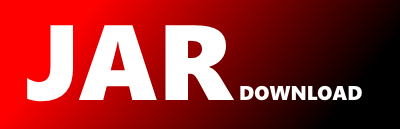
thomsonreuters.dss.api.extractions.subjectlists.entity.CriteriaList Maven / Gradle / Ivy
The newest version!
package thomsonreuters.dss.api.extractions.subjectlists.entity;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import thomsonreuters.dss.api.extractions.subjectlists.complex.CriteriaListFilter;
import thomsonreuters.dss.api.extractions.subjectlists.entity.SubjectList;
import thomsonreuters.dss.api.extractions.subjectlists.enums.CriteriaListType;
import thomsonreuters.dss.api.extractions.subjectlists.schema.SchemaInfo;
@JsonInclude(Include.NON_NULL)
@JsonPropertyOrder({
"@odata.type",
"Type",
"Created",
"Modified",
"Filters"})
public class CriteriaList extends SubjectList implements ODataEntityType {
@Override
public String odataTypeName() {
return "ThomsonReuters.Dss.Api.Extractions.SubjectLists.CriteriaList";
}
@JsonProperty("Type")
protected CriteriaListType type;
@JsonProperty("Created")
protected OffsetDateTime created;
@JsonProperty("Modified")
protected OffsetDateTime modified;
@JsonProperty("Filters")
protected List filters;
@JsonProperty("Filters@nextLink")
protected String filtersNextLink;
protected CriteriaList() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderCriteriaList() {
return new Builder();
}
public static final class Builder {
private String listId;
private String name;
private CriteriaListType type;
private OffsetDateTime created;
private OffsetDateTime modified;
private List filters;
private String filtersNextLink;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder listId(String listId) {
this.listId = listId;
this.changedFields = changedFields.add("ListId");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("Name");
return this;
}
public Builder type(CriteriaListType type) {
this.type = type;
this.changedFields = changedFields.add("Type");
return this;
}
public Builder created(OffsetDateTime created) {
this.created = created;
this.changedFields = changedFields.add("Created");
return this;
}
public Builder modified(OffsetDateTime modified) {
this.modified = modified;
this.changedFields = changedFields.add("Modified");
return this;
}
public Builder filters(List filters) {
this.filters = filters;
this.changedFields = changedFields.add("Filters");
return this;
}
public Builder filtersNextLink(String filtersNextLink) {
this.filtersNextLink = filtersNextLink;
this.changedFields = changedFields.add("Filters");
return this;
}
public CriteriaList build() {
CriteriaList _x = new CriteriaList();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "ThomsonReuters.Dss.Api.Extractions.SubjectLists.CriteriaList";
_x.listId = listId;
_x.name = name;
_x.type = type;
_x.created = created;
_x.modified = modified;
_x.filters = filters;
_x.filtersNextLink = filtersNextLink;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && listId != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(listId.toString()));
}
}
@Property(name="Type")
@JsonIgnore
public Optional getType() {
return Optional.ofNullable(type);
}
public CriteriaList withType(CriteriaListType type) {
CriteriaList _x = _copy();
_x.changedFields = changedFields.add("Type");
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.SubjectLists.CriteriaList");
_x.type = type;
return _x;
}
@Property(name="Created")
@JsonIgnore
public Optional getCreated() {
return Optional.ofNullable(created);
}
public CriteriaList withCreated(OffsetDateTime created) {
CriteriaList _x = _copy();
_x.changedFields = changedFields.add("Created");
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.SubjectLists.CriteriaList");
_x.created = created;
return _x;
}
@Property(name="Modified")
@JsonIgnore
public Optional getModified() {
return Optional.ofNullable(modified);
}
public CriteriaList withModified(OffsetDateTime modified) {
CriteriaList _x = _copy();
_x.changedFields = changedFields.add("Modified");
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Extractions.SubjectLists.CriteriaList");
_x.modified = modified;
return _x;
}
@Property(name="Filters")
@JsonIgnore
public CollectionPage getFilters() {
return new CollectionPage(contextPath, CriteriaListFilter.class, filters, Optional.ofNullable(filtersNextLink), SchemaInfo.INSTANCE, Collections.emptyList());
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public CriteriaList patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
CriteriaList _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public CriteriaList put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
CriteriaList _x = _copy();
_x.changedFields = null;
return _x;
}
private CriteriaList _copy() {
CriteriaList _x = new CriteriaList();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.listId = listId;
_x.name = name;
_x.type = type;
_x.created = created;
_x.modified = modified;
_x.filters = filters;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("CriteriaList[");
b.append("ListId=");
b.append(this.listId);
b.append(", ");
b.append("Name=");
b.append(this.name);
b.append(", ");
b.append("Type=");
b.append(this.type);
b.append(", ");
b.append("Created=");
b.append(this.created);
b.append(", ");
b.append("Modified=");
b.append(this.modified);
b.append(", ");
b.append("Filters=");
b.append(this.filters);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy