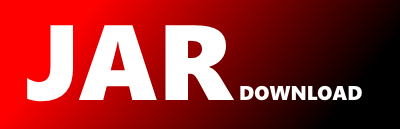
com.github.deansquirrel.tools.poi.WorkBookTool Maven / Gradle / Ivy
package com.github.deansquirrel.tools.poi;
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.streaming.SXSSFSheet;
import org.apache.poi.xssf.streaming.SXSSFWorkbook;
import org.apache.poi.xssf.usermodel.XSSFRichTextString;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileOutputStream;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.List;
public class WorkBookTool {
private WorkBookTool(){};
/**
* 获取字体
* @param book 表格对象
* @return 字体
*/
public static Font getFont(Workbook book) {
if(book == null) {
return null;
}
Font font = book.createFont();
font.setFontName("Calibri");
font.setBold(false);
font.setFontHeightInPoints((short) 11);
return font;
}
/**
* 获取日期时间类型的单元格样式
* @param book 表格对象
* @param font 字体文件
* @return 单元格样式
*/
public static CellStyle getDateTimeCellStyle(Workbook book, Font font) {
if(book == null) {
return null;
}
CreationHelper creationHelper = book.getCreationHelper();
CellStyle cellStyle = book.createCellStyle();
cellStyle.setDataFormat(creationHelper.createDataFormat().getFormat("yyyy-mm-dd hh:mm:ss"));
if(font != null) {
cellStyle.setFont(font);
}
return cellStyle;
}
/**
* 获取日期时间类型的单元格样式
* @param book 表格对象
* @return 单元格样式
*/
public static CellStyle getDateTimeCellStyle(Workbook book) {
return getDateTimeCellStyle(book, getFont(book));
}
/**
* 获取日期类型的单元格样式
* @param book 表格对象
* @param font 字体
* @return 单元格样式
*/
public static CellStyle getDateCellStyle(Workbook book, Font font) {
if(book == null) {
return null;
}
CreationHelper creationHelper = book.getCreationHelper();
CellStyle cellStyle = book.createCellStyle();
cellStyle.setDataFormat(creationHelper.createDataFormat().getFormat("yyyy-mm-dd"));
if(font != null) {
cellStyle.setFont(font);
}
return cellStyle;
}
/**
* 获取日期类型的单元格样式
* @param book 表格对象
* @return 单元格样式
*/
public static CellStyle getDateCellStyle(Workbook book) {
if(book == null) {
return null;
}
return getDateCellStyle(book, getFont(book));
}
/**
* 获取单元格样式
* @param book 表格对象
* @param font 字体
* @return 单元格样式
*/
public static CellStyle getCellStyle(Workbook book, Font font) {
if(book == null) {
return null;
}
CellStyle cellStyle = book.createCellStyle();
cellStyle.setFont(font);
return cellStyle;
}
/**
* 获取单元格样式
* @param book 表格对象
* @return 单元格样式
*/
public static CellStyle getCellStyle(Workbook book) {
if(book == null) {
return null;
}
CellStyle cellStyle = book.createCellStyle();
cellStyle.setFont(getFont(book));
return cellStyle;
}
private static final SimpleDateFormat checkHMS = new SimpleDateFormat("HHmmss");
private static final String DATE_ZERO = "000000";
private static CellStyle getCellStyle(Object d,
CellStyle dateStyle,
CellStyle dateTimeStyle,
CellStyle cellStyle) {
if(d == null) {
return cellStyle;
}
if(d instanceof Date) {
return DATE_ZERO.equals(checkHMS.format(d)) ? dateStyle : dateTimeStyle;
} else {
return cellStyle;
}
}
private static void createRow(Sheet sheet, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy