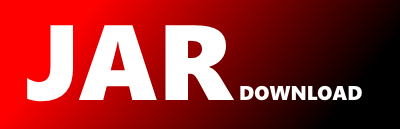
com.github.ddth.commons.utils.ValueUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ddth-commons-core Show documentation
Show all versions of ddth-commons-core Show documentation
DDTH's Java Common Libraries and Utilities
package com.github.ddth.commons.utils;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.fasterxml.jackson.databind.node.MissingNode;
import com.fasterxml.jackson.databind.node.NullNode;
import com.fasterxml.jackson.databind.node.NumericNode;
/**
* Utility class to convert values.
*
* @author Thanh Nguyen
* @since 0.6.1
*/
public class ValueUtils {
/**
* Convert a target object to a specified number type.
*
*
* Nullable:
*
* - If {@code clazz} is a concrete numeric class (e.g. {@link Integer} or {@code int}, this
* method will not return {@code null}. If {@code target} can not be converted to {@code clazz},
* {@code zero} will be return.
* - If {@code clazz} is {@link Number}, {@code null} will be returned if {@code Target} can
* not be converted to {@link Number}.
*
*
*
* @param target
* @param clazz
* one of {@link Number}, {@link Byte}, {@link byte},
* {@link Short}, {@link short}, {@link Integer}, {@link int},
* {@link Long}, {@link long}, {@link Float}, {@link float},
* {@link Double}, {@link double}, {@link BigInteger} or
* {@link BigDecimal}
* @return
*/
@SuppressWarnings("unchecked")
public static N convertNumber(Object target, Class clazz) throws NumberFormatException {
if (clazz == Number.class) {
return target instanceof Number ? (N) target : null;
}
if (clazz == Byte.class || clazz == byte.class) {
byte value = target instanceof Number ? ((Number) target).byteValue()
: target instanceof String ? Byte.parseByte(target.toString()) : 0;
return (N) Byte.valueOf(value);
}
if (clazz == Short.class || clazz == short.class) {
short value = target instanceof Number ? ((Number) target).shortValue()
: target instanceof String ? Short.parseShort(target.toString()) : 0;
return (N) Short.valueOf(value);
}
if (clazz == Integer.class || clazz == int.class) {
int value = target instanceof Number ? ((Number) target).intValue()
: target instanceof String ? Integer.parseInt(target.toString()) : 0;
return (N) Integer.valueOf(value);
}
if (clazz == Long.class || clazz == long.class) {
long value = target instanceof Number ? ((Number) target).longValue()
: target instanceof String ? Long.parseLong(target.toString()) : 0;
return (N) Long.valueOf(value);
}
if (clazz == Float.class || clazz == float.class) {
float value = target instanceof Number ? ((Number) target).floatValue()
: target instanceof String ? Float.parseFloat(target.toString()) : 0;
return (N) Float.valueOf(value);
}
if (clazz == Double.class || clazz == double.class) {
double value = target instanceof Number ? ((Number) target).doubleValue()
: target instanceof String ? Double.parseDouble(target.toString()) : 0;
return (N) Double.valueOf(value);
}
if (clazz == BigInteger.class) {
BigInteger value = target instanceof BigInteger ? (BigInteger) target
: target instanceof Number ? BigInteger.valueOf(((Number) target).longValue())
: target instanceof String
? BigInteger.valueOf(Long.parseLong(target.toString()))
: BigInteger.ZERO;
return (N) value;
}
if (clazz == BigDecimal.class) {
BigDecimal value = target instanceof BigDecimal ? (BigDecimal) target
: target instanceof Number ? BigDecimal.valueOf(((Number) target).doubleValue())
: target instanceof String
? BigDecimal.valueOf(Double.parseDouble(target.toString()))
: BigDecimal.ZERO;
return (N) value;
}
return null;
}
/**
* Convert a target object to {@link Boolean}.
*
*
* Nullable: this method does not return {@code null}. If {@code target} can not be converted to
* {@Link Boolean}, {@code false} is returned.
*
*
* @param target
* @return
*/
public static Boolean convertBoolean(Object target) {
return target instanceof Boolean ? (Boolean) target
: target instanceof String ? Boolean.parseBoolean(target.toString())
: Boolean.FALSE;
}
/**
* Convert a target object to {@link Character}.
*
*
* Nullable: this method does not return {@code null}. If {@code target} can not be converted to
* {@Link Character}, character {@code #0} is returned.
*
*
* @param target
* @return
*/
public static Character convertChar(Object target) {
return target instanceof Character ? (Character) target
: target instanceof Number ? (char) ((Number) target).intValue()
: target instanceof String
? target.toString().length() > 0 ? target.toString().charAt(0) : 0
: 0;
}
/**
* Convert a target object to {@link Date}.
*
* @param target
* @return
*/
public static Date convertDate(Object target) {
DateFormat df = new SimpleDateFormat();
try {
return target instanceof Date ? (Date) target
: target instanceof Number ? new Date(((Number) target).longValue())
: target instanceof String ? df.parse(target.toString()) : null;
} catch (ParseException e) {
return null;
}
}
/**
* Convert a target object to {@link Date}. If the target object is a string, parse it as a
* {@link Date} using the specified date-time format.
*
* @param target
* @param dateTimeFormat
* @return
* @since 0.6.3.1
*/
public static Date convertDate(Object target, String dateTimeFormat) {
return target instanceof Number ? new Date(((Number) target).longValue())
: (target instanceof String
? DateFormatUtils.fromString(target.toString(), dateTimeFormat)
: (target instanceof Date ? (Date) target : null));
}
/**
* Convert a target object to {@link List}.
*
* @param target
* @return
*/
public static List> convertArrayOrList(Object target) {
return target instanceof Object[] ? Arrays.asList((Object[]) target)
: target instanceof Collection ? new ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy