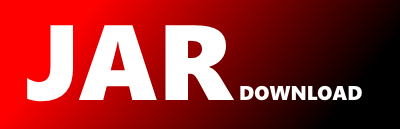
odata.msgraph.client.identitygovernance.entity.WorkflowBase Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.identitygovernance.entity;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.entity.request.UserRequest;
import odata.msgraph.client.identitygovernance.complex.WorkflowExecutionConditions;
import odata.msgraph.client.identitygovernance.entity.collection.request.TaskCollectionRequest;
import odata.msgraph.client.identitygovernance.enums.LifecycleWorkflowCategory;
/**
*
* Org.OData.Capabilities.V1.FilterRestrictions
*
* Filterable = true
*/@JsonPropertyOrder({
"@odata.type",
"category",
"createdDateTime",
"description",
"displayName",
"executionConditions",
"isEnabled",
"isSchedulingEnabled",
"lastModifiedDateTime",
"tasks"})
@JsonInclude(Include.NON_NULL)
public class WorkflowBase implements ODataEntityType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JacksonInject
@JsonIgnore
protected ChangedFields changedFields;
@Override
public String odataTypeName() {
return "microsoft.graph.identityGovernance.workflowBase";
}
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("category")
protected LifecycleWorkflowCategory category;
@JsonProperty("createdDateTime")
protected OffsetDateTime createdDateTime;
@JsonProperty("description")
protected String description;
@JsonProperty("displayName")
protected String displayName;
@JsonProperty("executionConditions")
protected WorkflowExecutionConditions executionConditions;
@JsonProperty("isEnabled")
protected Boolean isEnabled;
@JsonProperty("isSchedulingEnabled")
protected Boolean isSchedulingEnabled;
@JsonProperty("lastModifiedDateTime")
protected OffsetDateTime lastModifiedDateTime;
@JsonProperty("tasks")
protected List tasks;
protected WorkflowBase() {
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath) {
contextPath = contextPath.clearQueries();
}
}
@Property(name="category")
@JsonIgnore
public Optional getCategory() {
return Optional.ofNullable(category);
}
public WorkflowBase withCategory(LifecycleWorkflowCategory category) {
WorkflowBase _x = _copy();
_x.changedFields = changedFields.add("category");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.workflowBase");
_x.category = category;
return _x;
}
@Property(name="createdDateTime")
@JsonIgnore
public Optional getCreatedDateTime() {
return Optional.ofNullable(createdDateTime);
}
public WorkflowBase withCreatedDateTime(OffsetDateTime createdDateTime) {
WorkflowBase _x = _copy();
_x.changedFields = changedFields.add("createdDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.workflowBase");
_x.createdDateTime = createdDateTime;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public WorkflowBase withDescription(String description) {
WorkflowBase _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.workflowBase");
_x.description = description;
return _x;
}
@Property(name="displayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
public WorkflowBase withDisplayName(String displayName) {
WorkflowBase _x = _copy();
_x.changedFields = changedFields.add("displayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.workflowBase");
_x.displayName = displayName;
return _x;
}
@Property(name="executionConditions")
@JsonIgnore
public Optional getExecutionConditions() {
return Optional.ofNullable(executionConditions);
}
public WorkflowBase withExecutionConditions(WorkflowExecutionConditions executionConditions) {
WorkflowBase _x = _copy();
_x.changedFields = changedFields.add("executionConditions");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.workflowBase");
_x.executionConditions = executionConditions;
return _x;
}
@Property(name="isEnabled")
@JsonIgnore
public Optional getIsEnabled() {
return Optional.ofNullable(isEnabled);
}
public WorkflowBase withIsEnabled(Boolean isEnabled) {
WorkflowBase _x = _copy();
_x.changedFields = changedFields.add("isEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.workflowBase");
_x.isEnabled = isEnabled;
return _x;
}
@Property(name="isSchedulingEnabled")
@JsonIgnore
public Optional getIsSchedulingEnabled() {
return Optional.ofNullable(isSchedulingEnabled);
}
public WorkflowBase withIsSchedulingEnabled(Boolean isSchedulingEnabled) {
WorkflowBase _x = _copy();
_x.changedFields = changedFields.add("isSchedulingEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.workflowBase");
_x.isSchedulingEnabled = isSchedulingEnabled;
return _x;
}
@Property(name="lastModifiedDateTime")
@JsonIgnore
public Optional getLastModifiedDateTime() {
return Optional.ofNullable(lastModifiedDateTime);
}
public WorkflowBase withLastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
WorkflowBase _x = _copy();
_x.changedFields = changedFields.add("lastModifiedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.workflowBase");
_x.lastModifiedDateTime = lastModifiedDateTime;
return _x;
}
public WorkflowBase withUnmappedField(String name, Object value) {
WorkflowBase _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="createdBy")
@JsonIgnore
public UserRequest getCreatedBy() {
return new UserRequest(contextPath.addSegment("createdBy"), RequestHelper.getValue(unmappedFields, "createdBy"));
}
@NavigationProperty(name="lastModifiedBy")
@JsonIgnore
public UserRequest getLastModifiedBy() {
return new UserRequest(contextPath.addSegment("lastModifiedBy"), RequestHelper.getValue(unmappedFields, "lastModifiedBy"));
}
@NavigationProperty(name="tasks")
@JsonIgnore
public TaskCollectionRequest getTasks() {
return new TaskCollectionRequest(
contextPath.addSegment("tasks"), Optional.ofNullable(tasks));
}
public WorkflowBase withTasks(List tasks) {
WorkflowBase _x = _copy();
_x.changedFields = changedFields.add("tasks");
_x.odataType = Util.nvl(odataType, "microsoft.graph.identityGovernance.workflowBase");
_x.tasks = tasks;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WorkflowBase patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
WorkflowBase _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WorkflowBase put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
WorkflowBase _x = _copy();
_x.changedFields = null;
return _x;
}
private WorkflowBase _copy() {
WorkflowBase _x = new WorkflowBase();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.category = category;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.executionConditions = executionConditions;
_x.isEnabled = isEnabled;
_x.isSchedulingEnabled = isSchedulingEnabled;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.tasks = tasks;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("WorkflowBase[");
b.append("category=");
b.append(this.category);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("executionConditions=");
b.append(this.executionConditions);
b.append(", ");
b.append("isEnabled=");
b.append(this.isEnabled);
b.append(", ");
b.append("isSchedulingEnabled=");
b.append(this.isSchedulingEnabled);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("tasks=");
b.append(this.tasks);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}