the computers in the enterprise
* network. Data that comes from those computers will be considered part of the
* enterprise and protected. These locations will be considered a safe destination
* for enterprise data to be shared to”
*
* @param enterpriseIPRanges
* new value of {@code enterpriseIPRanges} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enterpriseIPRanges} field changed
*/
public WindowsInformationProtection withEnterpriseIPRanges(List enterpriseIPRanges) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("enterpriseIPRanges");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.enterpriseIPRanges = enterpriseIPRanges;
return _x;
}
/**
* “Sets the enterprise IP ranges that define the computers in the enterprise
* network. Data that comes from those computers will be considered part of the
* enterprise and protected. These locations will be considered a safe destination
* for enterprise data to be shared to”
*
* @param options
* specify connect and read timeouts
* @return property enterpriseIPRanges
*/
@Property(name="enterpriseIPRanges")
@JsonIgnore
public CollectionPage getEnterpriseIPRanges(HttpRequestOptions options) {
return new CollectionPage(contextPath, WindowsInformationProtectionIPRangeCollection.class, this.enterpriseIPRanges, Optional.ofNullable(enterpriseIPRangesNextLink), Collections.emptyList(), options);
}
/**
* “Boolean value that tells the client to accept the configured list and not to use
* heuristics to attempt to find other subnets. Default is false”
*
* @return property enterpriseIPRangesAreAuthoritative
*/
@Property(name="enterpriseIPRangesAreAuthoritative")
@JsonIgnore
public Optional getEnterpriseIPRangesAreAuthoritative() {
return Optional.ofNullable(enterpriseIPRangesAreAuthoritative);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* enterpriseIPRangesAreAuthoritative} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Boolean value that tells the client to accept the configured list and not to use
* heuristics to attempt to find other subnets. Default is false”
*
* @param enterpriseIPRangesAreAuthoritative
* new value of {@code enterpriseIPRangesAreAuthoritative} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enterpriseIPRangesAreAuthoritative} field changed
*/
public WindowsInformationProtection withEnterpriseIPRangesAreAuthoritative(Boolean enterpriseIPRangesAreAuthoritative) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("enterpriseIPRangesAreAuthoritative");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.enterpriseIPRangesAreAuthoritative = enterpriseIPRangesAreAuthoritative;
return _x;
}
/**
* “This is the list of domains that comprise the boundaries of the enterprise. Data
* from one of these domains that is sent to a device will be considered enterprise
* data and protected These locations will be considered a safe destination for
* enterprise data to be shared to”
*
* @return property enterpriseNetworkDomainNames
*/
@Property(name="enterpriseNetworkDomainNames")
@JsonIgnore
public CollectionPage getEnterpriseNetworkDomainNames() {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.enterpriseNetworkDomainNames, Optional.ofNullable(enterpriseNetworkDomainNamesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* enterpriseNetworkDomainNames} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “This is the list of domains that comprise the boundaries of the enterprise. Data
* from one of these domains that is sent to a device will be considered enterprise
* data and protected These locations will be considered a safe destination for
* enterprise data to be shared to”
*
* @param enterpriseNetworkDomainNames
* new value of {@code enterpriseNetworkDomainNames} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enterpriseNetworkDomainNames} field changed
*/
public WindowsInformationProtection withEnterpriseNetworkDomainNames(List enterpriseNetworkDomainNames) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("enterpriseNetworkDomainNames");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.enterpriseNetworkDomainNames = enterpriseNetworkDomainNames;
return _x;
}
/**
* “This is the list of domains that comprise the boundaries of the enterprise. Data
* from one of these domains that is sent to a device will be considered enterprise
* data and protected These locations will be considered a safe destination for
* enterprise data to be shared to”
*
* @param options
* specify connect and read timeouts
* @return property enterpriseNetworkDomainNames
*/
@Property(name="enterpriseNetworkDomainNames")
@JsonIgnore
public CollectionPage getEnterpriseNetworkDomainNames(HttpRequestOptions options) {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.enterpriseNetworkDomainNames, Optional.ofNullable(enterpriseNetworkDomainNamesNextLink), Collections.emptyList(), options);
}
/**
* “List of enterprise domains to be protected”
*
* @return property enterpriseProtectedDomainNames
*/
@Property(name="enterpriseProtectedDomainNames")
@JsonIgnore
public CollectionPage getEnterpriseProtectedDomainNames() {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.enterpriseProtectedDomainNames, Optional.ofNullable(enterpriseProtectedDomainNamesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* enterpriseProtectedDomainNames} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “List of enterprise domains to be protected”
*
* @param enterpriseProtectedDomainNames
* new value of {@code enterpriseProtectedDomainNames} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enterpriseProtectedDomainNames} field changed
*/
public WindowsInformationProtection withEnterpriseProtectedDomainNames(List enterpriseProtectedDomainNames) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("enterpriseProtectedDomainNames");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.enterpriseProtectedDomainNames = enterpriseProtectedDomainNames;
return _x;
}
/**
* “List of enterprise domains to be protected”
*
* @param options
* specify connect and read timeouts
* @return property enterpriseProtectedDomainNames
*/
@Property(name="enterpriseProtectedDomainNames")
@JsonIgnore
public CollectionPage getEnterpriseProtectedDomainNames(HttpRequestOptions options) {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.enterpriseProtectedDomainNames, Optional.ofNullable(enterpriseProtectedDomainNamesNextLink), Collections.emptyList(), options);
}
/**
* “Contains a list of Enterprise resource domains hosted in the cloud that need to
* be protected. Connections to these resources are considered enterprise data. If
* a proxy is paired with a cloud resource, traffic to the cloud resource will be
* routed through the enterprise network via the denoted proxy server (on Port 80).
* A proxy server used for this purpose must also be configured using the
* EnterpriseInternalProxyServers policy”
*
* @return property enterpriseProxiedDomains
*/
@Property(name="enterpriseProxiedDomains")
@JsonIgnore
public CollectionPage getEnterpriseProxiedDomains() {
return new CollectionPage(contextPath, WindowsInformationProtectionProxiedDomainCollection.class, this.enterpriseProxiedDomains, Optional.ofNullable(enterpriseProxiedDomainsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* enterpriseProxiedDomains} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Contains a list of Enterprise resource domains hosted in the cloud that need to
* be protected. Connections to these resources are considered enterprise data. If
* a proxy is paired with a cloud resource, traffic to the cloud resource will be
* routed through the enterprise network via the denoted proxy server (on Port 80).
* A proxy server used for this purpose must also be configured using the
* EnterpriseInternalProxyServers policy”
*
* @param enterpriseProxiedDomains
* new value of {@code enterpriseProxiedDomains} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enterpriseProxiedDomains} field changed
*/
public WindowsInformationProtection withEnterpriseProxiedDomains(List enterpriseProxiedDomains) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("enterpriseProxiedDomains");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.enterpriseProxiedDomains = enterpriseProxiedDomains;
return _x;
}
/**
* “Contains a list of Enterprise resource domains hosted in the cloud that need to
* be protected. Connections to these resources are considered enterprise data. If
* a proxy is paired with a cloud resource, traffic to the cloud resource will be
* routed through the enterprise network via the denoted proxy server (on Port 80).
* A proxy server used for this purpose must also be configured using the
* EnterpriseInternalProxyServers policy”
*
* @param options
* specify connect and read timeouts
* @return property enterpriseProxiedDomains
*/
@Property(name="enterpriseProxiedDomains")
@JsonIgnore
public CollectionPage getEnterpriseProxiedDomains(HttpRequestOptions options) {
return new CollectionPage(contextPath, WindowsInformationProtectionProxiedDomainCollection.class, this.enterpriseProxiedDomains, Optional.ofNullable(enterpriseProxiedDomainsNextLink), Collections.emptyList(), options);
}
/**
* “This is a list of proxy servers. Any server not on this list is considered non-
* enterprise”
*
* @return property enterpriseProxyServers
*/
@Property(name="enterpriseProxyServers")
@JsonIgnore
public CollectionPage getEnterpriseProxyServers() {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.enterpriseProxyServers, Optional.ofNullable(enterpriseProxyServersNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* enterpriseProxyServers} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “This is a list of proxy servers. Any server not on this list is considered non-
* enterprise”
*
* @param enterpriseProxyServers
* new value of {@code enterpriseProxyServers} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enterpriseProxyServers} field changed
*/
public WindowsInformationProtection withEnterpriseProxyServers(List enterpriseProxyServers) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("enterpriseProxyServers");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.enterpriseProxyServers = enterpriseProxyServers;
return _x;
}
/**
* “This is a list of proxy servers. Any server not on this list is considered non-
* enterprise”
*
* @param options
* specify connect and read timeouts
* @return property enterpriseProxyServers
*/
@Property(name="enterpriseProxyServers")
@JsonIgnore
public CollectionPage getEnterpriseProxyServers(HttpRequestOptions options) {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.enterpriseProxyServers, Optional.ofNullable(enterpriseProxyServersNextLink), Collections.emptyList(), options);
}
/**
* “Boolean value that tells the client to accept the configured list of proxies and
* not try to detect other work proxies. Default is false”
*
* @return property enterpriseProxyServersAreAuthoritative
*/
@Property(name="enterpriseProxyServersAreAuthoritative")
@JsonIgnore
public Optional getEnterpriseProxyServersAreAuthoritative() {
return Optional.ofNullable(enterpriseProxyServersAreAuthoritative);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* enterpriseProxyServersAreAuthoritative} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Boolean value that tells the client to accept the configured list of proxies and
* not try to detect other work proxies. Default is false”
*
* @param enterpriseProxyServersAreAuthoritative
* new value of {@code enterpriseProxyServersAreAuthoritative} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enterpriseProxyServersAreAuthoritative} field changed
*/
public WindowsInformationProtection withEnterpriseProxyServersAreAuthoritative(Boolean enterpriseProxyServersAreAuthoritative) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("enterpriseProxyServersAreAuthoritative");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.enterpriseProxyServersAreAuthoritative = enterpriseProxyServersAreAuthoritative;
return _x;
}
/**
* “Exempt applications can also access enterprise data, but the data handled by
* those applications are not protected. This is because some critical enterprise
* applications may have compatibility problems with encrypted data.”
*
* @return property exemptApps
*/
@Property(name="exemptApps")
@JsonIgnore
public CollectionPage getExemptApps() {
return new CollectionPage(contextPath, WindowsInformationProtectionApp.class, this.exemptApps, Optional.ofNullable(exemptAppsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code exemptApps} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Exempt applications can also access enterprise data, but the data handled by
* those applications are not protected. This is because some critical enterprise
* applications may have compatibility problems with encrypted data.”
*
* @param exemptApps
* new value of {@code exemptApps} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code exemptApps} field changed
*/
public WindowsInformationProtection withExemptApps(List exemptApps) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("exemptApps");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.exemptApps = exemptApps;
return _x;
}
/**
* “Exempt applications can also access enterprise data, but the data handled by
* those applications are not protected. This is because some critical enterprise
* applications may have compatibility problems with encrypted data.”
*
* @param options
* specify connect and read timeouts
* @return property exemptApps
*/
@Property(name="exemptApps")
@JsonIgnore
public CollectionPage getExemptApps(HttpRequestOptions options) {
return new CollectionPage(contextPath, WindowsInformationProtectionApp.class, this.exemptApps, Optional.ofNullable(exemptAppsNextLink), Collections.emptyList(), options);
}
/**
* “Determines whether overlays are added to icons for WIP protected files in
* Explorer and enterprise only app tiles in the Start menu. Starting in Windows 10
* , version 1703 this setting also configures the visibility of the WIP icon in
* the title bar of a WIP-protected app”
*
* @return property iconsVisible
*/
@Property(name="iconsVisible")
@JsonIgnore
public Optional getIconsVisible() {
return Optional.ofNullable(iconsVisible);
}
/**
* Returns an immutable copy of {@code this} with just the {@code iconsVisible}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Determines whether overlays are added to icons for WIP protected files in
* Explorer and enterprise only app tiles in the Start menu. Starting in Windows 10
* , version 1703 this setting also configures the visibility of the WIP icon in
* the title bar of a WIP-protected app”
*
* @param iconsVisible
* new value of {@code iconsVisible} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code iconsVisible} field changed
*/
public WindowsInformationProtection withIconsVisible(Boolean iconsVisible) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("iconsVisible");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.iconsVisible = iconsVisible;
return _x;
}
/**
* “This switch is for the Windows Search Indexer, to allow or disallow indexing of
* items”
*
* @return property indexingEncryptedStoresOrItemsBlocked
*/
@Property(name="indexingEncryptedStoresOrItemsBlocked")
@JsonIgnore
public Optional getIndexingEncryptedStoresOrItemsBlocked() {
return Optional.ofNullable(indexingEncryptedStoresOrItemsBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* indexingEncryptedStoresOrItemsBlocked} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “This switch is for the Windows Search Indexer, to allow or disallow indexing of
* items”
*
* @param indexingEncryptedStoresOrItemsBlocked
* new value of {@code indexingEncryptedStoresOrItemsBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code indexingEncryptedStoresOrItemsBlocked} field changed
*/
public WindowsInformationProtection withIndexingEncryptedStoresOrItemsBlocked(Boolean indexingEncryptedStoresOrItemsBlocked) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("indexingEncryptedStoresOrItemsBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.indexingEncryptedStoresOrItemsBlocked = indexingEncryptedStoresOrItemsBlocked;
return _x;
}
/**
* “Indicates if the policy is deployed to any inclusion groups or not.”
*
* @return property isAssigned
*/
@Property(name="isAssigned")
@JsonIgnore
public Optional getIsAssigned() {
return Optional.ofNullable(isAssigned);
}
/**
* Returns an immutable copy of {@code this} with just the {@code isAssigned} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Indicates if the policy is deployed to any inclusion groups or not.”
*
* @param isAssigned
* new value of {@code isAssigned} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code isAssigned} field changed
*/
public WindowsInformationProtection withIsAssigned(Boolean isAssigned) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("isAssigned");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.isAssigned = isAssigned;
return _x;
}
/**
* “List of domain names that can used for work or personal resource”
*
* @return property neutralDomainResources
*/
@Property(name="neutralDomainResources")
@JsonIgnore
public CollectionPage getNeutralDomainResources() {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.neutralDomainResources, Optional.ofNullable(neutralDomainResourcesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* neutralDomainResources} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “List of domain names that can used for work or personal resource”
*
* @param neutralDomainResources
* new value of {@code neutralDomainResources} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code neutralDomainResources} field changed
*/
public WindowsInformationProtection withNeutralDomainResources(List neutralDomainResources) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("neutralDomainResources");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.neutralDomainResources = neutralDomainResources;
return _x;
}
/**
* “List of domain names that can used for work or personal resource”
*
* @param options
* specify connect and read timeouts
* @return property neutralDomainResources
*/
@Property(name="neutralDomainResources")
@JsonIgnore
public CollectionPage getNeutralDomainResources(HttpRequestOptions options) {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.neutralDomainResources, Optional.ofNullable(neutralDomainResourcesNextLink), Collections.emptyList(), options);
}
/**
* “Protected applications can access enterprise data and the data handled by those
* applications are protected with encryption”
*
* @return property protectedApps
*/
@Property(name="protectedApps")
@JsonIgnore
public CollectionPage getProtectedApps() {
return new CollectionPage(contextPath, WindowsInformationProtectionApp.class, this.protectedApps, Optional.ofNullable(protectedAppsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code protectedApps}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Protected applications can access enterprise data and the data handled by those
* applications are protected with encryption”
*
* @param protectedApps
* new value of {@code protectedApps} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code protectedApps} field changed
*/
public WindowsInformationProtection withProtectedApps(List protectedApps) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("protectedApps");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.protectedApps = protectedApps;
return _x;
}
/**
* “Protected applications can access enterprise data and the data handled by those
* applications are protected with encryption”
*
* @param options
* specify connect and read timeouts
* @return property protectedApps
*/
@Property(name="protectedApps")
@JsonIgnore
public CollectionPage getProtectedApps(HttpRequestOptions options) {
return new CollectionPage(contextPath, WindowsInformationProtectionApp.class, this.protectedApps, Optional.ofNullable(protectedAppsNextLink), Collections.emptyList(), options);
}
/**
* “Specifies whether the protection under lock feature (also known as encrypt under
* pin) should be configured”
*
* @return property protectionUnderLockConfigRequired
*/
@Property(name="protectionUnderLockConfigRequired")
@JsonIgnore
public Optional getProtectionUnderLockConfigRequired() {
return Optional.ofNullable(protectionUnderLockConfigRequired);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* protectionUnderLockConfigRequired} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Specifies whether the protection under lock feature (also known as encrypt under
* pin) should be configured”
*
* @param protectionUnderLockConfigRequired
* new value of {@code protectionUnderLockConfigRequired} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code protectionUnderLockConfigRequired} field changed
*/
public WindowsInformationProtection withProtectionUnderLockConfigRequired(Boolean protectionUnderLockConfigRequired) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("protectionUnderLockConfigRequired");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.protectionUnderLockConfigRequired = protectionUnderLockConfigRequired;
return _x;
}
/**
* “This policy controls whether to revoke the WIP keys when a device unenrolls from
* the management service. If set to 1 (Don't revoke keys), the keys will not be
* revoked and the user will continue to have access to protected files after
* unenrollment. If the keys are not revoked, there will be no revoked file cleanup
* subsequently.”
*
* @return property revokeOnUnenrollDisabled
*/
@Property(name="revokeOnUnenrollDisabled")
@JsonIgnore
public Optional getRevokeOnUnenrollDisabled() {
return Optional.ofNullable(revokeOnUnenrollDisabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* revokeOnUnenrollDisabled} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “This policy controls whether to revoke the WIP keys when a device unenrolls from
* the management service. If set to 1 (Don't revoke keys), the keys will not be
* revoked and the user will continue to have access to protected files after
* unenrollment. If the keys are not revoked, there will be no revoked file cleanup
* subsequently.”
*
* @param revokeOnUnenrollDisabled
* new value of {@code revokeOnUnenrollDisabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code revokeOnUnenrollDisabled} field changed
*/
public WindowsInformationProtection withRevokeOnUnenrollDisabled(Boolean revokeOnUnenrollDisabled) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("revokeOnUnenrollDisabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.revokeOnUnenrollDisabled = revokeOnUnenrollDisabled;
return _x;
}
/**
* “TemplateID GUID to use for RMS encryption. The RMS template allows the IT admin
* to configure the details about who has access to RMS-protected file and how long
* they have access”
*
* @return property rightsManagementServicesTemplateId
*/
@Property(name="rightsManagementServicesTemplateId")
@JsonIgnore
public Optional getRightsManagementServicesTemplateId() {
return Optional.ofNullable(rightsManagementServicesTemplateId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* rightsManagementServicesTemplateId} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “TemplateID GUID to use for RMS encryption. The RMS template allows the IT admin
* to configure the details about who has access to RMS-protected file and how long
* they have access”
*
* @param rightsManagementServicesTemplateId
* new value of {@code rightsManagementServicesTemplateId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code rightsManagementServicesTemplateId} field changed
*/
public WindowsInformationProtection withRightsManagementServicesTemplateId(UUID rightsManagementServicesTemplateId) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("rightsManagementServicesTemplateId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.rightsManagementServicesTemplateId = rightsManagementServicesTemplateId;
return _x;
}
/**
* “Specifies a list of file extensions, so that files with these extensions are
* encrypted when copying from an SMB share within the corporate boundary”
*
* @return property smbAutoEncryptedFileExtensions
*/
@Property(name="smbAutoEncryptedFileExtensions")
@JsonIgnore
public CollectionPage getSmbAutoEncryptedFileExtensions() {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.smbAutoEncryptedFileExtensions, Optional.ofNullable(smbAutoEncryptedFileExtensionsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* smbAutoEncryptedFileExtensions} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Specifies a list of file extensions, so that files with these extensions are
* encrypted when copying from an SMB share within the corporate boundary”
*
* @param smbAutoEncryptedFileExtensions
* new value of {@code smbAutoEncryptedFileExtensions} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code smbAutoEncryptedFileExtensions} field changed
*/
public WindowsInformationProtection withSmbAutoEncryptedFileExtensions(List smbAutoEncryptedFileExtensions) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("smbAutoEncryptedFileExtensions");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.smbAutoEncryptedFileExtensions = smbAutoEncryptedFileExtensions;
return _x;
}
/**
* “Specifies a list of file extensions, so that files with these extensions are
* encrypted when copying from an SMB share within the corporate boundary”
*
* @param options
* specify connect and read timeouts
* @return property smbAutoEncryptedFileExtensions
*/
@Property(name="smbAutoEncryptedFileExtensions")
@JsonIgnore
public CollectionPage getSmbAutoEncryptedFileExtensions(HttpRequestOptions options) {
return new CollectionPage(contextPath, WindowsInformationProtectionResourceCollection.class, this.smbAutoEncryptedFileExtensions, Optional.ofNullable(smbAutoEncryptedFileExtensionsNextLink), Collections.emptyList(), options);
}
public WindowsInformationProtection withUnmappedField(String name, Object value) {
WindowsInformationProtection _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “Navigation property to list of security groups targeted for policy.”
