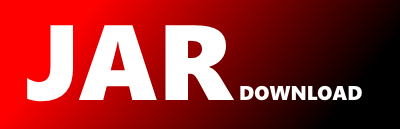
odata.msgraph.client.security.entity.ThreatIntelligence Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph Show documentation
Show all versions of odata-client-msgraph Show documentation
Java client for use with the Microsoft Graph v1.0 endpoint
package odata.msgraph.client.security.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.entity.Entity;
import odata.msgraph.client.security.entity.collection.request.ArticleCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.ArticleIndicatorCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.HostCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.HostComponentCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.HostCookieCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.HostPairCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.HostPortCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.HostSslCertificateCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.HostTrackerCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.IntelligenceProfileCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.IntelligenceProfileIndicatorCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.PassiveDnsRecordCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.SslCertificateCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.SubdomainCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.VulnerabilityCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.WhoisHistoryRecordCollectionRequest;
import odata.msgraph.client.security.entity.collection.request.WhoisRecordCollectionRequest;
@JsonPropertyOrder({
"@odata.type",
"articleIndicators",
"articles",
"hostComponents",
"hostCookies",
"hostPairs",
"hostPorts",
"hosts",
"hostSslCertificates",
"hostTrackers",
"intelligenceProfileIndicators",
"intelProfiles",
"passiveDnsRecords",
"sslCertificates",
"subdomains",
"vulnerabilities",
"whoisHistoryRecords",
"whoisRecords"})
@JsonInclude(Include.NON_NULL)
public class ThreatIntelligence extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.security.threatIntelligence";
}
@JsonProperty("articleIndicators")
protected List articleIndicators;
@JsonProperty("articles")
protected List articles;
@JsonProperty("hostComponents")
protected List hostComponents;
@JsonProperty("hostCookies")
protected List hostCookies;
@JsonProperty("hostPairs")
protected List hostPairs;
@JsonProperty("hostPorts")
protected List hostPorts;
@JsonProperty("hosts")
protected List hosts;
@JsonProperty("hostSslCertificates")
protected List hostSslCertificates;
@JsonProperty("hostTrackers")
protected List hostTrackers;
@JsonProperty("intelligenceProfileIndicators")
protected List intelligenceProfileIndicators;
@JsonProperty("intelProfiles")
protected List intelProfiles;
@JsonProperty("passiveDnsRecords")
protected List passiveDnsRecords;
@JsonProperty("sslCertificates")
protected List sslCertificates;
@JsonProperty("subdomains")
protected List subdomains;
@JsonProperty("vulnerabilities")
protected List vulnerabilities;
@JsonProperty("whoisHistoryRecords")
protected List whoisHistoryRecords;
@JsonProperty("whoisRecords")
protected List whoisRecords;
protected ThreatIntelligence() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderThreatIntelligence() {
return new Builder();
}
public static final class Builder {
private String id;
private List articleIndicators;
private List articles;
private List hostComponents;
private List hostCookies;
private List hostPairs;
private List hostPorts;
private List hosts;
private List hostSslCertificates;
private List hostTrackers;
private List intelligenceProfileIndicators;
private List intelProfiles;
private List passiveDnsRecords;
private List sslCertificates;
private List subdomains;
private List vulnerabilities;
private List whoisHistoryRecords;
private List whoisRecords;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder articleIndicators(List articleIndicators) {
this.articleIndicators = articleIndicators;
this.changedFields = changedFields.add("articleIndicators");
return this;
}
public Builder articleIndicators(ArticleIndicator... articleIndicators) {
return articleIndicators(Arrays.asList(articleIndicators));
}
public Builder articles(List articles) {
this.articles = articles;
this.changedFields = changedFields.add("articles");
return this;
}
public Builder articles(Article... articles) {
return articles(Arrays.asList(articles));
}
public Builder hostComponents(List hostComponents) {
this.hostComponents = hostComponents;
this.changedFields = changedFields.add("hostComponents");
return this;
}
public Builder hostComponents(HostComponent... hostComponents) {
return hostComponents(Arrays.asList(hostComponents));
}
public Builder hostCookies(List hostCookies) {
this.hostCookies = hostCookies;
this.changedFields = changedFields.add("hostCookies");
return this;
}
public Builder hostCookies(HostCookie... hostCookies) {
return hostCookies(Arrays.asList(hostCookies));
}
public Builder hostPairs(List hostPairs) {
this.hostPairs = hostPairs;
this.changedFields = changedFields.add("hostPairs");
return this;
}
public Builder hostPairs(HostPair... hostPairs) {
return hostPairs(Arrays.asList(hostPairs));
}
public Builder hostPorts(List hostPorts) {
this.hostPorts = hostPorts;
this.changedFields = changedFields.add("hostPorts");
return this;
}
public Builder hostPorts(HostPort... hostPorts) {
return hostPorts(Arrays.asList(hostPorts));
}
public Builder hosts(List hosts) {
this.hosts = hosts;
this.changedFields = changedFields.add("hosts");
return this;
}
public Builder hosts(Host... hosts) {
return hosts(Arrays.asList(hosts));
}
public Builder hostSslCertificates(List hostSslCertificates) {
this.hostSslCertificates = hostSslCertificates;
this.changedFields = changedFields.add("hostSslCertificates");
return this;
}
public Builder hostSslCertificates(HostSslCertificate... hostSslCertificates) {
return hostSslCertificates(Arrays.asList(hostSslCertificates));
}
public Builder hostTrackers(List hostTrackers) {
this.hostTrackers = hostTrackers;
this.changedFields = changedFields.add("hostTrackers");
return this;
}
public Builder hostTrackers(HostTracker... hostTrackers) {
return hostTrackers(Arrays.asList(hostTrackers));
}
public Builder intelligenceProfileIndicators(List intelligenceProfileIndicators) {
this.intelligenceProfileIndicators = intelligenceProfileIndicators;
this.changedFields = changedFields.add("intelligenceProfileIndicators");
return this;
}
public Builder intelligenceProfileIndicators(IntelligenceProfileIndicator... intelligenceProfileIndicators) {
return intelligenceProfileIndicators(Arrays.asList(intelligenceProfileIndicators));
}
public Builder intelProfiles(List intelProfiles) {
this.intelProfiles = intelProfiles;
this.changedFields = changedFields.add("intelProfiles");
return this;
}
public Builder intelProfiles(IntelligenceProfile... intelProfiles) {
return intelProfiles(Arrays.asList(intelProfiles));
}
public Builder passiveDnsRecords(List passiveDnsRecords) {
this.passiveDnsRecords = passiveDnsRecords;
this.changedFields = changedFields.add("passiveDnsRecords");
return this;
}
public Builder passiveDnsRecords(PassiveDnsRecord... passiveDnsRecords) {
return passiveDnsRecords(Arrays.asList(passiveDnsRecords));
}
public Builder sslCertificates(List sslCertificates) {
this.sslCertificates = sslCertificates;
this.changedFields = changedFields.add("sslCertificates");
return this;
}
public Builder sslCertificates(SslCertificate... sslCertificates) {
return sslCertificates(Arrays.asList(sslCertificates));
}
public Builder subdomains(List subdomains) {
this.subdomains = subdomains;
this.changedFields = changedFields.add("subdomains");
return this;
}
public Builder subdomains(Subdomain... subdomains) {
return subdomains(Arrays.asList(subdomains));
}
public Builder vulnerabilities(List vulnerabilities) {
this.vulnerabilities = vulnerabilities;
this.changedFields = changedFields.add("vulnerabilities");
return this;
}
public Builder vulnerabilities(Vulnerability... vulnerabilities) {
return vulnerabilities(Arrays.asList(vulnerabilities));
}
public Builder whoisHistoryRecords(List whoisHistoryRecords) {
this.whoisHistoryRecords = whoisHistoryRecords;
this.changedFields = changedFields.add("whoisHistoryRecords");
return this;
}
public Builder whoisHistoryRecords(WhoisHistoryRecord... whoisHistoryRecords) {
return whoisHistoryRecords(Arrays.asList(whoisHistoryRecords));
}
public Builder whoisRecords(List whoisRecords) {
this.whoisRecords = whoisRecords;
this.changedFields = changedFields.add("whoisRecords");
return this;
}
public Builder whoisRecords(WhoisRecord... whoisRecords) {
return whoisRecords(Arrays.asList(whoisRecords));
}
public ThreatIntelligence build() {
ThreatIntelligence _x = new ThreatIntelligence();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.security.threatIntelligence";
_x.id = id;
_x.articleIndicators = articleIndicators;
_x.articles = articles;
_x.hostComponents = hostComponents;
_x.hostCookies = hostCookies;
_x.hostPairs = hostPairs;
_x.hostPorts = hostPorts;
_x.hosts = hosts;
_x.hostSslCertificates = hostSslCertificates;
_x.hostTrackers = hostTrackers;
_x.intelligenceProfileIndicators = intelligenceProfileIndicators;
_x.intelProfiles = intelProfiles;
_x.passiveDnsRecords = passiveDnsRecords;
_x.sslCertificates = sslCertificates;
_x.subdomains = subdomains;
_x.vulnerabilities = vulnerabilities;
_x.whoisHistoryRecords = whoisHistoryRecords;
_x.whoisRecords = whoisRecords;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
public ThreatIntelligence withUnmappedField(String name, Object value) {
ThreatIntelligence _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="articleIndicators")
@JsonIgnore
public ArticleIndicatorCollectionRequest getArticleIndicators() {
return new ArticleIndicatorCollectionRequest(
contextPath.addSegment("articleIndicators"), Optional.ofNullable(articleIndicators));
}
@NavigationProperty(name="articles")
@JsonIgnore
public ArticleCollectionRequest getArticles() {
return new ArticleCollectionRequest(
contextPath.addSegment("articles"), Optional.ofNullable(articles));
}
@NavigationProperty(name="hostComponents")
@JsonIgnore
public HostComponentCollectionRequest getHostComponents() {
return new HostComponentCollectionRequest(
contextPath.addSegment("hostComponents"), Optional.ofNullable(hostComponents));
}
@NavigationProperty(name="hostCookies")
@JsonIgnore
public HostCookieCollectionRequest getHostCookies() {
return new HostCookieCollectionRequest(
contextPath.addSegment("hostCookies"), Optional.ofNullable(hostCookies));
}
@NavigationProperty(name="hostPairs")
@JsonIgnore
public HostPairCollectionRequest getHostPairs() {
return new HostPairCollectionRequest(
contextPath.addSegment("hostPairs"), Optional.ofNullable(hostPairs));
}
@NavigationProperty(name="hostPorts")
@JsonIgnore
public HostPortCollectionRequest getHostPorts() {
return new HostPortCollectionRequest(
contextPath.addSegment("hostPorts"), Optional.ofNullable(hostPorts));
}
@NavigationProperty(name="hosts")
@JsonIgnore
public HostCollectionRequest getHosts() {
return new HostCollectionRequest(
contextPath.addSegment("hosts"), Optional.ofNullable(hosts));
}
@NavigationProperty(name="hostSslCertificates")
@JsonIgnore
public HostSslCertificateCollectionRequest getHostSslCertificates() {
return new HostSslCertificateCollectionRequest(
contextPath.addSegment("hostSslCertificates"), Optional.ofNullable(hostSslCertificates));
}
@NavigationProperty(name="hostTrackers")
@JsonIgnore
public HostTrackerCollectionRequest getHostTrackers() {
return new HostTrackerCollectionRequest(
contextPath.addSegment("hostTrackers"), Optional.ofNullable(hostTrackers));
}
@NavigationProperty(name="intelligenceProfileIndicators")
@JsonIgnore
public IntelligenceProfileIndicatorCollectionRequest getIntelligenceProfileIndicators() {
return new IntelligenceProfileIndicatorCollectionRequest(
contextPath.addSegment("intelligenceProfileIndicators"), Optional.ofNullable(intelligenceProfileIndicators));
}
@NavigationProperty(name="intelProfiles")
@JsonIgnore
public IntelligenceProfileCollectionRequest getIntelProfiles() {
return new IntelligenceProfileCollectionRequest(
contextPath.addSegment("intelProfiles"), Optional.ofNullable(intelProfiles));
}
@NavigationProperty(name="passiveDnsRecords")
@JsonIgnore
public PassiveDnsRecordCollectionRequest getPassiveDnsRecords() {
return new PassiveDnsRecordCollectionRequest(
contextPath.addSegment("passiveDnsRecords"), Optional.ofNullable(passiveDnsRecords));
}
@NavigationProperty(name="sslCertificates")
@JsonIgnore
public SslCertificateCollectionRequest getSslCertificates() {
return new SslCertificateCollectionRequest(
contextPath.addSegment("sslCertificates"), Optional.ofNullable(sslCertificates));
}
@NavigationProperty(name="subdomains")
@JsonIgnore
public SubdomainCollectionRequest getSubdomains() {
return new SubdomainCollectionRequest(
contextPath.addSegment("subdomains"), Optional.ofNullable(subdomains));
}
@NavigationProperty(name="vulnerabilities")
@JsonIgnore
public VulnerabilityCollectionRequest getVulnerabilities() {
return new VulnerabilityCollectionRequest(
contextPath.addSegment("vulnerabilities"), Optional.ofNullable(vulnerabilities));
}
@NavigationProperty(name="whoisHistoryRecords")
@JsonIgnore
public WhoisHistoryRecordCollectionRequest getWhoisHistoryRecords() {
return new WhoisHistoryRecordCollectionRequest(
contextPath.addSegment("whoisHistoryRecords"), Optional.ofNullable(whoisHistoryRecords));
}
@NavigationProperty(name="whoisRecords")
@JsonIgnore
public WhoisRecordCollectionRequest getWhoisRecords() {
return new WhoisRecordCollectionRequest(
contextPath.addSegment("whoisRecords"), Optional.ofNullable(whoisRecords));
}
public ThreatIntelligence withArticleIndicators(List articleIndicators) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("articleIndicators");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.articleIndicators = articleIndicators;
return _x;
}
public ThreatIntelligence withArticles(List articles) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("articles");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.articles = articles;
return _x;
}
public ThreatIntelligence withHostComponents(List hostComponents) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("hostComponents");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.hostComponents = hostComponents;
return _x;
}
public ThreatIntelligence withHostCookies(List hostCookies) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("hostCookies");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.hostCookies = hostCookies;
return _x;
}
public ThreatIntelligence withHostPairs(List hostPairs) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("hostPairs");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.hostPairs = hostPairs;
return _x;
}
public ThreatIntelligence withHostPorts(List hostPorts) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("hostPorts");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.hostPorts = hostPorts;
return _x;
}
public ThreatIntelligence withHosts(List hosts) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("hosts");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.hosts = hosts;
return _x;
}
public ThreatIntelligence withHostSslCertificates(List hostSslCertificates) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("hostSslCertificates");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.hostSslCertificates = hostSslCertificates;
return _x;
}
public ThreatIntelligence withHostTrackers(List hostTrackers) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("hostTrackers");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.hostTrackers = hostTrackers;
return _x;
}
public ThreatIntelligence withIntelligenceProfileIndicators(List intelligenceProfileIndicators) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("intelligenceProfileIndicators");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.intelligenceProfileIndicators = intelligenceProfileIndicators;
return _x;
}
public ThreatIntelligence withIntelProfiles(List intelProfiles) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("intelProfiles");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.intelProfiles = intelProfiles;
return _x;
}
public ThreatIntelligence withPassiveDnsRecords(List passiveDnsRecords) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("passiveDnsRecords");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.passiveDnsRecords = passiveDnsRecords;
return _x;
}
public ThreatIntelligence withSslCertificates(List sslCertificates) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("sslCertificates");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.sslCertificates = sslCertificates;
return _x;
}
public ThreatIntelligence withSubdomains(List subdomains) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("subdomains");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.subdomains = subdomains;
return _x;
}
public ThreatIntelligence withVulnerabilities(List vulnerabilities) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("vulnerabilities");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.vulnerabilities = vulnerabilities;
return _x;
}
public ThreatIntelligence withWhoisHistoryRecords(List whoisHistoryRecords) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("whoisHistoryRecords");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.whoisHistoryRecords = whoisHistoryRecords;
return _x;
}
public ThreatIntelligence withWhoisRecords(List whoisRecords) {
ThreatIntelligence _x = _copy();
_x.changedFields = changedFields.add("whoisRecords");
_x.odataType = Util.nvl(odataType, "microsoft.graph.security.threatIntelligence");
_x.whoisRecords = whoisRecords;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ThreatIntelligence patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
ThreatIntelligence _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ThreatIntelligence put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
ThreatIntelligence _x = _copy();
_x.changedFields = null;
return _x;
}
private ThreatIntelligence _copy() {
ThreatIntelligence _x = new ThreatIntelligence();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.articleIndicators = articleIndicators;
_x.articles = articles;
_x.hostComponents = hostComponents;
_x.hostCookies = hostCookies;
_x.hostPairs = hostPairs;
_x.hostPorts = hostPorts;
_x.hosts = hosts;
_x.hostSslCertificates = hostSslCertificates;
_x.hostTrackers = hostTrackers;
_x.intelligenceProfileIndicators = intelligenceProfileIndicators;
_x.intelProfiles = intelProfiles;
_x.passiveDnsRecords = passiveDnsRecords;
_x.sslCertificates = sslCertificates;
_x.subdomains = subdomains;
_x.vulnerabilities = vulnerabilities;
_x.whoisHistoryRecords = whoisHistoryRecords;
_x.whoisRecords = whoisRecords;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ThreatIntelligence[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("articleIndicators=");
b.append(this.articleIndicators);
b.append(", ");
b.append("articles=");
b.append(this.articles);
b.append(", ");
b.append("hostComponents=");
b.append(this.hostComponents);
b.append(", ");
b.append("hostCookies=");
b.append(this.hostCookies);
b.append(", ");
b.append("hostPairs=");
b.append(this.hostPairs);
b.append(", ");
b.append("hostPorts=");
b.append(this.hostPorts);
b.append(", ");
b.append("hosts=");
b.append(this.hosts);
b.append(", ");
b.append("hostSslCertificates=");
b.append(this.hostSslCertificates);
b.append(", ");
b.append("hostTrackers=");
b.append(this.hostTrackers);
b.append(", ");
b.append("intelligenceProfileIndicators=");
b.append(this.intelligenceProfileIndicators);
b.append(", ");
b.append("intelProfiles=");
b.append(this.intelProfiles);
b.append(", ");
b.append("passiveDnsRecords=");
b.append(this.passiveDnsRecords);
b.append(", ");
b.append("sslCertificates=");
b.append(this.sslCertificates);
b.append(", ");
b.append("subdomains=");
b.append(this.subdomains);
b.append(", ");
b.append("vulnerabilities=");
b.append(this.vulnerabilities);
b.append(", ");
b.append("whoisHistoryRecords=");
b.append(this.whoisHistoryRecords);
b.append(", ");
b.append("whoisRecords=");
b.append(this.whoisRecords);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy