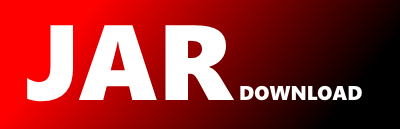
com.github.ddth.queue.jclient.impl.ThriftQueueClientFactory Maven / Gradle / Ivy
package com.github.ddth.queue.jclient.impl;
import java.util.concurrent.ExecutionException;
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import com.google.common.cache.RemovalListener;
import com.google.common.cache.RemovalNotification;
/**
* Factory to create {@link ThriftQueueClient}.
*
* @author Thanh Nguyen
* @since 0.1.0
*/
public class ThriftQueueClientFactory {
private static LoadingCache cache = CacheBuilder.newBuilder()
.removalListener(new RemovalListener() {
@Override
public void onRemoval(RemovalNotification notification) {
notification.getValue().destroy();
}
}).build(new CacheLoader() {
@Override
public ThriftQueueClient load(String hostsAndPorts) throws Exception {
ThriftQueueClient queueClient = new ThriftQueueClient(hostsAndPorts);
queueClient.init();
return queueClient;
}
});
public static void cleanup() {
cache.invalidateAll();
}
/**
* Helper method to create a new {@link ThriftQueueClient} instance.
*
* @param hostsAndPorts
* format {@code host1:port1,host2:port2,host3:port3...}
* @return
*/
public static ThriftQueueClient newQueueClient(String hostAndPort) {
try {
return cache.get(hostAndPort);
} catch (ExecutionException e) {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy