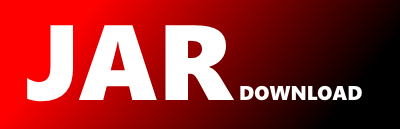
odata.msgraph.client.entity.WindowsPhone81GeneralConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.complex.AppListItem;
import odata.msgraph.client.enums.AppListType;
import odata.msgraph.client.enums.RequiredPasswordType;
/**
* “This topic provides descriptions of the declared methods, properties and
* relationships exposed by the windowsPhone81GeneralConfiguration resource.”
*/@JsonPropertyOrder({
"@odata.type",
"applyOnlyToWindowsPhone81",
"appsBlockCopyPaste",
"bluetoothBlocked",
"cameraBlocked",
"cellularBlockWifiTethering",
"compliantAppListType",
"compliantAppsList",
"diagnosticDataBlockSubmission",
"emailBlockAddingAccounts",
"locationServicesBlocked",
"microsoftAccountBlocked",
"nfcBlocked",
"passwordBlockSimple",
"passwordExpirationDays",
"passwordMinimumCharacterSetCount",
"passwordMinimumLength",
"passwordMinutesOfInactivityBeforeScreenTimeout",
"passwordPreviousPasswordBlockCount",
"passwordRequired",
"passwordRequiredType",
"passwordSignInFailureCountBeforeFactoryReset",
"screenCaptureBlocked",
"storageBlockRemovableStorage",
"storageRequireEncryption",
"webBrowserBlocked",
"wifiBlockAutomaticConnectHotspots",
"wifiBlocked",
"wifiBlockHotspotReporting",
"windowsStoreBlocked"})
@JsonInclude(Include.NON_NULL)
public class WindowsPhone81GeneralConfiguration extends DeviceConfiguration implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.windowsPhone81GeneralConfiguration";
}
@JsonProperty("applyOnlyToWindowsPhone81")
protected Boolean applyOnlyToWindowsPhone81;
@JsonProperty("appsBlockCopyPaste")
protected Boolean appsBlockCopyPaste;
@JsonProperty("bluetoothBlocked")
protected Boolean bluetoothBlocked;
@JsonProperty("cameraBlocked")
protected Boolean cameraBlocked;
@JsonProperty("cellularBlockWifiTethering")
protected Boolean cellularBlockWifiTethering;
@JsonProperty("compliantAppListType")
protected AppListType compliantAppListType;
@JsonProperty("compliantAppsList")
protected List compliantAppsList;
@JsonProperty("compliantAppsList@nextLink")
protected String compliantAppsListNextLink;
@JsonProperty("diagnosticDataBlockSubmission")
protected Boolean diagnosticDataBlockSubmission;
@JsonProperty("emailBlockAddingAccounts")
protected Boolean emailBlockAddingAccounts;
@JsonProperty("locationServicesBlocked")
protected Boolean locationServicesBlocked;
@JsonProperty("microsoftAccountBlocked")
protected Boolean microsoftAccountBlocked;
@JsonProperty("nfcBlocked")
protected Boolean nfcBlocked;
@JsonProperty("passwordBlockSimple")
protected Boolean passwordBlockSimple;
@JsonProperty("passwordExpirationDays")
protected Integer passwordExpirationDays;
@JsonProperty("passwordMinimumCharacterSetCount")
protected Integer passwordMinimumCharacterSetCount;
@JsonProperty("passwordMinimumLength")
protected Integer passwordMinimumLength;
@JsonProperty("passwordMinutesOfInactivityBeforeScreenTimeout")
protected Integer passwordMinutesOfInactivityBeforeScreenTimeout;
@JsonProperty("passwordPreviousPasswordBlockCount")
protected Integer passwordPreviousPasswordBlockCount;
@JsonProperty("passwordRequired")
protected Boolean passwordRequired;
@JsonProperty("passwordRequiredType")
protected RequiredPasswordType passwordRequiredType;
@JsonProperty("passwordSignInFailureCountBeforeFactoryReset")
protected Integer passwordSignInFailureCountBeforeFactoryReset;
@JsonProperty("screenCaptureBlocked")
protected Boolean screenCaptureBlocked;
@JsonProperty("storageBlockRemovableStorage")
protected Boolean storageBlockRemovableStorage;
@JsonProperty("storageRequireEncryption")
protected Boolean storageRequireEncryption;
@JsonProperty("webBrowserBlocked")
protected Boolean webBrowserBlocked;
@JsonProperty("wifiBlockAutomaticConnectHotspots")
protected Boolean wifiBlockAutomaticConnectHotspots;
@JsonProperty("wifiBlocked")
protected Boolean wifiBlocked;
@JsonProperty("wifiBlockHotspotReporting")
protected Boolean wifiBlockHotspotReporting;
@JsonProperty("windowsStoreBlocked")
protected Boolean windowsStoreBlocked;
protected WindowsPhone81GeneralConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderWindowsPhone81GeneralConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private Integer version;
private List assignments;
private List deviceSettingStateSummaries;
private List deviceStatuses;
private DeviceConfigurationDeviceOverview deviceStatusOverview;
private List userStatuses;
private DeviceConfigurationUserOverview userStatusOverview;
private Boolean applyOnlyToWindowsPhone81;
private Boolean appsBlockCopyPaste;
private Boolean bluetoothBlocked;
private Boolean cameraBlocked;
private Boolean cellularBlockWifiTethering;
private AppListType compliantAppListType;
private List compliantAppsList;
private String compliantAppsListNextLink;
private Boolean diagnosticDataBlockSubmission;
private Boolean emailBlockAddingAccounts;
private Boolean locationServicesBlocked;
private Boolean microsoftAccountBlocked;
private Boolean nfcBlocked;
private Boolean passwordBlockSimple;
private Integer passwordExpirationDays;
private Integer passwordMinimumCharacterSetCount;
private Integer passwordMinimumLength;
private Integer passwordMinutesOfInactivityBeforeScreenTimeout;
private Integer passwordPreviousPasswordBlockCount;
private Boolean passwordRequired;
private RequiredPasswordType passwordRequiredType;
private Integer passwordSignInFailureCountBeforeFactoryReset;
private Boolean screenCaptureBlocked;
private Boolean storageBlockRemovableStorage;
private Boolean storageRequireEncryption;
private Boolean webBrowserBlocked;
private Boolean wifiBlockAutomaticConnectHotspots;
private Boolean wifiBlocked;
private Boolean wifiBlockHotspotReporting;
private Boolean windowsStoreBlocked;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
public Builder assignments(List assignments) {
this.assignments = assignments;
this.changedFields = changedFields.add("assignments");
return this;
}
public Builder assignments(DeviceConfigurationAssignment... assignments) {
return assignments(Arrays.asList(assignments));
}
public Builder deviceSettingStateSummaries(List deviceSettingStateSummaries) {
this.deviceSettingStateSummaries = deviceSettingStateSummaries;
this.changedFields = changedFields.add("deviceSettingStateSummaries");
return this;
}
public Builder deviceSettingStateSummaries(SettingStateDeviceSummary... deviceSettingStateSummaries) {
return deviceSettingStateSummaries(Arrays.asList(deviceSettingStateSummaries));
}
public Builder deviceStatuses(List deviceStatuses) {
this.deviceStatuses = deviceStatuses;
this.changedFields = changedFields.add("deviceStatuses");
return this;
}
public Builder deviceStatuses(DeviceConfigurationDeviceStatus... deviceStatuses) {
return deviceStatuses(Arrays.asList(deviceStatuses));
}
public Builder deviceStatusOverview(DeviceConfigurationDeviceOverview deviceStatusOverview) {
this.deviceStatusOverview = deviceStatusOverview;
this.changedFields = changedFields.add("deviceStatusOverview");
return this;
}
public Builder userStatuses(List userStatuses) {
this.userStatuses = userStatuses;
this.changedFields = changedFields.add("userStatuses");
return this;
}
public Builder userStatuses(DeviceConfigurationUserStatus... userStatuses) {
return userStatuses(Arrays.asList(userStatuses));
}
public Builder userStatusOverview(DeviceConfigurationUserOverview userStatusOverview) {
this.userStatusOverview = userStatusOverview;
this.changedFields = changedFields.add("userStatusOverview");
return this;
}
/**
* “Value indicating whether this policy only applies to Windows Phone 8.1. This
* property is read-only.”
*
* Org.OData.Core.V1.Computed
*
* true
*
* Org.OData.Core.V1.Permissions
*
* @param applyOnlyToWindowsPhone81
* value of {@code applyOnlyToWindowsPhone81} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder applyOnlyToWindowsPhone81(Boolean applyOnlyToWindowsPhone81) {
this.applyOnlyToWindowsPhone81 = applyOnlyToWindowsPhone81;
this.changedFields = changedFields.add("applyOnlyToWindowsPhone81");
return this;
}
/**
* “Indicates whether or not to block copy paste.”
*
* @param appsBlockCopyPaste
* value of {@code appsBlockCopyPaste} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder appsBlockCopyPaste(Boolean appsBlockCopyPaste) {
this.appsBlockCopyPaste = appsBlockCopyPaste;
this.changedFields = changedFields.add("appsBlockCopyPaste");
return this;
}
/**
* “Indicates whether or not to block bluetooth.”
*
* @param bluetoothBlocked
* value of {@code bluetoothBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder bluetoothBlocked(Boolean bluetoothBlocked) {
this.bluetoothBlocked = bluetoothBlocked;
this.changedFields = changedFields.add("bluetoothBlocked");
return this;
}
/**
* “Indicates whether or not to block camera.”
*
* @param cameraBlocked
* value of {@code cameraBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder cameraBlocked(Boolean cameraBlocked) {
this.cameraBlocked = cameraBlocked;
this.changedFields = changedFields.add("cameraBlocked");
return this;
}
/**
* “Indicates whether or not to block Wi-Fi tethering. Has no impact if Wi-Fi is
* blocked.”
*
* @param cellularBlockWifiTethering
* value of {@code cellularBlockWifiTethering} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder cellularBlockWifiTethering(Boolean cellularBlockWifiTethering) {
this.cellularBlockWifiTethering = cellularBlockWifiTethering;
this.changedFields = changedFields.add("cellularBlockWifiTethering");
return this;
}
/**
* “List that is in the AppComplianceList.”
*
* @param compliantAppListType
* value of {@code compliantAppListType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder compliantAppListType(AppListType compliantAppListType) {
this.compliantAppListType = compliantAppListType;
this.changedFields = changedFields.add("compliantAppListType");
return this;
}
/**
* “List of apps in the compliance (either allow list or block list, controlled by
* CompliantAppListType). This collection can contain a maximum of 10000 elements.”
*
* @param compliantAppsList
* value of {@code compliantAppsList} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder compliantAppsList(List compliantAppsList) {
this.compliantAppsList = compliantAppsList;
this.changedFields = changedFields.add("compliantAppsList");
return this;
}
/**
* “List of apps in the compliance (either allow list or block list, controlled by
* CompliantAppListType). This collection can contain a maximum of 10000 elements.”
*
* @param compliantAppsList
* value of {@code compliantAppsList} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder compliantAppsList(AppListItem... compliantAppsList) {
return compliantAppsList(Arrays.asList(compliantAppsList));
}
/**
* “List of apps in the compliance (either allow list or block list, controlled by
* CompliantAppListType). This collection can contain a maximum of 10000 elements.”
*
* @param compliantAppsListNextLink
* value of {@code compliantAppsList@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder compliantAppsListNextLink(String compliantAppsListNextLink) {
this.compliantAppsListNextLink = compliantAppsListNextLink;
this.changedFields = changedFields.add("compliantAppsList");
return this;
}
/**
* “Indicates whether or not to block diagnostic data submission.”
*
* @param diagnosticDataBlockSubmission
* value of {@code diagnosticDataBlockSubmission} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder diagnosticDataBlockSubmission(Boolean diagnosticDataBlockSubmission) {
this.diagnosticDataBlockSubmission = diagnosticDataBlockSubmission;
this.changedFields = changedFields.add("diagnosticDataBlockSubmission");
return this;
}
/**
* “Indicates whether or not to block custom email accounts.”
*
* @param emailBlockAddingAccounts
* value of {@code emailBlockAddingAccounts} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder emailBlockAddingAccounts(Boolean emailBlockAddingAccounts) {
this.emailBlockAddingAccounts = emailBlockAddingAccounts;
this.changedFields = changedFields.add("emailBlockAddingAccounts");
return this;
}
/**
* “Indicates whether or not to block location services.”
*
* @param locationServicesBlocked
* value of {@code locationServicesBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder locationServicesBlocked(Boolean locationServicesBlocked) {
this.locationServicesBlocked = locationServicesBlocked;
this.changedFields = changedFields.add("locationServicesBlocked");
return this;
}
/**
* “Indicates whether or not to block using a Microsoft Account.”
*
* @param microsoftAccountBlocked
* value of {@code microsoftAccountBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder microsoftAccountBlocked(Boolean microsoftAccountBlocked) {
this.microsoftAccountBlocked = microsoftAccountBlocked;
this.changedFields = changedFields.add("microsoftAccountBlocked");
return this;
}
/**
* “Indicates whether or not to block Near-Field Communication.”
*
* @param nfcBlocked
* value of {@code nfcBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder nfcBlocked(Boolean nfcBlocked) {
this.nfcBlocked = nfcBlocked;
this.changedFields = changedFields.add("nfcBlocked");
return this;
}
/**
* “Indicates whether or not to block syncing the calendar.”
*
* @param passwordBlockSimple
* value of {@code passwordBlockSimple} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordBlockSimple(Boolean passwordBlockSimple) {
this.passwordBlockSimple = passwordBlockSimple;
this.changedFields = changedFields.add("passwordBlockSimple");
return this;
}
/**
* “Number of days before the password expires.”
*
* @param passwordExpirationDays
* value of {@code passwordExpirationDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordExpirationDays(Integer passwordExpirationDays) {
this.passwordExpirationDays = passwordExpirationDays;
this.changedFields = changedFields.add("passwordExpirationDays");
return this;
}
/**
* “Number of character sets a password must contain.”
*
* @param passwordMinimumCharacterSetCount
* value of {@code passwordMinimumCharacterSetCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinimumCharacterSetCount(Integer passwordMinimumCharacterSetCount) {
this.passwordMinimumCharacterSetCount = passwordMinimumCharacterSetCount;
this.changedFields = changedFields.add("passwordMinimumCharacterSetCount");
return this;
}
/**
* “Minimum length of passwords.”
*
* @param passwordMinimumLength
* value of {@code passwordMinimumLength} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinimumLength(Integer passwordMinimumLength) {
this.passwordMinimumLength = passwordMinimumLength;
this.changedFields = changedFields.add("passwordMinimumLength");
return this;
}
/**
* “Minutes of inactivity before screen timeout.”
*
* @param passwordMinutesOfInactivityBeforeScreenTimeout
* value of {@code passwordMinutesOfInactivityBeforeScreenTimeout} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinutesOfInactivityBeforeScreenTimeout(Integer passwordMinutesOfInactivityBeforeScreenTimeout) {
this.passwordMinutesOfInactivityBeforeScreenTimeout = passwordMinutesOfInactivityBeforeScreenTimeout;
this.changedFields = changedFields.add("passwordMinutesOfInactivityBeforeScreenTimeout");
return this;
}
/**
* “Number of previous passwords to block. Valid values 0 to 24”
*
* @param passwordPreviousPasswordBlockCount
* value of {@code passwordPreviousPasswordBlockCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordPreviousPasswordBlockCount(Integer passwordPreviousPasswordBlockCount) {
this.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
this.changedFields = changedFields.add("passwordPreviousPasswordBlockCount");
return this;
}
/**
* “Indicates whether or not to require a password.”
*
* @param passwordRequired
* value of {@code passwordRequired} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordRequired(Boolean passwordRequired) {
this.passwordRequired = passwordRequired;
this.changedFields = changedFields.add("passwordRequired");
return this;
}
/**
* “Password type that is required.”
*
* @param passwordRequiredType
* value of {@code passwordRequiredType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordRequiredType(RequiredPasswordType passwordRequiredType) {
this.passwordRequiredType = passwordRequiredType;
this.changedFields = changedFields.add("passwordRequiredType");
return this;
}
/**
* “Number of sign in failures allowed before factory reset.”
*
* @param passwordSignInFailureCountBeforeFactoryReset
* value of {@code passwordSignInFailureCountBeforeFactoryReset} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordSignInFailureCountBeforeFactoryReset(Integer passwordSignInFailureCountBeforeFactoryReset) {
this.passwordSignInFailureCountBeforeFactoryReset = passwordSignInFailureCountBeforeFactoryReset;
this.changedFields = changedFields.add("passwordSignInFailureCountBeforeFactoryReset");
return this;
}
/**
* “Indicates whether or not to block screenshots.”
*
* @param screenCaptureBlocked
* value of {@code screenCaptureBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder screenCaptureBlocked(Boolean screenCaptureBlocked) {
this.screenCaptureBlocked = screenCaptureBlocked;
this.changedFields = changedFields.add("screenCaptureBlocked");
return this;
}
/**
* “Indicates whether or not to block removable storage.”
*
* @param storageBlockRemovableStorage
* value of {@code storageBlockRemovableStorage} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder storageBlockRemovableStorage(Boolean storageBlockRemovableStorage) {
this.storageBlockRemovableStorage = storageBlockRemovableStorage;
this.changedFields = changedFields.add("storageBlockRemovableStorage");
return this;
}
/**
* “Indicates whether or not to require encryption.”
*
* @param storageRequireEncryption
* value of {@code storageRequireEncryption} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder storageRequireEncryption(Boolean storageRequireEncryption) {
this.storageRequireEncryption = storageRequireEncryption;
this.changedFields = changedFields.add("storageRequireEncryption");
return this;
}
/**
* “Indicates whether or not to block the web browser.”
*
* @param webBrowserBlocked
* value of {@code webBrowserBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder webBrowserBlocked(Boolean webBrowserBlocked) {
this.webBrowserBlocked = webBrowserBlocked;
this.changedFields = changedFields.add("webBrowserBlocked");
return this;
}
/**
* “Indicates whether or not to block automatically connecting to Wi-Fi hotspots.
* Has no impact if Wi-Fi is blocked.”
*
* @param wifiBlockAutomaticConnectHotspots
* value of {@code wifiBlockAutomaticConnectHotspots} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder wifiBlockAutomaticConnectHotspots(Boolean wifiBlockAutomaticConnectHotspots) {
this.wifiBlockAutomaticConnectHotspots = wifiBlockAutomaticConnectHotspots;
this.changedFields = changedFields.add("wifiBlockAutomaticConnectHotspots");
return this;
}
/**
* “Indicates whether or not to block Wi-Fi.”
*
* @param wifiBlocked
* value of {@code wifiBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder wifiBlocked(Boolean wifiBlocked) {
this.wifiBlocked = wifiBlocked;
this.changedFields = changedFields.add("wifiBlocked");
return this;
}
/**
* “Indicates whether or not to block Wi-Fi hotspot reporting. Has no impact if Wi-
* Fi is blocked.”
*
* @param wifiBlockHotspotReporting
* value of {@code wifiBlockHotspotReporting} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder wifiBlockHotspotReporting(Boolean wifiBlockHotspotReporting) {
this.wifiBlockHotspotReporting = wifiBlockHotspotReporting;
this.changedFields = changedFields.add("wifiBlockHotspotReporting");
return this;
}
/**
* “Indicates whether or not to block the Windows Store.”
*
* @param windowsStoreBlocked
* value of {@code windowsStoreBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder windowsStoreBlocked(Boolean windowsStoreBlocked) {
this.windowsStoreBlocked = windowsStoreBlocked;
this.changedFields = changedFields.add("windowsStoreBlocked");
return this;
}
public WindowsPhone81GeneralConfiguration build() {
WindowsPhone81GeneralConfiguration _x = new WindowsPhone81GeneralConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.windowsPhone81GeneralConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.version = version;
_x.assignments = assignments;
_x.deviceSettingStateSummaries = deviceSettingStateSummaries;
_x.deviceStatuses = deviceStatuses;
_x.deviceStatusOverview = deviceStatusOverview;
_x.userStatuses = userStatuses;
_x.userStatusOverview = userStatusOverview;
_x.applyOnlyToWindowsPhone81 = applyOnlyToWindowsPhone81;
_x.appsBlockCopyPaste = appsBlockCopyPaste;
_x.bluetoothBlocked = bluetoothBlocked;
_x.cameraBlocked = cameraBlocked;
_x.cellularBlockWifiTethering = cellularBlockWifiTethering;
_x.compliantAppListType = compliantAppListType;
_x.compliantAppsList = compliantAppsList;
_x.compliantAppsListNextLink = compliantAppsListNextLink;
_x.diagnosticDataBlockSubmission = diagnosticDataBlockSubmission;
_x.emailBlockAddingAccounts = emailBlockAddingAccounts;
_x.locationServicesBlocked = locationServicesBlocked;
_x.microsoftAccountBlocked = microsoftAccountBlocked;
_x.nfcBlocked = nfcBlocked;
_x.passwordBlockSimple = passwordBlockSimple;
_x.passwordExpirationDays = passwordExpirationDays;
_x.passwordMinimumCharacterSetCount = passwordMinimumCharacterSetCount;
_x.passwordMinimumLength = passwordMinimumLength;
_x.passwordMinutesOfInactivityBeforeScreenTimeout = passwordMinutesOfInactivityBeforeScreenTimeout;
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
_x.passwordRequired = passwordRequired;
_x.passwordRequiredType = passwordRequiredType;
_x.passwordSignInFailureCountBeforeFactoryReset = passwordSignInFailureCountBeforeFactoryReset;
_x.screenCaptureBlocked = screenCaptureBlocked;
_x.storageBlockRemovableStorage = storageBlockRemovableStorage;
_x.storageRequireEncryption = storageRequireEncryption;
_x.webBrowserBlocked = webBrowserBlocked;
_x.wifiBlockAutomaticConnectHotspots = wifiBlockAutomaticConnectHotspots;
_x.wifiBlocked = wifiBlocked;
_x.wifiBlockHotspotReporting = wifiBlockHotspotReporting;
_x.windowsStoreBlocked = windowsStoreBlocked;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “Value indicating whether this policy only applies to Windows Phone 8.1. This
* property is read-only.”
*
* Org.OData.Core.V1.Computed
*
* true
*
* Org.OData.Core.V1.Permissions
*
* @return property applyOnlyToWindowsPhone81
*/
@Property(name="applyOnlyToWindowsPhone81")
@JsonIgnore
public Optional getApplyOnlyToWindowsPhone81() {
return Optional.ofNullable(applyOnlyToWindowsPhone81);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* applyOnlyToWindowsPhone81} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Value indicating whether this policy only applies to Windows Phone 8.1. This
* property is read-only.”
*
* Org.OData.Core.V1.Computed
*
* true
*
* Org.OData.Core.V1.Permissions
*
* @param applyOnlyToWindowsPhone81
* new value of {@code applyOnlyToWindowsPhone81} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code applyOnlyToWindowsPhone81} field changed
*/
public WindowsPhone81GeneralConfiguration withApplyOnlyToWindowsPhone81(Boolean applyOnlyToWindowsPhone81) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("applyOnlyToWindowsPhone81");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.applyOnlyToWindowsPhone81 = applyOnlyToWindowsPhone81;
return _x;
}
/**
* “Indicates whether or not to block copy paste.”
*
* @return property appsBlockCopyPaste
*/
@Property(name="appsBlockCopyPaste")
@JsonIgnore
public Optional getAppsBlockCopyPaste() {
return Optional.ofNullable(appsBlockCopyPaste);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* appsBlockCopyPaste} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Indicates whether or not to block copy paste.”
*
* @param appsBlockCopyPaste
* new value of {@code appsBlockCopyPaste} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code appsBlockCopyPaste} field changed
*/
public WindowsPhone81GeneralConfiguration withAppsBlockCopyPaste(Boolean appsBlockCopyPaste) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("appsBlockCopyPaste");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.appsBlockCopyPaste = appsBlockCopyPaste;
return _x;
}
/**
* “Indicates whether or not to block bluetooth.”
*
* @return property bluetoothBlocked
*/
@Property(name="bluetoothBlocked")
@JsonIgnore
public Optional getBluetoothBlocked() {
return Optional.ofNullable(bluetoothBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code bluetoothBlocked}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether or not to block bluetooth.”
*
* @param bluetoothBlocked
* new value of {@code bluetoothBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code bluetoothBlocked} field changed
*/
public WindowsPhone81GeneralConfiguration withBluetoothBlocked(Boolean bluetoothBlocked) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("bluetoothBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.bluetoothBlocked = bluetoothBlocked;
return _x;
}
/**
* “Indicates whether or not to block camera.”
*
* @return property cameraBlocked
*/
@Property(name="cameraBlocked")
@JsonIgnore
public Optional getCameraBlocked() {
return Optional.ofNullable(cameraBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code cameraBlocked}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether or not to block camera.”
*
* @param cameraBlocked
* new value of {@code cameraBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code cameraBlocked} field changed
*/
public WindowsPhone81GeneralConfiguration withCameraBlocked(Boolean cameraBlocked) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("cameraBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.cameraBlocked = cameraBlocked;
return _x;
}
/**
* “Indicates whether or not to block Wi-Fi tethering. Has no impact if Wi-Fi is
* blocked.”
*
* @return property cellularBlockWifiTethering
*/
@Property(name="cellularBlockWifiTethering")
@JsonIgnore
public Optional getCellularBlockWifiTethering() {
return Optional.ofNullable(cellularBlockWifiTethering);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* cellularBlockWifiTethering} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to block Wi-Fi tethering. Has no impact if Wi-Fi is
* blocked.”
*
* @param cellularBlockWifiTethering
* new value of {@code cellularBlockWifiTethering} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code cellularBlockWifiTethering} field changed
*/
public WindowsPhone81GeneralConfiguration withCellularBlockWifiTethering(Boolean cellularBlockWifiTethering) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("cellularBlockWifiTethering");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.cellularBlockWifiTethering = cellularBlockWifiTethering;
return _x;
}
/**
* “List that is in the AppComplianceList.”
*
* @return property compliantAppListType
*/
@Property(name="compliantAppListType")
@JsonIgnore
public Optional getCompliantAppListType() {
return Optional.ofNullable(compliantAppListType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* compliantAppListType} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “List that is in the AppComplianceList.”
*
* @param compliantAppListType
* new value of {@code compliantAppListType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code compliantAppListType} field changed
*/
public WindowsPhone81GeneralConfiguration withCompliantAppListType(AppListType compliantAppListType) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("compliantAppListType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.compliantAppListType = compliantAppListType;
return _x;
}
/**
* “List of apps in the compliance (either allow list or block list, controlled by
* CompliantAppListType). This collection can contain a maximum of 10000 elements.”
*
* @return property compliantAppsList
*/
@Property(name="compliantAppsList")
@JsonIgnore
public CollectionPage getCompliantAppsList() {
return new CollectionPage(contextPath, AppListItem.class, this.compliantAppsList, Optional.ofNullable(compliantAppsListNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code compliantAppsList
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “List of apps in the compliance (either allow list or block list, controlled by
* CompliantAppListType). This collection can contain a maximum of 10000 elements.”
*
* @param compliantAppsList
* new value of {@code compliantAppsList} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code compliantAppsList} field changed
*/
public WindowsPhone81GeneralConfiguration withCompliantAppsList(List compliantAppsList) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("compliantAppsList");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.compliantAppsList = compliantAppsList;
return _x;
}
/**
* “List of apps in the compliance (either allow list or block list, controlled by
* CompliantAppListType). This collection can contain a maximum of 10000 elements.”
*
* @param options
* specify connect and read timeouts
* @return property compliantAppsList
*/
@Property(name="compliantAppsList")
@JsonIgnore
public CollectionPage getCompliantAppsList(HttpRequestOptions options) {
return new CollectionPage(contextPath, AppListItem.class, this.compliantAppsList, Optional.ofNullable(compliantAppsListNextLink), Collections.emptyList(), options);
}
/**
* “Indicates whether or not to block diagnostic data submission.”
*
* @return property diagnosticDataBlockSubmission
*/
@Property(name="diagnosticDataBlockSubmission")
@JsonIgnore
public Optional getDiagnosticDataBlockSubmission() {
return Optional.ofNullable(diagnosticDataBlockSubmission);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* diagnosticDataBlockSubmission} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to block diagnostic data submission.”
*
* @param diagnosticDataBlockSubmission
* new value of {@code diagnosticDataBlockSubmission} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code diagnosticDataBlockSubmission} field changed
*/
public WindowsPhone81GeneralConfiguration withDiagnosticDataBlockSubmission(Boolean diagnosticDataBlockSubmission) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("diagnosticDataBlockSubmission");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.diagnosticDataBlockSubmission = diagnosticDataBlockSubmission;
return _x;
}
/**
* “Indicates whether or not to block custom email accounts.”
*
* @return property emailBlockAddingAccounts
*/
@Property(name="emailBlockAddingAccounts")
@JsonIgnore
public Optional getEmailBlockAddingAccounts() {
return Optional.ofNullable(emailBlockAddingAccounts);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* emailBlockAddingAccounts} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to block custom email accounts.”
*
* @param emailBlockAddingAccounts
* new value of {@code emailBlockAddingAccounts} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code emailBlockAddingAccounts} field changed
*/
public WindowsPhone81GeneralConfiguration withEmailBlockAddingAccounts(Boolean emailBlockAddingAccounts) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("emailBlockAddingAccounts");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.emailBlockAddingAccounts = emailBlockAddingAccounts;
return _x;
}
/**
* “Indicates whether or not to block location services.”
*
* @return property locationServicesBlocked
*/
@Property(name="locationServicesBlocked")
@JsonIgnore
public Optional getLocationServicesBlocked() {
return Optional.ofNullable(locationServicesBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* locationServicesBlocked} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to block location services.”
*
* @param locationServicesBlocked
* new value of {@code locationServicesBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code locationServicesBlocked} field changed
*/
public WindowsPhone81GeneralConfiguration withLocationServicesBlocked(Boolean locationServicesBlocked) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("locationServicesBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.locationServicesBlocked = locationServicesBlocked;
return _x;
}
/**
* “Indicates whether or not to block using a Microsoft Account.”
*
* @return property microsoftAccountBlocked
*/
@Property(name="microsoftAccountBlocked")
@JsonIgnore
public Optional getMicrosoftAccountBlocked() {
return Optional.ofNullable(microsoftAccountBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* microsoftAccountBlocked} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to block using a Microsoft Account.”
*
* @param microsoftAccountBlocked
* new value of {@code microsoftAccountBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code microsoftAccountBlocked} field changed
*/
public WindowsPhone81GeneralConfiguration withMicrosoftAccountBlocked(Boolean microsoftAccountBlocked) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("microsoftAccountBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.microsoftAccountBlocked = microsoftAccountBlocked;
return _x;
}
/**
* “Indicates whether or not to block Near-Field Communication.”
*
* @return property nfcBlocked
*/
@Property(name="nfcBlocked")
@JsonIgnore
public Optional getNfcBlocked() {
return Optional.ofNullable(nfcBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code nfcBlocked} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Indicates whether or not to block Near-Field Communication.”
*
* @param nfcBlocked
* new value of {@code nfcBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code nfcBlocked} field changed
*/
public WindowsPhone81GeneralConfiguration withNfcBlocked(Boolean nfcBlocked) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("nfcBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.nfcBlocked = nfcBlocked;
return _x;
}
/**
* “Indicates whether or not to block syncing the calendar.”
*
* @return property passwordBlockSimple
*/
@Property(name="passwordBlockSimple")
@JsonIgnore
public Optional getPasswordBlockSimple() {
return Optional.ofNullable(passwordBlockSimple);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordBlockSimple} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Indicates whether or not to block syncing the calendar.”
*
* @param passwordBlockSimple
* new value of {@code passwordBlockSimple} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordBlockSimple} field changed
*/
public WindowsPhone81GeneralConfiguration withPasswordBlockSimple(Boolean passwordBlockSimple) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordBlockSimple");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.passwordBlockSimple = passwordBlockSimple;
return _x;
}
/**
* “Number of days before the password expires.”
*
* @return property passwordExpirationDays
*/
@Property(name="passwordExpirationDays")
@JsonIgnore
public Optional getPasswordExpirationDays() {
return Optional.ofNullable(passwordExpirationDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordExpirationDays} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Number of days before the password expires.”
*
* @param passwordExpirationDays
* new value of {@code passwordExpirationDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordExpirationDays} field changed
*/
public WindowsPhone81GeneralConfiguration withPasswordExpirationDays(Integer passwordExpirationDays) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordExpirationDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.passwordExpirationDays = passwordExpirationDays;
return _x;
}
/**
* “Number of character sets a password must contain.”
*
* @return property passwordMinimumCharacterSetCount
*/
@Property(name="passwordMinimumCharacterSetCount")
@JsonIgnore
public Optional getPasswordMinimumCharacterSetCount() {
return Optional.ofNullable(passwordMinimumCharacterSetCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinimumCharacterSetCount} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Number of character sets a password must contain.”
*
* @param passwordMinimumCharacterSetCount
* new value of {@code passwordMinimumCharacterSetCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinimumCharacterSetCount} field changed
*/
public WindowsPhone81GeneralConfiguration withPasswordMinimumCharacterSetCount(Integer passwordMinimumCharacterSetCount) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordMinimumCharacterSetCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.passwordMinimumCharacterSetCount = passwordMinimumCharacterSetCount;
return _x;
}
/**
* “Minimum length of passwords.”
*
* @return property passwordMinimumLength
*/
@Property(name="passwordMinimumLength")
@JsonIgnore
public Optional getPasswordMinimumLength() {
return Optional.ofNullable(passwordMinimumLength);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinimumLength} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Minimum length of passwords.”
*
* @param passwordMinimumLength
* new value of {@code passwordMinimumLength} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinimumLength} field changed
*/
public WindowsPhone81GeneralConfiguration withPasswordMinimumLength(Integer passwordMinimumLength) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordMinimumLength");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.passwordMinimumLength = passwordMinimumLength;
return _x;
}
/**
* “Minutes of inactivity before screen timeout.”
*
* @return property passwordMinutesOfInactivityBeforeScreenTimeout
*/
@Property(name="passwordMinutesOfInactivityBeforeScreenTimeout")
@JsonIgnore
public Optional getPasswordMinutesOfInactivityBeforeScreenTimeout() {
return Optional.ofNullable(passwordMinutesOfInactivityBeforeScreenTimeout);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinutesOfInactivityBeforeScreenTimeout} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Minutes of inactivity before screen timeout.”
*
* @param passwordMinutesOfInactivityBeforeScreenTimeout
* new value of {@code passwordMinutesOfInactivityBeforeScreenTimeout} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinutesOfInactivityBeforeScreenTimeout} field changed
*/
public WindowsPhone81GeneralConfiguration withPasswordMinutesOfInactivityBeforeScreenTimeout(Integer passwordMinutesOfInactivityBeforeScreenTimeout) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordMinutesOfInactivityBeforeScreenTimeout");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.passwordMinutesOfInactivityBeforeScreenTimeout = passwordMinutesOfInactivityBeforeScreenTimeout;
return _x;
}
/**
* “Number of previous passwords to block. Valid values 0 to 24”
*
* @return property passwordPreviousPasswordBlockCount
*/
@Property(name="passwordPreviousPasswordBlockCount")
@JsonIgnore
public Optional getPasswordPreviousPasswordBlockCount() {
return Optional.ofNullable(passwordPreviousPasswordBlockCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordPreviousPasswordBlockCount} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Number of previous passwords to block. Valid values 0 to 24”
*
* @param passwordPreviousPasswordBlockCount
* new value of {@code passwordPreviousPasswordBlockCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordPreviousPasswordBlockCount} field changed
*/
public WindowsPhone81GeneralConfiguration withPasswordPreviousPasswordBlockCount(Integer passwordPreviousPasswordBlockCount) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordPreviousPasswordBlockCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
return _x;
}
/**
* “Indicates whether or not to require a password.”
*
* @return property passwordRequired
*/
@Property(name="passwordRequired")
@JsonIgnore
public Optional getPasswordRequired() {
return Optional.ofNullable(passwordRequired);
}
/**
* Returns an immutable copy of {@code this} with just the {@code passwordRequired}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether or not to require a password.”
*
* @param passwordRequired
* new value of {@code passwordRequired} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordRequired} field changed
*/
public WindowsPhone81GeneralConfiguration withPasswordRequired(Boolean passwordRequired) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordRequired");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.passwordRequired = passwordRequired;
return _x;
}
/**
* “Password type that is required.”
*
* @return property passwordRequiredType
*/
@Property(name="passwordRequiredType")
@JsonIgnore
public Optional getPasswordRequiredType() {
return Optional.ofNullable(passwordRequiredType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordRequiredType} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Password type that is required.”
*
* @param passwordRequiredType
* new value of {@code passwordRequiredType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordRequiredType} field changed
*/
public WindowsPhone81GeneralConfiguration withPasswordRequiredType(RequiredPasswordType passwordRequiredType) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordRequiredType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.passwordRequiredType = passwordRequiredType;
return _x;
}
/**
* “Number of sign in failures allowed before factory reset.”
*
* @return property passwordSignInFailureCountBeforeFactoryReset
*/
@Property(name="passwordSignInFailureCountBeforeFactoryReset")
@JsonIgnore
public Optional getPasswordSignInFailureCountBeforeFactoryReset() {
return Optional.ofNullable(passwordSignInFailureCountBeforeFactoryReset);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordSignInFailureCountBeforeFactoryReset} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Number of sign in failures allowed before factory reset.”
*
* @param passwordSignInFailureCountBeforeFactoryReset
* new value of {@code passwordSignInFailureCountBeforeFactoryReset} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordSignInFailureCountBeforeFactoryReset} field changed
*/
public WindowsPhone81GeneralConfiguration withPasswordSignInFailureCountBeforeFactoryReset(Integer passwordSignInFailureCountBeforeFactoryReset) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordSignInFailureCountBeforeFactoryReset");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.passwordSignInFailureCountBeforeFactoryReset = passwordSignInFailureCountBeforeFactoryReset;
return _x;
}
/**
* “Indicates whether or not to block screenshots.”
*
* @return property screenCaptureBlocked
*/
@Property(name="screenCaptureBlocked")
@JsonIgnore
public Optional getScreenCaptureBlocked() {
return Optional.ofNullable(screenCaptureBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* screenCaptureBlocked} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Indicates whether or not to block screenshots.”
*
* @param screenCaptureBlocked
* new value of {@code screenCaptureBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code screenCaptureBlocked} field changed
*/
public WindowsPhone81GeneralConfiguration withScreenCaptureBlocked(Boolean screenCaptureBlocked) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("screenCaptureBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.screenCaptureBlocked = screenCaptureBlocked;
return _x;
}
/**
* “Indicates whether or not to block removable storage.”
*
* @return property storageBlockRemovableStorage
*/
@Property(name="storageBlockRemovableStorage")
@JsonIgnore
public Optional getStorageBlockRemovableStorage() {
return Optional.ofNullable(storageBlockRemovableStorage);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* storageBlockRemovableStorage} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to block removable storage.”
*
* @param storageBlockRemovableStorage
* new value of {@code storageBlockRemovableStorage} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code storageBlockRemovableStorage} field changed
*/
public WindowsPhone81GeneralConfiguration withStorageBlockRemovableStorage(Boolean storageBlockRemovableStorage) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("storageBlockRemovableStorage");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.storageBlockRemovableStorage = storageBlockRemovableStorage;
return _x;
}
/**
* “Indicates whether or not to require encryption.”
*
* @return property storageRequireEncryption
*/
@Property(name="storageRequireEncryption")
@JsonIgnore
public Optional getStorageRequireEncryption() {
return Optional.ofNullable(storageRequireEncryption);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* storageRequireEncryption} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to require encryption.”
*
* @param storageRequireEncryption
* new value of {@code storageRequireEncryption} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code storageRequireEncryption} field changed
*/
public WindowsPhone81GeneralConfiguration withStorageRequireEncryption(Boolean storageRequireEncryption) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("storageRequireEncryption");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.storageRequireEncryption = storageRequireEncryption;
return _x;
}
/**
* “Indicates whether or not to block the web browser.”
*
* @return property webBrowserBlocked
*/
@Property(name="webBrowserBlocked")
@JsonIgnore
public Optional getWebBrowserBlocked() {
return Optional.ofNullable(webBrowserBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code webBrowserBlocked
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether or not to block the web browser.”
*
* @param webBrowserBlocked
* new value of {@code webBrowserBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code webBrowserBlocked} field changed
*/
public WindowsPhone81GeneralConfiguration withWebBrowserBlocked(Boolean webBrowserBlocked) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("webBrowserBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.webBrowserBlocked = webBrowserBlocked;
return _x;
}
/**
* “Indicates whether or not to block automatically connecting to Wi-Fi hotspots.
* Has no impact if Wi-Fi is blocked.”
*
* @return property wifiBlockAutomaticConnectHotspots
*/
@Property(name="wifiBlockAutomaticConnectHotspots")
@JsonIgnore
public Optional getWifiBlockAutomaticConnectHotspots() {
return Optional.ofNullable(wifiBlockAutomaticConnectHotspots);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* wifiBlockAutomaticConnectHotspots} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates whether or not to block automatically connecting to Wi-Fi hotspots.
* Has no impact if Wi-Fi is blocked.”
*
* @param wifiBlockAutomaticConnectHotspots
* new value of {@code wifiBlockAutomaticConnectHotspots} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code wifiBlockAutomaticConnectHotspots} field changed
*/
public WindowsPhone81GeneralConfiguration withWifiBlockAutomaticConnectHotspots(Boolean wifiBlockAutomaticConnectHotspots) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("wifiBlockAutomaticConnectHotspots");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.wifiBlockAutomaticConnectHotspots = wifiBlockAutomaticConnectHotspots;
return _x;
}
/**
* “Indicates whether or not to block Wi-Fi.”
*
* @return property wifiBlocked
*/
@Property(name="wifiBlocked")
@JsonIgnore
public Optional getWifiBlocked() {
return Optional.ofNullable(wifiBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code wifiBlocked}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether or not to block Wi-Fi.”
*
* @param wifiBlocked
* new value of {@code wifiBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code wifiBlocked} field changed
*/
public WindowsPhone81GeneralConfiguration withWifiBlocked(Boolean wifiBlocked) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("wifiBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.wifiBlocked = wifiBlocked;
return _x;
}
/**
* “Indicates whether or not to block Wi-Fi hotspot reporting. Has no impact if Wi-
* Fi is blocked.”
*
* @return property wifiBlockHotspotReporting
*/
@Property(name="wifiBlockHotspotReporting")
@JsonIgnore
public Optional getWifiBlockHotspotReporting() {
return Optional.ofNullable(wifiBlockHotspotReporting);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* wifiBlockHotspotReporting} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to block Wi-Fi hotspot reporting. Has no impact if Wi-
* Fi is blocked.”
*
* @param wifiBlockHotspotReporting
* new value of {@code wifiBlockHotspotReporting} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code wifiBlockHotspotReporting} field changed
*/
public WindowsPhone81GeneralConfiguration withWifiBlockHotspotReporting(Boolean wifiBlockHotspotReporting) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("wifiBlockHotspotReporting");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.wifiBlockHotspotReporting = wifiBlockHotspotReporting;
return _x;
}
/**
* “Indicates whether or not to block the Windows Store.”
*
* @return property windowsStoreBlocked
*/
@Property(name="windowsStoreBlocked")
@JsonIgnore
public Optional getWindowsStoreBlocked() {
return Optional.ofNullable(windowsStoreBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* windowsStoreBlocked} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Indicates whether or not to block the Windows Store.”
*
* @param windowsStoreBlocked
* new value of {@code windowsStoreBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code windowsStoreBlocked} field changed
*/
public WindowsPhone81GeneralConfiguration withWindowsStoreBlocked(Boolean windowsStoreBlocked) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = changedFields.add("windowsStoreBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsPhone81GeneralConfiguration");
_x.windowsStoreBlocked = windowsStoreBlocked;
return _x;
}
public WindowsPhone81GeneralConfiguration withUnmappedField(String name, Object value) {
WindowsPhone81GeneralConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsPhone81GeneralConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsPhone81GeneralConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
WindowsPhone81GeneralConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private WindowsPhone81GeneralConfiguration _copy() {
WindowsPhone81GeneralConfiguration _x = new WindowsPhone81GeneralConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.version = version;
_x.assignments = assignments;
_x.deviceSettingStateSummaries = deviceSettingStateSummaries;
_x.deviceStatuses = deviceStatuses;
_x.deviceStatusOverview = deviceStatusOverview;
_x.userStatuses = userStatuses;
_x.userStatusOverview = userStatusOverview;
_x.applyOnlyToWindowsPhone81 = applyOnlyToWindowsPhone81;
_x.appsBlockCopyPaste = appsBlockCopyPaste;
_x.bluetoothBlocked = bluetoothBlocked;
_x.cameraBlocked = cameraBlocked;
_x.cellularBlockWifiTethering = cellularBlockWifiTethering;
_x.compliantAppListType = compliantAppListType;
_x.compliantAppsList = compliantAppsList;
_x.diagnosticDataBlockSubmission = diagnosticDataBlockSubmission;
_x.emailBlockAddingAccounts = emailBlockAddingAccounts;
_x.locationServicesBlocked = locationServicesBlocked;
_x.microsoftAccountBlocked = microsoftAccountBlocked;
_x.nfcBlocked = nfcBlocked;
_x.passwordBlockSimple = passwordBlockSimple;
_x.passwordExpirationDays = passwordExpirationDays;
_x.passwordMinimumCharacterSetCount = passwordMinimumCharacterSetCount;
_x.passwordMinimumLength = passwordMinimumLength;
_x.passwordMinutesOfInactivityBeforeScreenTimeout = passwordMinutesOfInactivityBeforeScreenTimeout;
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
_x.passwordRequired = passwordRequired;
_x.passwordRequiredType = passwordRequiredType;
_x.passwordSignInFailureCountBeforeFactoryReset = passwordSignInFailureCountBeforeFactoryReset;
_x.screenCaptureBlocked = screenCaptureBlocked;
_x.storageBlockRemovableStorage = storageBlockRemovableStorage;
_x.storageRequireEncryption = storageRequireEncryption;
_x.webBrowserBlocked = webBrowserBlocked;
_x.wifiBlockAutomaticConnectHotspots = wifiBlockAutomaticConnectHotspots;
_x.wifiBlocked = wifiBlocked;
_x.wifiBlockHotspotReporting = wifiBlockHotspotReporting;
_x.windowsStoreBlocked = windowsStoreBlocked;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("WindowsPhone81GeneralConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("assignments=");
b.append(this.assignments);
b.append(", ");
b.append("deviceSettingStateSummaries=");
b.append(this.deviceSettingStateSummaries);
b.append(", ");
b.append("deviceStatuses=");
b.append(this.deviceStatuses);
b.append(", ");
b.append("deviceStatusOverview=");
b.append(this.deviceStatusOverview);
b.append(", ");
b.append("userStatuses=");
b.append(this.userStatuses);
b.append(", ");
b.append("userStatusOverview=");
b.append(this.userStatusOverview);
b.append(", ");
b.append("applyOnlyToWindowsPhone81=");
b.append(this.applyOnlyToWindowsPhone81);
b.append(", ");
b.append("appsBlockCopyPaste=");
b.append(this.appsBlockCopyPaste);
b.append(", ");
b.append("bluetoothBlocked=");
b.append(this.bluetoothBlocked);
b.append(", ");
b.append("cameraBlocked=");
b.append(this.cameraBlocked);
b.append(", ");
b.append("cellularBlockWifiTethering=");
b.append(this.cellularBlockWifiTethering);
b.append(", ");
b.append("compliantAppListType=");
b.append(this.compliantAppListType);
b.append(", ");
b.append("compliantAppsList=");
b.append(this.compliantAppsList);
b.append(", ");
b.append("diagnosticDataBlockSubmission=");
b.append(this.diagnosticDataBlockSubmission);
b.append(", ");
b.append("emailBlockAddingAccounts=");
b.append(this.emailBlockAddingAccounts);
b.append(", ");
b.append("locationServicesBlocked=");
b.append(this.locationServicesBlocked);
b.append(", ");
b.append("microsoftAccountBlocked=");
b.append(this.microsoftAccountBlocked);
b.append(", ");
b.append("nfcBlocked=");
b.append(this.nfcBlocked);
b.append(", ");
b.append("passwordBlockSimple=");
b.append(this.passwordBlockSimple);
b.append(", ");
b.append("passwordExpirationDays=");
b.append(this.passwordExpirationDays);
b.append(", ");
b.append("passwordMinimumCharacterSetCount=");
b.append(this.passwordMinimumCharacterSetCount);
b.append(", ");
b.append("passwordMinimumLength=");
b.append(this.passwordMinimumLength);
b.append(", ");
b.append("passwordMinutesOfInactivityBeforeScreenTimeout=");
b.append(this.passwordMinutesOfInactivityBeforeScreenTimeout);
b.append(", ");
b.append("passwordPreviousPasswordBlockCount=");
b.append(this.passwordPreviousPasswordBlockCount);
b.append(", ");
b.append("passwordRequired=");
b.append(this.passwordRequired);
b.append(", ");
b.append("passwordRequiredType=");
b.append(this.passwordRequiredType);
b.append(", ");
b.append("passwordSignInFailureCountBeforeFactoryReset=");
b.append(this.passwordSignInFailureCountBeforeFactoryReset);
b.append(", ");
b.append("screenCaptureBlocked=");
b.append(this.screenCaptureBlocked);
b.append(", ");
b.append("storageBlockRemovableStorage=");
b.append(this.storageBlockRemovableStorage);
b.append(", ");
b.append("storageRequireEncryption=");
b.append(this.storageRequireEncryption);
b.append(", ");
b.append("webBrowserBlocked=");
b.append(this.webBrowserBlocked);
b.append(", ");
b.append("wifiBlockAutomaticConnectHotspots=");
b.append(this.wifiBlockAutomaticConnectHotspots);
b.append(", ");
b.append("wifiBlocked=");
b.append(this.wifiBlocked);
b.append(", ");
b.append("wifiBlockHotspotReporting=");
b.append(this.wifiBlockHotspotReporting);
b.append(", ");
b.append("windowsStoreBlocked=");
b.append(this.windowsStoreBlocked);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}