*
* @return navigational property assignments
*/
@NavigationProperty(name="assignments")
@JsonIgnore
public TargetedManagedAppPolicyAssignmentCollectionRequest getAssignments() {
return new TargetedManagedAppPolicyAssignmentCollectionRequest(
contextPath.addSegment("assignments"), Optional.ofNullable(assignments));
}
/**
* “Another way to input exempt apps through xml files”
*
* @return navigational property exemptAppLockerFiles
*/
@NavigationProperty(name="exemptAppLockerFiles")
@JsonIgnore
public WindowsInformationProtectionAppLockerFileCollectionRequest getExemptAppLockerFiles() {
return new WindowsInformationProtectionAppLockerFileCollectionRequest(
contextPath.addSegment("exemptAppLockerFiles"), Optional.ofNullable(exemptAppLockerFiles));
}
/**
* “Another way to input protected apps through xml files”
*
* @return navigational property protectedAppLockerFiles
*/
@NavigationProperty(name="protectedAppLockerFiles")
@JsonIgnore
public WindowsInformationProtectionAppLockerFileCollectionRequest getProtectedAppLockerFiles() {
return new WindowsInformationProtectionAppLockerFileCollectionRequest(
contextPath.addSegment("protectedAppLockerFiles"), Optional.ofNullable(protectedAppLockerFiles));
}
/**
* Returns an immutable copy of {@code this} with just the {@code assignments}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Navigation property to list of security groups targeted for policy.”
*
* @param assignments
* new value of {@code assignments} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code assignments} field changed
*/
public WindowsInformationProtection withAssignments(List assignments) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("assignments");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.assignments = assignments;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* exemptAppLockerFiles} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Another way to input exempt apps through xml files”
*
* @param exemptAppLockerFiles
* new value of {@code exemptAppLockerFiles} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code exemptAppLockerFiles} field changed
*/
public WindowsInformationProtection withExemptAppLockerFiles(List exemptAppLockerFiles) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("exemptAppLockerFiles");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.exemptAppLockerFiles = exemptAppLockerFiles;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* protectedAppLockerFiles} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Another way to input protected apps through xml files”
*
* @param protectedAppLockerFiles
* new value of {@code protectedAppLockerFiles} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code protectedAppLockerFiles} field changed
*/
public WindowsInformationProtection withProtectedAppLockerFiles(List protectedAppLockerFiles) {
WindowsInformationProtection _x = _copy();
_x.changedFields = changedFields.add("protectedAppLockerFiles");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsInformationProtection");
_x.protectedAppLockerFiles = protectedAppLockerFiles;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsInformationProtection patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
WindowsInformationProtection _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsInformationProtection put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
WindowsInformationProtection _x = _copy();
_x.changedFields = null;
return _x;
}
private WindowsInformationProtection _copy() {
WindowsInformationProtection _x = new WindowsInformationProtection();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.version = version;
_x.azureRightsManagementServicesAllowed = azureRightsManagementServicesAllowed;
_x.dataRecoveryCertificate = dataRecoveryCertificate;
_x.enforcementLevel = enforcementLevel;
_x.enterpriseDomain = enterpriseDomain;
_x.enterpriseInternalProxyServers = enterpriseInternalProxyServers;
_x.enterpriseIPRanges = enterpriseIPRanges;
_x.enterpriseIPRangesAreAuthoritative = enterpriseIPRangesAreAuthoritative;
_x.enterpriseNetworkDomainNames = enterpriseNetworkDomainNames;
_x.enterpriseProtectedDomainNames = enterpriseProtectedDomainNames;
_x.enterpriseProxiedDomains = enterpriseProxiedDomains;
_x.enterpriseProxyServers = enterpriseProxyServers;
_x.enterpriseProxyServersAreAuthoritative = enterpriseProxyServersAreAuthoritative;
_x.exemptApps = exemptApps;
_x.iconsVisible = iconsVisible;
_x.indexingEncryptedStoresOrItemsBlocked = indexingEncryptedStoresOrItemsBlocked;
_x.isAssigned = isAssigned;
_x.neutralDomainResources = neutralDomainResources;
_x.protectedApps = protectedApps;
_x.protectionUnderLockConfigRequired = protectionUnderLockConfigRequired;
_x.revokeOnUnenrollDisabled = revokeOnUnenrollDisabled;
_x.rightsManagementServicesTemplateId = rightsManagementServicesTemplateId;
_x.smbAutoEncryptedFileExtensions = smbAutoEncryptedFileExtensions;
_x.assignments = assignments;
_x.exemptAppLockerFiles = exemptAppLockerFiles;
_x.protectedAppLockerFiles = protectedAppLockerFiles;
return _x;
}
@Action(name = "assign")
@JsonIgnore
public ActionRequestNoReturn assign(List assignments) {
Map _parameters = ParameterMap
.put("assignments", "Collection(microsoft.graph.targetedManagedAppPolicyAssignment)", assignments)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.assign"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("WindowsInformationProtection[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("azureRightsManagementServicesAllowed=");
b.append(this.azureRightsManagementServicesAllowed);
b.append(", ");
b.append("dataRecoveryCertificate=");
b.append(this.dataRecoveryCertificate);
b.append(", ");
b.append("enforcementLevel=");
b.append(this.enforcementLevel);
b.append(", ");
b.append("enterpriseDomain=");
b.append(this.enterpriseDomain);
b.append(", ");
b.append("enterpriseInternalProxyServers=");
b.append(this.enterpriseInternalProxyServers);
b.append(", ");
b.append("enterpriseIPRanges=");
b.append(this.enterpriseIPRanges);
b.append(", ");
b.append("enterpriseIPRangesAreAuthoritative=");
b.append(this.enterpriseIPRangesAreAuthoritative);
b.append(", ");
b.append("enterpriseNetworkDomainNames=");
b.append(this.enterpriseNetworkDomainNames);
b.append(", ");
b.append("enterpriseProtectedDomainNames=");
b.append(this.enterpriseProtectedDomainNames);
b.append(", ");
b.append("enterpriseProxiedDomains=");
b.append(this.enterpriseProxiedDomains);
b.append(", ");
b.append("enterpriseProxyServers=");
b.append(this.enterpriseProxyServers);
b.append(", ");
b.append("enterpriseProxyServersAreAuthoritative=");
b.append(this.enterpriseProxyServersAreAuthoritative);
b.append(", ");
b.append("exemptApps=");
b.append(this.exemptApps);
b.append(", ");
b.append("iconsVisible=");
b.append(this.iconsVisible);
b.append(", ");
b.append("indexingEncryptedStoresOrItemsBlocked=");
b.append(this.indexingEncryptedStoresOrItemsBlocked);
b.append(", ");
b.append("isAssigned=");
b.append(this.isAssigned);
b.append(", ");
b.append("neutralDomainResources=");
b.append(this.neutralDomainResources);
b.append(", ");
b.append("protectedApps=");
b.append(this.protectedApps);
b.append(", ");
b.append("protectionUnderLockConfigRequired=");
b.append(this.protectionUnderLockConfigRequired);
b.append(", ");
b.append("revokeOnUnenrollDisabled=");
b.append(this.revokeOnUnenrollDisabled);
b.append(", ");
b.append("rightsManagementServicesTemplateId=");
b.append(this.rightsManagementServicesTemplateId);
b.append(", ");
b.append("smbAutoEncryptedFileExtensions=");
b.append(this.smbAutoEncryptedFileExtensions);
b.append(", ");
b.append("assignments=");
b.append(this.assignments);
b.append(", ");
b.append("exemptAppLockerFiles=");
b.append(this.exemptAppLockerFiles);
b.append(", ");
b.append("protectedAppLockerFiles=");
b.append(this.protectedAppLockerFiles);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}