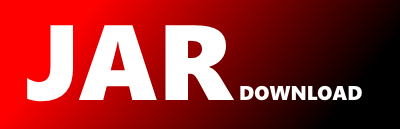
thomsonreuters.dss.api.extractions.reporttemplates.complex.ContentFieldNumberFormat Maven / Gradle / Ivy
The newest version!
package thomsonreuters.dss.api.extractions.reporttemplates.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Optional;
import thomsonreuters.dss.api.extractions.reporttemplates.complex.ContentFieldFormat;
import thomsonreuters.dss.api.extractions.reporttemplates.enums.ContentFieldDecimalSeparator;
import thomsonreuters.dss.api.extractions.reporttemplates.enums.ContentFieldNegativeSignPosition;
import thomsonreuters.dss.api.extractions.reporttemplates.enums.ContentFieldThousandsSeparator;
@JsonInclude(Include.NON_NULL)
@JsonPropertyOrder({
"@odata.type",
"DecimalPlaces",
"DecimalSeparator",
"IntegerPlaces",
"UseLeadingZero",
"NegativeSignPosition",
"ThousandSeparator",
"UseThousandSeparator",
"UseTrailingZero"})
public class ContentFieldNumberFormat extends ContentFieldFormat implements ODataType {
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("DecimalPlaces")
protected Integer decimalPlaces;
@JsonProperty("DecimalSeparator")
protected ContentFieldDecimalSeparator decimalSeparator;
@JsonProperty("IntegerPlaces")
protected Integer integerPlaces;
@JsonProperty("UseLeadingZero")
protected Boolean useLeadingZero;
@JsonProperty("NegativeSignPosition")
protected ContentFieldNegativeSignPosition negativeSignPosition;
@JsonProperty("ThousandSeparator")
protected ContentFieldThousandsSeparator thousandSeparator;
@JsonProperty("UseThousandSeparator")
protected Boolean useThousandSeparator;
@JsonProperty("UseTrailingZero")
protected Boolean useTrailingZero;
protected ContentFieldNumberFormat() {
super();
}
@Override
public String odataTypeName() {
return "ThomsonReuters.Dss.Api.Extractions.ReportTemplates.ContentFieldNumberFormat";
}
@Property(name="DecimalPlaces")
@JsonIgnore
public Optional getDecimalPlaces() {
return Optional.ofNullable(decimalPlaces);
}
public ContentFieldNumberFormat withDecimalPlaces(Integer decimalPlaces) {
ContentFieldNumberFormat _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.ReportTemplates.ContentFieldNumberFormat");
_x.decimalPlaces = decimalPlaces;
return _x;
}
@Property(name="DecimalSeparator")
@JsonIgnore
public Optional getDecimalSeparator() {
return Optional.ofNullable(decimalSeparator);
}
public ContentFieldNumberFormat withDecimalSeparator(ContentFieldDecimalSeparator decimalSeparator) {
ContentFieldNumberFormat _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.ReportTemplates.ContentFieldNumberFormat");
_x.decimalSeparator = decimalSeparator;
return _x;
}
@Property(name="IntegerPlaces")
@JsonIgnore
public Optional getIntegerPlaces() {
return Optional.ofNullable(integerPlaces);
}
public ContentFieldNumberFormat withIntegerPlaces(Integer integerPlaces) {
ContentFieldNumberFormat _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.ReportTemplates.ContentFieldNumberFormat");
_x.integerPlaces = integerPlaces;
return _x;
}
@Property(name="UseLeadingZero")
@JsonIgnore
public Optional getUseLeadingZero() {
return Optional.ofNullable(useLeadingZero);
}
public ContentFieldNumberFormat withUseLeadingZero(Boolean useLeadingZero) {
ContentFieldNumberFormat _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.ReportTemplates.ContentFieldNumberFormat");
_x.useLeadingZero = useLeadingZero;
return _x;
}
@Property(name="NegativeSignPosition")
@JsonIgnore
public Optional getNegativeSignPosition() {
return Optional.ofNullable(negativeSignPosition);
}
public ContentFieldNumberFormat withNegativeSignPosition(ContentFieldNegativeSignPosition negativeSignPosition) {
ContentFieldNumberFormat _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.ReportTemplates.ContentFieldNumberFormat");
_x.negativeSignPosition = negativeSignPosition;
return _x;
}
@Property(name="ThousandSeparator")
@JsonIgnore
public Optional getThousandSeparator() {
return Optional.ofNullable(thousandSeparator);
}
public ContentFieldNumberFormat withThousandSeparator(ContentFieldThousandsSeparator thousandSeparator) {
ContentFieldNumberFormat _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.ReportTemplates.ContentFieldNumberFormat");
_x.thousandSeparator = thousandSeparator;
return _x;
}
@Property(name="UseThousandSeparator")
@JsonIgnore
public Optional getUseThousandSeparator() {
return Optional.ofNullable(useThousandSeparator);
}
public ContentFieldNumberFormat withUseThousandSeparator(Boolean useThousandSeparator) {
ContentFieldNumberFormat _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.ReportTemplates.ContentFieldNumberFormat");
_x.useThousandSeparator = useThousandSeparator;
return _x;
}
@Property(name="UseTrailingZero")
@JsonIgnore
public Optional getUseTrailingZero() {
return Optional.ofNullable(useTrailingZero);
}
public ContentFieldNumberFormat withUseTrailingZero(Boolean useTrailingZero) {
ContentFieldNumberFormat _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.ReportTemplates.ContentFieldNumberFormat");
_x.useTrailingZero = useTrailingZero;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderContentFieldNumberFormat() {
return new Builder();
}
public static final class Builder {
private Integer decimalPlaces;
private ContentFieldDecimalSeparator decimalSeparator;
private Integer integerPlaces;
private Boolean useLeadingZero;
private ContentFieldNegativeSignPosition negativeSignPosition;
private ContentFieldThousandsSeparator thousandSeparator;
private Boolean useThousandSeparator;
private Boolean useTrailingZero;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder decimalPlaces(Integer decimalPlaces) {
this.decimalPlaces = decimalPlaces;
this.changedFields = changedFields.add("DecimalPlaces");
return this;
}
public Builder decimalSeparator(ContentFieldDecimalSeparator decimalSeparator) {
this.decimalSeparator = decimalSeparator;
this.changedFields = changedFields.add("DecimalSeparator");
return this;
}
public Builder integerPlaces(Integer integerPlaces) {
this.integerPlaces = integerPlaces;
this.changedFields = changedFields.add("IntegerPlaces");
return this;
}
public Builder useLeadingZero(Boolean useLeadingZero) {
this.useLeadingZero = useLeadingZero;
this.changedFields = changedFields.add("UseLeadingZero");
return this;
}
public Builder negativeSignPosition(ContentFieldNegativeSignPosition negativeSignPosition) {
this.negativeSignPosition = negativeSignPosition;
this.changedFields = changedFields.add("NegativeSignPosition");
return this;
}
public Builder thousandSeparator(ContentFieldThousandsSeparator thousandSeparator) {
this.thousandSeparator = thousandSeparator;
this.changedFields = changedFields.add("ThousandSeparator");
return this;
}
public Builder useThousandSeparator(Boolean useThousandSeparator) {
this.useThousandSeparator = useThousandSeparator;
this.changedFields = changedFields.add("UseThousandSeparator");
return this;
}
public Builder useTrailingZero(Boolean useTrailingZero) {
this.useTrailingZero = useTrailingZero;
this.changedFields = changedFields.add("UseTrailingZero");
return this;
}
public ContentFieldNumberFormat build() {
ContentFieldNumberFormat _x = new ContentFieldNumberFormat();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "ThomsonReuters.Dss.Api.Extractions.ReportTemplates.ContentFieldNumberFormat";
_x.decimalPlaces = decimalPlaces;
_x.decimalSeparator = decimalSeparator;
_x.integerPlaces = integerPlaces;
_x.useLeadingZero = useLeadingZero;
_x.negativeSignPosition = negativeSignPosition;
_x.thousandSeparator = thousandSeparator;
_x.useThousandSeparator = useThousandSeparator;
_x.useTrailingZero = useTrailingZero;
return _x;
}
}
private ContentFieldNumberFormat _copy() {
ContentFieldNumberFormat _x = new ContentFieldNumberFormat();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.decimalPlaces = decimalPlaces;
_x.decimalSeparator = decimalSeparator;
_x.integerPlaces = integerPlaces;
_x.useLeadingZero = useLeadingZero;
_x.negativeSignPosition = negativeSignPosition;
_x.thousandSeparator = thousandSeparator;
_x.useThousandSeparator = useThousandSeparator;
_x.useTrailingZero = useTrailingZero;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ContentFieldNumberFormat[");
b.append("DecimalPlaces=");
b.append(this.decimalPlaces);
b.append(", ");
b.append("DecimalSeparator=");
b.append(this.decimalSeparator);
b.append(", ");
b.append("IntegerPlaces=");
b.append(this.integerPlaces);
b.append(", ");
b.append("UseLeadingZero=");
b.append(this.useLeadingZero);
b.append(", ");
b.append("NegativeSignPosition=");
b.append(this.negativeSignPosition);
b.append(", ");
b.append("ThousandSeparator=");
b.append(this.thousandSeparator);
b.append(", ");
b.append("UseThousandSeparator=");
b.append(this.useThousandSeparator);
b.append(", ");
b.append("UseTrailingZero=");
b.append(this.useTrailingZero);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy