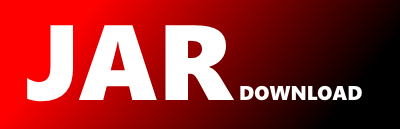
pet.store.schema.NewPet Maven / Gradle / Ivy
The newest version!
package pet.store.schema;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.github.davidmoten.guavamini.Maps;
import jakarta.annotation.Generated;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import org.davidmoten.oa3.codegen.runtime.Preconditions;
import org.davidmoten.oa3.codegen.util.Util;
import pet.store.Globals;
@JsonInclude(Include.NON_ABSENT)
@JsonAutoDetect(
fieldVisibility = JsonAutoDetect.Visibility.ANY,
creatorVisibility = JsonAutoDetect.Visibility.ANY,
setterVisibility = JsonAutoDetect.Visibility.ANY)
@Generated(value = "com.github.davidmoten:openapi-codegen-runtime:0.1.22")
public final class NewPet {
@JsonProperty("name")
private final String name;
@JsonProperty("tag")
private final Optional tag;
@JsonCreator
public NewPet(
@JsonProperty("name") String name,
@JsonProperty("tag") Optional tag) {
if (Globals.config().validateInConstructor().test(NewPet.class)) {
Preconditions.checkNotNull(name, "name");
Preconditions.checkNotNull(tag, "tag");
}
this.name = name;
this.tag = tag;
}
public String name() {
return name;
}
public Optional tag() {
return tag;
}
Map _internal_properties() {
return Maps
.put("name", (Object) name)
.put("tag", (Object) tag)
.build();
}
public NewPet withName(String name) {
return new NewPet(name, tag);
}
public NewPet withTag(Optional tag) {
return new NewPet(name, tag);
}
public NewPet withTag(String tag) {
return new NewPet(name, Optional.of(tag));
}
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String name;
private Optional tag = Optional.empty();
Builder() {
}
public BuilderWithName name(String name) {
this.name = name;
return new BuilderWithName(this);
}
}
public static final class BuilderWithName {
private final Builder b;
BuilderWithName(Builder b) {
this.b = b;
}
public BuilderWithName tag(String tag) {
this.b.tag = Optional.of(tag);
return this;
}
public BuilderWithName tag(Optional tag) {
this.b.tag = tag;
return this;
}
public NewPet build() {
return new NewPet(this.b.name, this.b.tag);
}
}
public static BuilderWithName name(String name) {
return builder().name(name);
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
NewPet other = (NewPet) o;
return
Objects.deepEquals(this.name, other.name) &&
Objects.deepEquals(this.tag, other.tag);
}
@Override
public int hashCode() {
return Objects.hash(name, tag);
}
@Override
public String toString() {
return Util.toString(NewPet.class, "name", name, "tag", tag);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy