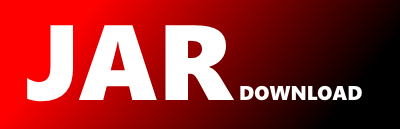
com.github.ddth.commons.utils.SpringUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ddth-commons-core Show documentation
Show all versions of ddth-commons-core Show documentation
DDTH's Java Common Libraries and Utilities
package com.github.ddth.commons.utils;
import java.lang.annotation.Annotation;
import java.util.HashMap;
import java.util.Map;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
/**
* Spring-related utilities.
*
* @author Thanh Ba Nguyen
* @since 0.1.1
*/
public class SpringUtils {
/**
* Gets a bean by its id.
*
* @param appContext
* @param name
* @return
*/
public static Object getSpringBean(ApplicationContext appContext, String id) {
try {
Object bean = appContext.getBean(id);
return bean;
} catch (BeansException e) {
return null;
}
}
/**
* Gets a bean by its class.
*
* @param
* @param appContext
* @param clazz
* @return
*/
public static T getBean(ApplicationContext appContext, Class clazz) {
try {
return appContext.getBean(clazz);
} catch (BeansException e) {
return null;
}
}
/**
* Gets a bean by its id and class.
*
* @param appContext
* @param id
* @param clazz
* @return
*/
public static T getBean(ApplicationContext appContext, String id, Class clazz) {
try {
return appContext.getBean(id, clazz);
} catch (BeansException e) {
return null;
}
}
/**
* Gets all beans of a given type.
*
* @param appContext
* @param clazz
* @return a Map with the matching beans, containing the bean names as keys
* and the corresponding bean instances as values
*/
public static Map getBeansOfType(ApplicationContext appContext, Class clazz) {
try {
return appContext.getBeansOfType(clazz);
} catch (BeansException e) {
return new HashMap();
}
}
/**
* Gets all beans whose Class has the supplied Annotation type.
*
* @param appContext
* @param annotationType
* @return a Map with the matching beans, containing the bean names as keys
* and the corresponding bean instances as values
*/
public static Map getBeansWithAnnotation(ApplicationContext appContext,
Class extends Annotation> annotationType) {
try {
return appContext.getBeansWithAnnotation(annotationType);
} catch (BeansException e) {
return new HashMap();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy