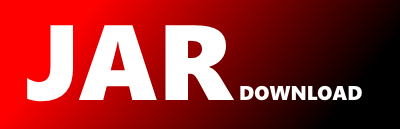
thomsonreuters.dss.api.extractions.subjectlists.complex.InstrumentListValidationOptions Maven / Gradle / Ivy
package thomsonreuters.dss.api.extractions.subjectlists.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Optional;
import thomsonreuters.dss.api.extractions.subjectlists.complex.InstrumentValidationOptions;
@JsonInclude(Include.NON_NULL)
@JsonPropertyOrder({
"@odata.type",
"AllowDuplicateInstruments"})
public class InstrumentListValidationOptions extends InstrumentValidationOptions implements ODataType {
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("AllowDuplicateInstruments")
protected Boolean allowDuplicateInstruments;
protected InstrumentListValidationOptions() {
super();
}
@Override
public String odataTypeName() {
return "ThomsonReuters.Dss.Api.Extractions.SubjectLists.InstrumentListValidationOptions";
}
@Property(name="AllowDuplicateInstruments")
@JsonIgnore
public Optional getAllowDuplicateInstruments() {
return Optional.ofNullable(allowDuplicateInstruments);
}
public InstrumentListValidationOptions withAllowDuplicateInstruments(Boolean allowDuplicateInstruments) {
InstrumentListValidationOptions _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.SubjectLists.InstrumentListValidationOptions");
_x.allowDuplicateInstruments = allowDuplicateInstruments;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderInstrumentListValidationOptions() {
return new Builder();
}
public static final class Builder {
private Boolean allowOpenAccessInstruments;
private Boolean allowHistoricalInstruments;
private Boolean allowLimitedTermInstruments;
private Boolean allowInactiveInstruments;
private Boolean allowUnsupportedInstruments;
private Boolean excludeFinrAsPricingSourceForBonds;
private Boolean useExchangeCodeInsteadOfLipper;
private Boolean useUsQuoteInsteadOfCanadian;
private Boolean useConsolidatedQuoteSourceForUsa;
private Boolean useConsolidatedQuoteSourceForCanada;
private Boolean useDebtOverEquity;
private Boolean useOtcPqSource;
private Boolean allowSubclassImport;
private Boolean allowDuplicateInstruments;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder allowOpenAccessInstruments(Boolean allowOpenAccessInstruments) {
this.allowOpenAccessInstruments = allowOpenAccessInstruments;
this.changedFields = changedFields.add("AllowOpenAccessInstruments");
return this;
}
public Builder allowHistoricalInstruments(Boolean allowHistoricalInstruments) {
this.allowHistoricalInstruments = allowHistoricalInstruments;
this.changedFields = changedFields.add("AllowHistoricalInstruments");
return this;
}
public Builder allowLimitedTermInstruments(Boolean allowLimitedTermInstruments) {
this.allowLimitedTermInstruments = allowLimitedTermInstruments;
this.changedFields = changedFields.add("AllowLimitedTermInstruments");
return this;
}
public Builder allowInactiveInstruments(Boolean allowInactiveInstruments) {
this.allowInactiveInstruments = allowInactiveInstruments;
this.changedFields = changedFields.add("AllowInactiveInstruments");
return this;
}
public Builder allowUnsupportedInstruments(Boolean allowUnsupportedInstruments) {
this.allowUnsupportedInstruments = allowUnsupportedInstruments;
this.changedFields = changedFields.add("AllowUnsupportedInstruments");
return this;
}
public Builder excludeFinrAsPricingSourceForBonds(Boolean excludeFinrAsPricingSourceForBonds) {
this.excludeFinrAsPricingSourceForBonds = excludeFinrAsPricingSourceForBonds;
this.changedFields = changedFields.add("ExcludeFinrAsPricingSourceForBonds");
return this;
}
public Builder useExchangeCodeInsteadOfLipper(Boolean useExchangeCodeInsteadOfLipper) {
this.useExchangeCodeInsteadOfLipper = useExchangeCodeInsteadOfLipper;
this.changedFields = changedFields.add("UseExchangeCodeInsteadOfLipper");
return this;
}
public Builder useUsQuoteInsteadOfCanadian(Boolean useUsQuoteInsteadOfCanadian) {
this.useUsQuoteInsteadOfCanadian = useUsQuoteInsteadOfCanadian;
this.changedFields = changedFields.add("UseUsQuoteInsteadOfCanadian");
return this;
}
public Builder useConsolidatedQuoteSourceForUsa(Boolean useConsolidatedQuoteSourceForUsa) {
this.useConsolidatedQuoteSourceForUsa = useConsolidatedQuoteSourceForUsa;
this.changedFields = changedFields.add("UseConsolidatedQuoteSourceForUsa");
return this;
}
public Builder useConsolidatedQuoteSourceForCanada(Boolean useConsolidatedQuoteSourceForCanada) {
this.useConsolidatedQuoteSourceForCanada = useConsolidatedQuoteSourceForCanada;
this.changedFields = changedFields.add("UseConsolidatedQuoteSourceForCanada");
return this;
}
public Builder useDebtOverEquity(Boolean useDebtOverEquity) {
this.useDebtOverEquity = useDebtOverEquity;
this.changedFields = changedFields.add("UseDebtOverEquity");
return this;
}
public Builder useOtcPqSource(Boolean useOtcPqSource) {
this.useOtcPqSource = useOtcPqSource;
this.changedFields = changedFields.add("UseOtcPqSource");
return this;
}
public Builder allowSubclassImport(Boolean allowSubclassImport) {
this.allowSubclassImport = allowSubclassImport;
this.changedFields = changedFields.add("AllowSubclassImport");
return this;
}
public Builder allowDuplicateInstruments(Boolean allowDuplicateInstruments) {
this.allowDuplicateInstruments = allowDuplicateInstruments;
this.changedFields = changedFields.add("AllowDuplicateInstruments");
return this;
}
public InstrumentListValidationOptions build() {
InstrumentListValidationOptions _x = new InstrumentListValidationOptions();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "ThomsonReuters.Dss.Api.Extractions.SubjectLists.InstrumentListValidationOptions";
_x.allowOpenAccessInstruments = allowOpenAccessInstruments;
_x.allowHistoricalInstruments = allowHistoricalInstruments;
_x.allowLimitedTermInstruments = allowLimitedTermInstruments;
_x.allowInactiveInstruments = allowInactiveInstruments;
_x.allowUnsupportedInstruments = allowUnsupportedInstruments;
_x.excludeFinrAsPricingSourceForBonds = excludeFinrAsPricingSourceForBonds;
_x.useExchangeCodeInsteadOfLipper = useExchangeCodeInsteadOfLipper;
_x.useUsQuoteInsteadOfCanadian = useUsQuoteInsteadOfCanadian;
_x.useConsolidatedQuoteSourceForUsa = useConsolidatedQuoteSourceForUsa;
_x.useConsolidatedQuoteSourceForCanada = useConsolidatedQuoteSourceForCanada;
_x.useDebtOverEquity = useDebtOverEquity;
_x.useOtcPqSource = useOtcPqSource;
_x.allowSubclassImport = allowSubclassImport;
_x.allowDuplicateInstruments = allowDuplicateInstruments;
return _x;
}
}
private InstrumentListValidationOptions _copy() {
InstrumentListValidationOptions _x = new InstrumentListValidationOptions();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.allowOpenAccessInstruments = allowOpenAccessInstruments;
_x.allowHistoricalInstruments = allowHistoricalInstruments;
_x.allowLimitedTermInstruments = allowLimitedTermInstruments;
_x.allowInactiveInstruments = allowInactiveInstruments;
_x.allowUnsupportedInstruments = allowUnsupportedInstruments;
_x.excludeFinrAsPricingSourceForBonds = excludeFinrAsPricingSourceForBonds;
_x.useExchangeCodeInsteadOfLipper = useExchangeCodeInsteadOfLipper;
_x.useUsQuoteInsteadOfCanadian = useUsQuoteInsteadOfCanadian;
_x.useConsolidatedQuoteSourceForUsa = useConsolidatedQuoteSourceForUsa;
_x.useConsolidatedQuoteSourceForCanada = useConsolidatedQuoteSourceForCanada;
_x.useDebtOverEquity = useDebtOverEquity;
_x.useOtcPqSource = useOtcPqSource;
_x.allowSubclassImport = allowSubclassImport;
_x.allowDuplicateInstruments = allowDuplicateInstruments;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("InstrumentListValidationOptions[");
b.append("AllowOpenAccessInstruments=");
b.append(this.allowOpenAccessInstruments);
b.append(", ");
b.append("AllowHistoricalInstruments=");
b.append(this.allowHistoricalInstruments);
b.append(", ");
b.append("AllowLimitedTermInstruments=");
b.append(this.allowLimitedTermInstruments);
b.append(", ");
b.append("AllowInactiveInstruments=");
b.append(this.allowInactiveInstruments);
b.append(", ");
b.append("AllowUnsupportedInstruments=");
b.append(this.allowUnsupportedInstruments);
b.append(", ");
b.append("ExcludeFinrAsPricingSourceForBonds=");
b.append(this.excludeFinrAsPricingSourceForBonds);
b.append(", ");
b.append("UseExchangeCodeInsteadOfLipper=");
b.append(this.useExchangeCodeInsteadOfLipper);
b.append(", ");
b.append("UseUsQuoteInsteadOfCanadian=");
b.append(this.useUsQuoteInsteadOfCanadian);
b.append(", ");
b.append("UseConsolidatedQuoteSourceForUsa=");
b.append(this.useConsolidatedQuoteSourceForUsa);
b.append(", ");
b.append("UseConsolidatedQuoteSourceForCanada=");
b.append(this.useConsolidatedQuoteSourceForCanada);
b.append(", ");
b.append("UseDebtOverEquity=");
b.append(this.useDebtOverEquity);
b.append(", ");
b.append("UseOtcPqSource=");
b.append(this.useOtcPqSource);
b.append(", ");
b.append("AllowSubclassImport=");
b.append(this.allowSubclassImport);
b.append(", ");
b.append("AllowDuplicateInstruments=");
b.append(this.allowDuplicateInstruments);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy