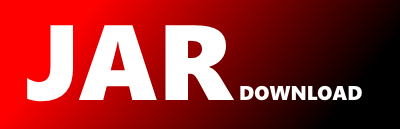
com.github.ddth.queue.IQueue Maven / Gradle / Ivy
Show all versions of ddth-queue-core Show documentation
package com.github.ddth.queue;
import java.util.Collection;
/**
* APIs to interact with queue.
*
*
* Queue implementation flow:
*
* - Call {@link #take()} to get a {@link IQueueMessage} from queue.
* - Do something with the message.
*
* - If done with the message, call {@link #finish(IQueueMessage)}.
* - Otherwise, call {@link #requeue(IQueueMessage)} or
* {@link #requeueSilent(IQueueMessage)} to requeue the message.
*
*
*
*
*
* @author Thanh Ba Nguyen
* @since 0.1.0
*/
public interface IQueue {
/**
* Queues a message.
*
*
* Implementation flow:
*
* - Put message to tail of queue storage.
*
*
*
* @param msg
* @return
*/
public boolean queue(IQueueMessage msg);
/**
* Re-queues a message.
*
*
* Implementation flow:
*
* - Put message to tail of queue storage; and increase message's re-queue
* count & update message's queue timestamp.
* - Remove message from ephemeral storage.
*
*
*
*
* Note: ephemeral storage implementation is optional, depends on
* implementation.
*
*
* @param msg
* @return
*/
public boolean requeue(IQueueMessage msg);
/**
* Silently re-queues a message.
*
*
* Implementation flow:
*
* - Put message to tail of queue storage; do NOT increase message's
* re-queue count and do NOT update message's queue timestamp.
* - Remove message from ephemeral storage.
*
*
*
*
* Note: ephemeral storage implementation is optional, depends on
* implementation.
*
*
* @param msg
* @return
*/
public boolean requeueSilent(IQueueMessage msg);
/**
* Called when finish processing the message to cleanup ephemeral storage.
*
*
* Implementation flow:
*
* - Remove message from ephemeral storage.
*
*
*
*
* Note: ephemeral storage implementation is optional, depends on
* implementation.
*
*
* @param msg
*/
public void finish(IQueueMessage msg);
/**
* Takes a message out of queue.
*
*
* Implementation flow:
*
* - Read message from head of queue storage.
* - Write message to ephemeral storage.
* - Remove message from queue storage.
*
*
*
*
* Note: ephemeral storage implementation is optional, depends on
* implementation.
*
*
* @return
*/
public IQueueMessage take();
/**
* Gets all orphan messages (messages that were left in ephemeral storage
* for a long time).
*
* @param thresholdTimestampMs
* get all orphan messages that were queued
* before this timestamp
* @return
* @since 0.2.0
*/
public Collection getOrphanMessages(long thresholdTimestampMs);
/**
* Moves a message from ephemeral back to queue storage. Useful when dealing
* with orphan messages.
*
*
* Implementation flow:
*
* - Read message from the ephemeral storage.
* - Put the message back to queue.
* - Remove the message from the ephemeral storage.
*
*
*
* @param msg
* @return {@code true} if a move has been made, {@code false} otherwise
* (e.g. the message didn't exist in ephemeral storage)
* @since 0.2.1
*/
public boolean moveFromEphemeralToQueueStorage(IQueueMessage msg);
/**
* Gets queue's number of items.
*
* @return
*/
public int queueSize();
/**
* Gets ephemeral-storage's number of items.
*
*
* Note: ephemeral storage implementation is optional, depends on
* implementation.
*
*
* @return
*/
public int ephemeralSize();
}