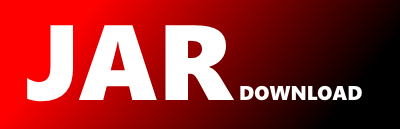
com.github.ddth.queue.impl.InmemQueue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ddth-queue-core Show documentation
Show all versions of ddth-queue-core Show documentation
DDTH's Libary to interact with various queue implementations
package com.github.ddth.queue.impl;
import java.io.Closeable;
import java.util.Collection;
import java.util.Date;
import java.util.HashSet;
import java.util.Map.Entry;
import java.util.Queue;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.concurrent.ConcurrentMap;
import java.util.concurrent.LinkedBlockingQueue;
import com.github.ddth.queue.IQueue;
import com.github.ddth.queue.IQueueMessage;
/**
* In-Memory implementation of {@link IQueue}.
*
*
* Implementation:
*
* - A {@link Queue} as queue storage.
* - A {@link ConcurrentMap} as ephemeral storage.
*
*
*
* @author Thanh Ba Nguyen
* @since 0.4.0
*/
public class InmemQueue implements IQueue, Closeable, AutoCloseable {
private Queue queue;
private ConcurrentMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy