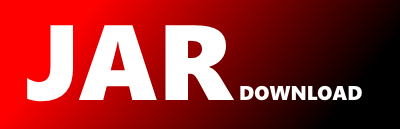
thomsonreuters.dss.api.search.complex.HistoricalCriteriaSearchRequest Maven / Gradle / Ivy
The newest version!
package thomsonreuters.dss.api.search.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.EdmSchemaInfo;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import thomsonreuters.dss.api.search.complex.DateTimeRange;
import thomsonreuters.dss.api.search.complex.DecimalValueRange;
import thomsonreuters.dss.api.search.enums.HistoricalResultsBy;
@JsonInclude(Include.NON_NULL)
@JsonPropertyOrder({
"@odata.type",
"RicPattern",
"DescriptionPattern",
"BondTypeCodes",
"ContributorIds",
"CountryCodes",
"CurrencyCodes",
"DomainCodes",
"ExchangeCodes",
"FutureMonthCodes",
"InstrumentTypeCodes",
"OptionMonthCodes",
"OptionTypeCodes",
"CouponRate",
"StrikePrice",
"ExpiryDate",
"MaturityDate",
"Range",
"ResultsBy"})
public class HistoricalCriteriaSearchRequest implements ODataType {
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("RicPattern")
protected String ricPattern;
@JsonProperty("DescriptionPattern")
protected String descriptionPattern;
@JsonProperty("BondTypeCodes")
protected List bondTypeCodes;
@JsonProperty("BondTypeCodes@nextLink")
protected String bondTypeCodesNextLink;
@JsonProperty("ContributorIds")
protected List contributorIds;
@JsonProperty("ContributorIds@nextLink")
protected String contributorIdsNextLink;
@JsonProperty("CountryCodes")
protected List countryCodes;
@JsonProperty("CountryCodes@nextLink")
protected String countryCodesNextLink;
@JsonProperty("CurrencyCodes")
protected List currencyCodes;
@JsonProperty("CurrencyCodes@nextLink")
protected String currencyCodesNextLink;
@JsonProperty("DomainCodes")
protected List domainCodes;
@JsonProperty("DomainCodes@nextLink")
protected String domainCodesNextLink;
@JsonProperty("ExchangeCodes")
protected List exchangeCodes;
@JsonProperty("ExchangeCodes@nextLink")
protected String exchangeCodesNextLink;
@JsonProperty("FutureMonthCodes")
protected List futureMonthCodes;
@JsonProperty("FutureMonthCodes@nextLink")
protected String futureMonthCodesNextLink;
@JsonProperty("InstrumentTypeCodes")
protected List instrumentTypeCodes;
@JsonProperty("InstrumentTypeCodes@nextLink")
protected String instrumentTypeCodesNextLink;
@JsonProperty("OptionMonthCodes")
protected List optionMonthCodes;
@JsonProperty("OptionMonthCodes@nextLink")
protected String optionMonthCodesNextLink;
@JsonProperty("OptionTypeCodes")
protected List optionTypeCodes;
@JsonProperty("OptionTypeCodes@nextLink")
protected String optionTypeCodesNextLink;
@JsonProperty("CouponRate")
protected DecimalValueRange couponRate;
@JsonProperty("StrikePrice")
protected DecimalValueRange strikePrice;
@JsonProperty("ExpiryDate")
protected DateTimeRange expiryDate;
@JsonProperty("MaturityDate")
protected DateTimeRange maturityDate;
@JsonProperty("Range")
protected DateTimeRange range;
@JsonProperty("ResultsBy")
protected HistoricalResultsBy resultsBy;
protected HistoricalCriteriaSearchRequest() {
}
@Override
public String odataTypeName() {
return "ThomsonReuters.Dss.Api.Search.HistoricalCriteriaSearchRequest";
}
@Property(name="RicPattern")
@JsonIgnore
public Optional getRicPattern() {
return Optional.ofNullable(ricPattern);
}
public HistoricalCriteriaSearchRequest withRicPattern(String ricPattern) {
HistoricalCriteriaSearchRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.HistoricalCriteriaSearchRequest");
_x.ricPattern = ricPattern;
return _x;
}
@Property(name="DescriptionPattern")
@JsonIgnore
public Optional getDescriptionPattern() {
return Optional.ofNullable(descriptionPattern);
}
public HistoricalCriteriaSearchRequest withDescriptionPattern(String descriptionPattern) {
HistoricalCriteriaSearchRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.HistoricalCriteriaSearchRequest");
_x.descriptionPattern = descriptionPattern;
return _x;
}
@Property(name="BondTypeCodes")
@JsonIgnore
public CollectionPage getBondTypeCodes() {
return new CollectionPage(contextPath, String.class, bondTypeCodes, Optional.ofNullable(bondTypeCodesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList());
}
@Property(name="ContributorIds")
@JsonIgnore
public CollectionPage getContributorIds() {
return new CollectionPage(contextPath, String.class, contributorIds, Optional.ofNullable(contributorIdsNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList());
}
@Property(name="CountryCodes")
@JsonIgnore
public CollectionPage getCountryCodes() {
return new CollectionPage(contextPath, String.class, countryCodes, Optional.ofNullable(countryCodesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList());
}
@Property(name="CurrencyCodes")
@JsonIgnore
public CollectionPage getCurrencyCodes() {
return new CollectionPage(contextPath, String.class, currencyCodes, Optional.ofNullable(currencyCodesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList());
}
@Property(name="DomainCodes")
@JsonIgnore
public CollectionPage getDomainCodes() {
return new CollectionPage(contextPath, String.class, domainCodes, Optional.ofNullable(domainCodesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList());
}
@Property(name="ExchangeCodes")
@JsonIgnore
public CollectionPage getExchangeCodes() {
return new CollectionPage(contextPath, String.class, exchangeCodes, Optional.ofNullable(exchangeCodesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList());
}
@Property(name="FutureMonthCodes")
@JsonIgnore
public CollectionPage getFutureMonthCodes() {
return new CollectionPage(contextPath, String.class, futureMonthCodes, Optional.ofNullable(futureMonthCodesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList());
}
@Property(name="InstrumentTypeCodes")
@JsonIgnore
public CollectionPage getInstrumentTypeCodes() {
return new CollectionPage(contextPath, String.class, instrumentTypeCodes, Optional.ofNullable(instrumentTypeCodesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList());
}
@Property(name="OptionMonthCodes")
@JsonIgnore
public CollectionPage getOptionMonthCodes() {
return new CollectionPage(contextPath, String.class, optionMonthCodes, Optional.ofNullable(optionMonthCodesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList());
}
@Property(name="OptionTypeCodes")
@JsonIgnore
public CollectionPage getOptionTypeCodes() {
return new CollectionPage(contextPath, String.class, optionTypeCodes, Optional.ofNullable(optionTypeCodesNextLink), EdmSchemaInfo.INSTANCE, Collections.emptyList());
}
@Property(name="CouponRate")
@JsonIgnore
public Optional getCouponRate() {
return Optional.ofNullable(couponRate);
}
public HistoricalCriteriaSearchRequest withCouponRate(DecimalValueRange couponRate) {
HistoricalCriteriaSearchRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.HistoricalCriteriaSearchRequest");
_x.couponRate = couponRate;
return _x;
}
@Property(name="StrikePrice")
@JsonIgnore
public Optional getStrikePrice() {
return Optional.ofNullable(strikePrice);
}
public HistoricalCriteriaSearchRequest withStrikePrice(DecimalValueRange strikePrice) {
HistoricalCriteriaSearchRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.HistoricalCriteriaSearchRequest");
_x.strikePrice = strikePrice;
return _x;
}
@Property(name="ExpiryDate")
@JsonIgnore
public Optional getExpiryDate() {
return Optional.ofNullable(expiryDate);
}
public HistoricalCriteriaSearchRequest withExpiryDate(DateTimeRange expiryDate) {
HistoricalCriteriaSearchRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.HistoricalCriteriaSearchRequest");
_x.expiryDate = expiryDate;
return _x;
}
@Property(name="MaturityDate")
@JsonIgnore
public Optional getMaturityDate() {
return Optional.ofNullable(maturityDate);
}
public HistoricalCriteriaSearchRequest withMaturityDate(DateTimeRange maturityDate) {
HistoricalCriteriaSearchRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.HistoricalCriteriaSearchRequest");
_x.maturityDate = maturityDate;
return _x;
}
@Property(name="Range")
@JsonIgnore
public Optional getRange() {
return Optional.ofNullable(range);
}
public HistoricalCriteriaSearchRequest withRange(DateTimeRange range) {
HistoricalCriteriaSearchRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.HistoricalCriteriaSearchRequest");
_x.range = range;
return _x;
}
@Property(name="ResultsBy")
@JsonIgnore
public Optional getResultsBy() {
return Optional.ofNullable(resultsBy);
}
public HistoricalCriteriaSearchRequest withResultsBy(HistoricalResultsBy resultsBy) {
HistoricalCriteriaSearchRequest _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.HistoricalCriteriaSearchRequest");
_x.resultsBy = resultsBy;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String ricPattern;
private String descriptionPattern;
private List bondTypeCodes;
private String bondTypeCodesNextLink;
private List contributorIds;
private String contributorIdsNextLink;
private List countryCodes;
private String countryCodesNextLink;
private List currencyCodes;
private String currencyCodesNextLink;
private List domainCodes;
private String domainCodesNextLink;
private List exchangeCodes;
private String exchangeCodesNextLink;
private List futureMonthCodes;
private String futureMonthCodesNextLink;
private List instrumentTypeCodes;
private String instrumentTypeCodesNextLink;
private List optionMonthCodes;
private String optionMonthCodesNextLink;
private List optionTypeCodes;
private String optionTypeCodesNextLink;
private DecimalValueRange couponRate;
private DecimalValueRange strikePrice;
private DateTimeRange expiryDate;
private DateTimeRange maturityDate;
private DateTimeRange range;
private HistoricalResultsBy resultsBy;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder ricPattern(String ricPattern) {
this.ricPattern = ricPattern;
this.changedFields = changedFields.add("RicPattern");
return this;
}
public Builder descriptionPattern(String descriptionPattern) {
this.descriptionPattern = descriptionPattern;
this.changedFields = changedFields.add("DescriptionPattern");
return this;
}
public Builder bondTypeCodes(List bondTypeCodes) {
this.bondTypeCodes = bondTypeCodes;
this.changedFields = changedFields.add("BondTypeCodes");
return this;
}
public Builder bondTypeCodesNextLink(String bondTypeCodesNextLink) {
this.bondTypeCodesNextLink = bondTypeCodesNextLink;
this.changedFields = changedFields.add("BondTypeCodes");
return this;
}
public Builder contributorIds(List contributorIds) {
this.contributorIds = contributorIds;
this.changedFields = changedFields.add("ContributorIds");
return this;
}
public Builder contributorIdsNextLink(String contributorIdsNextLink) {
this.contributorIdsNextLink = contributorIdsNextLink;
this.changedFields = changedFields.add("ContributorIds");
return this;
}
public Builder countryCodes(List countryCodes) {
this.countryCodes = countryCodes;
this.changedFields = changedFields.add("CountryCodes");
return this;
}
public Builder countryCodesNextLink(String countryCodesNextLink) {
this.countryCodesNextLink = countryCodesNextLink;
this.changedFields = changedFields.add("CountryCodes");
return this;
}
public Builder currencyCodes(List currencyCodes) {
this.currencyCodes = currencyCodes;
this.changedFields = changedFields.add("CurrencyCodes");
return this;
}
public Builder currencyCodesNextLink(String currencyCodesNextLink) {
this.currencyCodesNextLink = currencyCodesNextLink;
this.changedFields = changedFields.add("CurrencyCodes");
return this;
}
public Builder domainCodes(List domainCodes) {
this.domainCodes = domainCodes;
this.changedFields = changedFields.add("DomainCodes");
return this;
}
public Builder domainCodesNextLink(String domainCodesNextLink) {
this.domainCodesNextLink = domainCodesNextLink;
this.changedFields = changedFields.add("DomainCodes");
return this;
}
public Builder exchangeCodes(List exchangeCodes) {
this.exchangeCodes = exchangeCodes;
this.changedFields = changedFields.add("ExchangeCodes");
return this;
}
public Builder exchangeCodesNextLink(String exchangeCodesNextLink) {
this.exchangeCodesNextLink = exchangeCodesNextLink;
this.changedFields = changedFields.add("ExchangeCodes");
return this;
}
public Builder futureMonthCodes(List futureMonthCodes) {
this.futureMonthCodes = futureMonthCodes;
this.changedFields = changedFields.add("FutureMonthCodes");
return this;
}
public Builder futureMonthCodesNextLink(String futureMonthCodesNextLink) {
this.futureMonthCodesNextLink = futureMonthCodesNextLink;
this.changedFields = changedFields.add("FutureMonthCodes");
return this;
}
public Builder instrumentTypeCodes(List instrumentTypeCodes) {
this.instrumentTypeCodes = instrumentTypeCodes;
this.changedFields = changedFields.add("InstrumentTypeCodes");
return this;
}
public Builder instrumentTypeCodesNextLink(String instrumentTypeCodesNextLink) {
this.instrumentTypeCodesNextLink = instrumentTypeCodesNextLink;
this.changedFields = changedFields.add("InstrumentTypeCodes");
return this;
}
public Builder optionMonthCodes(List optionMonthCodes) {
this.optionMonthCodes = optionMonthCodes;
this.changedFields = changedFields.add("OptionMonthCodes");
return this;
}
public Builder optionMonthCodesNextLink(String optionMonthCodesNextLink) {
this.optionMonthCodesNextLink = optionMonthCodesNextLink;
this.changedFields = changedFields.add("OptionMonthCodes");
return this;
}
public Builder optionTypeCodes(List optionTypeCodes) {
this.optionTypeCodes = optionTypeCodes;
this.changedFields = changedFields.add("OptionTypeCodes");
return this;
}
public Builder optionTypeCodesNextLink(String optionTypeCodesNextLink) {
this.optionTypeCodesNextLink = optionTypeCodesNextLink;
this.changedFields = changedFields.add("OptionTypeCodes");
return this;
}
public Builder couponRate(DecimalValueRange couponRate) {
this.couponRate = couponRate;
this.changedFields = changedFields.add("CouponRate");
return this;
}
public Builder strikePrice(DecimalValueRange strikePrice) {
this.strikePrice = strikePrice;
this.changedFields = changedFields.add("StrikePrice");
return this;
}
public Builder expiryDate(DateTimeRange expiryDate) {
this.expiryDate = expiryDate;
this.changedFields = changedFields.add("ExpiryDate");
return this;
}
public Builder maturityDate(DateTimeRange maturityDate) {
this.maturityDate = maturityDate;
this.changedFields = changedFields.add("MaturityDate");
return this;
}
public Builder range(DateTimeRange range) {
this.range = range;
this.changedFields = changedFields.add("Range");
return this;
}
public Builder resultsBy(HistoricalResultsBy resultsBy) {
this.resultsBy = resultsBy;
this.changedFields = changedFields.add("ResultsBy");
return this;
}
public HistoricalCriteriaSearchRequest build() {
HistoricalCriteriaSearchRequest _x = new HistoricalCriteriaSearchRequest();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "ThomsonReuters.Dss.Api.Search.HistoricalCriteriaSearchRequest";
_x.ricPattern = ricPattern;
_x.descriptionPattern = descriptionPattern;
_x.bondTypeCodes = bondTypeCodes;
_x.bondTypeCodesNextLink = bondTypeCodesNextLink;
_x.contributorIds = contributorIds;
_x.contributorIdsNextLink = contributorIdsNextLink;
_x.countryCodes = countryCodes;
_x.countryCodesNextLink = countryCodesNextLink;
_x.currencyCodes = currencyCodes;
_x.currencyCodesNextLink = currencyCodesNextLink;
_x.domainCodes = domainCodes;
_x.domainCodesNextLink = domainCodesNextLink;
_x.exchangeCodes = exchangeCodes;
_x.exchangeCodesNextLink = exchangeCodesNextLink;
_x.futureMonthCodes = futureMonthCodes;
_x.futureMonthCodesNextLink = futureMonthCodesNextLink;
_x.instrumentTypeCodes = instrumentTypeCodes;
_x.instrumentTypeCodesNextLink = instrumentTypeCodesNextLink;
_x.optionMonthCodes = optionMonthCodes;
_x.optionMonthCodesNextLink = optionMonthCodesNextLink;
_x.optionTypeCodes = optionTypeCodes;
_x.optionTypeCodesNextLink = optionTypeCodesNextLink;
_x.couponRate = couponRate;
_x.strikePrice = strikePrice;
_x.expiryDate = expiryDate;
_x.maturityDate = maturityDate;
_x.range = range;
_x.resultsBy = resultsBy;
return _x;
}
}
private HistoricalCriteriaSearchRequest _copy() {
HistoricalCriteriaSearchRequest _x = new HistoricalCriteriaSearchRequest();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.ricPattern = ricPattern;
_x.descriptionPattern = descriptionPattern;
_x.bondTypeCodes = bondTypeCodes;
_x.contributorIds = contributorIds;
_x.countryCodes = countryCodes;
_x.currencyCodes = currencyCodes;
_x.domainCodes = domainCodes;
_x.exchangeCodes = exchangeCodes;
_x.futureMonthCodes = futureMonthCodes;
_x.instrumentTypeCodes = instrumentTypeCodes;
_x.optionMonthCodes = optionMonthCodes;
_x.optionTypeCodes = optionTypeCodes;
_x.couponRate = couponRate;
_x.strikePrice = strikePrice;
_x.expiryDate = expiryDate;
_x.maturityDate = maturityDate;
_x.range = range;
_x.resultsBy = resultsBy;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("HistoricalCriteriaSearchRequest[");
b.append("RicPattern=");
b.append(this.ricPattern);
b.append(", ");
b.append("DescriptionPattern=");
b.append(this.descriptionPattern);
b.append(", ");
b.append("BondTypeCodes=");
b.append(this.bondTypeCodes);
b.append(", ");
b.append("ContributorIds=");
b.append(this.contributorIds);
b.append(", ");
b.append("CountryCodes=");
b.append(this.countryCodes);
b.append(", ");
b.append("CurrencyCodes=");
b.append(this.currencyCodes);
b.append(", ");
b.append("DomainCodes=");
b.append(this.domainCodes);
b.append(", ");
b.append("ExchangeCodes=");
b.append(this.exchangeCodes);
b.append(", ");
b.append("FutureMonthCodes=");
b.append(this.futureMonthCodes);
b.append(", ");
b.append("InstrumentTypeCodes=");
b.append(this.instrumentTypeCodes);
b.append(", ");
b.append("OptionMonthCodes=");
b.append(this.optionMonthCodes);
b.append(", ");
b.append("OptionTypeCodes=");
b.append(this.optionTypeCodes);
b.append(", ");
b.append("CouponRate=");
b.append(this.couponRate);
b.append(", ");
b.append("StrikePrice=");
b.append(this.strikePrice);
b.append(", ");
b.append("ExpiryDate=");
b.append(this.expiryDate);
b.append(", ");
b.append("MaturityDate=");
b.append(this.maturityDate);
b.append(", ");
b.append("Range=");
b.append(this.range);
b.append(", ");
b.append("ResultsBy=");
b.append(this.resultsBy);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy