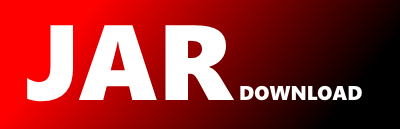
odata.msgraph.client.identitygovernance.entity.Task Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.identitygovernance.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.complex.KeyValuePair;
import odata.msgraph.client.entity.Entity;
import odata.msgraph.client.identitygovernance.entity.collection.request.TaskProcessingResultCollectionRequest;
import odata.msgraph.client.identitygovernance.enums.LifecycleTaskCategory;
/**
*
* Org.OData.Capabilities.V1.CountRestrictions
*
* Countable = true
*
* Org.OData.Capabilities.V1.ExpandRestrictions
*
* Expandable = true
*
* Org.OData.Capabilities.V1.FilterRestrictions
*
* Filterable = true
*
* Org.OData.Capabilities.V1.SearchRestrictions
*
* Searchable = true
*
* Org.OData.Capabilities.V1.SelectRestrictions
*
* Selectable = false
*
* Org.OData.Capabilities.V1.SkipSupported
*
* false
*
* Org.OData.Capabilities.V1.SortRestrictions
*
* Sortable = false
*
* Org.OData.Capabilities.V1.TopSupported
*
* false
*/@JsonPropertyOrder({
"@odata.type",
"arguments",
"category",
"continueOnError",
"description",
"displayName",
"executionSequence",
"isEnabled",
"taskDefinitionId",
"taskProcessingResults"})
@JsonInclude(Include.NON_NULL)
public class Task extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.identityGovernance.task";
}
@JsonProperty("arguments")
protected List arguments;
@JsonProperty("arguments@nextLink")
protected String argumentsNextLink;
@JsonProperty("category")
protected LifecycleTaskCategory category;
@JsonProperty("continueOnError")
protected Boolean continueOnError;
@JsonProperty("description")
protected String description;
@JsonProperty("displayName")
protected String displayName;
@JsonProperty("executionSequence")
protected Integer executionSequence;
@JsonProperty("isEnabled")
protected Boolean isEnabled;
@JsonProperty("taskDefinitionId")
protected String taskDefinitionId;
@JsonProperty("taskProcessingResults")
protected List taskProcessingResults;
protected Task() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderTask() {
return new Builder();
}
public static final class Builder {
private String id;
private List arguments;
private String argumentsNextLink;
private LifecycleTaskCategory category;
private Boolean continueOnError;
private String description;
private String displayName;
private Integer executionSequence;
private Boolean isEnabled;
private String taskDefinitionId;
private List taskProcessingResults;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder arguments(List arguments) {
this.arguments = arguments;
this.changedFields = changedFields.add("arguments");
return this;
}
public Builder arguments(KeyValuePair... arguments) {
return arguments(Arrays.asList(arguments));
}
public Builder argumentsNextLink(String argumentsNextLink) {
this.argumentsNextLink = argumentsNextLink;
this.changedFields = changedFields.add("arguments");
return this;
}
public Builder category(LifecycleTaskCategory category) {
this.category = category;
this.changedFields = changedFields.add("category");
return this;
}
public Builder continueOnError(Boolean continueOnError) {
this.continueOnError = continueOnError;
this.changedFields = changedFields.add("continueOnError");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder executionSequence(Integer executionSequence) {
this.executionSequence = executionSequence;
this.changedFields = changedFields.add("executionSequence");
return this;
}
public Builder isEnabled(Boolean isEnabled) {
this.isEnabled = isEnabled;
this.changedFields = changedFields.add("isEnabled");
return this;
}
public Builder taskDefinitionId(String taskDefinitionId) {
this.taskDefinitionId = taskDefinitionId;
this.changedFields = changedFields.add("taskDefinitionId");
return this;
}
/**
*
* Org.OData.Capabilities.V1.DeleteRestrictions
*
* Deletable = false
*
* Org.OData.Capabilities.V1.InsertRestrictions
*
* Insertable = false
*
* Org.OData.Capabilities.V1.UpdateRestrictions
*
* Updatable = false
*
* @param taskProcessingResults
* value of {@code taskProcessingResults} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder taskProcessingResults(List taskProcessingResults) {
this.taskProcessingResults = taskProcessingResults;
this.changedFields = changedFields.add("taskProcessingResults");
return this;
}
/**
*
* Org.OData.Capabilities.V1.DeleteRestrictions
*
* Deletable = false
*
* Org.OData.Capabilities.V1.InsertRestrictions
*
* Insertable = false
*
* Org.OData.Capabilities.V1.UpdateRestrictions
*
* Updatable = false
*
* @param taskProcessingResults
* value of {@code taskProcessingResults} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder taskProcessingResults(TaskProcessingResult... taskProcessingResults) {
return taskProcessingResults(Arrays.asList(taskProcessingResults));
}
public Task build() {
Task _x = new Task();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.identityGovernance.task";
_x.id = id;
_x.arguments = arguments;
_x.argumentsNextLink = argumentsNextLink;
_x.category = category;
_x.continueOnError = continueOnError;
_x.description = description;
_x.displayName = displayName;
_x.executionSequence = executionSequence;
_x.isEnabled = isEnabled;
_x.taskDefinitionId = taskDefinitionId;
_x.taskProcessingResults = taskProcessingResults;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
@Property(name="arguments")
@JsonIgnore
public CollectionPage getArguments() {
return new CollectionPage(contextPath, KeyValuePair.class, this.arguments, Optional.ofNullable(argumentsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public Task withArguments(List arguments) {
Task _x = _copy();
_x.changedFields = changedFields.add("arguments");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.task");
_x.arguments = arguments;
return _x;
}
@Property(name="arguments")
@JsonIgnore
public CollectionPage getArguments(HttpRequestOptions options) {
return new CollectionPage(contextPath, KeyValuePair.class, this.arguments, Optional.ofNullable(argumentsNextLink), Collections.emptyList(), options);
}
@Property(name="category")
@JsonIgnore
public Optional getCategory() {
return Optional.ofNullable(category);
}
public Task withCategory(LifecycleTaskCategory category) {
Task _x = _copy();
_x.changedFields = changedFields.add("category");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.task");
_x.category = category;
return _x;
}
@Property(name="continueOnError")
@JsonIgnore
public Optional getContinueOnError() {
return Optional.ofNullable(continueOnError);
}
public Task withContinueOnError(Boolean continueOnError) {
Task _x = _copy();
_x.changedFields = changedFields.add("continueOnError");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.task");
_x.continueOnError = continueOnError;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public Task withDescription(String description) {
Task _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.task");
_x.description = description;
return _x;
}
@Property(name="displayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
public Task withDisplayName(String displayName) {
Task _x = _copy();
_x.changedFields = changedFields.add("displayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.task");
_x.displayName = displayName;
return _x;
}
@Property(name="executionSequence")
@JsonIgnore
public Optional getExecutionSequence() {
return Optional.ofNullable(executionSequence);
}
public Task withExecutionSequence(Integer executionSequence) {
Task _x = _copy();
_x.changedFields = changedFields.add("executionSequence");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.task");
_x.executionSequence = executionSequence;
return _x;
}
@Property(name="isEnabled")
@JsonIgnore
public Optional getIsEnabled() {
return Optional.ofNullable(isEnabled);
}
public Task withIsEnabled(Boolean isEnabled) {
Task _x = _copy();
_x.changedFields = changedFields.add("isEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.task");
_x.isEnabled = isEnabled;
return _x;
}
@Property(name="taskDefinitionId")
@JsonIgnore
public Optional getTaskDefinitionId() {
return Optional.ofNullable(taskDefinitionId);
}
public Task withTaskDefinitionId(String taskDefinitionId) {
Task _x = _copy();
_x.changedFields = changedFields.add("taskDefinitionId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.task");
_x.taskDefinitionId = taskDefinitionId;
return _x;
}
public Task withUnmappedField(String name, Object value) {
Task _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
*
* Org.OData.Capabilities.V1.DeleteRestrictions
*
* Deletable = false
*
* Org.OData.Capabilities.V1.InsertRestrictions
*
* Insertable = false
*
* Org.OData.Capabilities.V1.UpdateRestrictions
*
* Updatable = false
*
* @return navigational property taskProcessingResults
*/
@NavigationProperty(name="taskProcessingResults")
@JsonIgnore
public TaskProcessingResultCollectionRequest getTaskProcessingResults() {
return new TaskProcessingResultCollectionRequest(
contextPath.addSegment("taskProcessingResults"), Optional.ofNullable(taskProcessingResults));
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* taskProcessingResults} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* Org.OData.Capabilities.V1.DeleteRestrictions
*
* Deletable = false
*
* Org.OData.Capabilities.V1.InsertRestrictions
*
* Insertable = false
*
* Org.OData.Capabilities.V1.UpdateRestrictions
*
* Updatable = false
*
* @param taskProcessingResults
* new value of {@code taskProcessingResults} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code taskProcessingResults} field changed
*/
public Task withTaskProcessingResults(List taskProcessingResults) {
Task _x = _copy();
_x.changedFields = changedFields.add("taskProcessingResults");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.task");
_x.taskProcessingResults = taskProcessingResults;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Task patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Task _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Task put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Task _x = _copy();
_x.changedFields = null;
return _x;
}
private Task _copy() {
Task _x = new Task();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.arguments = arguments;
_x.category = category;
_x.continueOnError = continueOnError;
_x.description = description;
_x.displayName = displayName;
_x.executionSequence = executionSequence;
_x.isEnabled = isEnabled;
_x.taskDefinitionId = taskDefinitionId;
_x.taskProcessingResults = taskProcessingResults;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Task[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("arguments=");
b.append(this.arguments);
b.append(", ");
b.append("category=");
b.append(this.category);
b.append(", ");
b.append("continueOnError=");
b.append(this.continueOnError);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("executionSequence=");
b.append(this.executionSequence);
b.append(", ");
b.append("isEnabled=");
b.append(this.isEnabled);
b.append(", ");
b.append("taskDefinitionId=");
b.append(this.taskDefinitionId);
b.append(", ");
b.append("taskProcessingResults=");
b.append(this.taskProcessingResults);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}