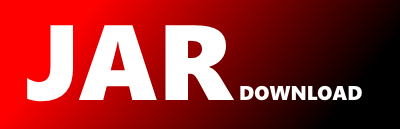
thomsonreuters.dss.api.search.complex.MifidSubclassSearchResult Maven / Gradle / Ivy
package thomsonreuters.dss.api.search.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFields;
import java.time.OffsetDateTime;
import java.util.Optional;
import thomsonreuters.dss.api.content.complex.ValidatedInstrument;
import thomsonreuters.dss.api.content.enums.IdentifierType;
import thomsonreuters.dss.api.content.enums.InstrumentType;
import thomsonreuters.dss.api.content.enums.ValidityStatus;
@JsonInclude(Include.NON_NULL)
@JsonPropertyOrder({
"@odata.type",
"AssetClassCode",
"AssetClassDescription",
"SubAssetClassCode",
"SubAssetClassDescription",
"SubAssetClassPermId",
"SubClassPermId",
"CdsSubClass",
"DeliveryCashSettleLocation",
"FreightType",
"FreightSubType",
"FreightSize",
"LoadType",
"MifidContractType",
"MifidLiquidityFlagEsma",
"ThresholdEffectiveDate",
"ThresholdEndDate",
"NotionalCurrency",
"NotionalCurrencyPair",
"Parameter",
"PostTradeLisThresholdValue",
"PostTradeSstiThresholdValue",
"PreTradeLisThresholdValue",
"PreTradeSstiThresholdValue",
"SettlementType",
"SpecificRouteOrTimeCharterAverage",
"UnderlyingAssetType",
"UnderlyingCommodity",
"TermOfTheUnderlyingInterestRate",
"TermOfTheUnderlyingBond",
"TimeToMaturityBucket",
"TimeToMaturityBucketOfTheOption",
"MifidUnderlyingIndexName",
"UnderlyingInterestRate",
"UnderlyingIsin",
"UnderlyingIssuerLei",
"UnderlyingReferenceEntityType"})
public class MifidSubclassSearchResult extends ValidatedInstrument implements ODataType {
@JacksonInject
@JsonIgnore
protected UnmappedFields unmappedFields;
@JsonProperty("AssetClassCode")
protected String assetClassCode;
@JsonProperty("AssetClassDescription")
protected String assetClassDescription;
@JsonProperty("SubAssetClassCode")
protected String subAssetClassCode;
@JsonProperty("SubAssetClassDescription")
protected String subAssetClassDescription;
@JsonProperty("SubAssetClassPermId")
protected String subAssetClassPermId;
@JsonProperty("SubClassPermId")
protected String subClassPermId;
@JsonProperty("CdsSubClass")
protected String cdsSubClass;
@JsonProperty("DeliveryCashSettleLocation")
protected String deliveryCashSettleLocation;
@JsonProperty("FreightType")
protected String freightType;
@JsonProperty("FreightSubType")
protected String freightSubType;
@JsonProperty("FreightSize")
protected String freightSize;
@JsonProperty("LoadType")
protected String loadType;
@JsonProperty("MifidContractType")
protected String mifidContractType;
@JsonProperty("MifidLiquidityFlagEsma")
protected String mifidLiquidityFlagEsma;
@JsonProperty("ThresholdEffectiveDate")
protected OffsetDateTime thresholdEffectiveDate;
@JsonProperty("ThresholdEndDate")
protected OffsetDateTime thresholdEndDate;
@JsonProperty("NotionalCurrency")
protected String notionalCurrency;
@JsonProperty("NotionalCurrencyPair")
protected String notionalCurrencyPair;
@JsonProperty("Parameter")
protected String parameter;
@JsonProperty("PostTradeLisThresholdValue")
protected Long postTradeLisThresholdValue;
@JsonProperty("PostTradeSstiThresholdValue")
protected Long postTradeSstiThresholdValue;
@JsonProperty("PreTradeLisThresholdValue")
protected Long preTradeLisThresholdValue;
@JsonProperty("PreTradeSstiThresholdValue")
protected Long preTradeSstiThresholdValue;
@JsonProperty("SettlementType")
protected String settlementType;
@JsonProperty("SpecificRouteOrTimeCharterAverage")
protected String specificRouteOrTimeCharterAverage;
@JsonProperty("UnderlyingAssetType")
protected String underlyingAssetType;
@JsonProperty("UnderlyingCommodity")
protected String underlyingCommodity;
@JsonProperty("TermOfTheUnderlyingInterestRate")
protected String termOfTheUnderlyingInterestRate;
@JsonProperty("TermOfTheUnderlyingBond")
protected String termOfTheUnderlyingBond;
@JsonProperty("TimeToMaturityBucket")
protected String timeToMaturityBucket;
@JsonProperty("TimeToMaturityBucketOfTheOption")
protected String timeToMaturityBucketOfTheOption;
@JsonProperty("MifidUnderlyingIndexName")
protected String mifidUnderlyingIndexName;
@JsonProperty("UnderlyingInterestRate")
protected String underlyingInterestRate;
@JsonProperty("UnderlyingIsin")
protected String underlyingIsin;
@JsonProperty("UnderlyingIssuerLei")
protected String underlyingIssuerLei;
@JsonProperty("UnderlyingReferenceEntityType")
protected String underlyingReferenceEntityType;
protected MifidSubclassSearchResult() {
super();
}
@Override
public String odataTypeName() {
return "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult";
}
@Property(name="AssetClassCode")
@JsonIgnore
public Optional getAssetClassCode() {
return Optional.ofNullable(assetClassCode);
}
public MifidSubclassSearchResult withAssetClassCode(String assetClassCode) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.assetClassCode = assetClassCode;
return _x;
}
@Property(name="AssetClassDescription")
@JsonIgnore
public Optional getAssetClassDescription() {
return Optional.ofNullable(assetClassDescription);
}
public MifidSubclassSearchResult withAssetClassDescription(String assetClassDescription) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.assetClassDescription = assetClassDescription;
return _x;
}
@Property(name="SubAssetClassCode")
@JsonIgnore
public Optional getSubAssetClassCode() {
return Optional.ofNullable(subAssetClassCode);
}
public MifidSubclassSearchResult withSubAssetClassCode(String subAssetClassCode) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.subAssetClassCode = subAssetClassCode;
return _x;
}
@Property(name="SubAssetClassDescription")
@JsonIgnore
public Optional getSubAssetClassDescription() {
return Optional.ofNullable(subAssetClassDescription);
}
public MifidSubclassSearchResult withSubAssetClassDescription(String subAssetClassDescription) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.subAssetClassDescription = subAssetClassDescription;
return _x;
}
@Property(name="SubAssetClassPermId")
@JsonIgnore
public Optional getSubAssetClassPermId() {
return Optional.ofNullable(subAssetClassPermId);
}
public MifidSubclassSearchResult withSubAssetClassPermId(String subAssetClassPermId) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.subAssetClassPermId = subAssetClassPermId;
return _x;
}
@Property(name="SubClassPermId")
@JsonIgnore
public Optional getSubClassPermId() {
return Optional.ofNullable(subClassPermId);
}
public MifidSubclassSearchResult withSubClassPermId(String subClassPermId) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.subClassPermId = subClassPermId;
return _x;
}
@Property(name="CdsSubClass")
@JsonIgnore
public Optional getCdsSubClass() {
return Optional.ofNullable(cdsSubClass);
}
public MifidSubclassSearchResult withCdsSubClass(String cdsSubClass) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.cdsSubClass = cdsSubClass;
return _x;
}
@Property(name="DeliveryCashSettleLocation")
@JsonIgnore
public Optional getDeliveryCashSettleLocation() {
return Optional.ofNullable(deliveryCashSettleLocation);
}
public MifidSubclassSearchResult withDeliveryCashSettleLocation(String deliveryCashSettleLocation) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.deliveryCashSettleLocation = deliveryCashSettleLocation;
return _x;
}
@Property(name="FreightType")
@JsonIgnore
public Optional getFreightType() {
return Optional.ofNullable(freightType);
}
public MifidSubclassSearchResult withFreightType(String freightType) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.freightType = freightType;
return _x;
}
@Property(name="FreightSubType")
@JsonIgnore
public Optional getFreightSubType() {
return Optional.ofNullable(freightSubType);
}
public MifidSubclassSearchResult withFreightSubType(String freightSubType) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.freightSubType = freightSubType;
return _x;
}
@Property(name="FreightSize")
@JsonIgnore
public Optional getFreightSize() {
return Optional.ofNullable(freightSize);
}
public MifidSubclassSearchResult withFreightSize(String freightSize) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.freightSize = freightSize;
return _x;
}
@Property(name="LoadType")
@JsonIgnore
public Optional getLoadType() {
return Optional.ofNullable(loadType);
}
public MifidSubclassSearchResult withLoadType(String loadType) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.loadType = loadType;
return _x;
}
@Property(name="MifidContractType")
@JsonIgnore
public Optional getMifidContractType() {
return Optional.ofNullable(mifidContractType);
}
public MifidSubclassSearchResult withMifidContractType(String mifidContractType) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.mifidContractType = mifidContractType;
return _x;
}
@Property(name="MifidLiquidityFlagEsma")
@JsonIgnore
public Optional getMifidLiquidityFlagEsma() {
return Optional.ofNullable(mifidLiquidityFlagEsma);
}
public MifidSubclassSearchResult withMifidLiquidityFlagEsma(String mifidLiquidityFlagEsma) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.mifidLiquidityFlagEsma = mifidLiquidityFlagEsma;
return _x;
}
@Property(name="ThresholdEffectiveDate")
@JsonIgnore
public Optional getThresholdEffectiveDate() {
return Optional.ofNullable(thresholdEffectiveDate);
}
public MifidSubclassSearchResult withThresholdEffectiveDate(OffsetDateTime thresholdEffectiveDate) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.thresholdEffectiveDate = thresholdEffectiveDate;
return _x;
}
@Property(name="ThresholdEndDate")
@JsonIgnore
public Optional getThresholdEndDate() {
return Optional.ofNullable(thresholdEndDate);
}
public MifidSubclassSearchResult withThresholdEndDate(OffsetDateTime thresholdEndDate) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.thresholdEndDate = thresholdEndDate;
return _x;
}
@Property(name="NotionalCurrency")
@JsonIgnore
public Optional getNotionalCurrency() {
return Optional.ofNullable(notionalCurrency);
}
public MifidSubclassSearchResult withNotionalCurrency(String notionalCurrency) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.notionalCurrency = notionalCurrency;
return _x;
}
@Property(name="NotionalCurrencyPair")
@JsonIgnore
public Optional getNotionalCurrencyPair() {
return Optional.ofNullable(notionalCurrencyPair);
}
public MifidSubclassSearchResult withNotionalCurrencyPair(String notionalCurrencyPair) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.notionalCurrencyPair = notionalCurrencyPair;
return _x;
}
@Property(name="Parameter")
@JsonIgnore
public Optional getParameter() {
return Optional.ofNullable(parameter);
}
public MifidSubclassSearchResult withParameter(String parameter) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.parameter = parameter;
return _x;
}
@Property(name="PostTradeLisThresholdValue")
@JsonIgnore
public Optional getPostTradeLisThresholdValue() {
return Optional.ofNullable(postTradeLisThresholdValue);
}
public MifidSubclassSearchResult withPostTradeLisThresholdValue(Long postTradeLisThresholdValue) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.postTradeLisThresholdValue = postTradeLisThresholdValue;
return _x;
}
@Property(name="PostTradeSstiThresholdValue")
@JsonIgnore
public Optional getPostTradeSstiThresholdValue() {
return Optional.ofNullable(postTradeSstiThresholdValue);
}
public MifidSubclassSearchResult withPostTradeSstiThresholdValue(Long postTradeSstiThresholdValue) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.postTradeSstiThresholdValue = postTradeSstiThresholdValue;
return _x;
}
@Property(name="PreTradeLisThresholdValue")
@JsonIgnore
public Optional getPreTradeLisThresholdValue() {
return Optional.ofNullable(preTradeLisThresholdValue);
}
public MifidSubclassSearchResult withPreTradeLisThresholdValue(Long preTradeLisThresholdValue) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.preTradeLisThresholdValue = preTradeLisThresholdValue;
return _x;
}
@Property(name="PreTradeSstiThresholdValue")
@JsonIgnore
public Optional getPreTradeSstiThresholdValue() {
return Optional.ofNullable(preTradeSstiThresholdValue);
}
public MifidSubclassSearchResult withPreTradeSstiThresholdValue(Long preTradeSstiThresholdValue) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.preTradeSstiThresholdValue = preTradeSstiThresholdValue;
return _x;
}
@Property(name="SettlementType")
@JsonIgnore
public Optional getSettlementType() {
return Optional.ofNullable(settlementType);
}
public MifidSubclassSearchResult withSettlementType(String settlementType) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.settlementType = settlementType;
return _x;
}
@Property(name="SpecificRouteOrTimeCharterAverage")
@JsonIgnore
public Optional getSpecificRouteOrTimeCharterAverage() {
return Optional.ofNullable(specificRouteOrTimeCharterAverage);
}
public MifidSubclassSearchResult withSpecificRouteOrTimeCharterAverage(String specificRouteOrTimeCharterAverage) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.specificRouteOrTimeCharterAverage = specificRouteOrTimeCharterAverage;
return _x;
}
@Property(name="UnderlyingAssetType")
@JsonIgnore
public Optional getUnderlyingAssetType() {
return Optional.ofNullable(underlyingAssetType);
}
public MifidSubclassSearchResult withUnderlyingAssetType(String underlyingAssetType) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.underlyingAssetType = underlyingAssetType;
return _x;
}
@Property(name="UnderlyingCommodity")
@JsonIgnore
public Optional getUnderlyingCommodity() {
return Optional.ofNullable(underlyingCommodity);
}
public MifidSubclassSearchResult withUnderlyingCommodity(String underlyingCommodity) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.underlyingCommodity = underlyingCommodity;
return _x;
}
@Property(name="TermOfTheUnderlyingInterestRate")
@JsonIgnore
public Optional getTermOfTheUnderlyingInterestRate() {
return Optional.ofNullable(termOfTheUnderlyingInterestRate);
}
public MifidSubclassSearchResult withTermOfTheUnderlyingInterestRate(String termOfTheUnderlyingInterestRate) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.termOfTheUnderlyingInterestRate = termOfTheUnderlyingInterestRate;
return _x;
}
@Property(name="TermOfTheUnderlyingBond")
@JsonIgnore
public Optional getTermOfTheUnderlyingBond() {
return Optional.ofNullable(termOfTheUnderlyingBond);
}
public MifidSubclassSearchResult withTermOfTheUnderlyingBond(String termOfTheUnderlyingBond) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.termOfTheUnderlyingBond = termOfTheUnderlyingBond;
return _x;
}
@Property(name="TimeToMaturityBucket")
@JsonIgnore
public Optional getTimeToMaturityBucket() {
return Optional.ofNullable(timeToMaturityBucket);
}
public MifidSubclassSearchResult withTimeToMaturityBucket(String timeToMaturityBucket) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.timeToMaturityBucket = timeToMaturityBucket;
return _x;
}
@Property(name="TimeToMaturityBucketOfTheOption")
@JsonIgnore
public Optional getTimeToMaturityBucketOfTheOption() {
return Optional.ofNullable(timeToMaturityBucketOfTheOption);
}
public MifidSubclassSearchResult withTimeToMaturityBucketOfTheOption(String timeToMaturityBucketOfTheOption) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.timeToMaturityBucketOfTheOption = timeToMaturityBucketOfTheOption;
return _x;
}
@Property(name="MifidUnderlyingIndexName")
@JsonIgnore
public Optional getMifidUnderlyingIndexName() {
return Optional.ofNullable(mifidUnderlyingIndexName);
}
public MifidSubclassSearchResult withMifidUnderlyingIndexName(String mifidUnderlyingIndexName) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.mifidUnderlyingIndexName = mifidUnderlyingIndexName;
return _x;
}
@Property(name="UnderlyingInterestRate")
@JsonIgnore
public Optional getUnderlyingInterestRate() {
return Optional.ofNullable(underlyingInterestRate);
}
public MifidSubclassSearchResult withUnderlyingInterestRate(String underlyingInterestRate) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.underlyingInterestRate = underlyingInterestRate;
return _x;
}
@Property(name="UnderlyingIsin")
@JsonIgnore
public Optional getUnderlyingIsin() {
return Optional.ofNullable(underlyingIsin);
}
public MifidSubclassSearchResult withUnderlyingIsin(String underlyingIsin) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.underlyingIsin = underlyingIsin;
return _x;
}
@Property(name="UnderlyingIssuerLei")
@JsonIgnore
public Optional getUnderlyingIssuerLei() {
return Optional.ofNullable(underlyingIssuerLei);
}
public MifidSubclassSearchResult withUnderlyingIssuerLei(String underlyingIssuerLei) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.underlyingIssuerLei = underlyingIssuerLei;
return _x;
}
@Property(name="UnderlyingReferenceEntityType")
@JsonIgnore
public Optional getUnderlyingReferenceEntityType() {
return Optional.ofNullable(underlyingReferenceEntityType);
}
public MifidSubclassSearchResult withUnderlyingReferenceEntityType(String underlyingReferenceEntityType) {
MifidSubclassSearchResult _x = _copy();
_x.odataType = Util.nvl(odataType, "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult");
_x.underlyingReferenceEntityType = underlyingReferenceEntityType;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFields();
}
unmappedFields.put(name, value);
}
@Override
@JsonIgnore
public UnmappedFields getUnmappedFields() {
return unmappedFields == null ? new UnmappedFields() : unmappedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderMifidSubclassSearchResult() {
return new Builder();
}
public static final class Builder {
private String key;
private String description;
private InstrumentType instrumentType;
private ValidityStatus status;
private String source;
private String identifier;
private IdentifierType identifierType;
private String userDefinedIdentifier;
private String userDefinedIdentifier2;
private String userDefinedIdentifier3;
private String userDefinedIdentifier4;
private String userDefinedIdentifier5;
private String userDefinedIdentifier6;
private String assetClassCode;
private String assetClassDescription;
private String subAssetClassCode;
private String subAssetClassDescription;
private String subAssetClassPermId;
private String subClassPermId;
private String cdsSubClass;
private String deliveryCashSettleLocation;
private String freightType;
private String freightSubType;
private String freightSize;
private String loadType;
private String mifidContractType;
private String mifidLiquidityFlagEsma;
private OffsetDateTime thresholdEffectiveDate;
private OffsetDateTime thresholdEndDate;
private String notionalCurrency;
private String notionalCurrencyPair;
private String parameter;
private Long postTradeLisThresholdValue;
private Long postTradeSstiThresholdValue;
private Long preTradeLisThresholdValue;
private Long preTradeSstiThresholdValue;
private String settlementType;
private String specificRouteOrTimeCharterAverage;
private String underlyingAssetType;
private String underlyingCommodity;
private String termOfTheUnderlyingInterestRate;
private String termOfTheUnderlyingBond;
private String timeToMaturityBucket;
private String timeToMaturityBucketOfTheOption;
private String mifidUnderlyingIndexName;
private String underlyingInterestRate;
private String underlyingIsin;
private String underlyingIssuerLei;
private String underlyingReferenceEntityType;
private ChangedFields changedFields = new ChangedFields();
Builder() {
// prevent instantiation
}
public Builder key(String key) {
this.key = key;
this.changedFields = changedFields.add("Key");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("Description");
return this;
}
public Builder instrumentType(InstrumentType instrumentType) {
this.instrumentType = instrumentType;
this.changedFields = changedFields.add("InstrumentType");
return this;
}
public Builder status(ValidityStatus status) {
this.status = status;
this.changedFields = changedFields.add("Status");
return this;
}
public Builder source(String source) {
this.source = source;
this.changedFields = changedFields.add("Source");
return this;
}
public Builder identifier(String identifier) {
this.identifier = identifier;
this.changedFields = changedFields.add("Identifier");
return this;
}
public Builder identifierType(IdentifierType identifierType) {
this.identifierType = identifierType;
this.changedFields = changedFields.add("IdentifierType");
return this;
}
public Builder userDefinedIdentifier(String userDefinedIdentifier) {
this.userDefinedIdentifier = userDefinedIdentifier;
this.changedFields = changedFields.add("UserDefinedIdentifier");
return this;
}
public Builder userDefinedIdentifier2(String userDefinedIdentifier2) {
this.userDefinedIdentifier2 = userDefinedIdentifier2;
this.changedFields = changedFields.add("UserDefinedIdentifier2");
return this;
}
public Builder userDefinedIdentifier3(String userDefinedIdentifier3) {
this.userDefinedIdentifier3 = userDefinedIdentifier3;
this.changedFields = changedFields.add("UserDefinedIdentifier3");
return this;
}
public Builder userDefinedIdentifier4(String userDefinedIdentifier4) {
this.userDefinedIdentifier4 = userDefinedIdentifier4;
this.changedFields = changedFields.add("UserDefinedIdentifier4");
return this;
}
public Builder userDefinedIdentifier5(String userDefinedIdentifier5) {
this.userDefinedIdentifier5 = userDefinedIdentifier5;
this.changedFields = changedFields.add("UserDefinedIdentifier5");
return this;
}
public Builder userDefinedIdentifier6(String userDefinedIdentifier6) {
this.userDefinedIdentifier6 = userDefinedIdentifier6;
this.changedFields = changedFields.add("UserDefinedIdentifier6");
return this;
}
public Builder assetClassCode(String assetClassCode) {
this.assetClassCode = assetClassCode;
this.changedFields = changedFields.add("AssetClassCode");
return this;
}
public Builder assetClassDescription(String assetClassDescription) {
this.assetClassDescription = assetClassDescription;
this.changedFields = changedFields.add("AssetClassDescription");
return this;
}
public Builder subAssetClassCode(String subAssetClassCode) {
this.subAssetClassCode = subAssetClassCode;
this.changedFields = changedFields.add("SubAssetClassCode");
return this;
}
public Builder subAssetClassDescription(String subAssetClassDescription) {
this.subAssetClassDescription = subAssetClassDescription;
this.changedFields = changedFields.add("SubAssetClassDescription");
return this;
}
public Builder subAssetClassPermId(String subAssetClassPermId) {
this.subAssetClassPermId = subAssetClassPermId;
this.changedFields = changedFields.add("SubAssetClassPermId");
return this;
}
public Builder subClassPermId(String subClassPermId) {
this.subClassPermId = subClassPermId;
this.changedFields = changedFields.add("SubClassPermId");
return this;
}
public Builder cdsSubClass(String cdsSubClass) {
this.cdsSubClass = cdsSubClass;
this.changedFields = changedFields.add("CdsSubClass");
return this;
}
public Builder deliveryCashSettleLocation(String deliveryCashSettleLocation) {
this.deliveryCashSettleLocation = deliveryCashSettleLocation;
this.changedFields = changedFields.add("DeliveryCashSettleLocation");
return this;
}
public Builder freightType(String freightType) {
this.freightType = freightType;
this.changedFields = changedFields.add("FreightType");
return this;
}
public Builder freightSubType(String freightSubType) {
this.freightSubType = freightSubType;
this.changedFields = changedFields.add("FreightSubType");
return this;
}
public Builder freightSize(String freightSize) {
this.freightSize = freightSize;
this.changedFields = changedFields.add("FreightSize");
return this;
}
public Builder loadType(String loadType) {
this.loadType = loadType;
this.changedFields = changedFields.add("LoadType");
return this;
}
public Builder mifidContractType(String mifidContractType) {
this.mifidContractType = mifidContractType;
this.changedFields = changedFields.add("MifidContractType");
return this;
}
public Builder mifidLiquidityFlagEsma(String mifidLiquidityFlagEsma) {
this.mifidLiquidityFlagEsma = mifidLiquidityFlagEsma;
this.changedFields = changedFields.add("MifidLiquidityFlagEsma");
return this;
}
public Builder thresholdEffectiveDate(OffsetDateTime thresholdEffectiveDate) {
this.thresholdEffectiveDate = thresholdEffectiveDate;
this.changedFields = changedFields.add("ThresholdEffectiveDate");
return this;
}
public Builder thresholdEndDate(OffsetDateTime thresholdEndDate) {
this.thresholdEndDate = thresholdEndDate;
this.changedFields = changedFields.add("ThresholdEndDate");
return this;
}
public Builder notionalCurrency(String notionalCurrency) {
this.notionalCurrency = notionalCurrency;
this.changedFields = changedFields.add("NotionalCurrency");
return this;
}
public Builder notionalCurrencyPair(String notionalCurrencyPair) {
this.notionalCurrencyPair = notionalCurrencyPair;
this.changedFields = changedFields.add("NotionalCurrencyPair");
return this;
}
public Builder parameter(String parameter) {
this.parameter = parameter;
this.changedFields = changedFields.add("Parameter");
return this;
}
public Builder postTradeLisThresholdValue(Long postTradeLisThresholdValue) {
this.postTradeLisThresholdValue = postTradeLisThresholdValue;
this.changedFields = changedFields.add("PostTradeLisThresholdValue");
return this;
}
public Builder postTradeSstiThresholdValue(Long postTradeSstiThresholdValue) {
this.postTradeSstiThresholdValue = postTradeSstiThresholdValue;
this.changedFields = changedFields.add("PostTradeSstiThresholdValue");
return this;
}
public Builder preTradeLisThresholdValue(Long preTradeLisThresholdValue) {
this.preTradeLisThresholdValue = preTradeLisThresholdValue;
this.changedFields = changedFields.add("PreTradeLisThresholdValue");
return this;
}
public Builder preTradeSstiThresholdValue(Long preTradeSstiThresholdValue) {
this.preTradeSstiThresholdValue = preTradeSstiThresholdValue;
this.changedFields = changedFields.add("PreTradeSstiThresholdValue");
return this;
}
public Builder settlementType(String settlementType) {
this.settlementType = settlementType;
this.changedFields = changedFields.add("SettlementType");
return this;
}
public Builder specificRouteOrTimeCharterAverage(String specificRouteOrTimeCharterAverage) {
this.specificRouteOrTimeCharterAverage = specificRouteOrTimeCharterAverage;
this.changedFields = changedFields.add("SpecificRouteOrTimeCharterAverage");
return this;
}
public Builder underlyingAssetType(String underlyingAssetType) {
this.underlyingAssetType = underlyingAssetType;
this.changedFields = changedFields.add("UnderlyingAssetType");
return this;
}
public Builder underlyingCommodity(String underlyingCommodity) {
this.underlyingCommodity = underlyingCommodity;
this.changedFields = changedFields.add("UnderlyingCommodity");
return this;
}
public Builder termOfTheUnderlyingInterestRate(String termOfTheUnderlyingInterestRate) {
this.termOfTheUnderlyingInterestRate = termOfTheUnderlyingInterestRate;
this.changedFields = changedFields.add("TermOfTheUnderlyingInterestRate");
return this;
}
public Builder termOfTheUnderlyingBond(String termOfTheUnderlyingBond) {
this.termOfTheUnderlyingBond = termOfTheUnderlyingBond;
this.changedFields = changedFields.add("TermOfTheUnderlyingBond");
return this;
}
public Builder timeToMaturityBucket(String timeToMaturityBucket) {
this.timeToMaturityBucket = timeToMaturityBucket;
this.changedFields = changedFields.add("TimeToMaturityBucket");
return this;
}
public Builder timeToMaturityBucketOfTheOption(String timeToMaturityBucketOfTheOption) {
this.timeToMaturityBucketOfTheOption = timeToMaturityBucketOfTheOption;
this.changedFields = changedFields.add("TimeToMaturityBucketOfTheOption");
return this;
}
public Builder mifidUnderlyingIndexName(String mifidUnderlyingIndexName) {
this.mifidUnderlyingIndexName = mifidUnderlyingIndexName;
this.changedFields = changedFields.add("MifidUnderlyingIndexName");
return this;
}
public Builder underlyingInterestRate(String underlyingInterestRate) {
this.underlyingInterestRate = underlyingInterestRate;
this.changedFields = changedFields.add("UnderlyingInterestRate");
return this;
}
public Builder underlyingIsin(String underlyingIsin) {
this.underlyingIsin = underlyingIsin;
this.changedFields = changedFields.add("UnderlyingIsin");
return this;
}
public Builder underlyingIssuerLei(String underlyingIssuerLei) {
this.underlyingIssuerLei = underlyingIssuerLei;
this.changedFields = changedFields.add("UnderlyingIssuerLei");
return this;
}
public Builder underlyingReferenceEntityType(String underlyingReferenceEntityType) {
this.underlyingReferenceEntityType = underlyingReferenceEntityType;
this.changedFields = changedFields.add("UnderlyingReferenceEntityType");
return this;
}
public MifidSubclassSearchResult build() {
MifidSubclassSearchResult _x = new MifidSubclassSearchResult();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFields();
_x.odataType = "ThomsonReuters.Dss.Api.Search.MifidSubclassSearchResult";
_x.key = key;
_x.description = description;
_x.instrumentType = instrumentType;
_x.status = status;
_x.source = source;
_x.identifier = identifier;
_x.identifierType = identifierType;
_x.userDefinedIdentifier = userDefinedIdentifier;
_x.userDefinedIdentifier2 = userDefinedIdentifier2;
_x.userDefinedIdentifier3 = userDefinedIdentifier3;
_x.userDefinedIdentifier4 = userDefinedIdentifier4;
_x.userDefinedIdentifier5 = userDefinedIdentifier5;
_x.userDefinedIdentifier6 = userDefinedIdentifier6;
_x.assetClassCode = assetClassCode;
_x.assetClassDescription = assetClassDescription;
_x.subAssetClassCode = subAssetClassCode;
_x.subAssetClassDescription = subAssetClassDescription;
_x.subAssetClassPermId = subAssetClassPermId;
_x.subClassPermId = subClassPermId;
_x.cdsSubClass = cdsSubClass;
_x.deliveryCashSettleLocation = deliveryCashSettleLocation;
_x.freightType = freightType;
_x.freightSubType = freightSubType;
_x.freightSize = freightSize;
_x.loadType = loadType;
_x.mifidContractType = mifidContractType;
_x.mifidLiquidityFlagEsma = mifidLiquidityFlagEsma;
_x.thresholdEffectiveDate = thresholdEffectiveDate;
_x.thresholdEndDate = thresholdEndDate;
_x.notionalCurrency = notionalCurrency;
_x.notionalCurrencyPair = notionalCurrencyPair;
_x.parameter = parameter;
_x.postTradeLisThresholdValue = postTradeLisThresholdValue;
_x.postTradeSstiThresholdValue = postTradeSstiThresholdValue;
_x.preTradeLisThresholdValue = preTradeLisThresholdValue;
_x.preTradeSstiThresholdValue = preTradeSstiThresholdValue;
_x.settlementType = settlementType;
_x.specificRouteOrTimeCharterAverage = specificRouteOrTimeCharterAverage;
_x.underlyingAssetType = underlyingAssetType;
_x.underlyingCommodity = underlyingCommodity;
_x.termOfTheUnderlyingInterestRate = termOfTheUnderlyingInterestRate;
_x.termOfTheUnderlyingBond = termOfTheUnderlyingBond;
_x.timeToMaturityBucket = timeToMaturityBucket;
_x.timeToMaturityBucketOfTheOption = timeToMaturityBucketOfTheOption;
_x.mifidUnderlyingIndexName = mifidUnderlyingIndexName;
_x.underlyingInterestRate = underlyingInterestRate;
_x.underlyingIsin = underlyingIsin;
_x.underlyingIssuerLei = underlyingIssuerLei;
_x.underlyingReferenceEntityType = underlyingReferenceEntityType;
return _x;
}
}
private MifidSubclassSearchResult _copy() {
MifidSubclassSearchResult _x = new MifidSubclassSearchResult();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields;
_x.odataType = odataType;
_x.key = key;
_x.description = description;
_x.instrumentType = instrumentType;
_x.status = status;
_x.source = source;
_x.identifier = identifier;
_x.identifierType = identifierType;
_x.userDefinedIdentifier = userDefinedIdentifier;
_x.userDefinedIdentifier2 = userDefinedIdentifier2;
_x.userDefinedIdentifier3 = userDefinedIdentifier3;
_x.userDefinedIdentifier4 = userDefinedIdentifier4;
_x.userDefinedIdentifier5 = userDefinedIdentifier5;
_x.userDefinedIdentifier6 = userDefinedIdentifier6;
_x.assetClassCode = assetClassCode;
_x.assetClassDescription = assetClassDescription;
_x.subAssetClassCode = subAssetClassCode;
_x.subAssetClassDescription = subAssetClassDescription;
_x.subAssetClassPermId = subAssetClassPermId;
_x.subClassPermId = subClassPermId;
_x.cdsSubClass = cdsSubClass;
_x.deliveryCashSettleLocation = deliveryCashSettleLocation;
_x.freightType = freightType;
_x.freightSubType = freightSubType;
_x.freightSize = freightSize;
_x.loadType = loadType;
_x.mifidContractType = mifidContractType;
_x.mifidLiquidityFlagEsma = mifidLiquidityFlagEsma;
_x.thresholdEffectiveDate = thresholdEffectiveDate;
_x.thresholdEndDate = thresholdEndDate;
_x.notionalCurrency = notionalCurrency;
_x.notionalCurrencyPair = notionalCurrencyPair;
_x.parameter = parameter;
_x.postTradeLisThresholdValue = postTradeLisThresholdValue;
_x.postTradeSstiThresholdValue = postTradeSstiThresholdValue;
_x.preTradeLisThresholdValue = preTradeLisThresholdValue;
_x.preTradeSstiThresholdValue = preTradeSstiThresholdValue;
_x.settlementType = settlementType;
_x.specificRouteOrTimeCharterAverage = specificRouteOrTimeCharterAverage;
_x.underlyingAssetType = underlyingAssetType;
_x.underlyingCommodity = underlyingCommodity;
_x.termOfTheUnderlyingInterestRate = termOfTheUnderlyingInterestRate;
_x.termOfTheUnderlyingBond = termOfTheUnderlyingBond;
_x.timeToMaturityBucket = timeToMaturityBucket;
_x.timeToMaturityBucketOfTheOption = timeToMaturityBucketOfTheOption;
_x.mifidUnderlyingIndexName = mifidUnderlyingIndexName;
_x.underlyingInterestRate = underlyingInterestRate;
_x.underlyingIsin = underlyingIsin;
_x.underlyingIssuerLei = underlyingIssuerLei;
_x.underlyingReferenceEntityType = underlyingReferenceEntityType;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("MifidSubclassSearchResult[");
b.append("Key=");
b.append(this.key);
b.append(", ");
b.append("Description=");
b.append(this.description);
b.append(", ");
b.append("InstrumentType=");
b.append(this.instrumentType);
b.append(", ");
b.append("Status=");
b.append(this.status);
b.append(", ");
b.append("Source=");
b.append(this.source);
b.append(", ");
b.append("Identifier=");
b.append(this.identifier);
b.append(", ");
b.append("IdentifierType=");
b.append(this.identifierType);
b.append(", ");
b.append("UserDefinedIdentifier=");
b.append(this.userDefinedIdentifier);
b.append(", ");
b.append("UserDefinedIdentifier2=");
b.append(this.userDefinedIdentifier2);
b.append(", ");
b.append("UserDefinedIdentifier3=");
b.append(this.userDefinedIdentifier3);
b.append(", ");
b.append("UserDefinedIdentifier4=");
b.append(this.userDefinedIdentifier4);
b.append(", ");
b.append("UserDefinedIdentifier5=");
b.append(this.userDefinedIdentifier5);
b.append(", ");
b.append("UserDefinedIdentifier6=");
b.append(this.userDefinedIdentifier6);
b.append(", ");
b.append("AssetClassCode=");
b.append(this.assetClassCode);
b.append(", ");
b.append("AssetClassDescription=");
b.append(this.assetClassDescription);
b.append(", ");
b.append("SubAssetClassCode=");
b.append(this.subAssetClassCode);
b.append(", ");
b.append("SubAssetClassDescription=");
b.append(this.subAssetClassDescription);
b.append(", ");
b.append("SubAssetClassPermId=");
b.append(this.subAssetClassPermId);
b.append(", ");
b.append("SubClassPermId=");
b.append(this.subClassPermId);
b.append(", ");
b.append("CdsSubClass=");
b.append(this.cdsSubClass);
b.append(", ");
b.append("DeliveryCashSettleLocation=");
b.append(this.deliveryCashSettleLocation);
b.append(", ");
b.append("FreightType=");
b.append(this.freightType);
b.append(", ");
b.append("FreightSubType=");
b.append(this.freightSubType);
b.append(", ");
b.append("FreightSize=");
b.append(this.freightSize);
b.append(", ");
b.append("LoadType=");
b.append(this.loadType);
b.append(", ");
b.append("MifidContractType=");
b.append(this.mifidContractType);
b.append(", ");
b.append("MifidLiquidityFlagEsma=");
b.append(this.mifidLiquidityFlagEsma);
b.append(", ");
b.append("ThresholdEffectiveDate=");
b.append(this.thresholdEffectiveDate);
b.append(", ");
b.append("ThresholdEndDate=");
b.append(this.thresholdEndDate);
b.append(", ");
b.append("NotionalCurrency=");
b.append(this.notionalCurrency);
b.append(", ");
b.append("NotionalCurrencyPair=");
b.append(this.notionalCurrencyPair);
b.append(", ");
b.append("Parameter=");
b.append(this.parameter);
b.append(", ");
b.append("PostTradeLisThresholdValue=");
b.append(this.postTradeLisThresholdValue);
b.append(", ");
b.append("PostTradeSstiThresholdValue=");
b.append(this.postTradeSstiThresholdValue);
b.append(", ");
b.append("PreTradeLisThresholdValue=");
b.append(this.preTradeLisThresholdValue);
b.append(", ");
b.append("PreTradeSstiThresholdValue=");
b.append(this.preTradeSstiThresholdValue);
b.append(", ");
b.append("SettlementType=");
b.append(this.settlementType);
b.append(", ");
b.append("SpecificRouteOrTimeCharterAverage=");
b.append(this.specificRouteOrTimeCharterAverage);
b.append(", ");
b.append("UnderlyingAssetType=");
b.append(this.underlyingAssetType);
b.append(", ");
b.append("UnderlyingCommodity=");
b.append(this.underlyingCommodity);
b.append(", ");
b.append("TermOfTheUnderlyingInterestRate=");
b.append(this.termOfTheUnderlyingInterestRate);
b.append(", ");
b.append("TermOfTheUnderlyingBond=");
b.append(this.termOfTheUnderlyingBond);
b.append(", ");
b.append("TimeToMaturityBucket=");
b.append(this.timeToMaturityBucket);
b.append(", ");
b.append("TimeToMaturityBucketOfTheOption=");
b.append(this.timeToMaturityBucketOfTheOption);
b.append(", ");
b.append("MifidUnderlyingIndexName=");
b.append(this.mifidUnderlyingIndexName);
b.append(", ");
b.append("UnderlyingInterestRate=");
b.append(this.underlyingInterestRate);
b.append(", ");
b.append("UnderlyingIsin=");
b.append(this.underlyingIsin);
b.append(", ");
b.append("UnderlyingIssuerLei=");
b.append(this.underlyingIssuerLei);
b.append(", ");
b.append("UnderlyingReferenceEntityType=");
b.append(this.underlyingReferenceEntityType);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy