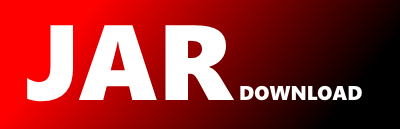
com.github.dekobon.config.MapConfigContext Maven / Gradle / Ivy
package com.github.dekobon.config;
import com.github.dekobon.CloudApiUtils;
import java.util.Map;
import static com.github.dekobon.config.EnvVarConfigContext.*;
/**
* {@link ConfigContext} implementation that is used for configuring instances
* from a Map.
*
* @author Elijah Zupancic
*/
public class MapConfigContext implements ConfigContext {
/**
* Property key for looking up a CloudAPI URL.
*/
public static final String URL_KEY = "triton.url";
/**
* Property key for looking up a CloudAPI account.
*/
public static final String USER_KEY = "triton.user";
/**
* Property key for looking up a RSA fingerprint.
*/
public static final String KEY_ID_KEY = "triton.key_id";
/**
* Property key for looking up a RSA private key path.
*/
public static final String KEY_PATH_KEY = "triton.key_path";
/**
* Property key for looking up a CloudAPI timeout.
*/
public static final String TIMEOUT_KEY = "triton.timeout";
/**
* Property key for number of times to retry failed requests.
*/
public static final String RETRIES_KEY = "triton.retries";
/**
* Property key for the maximum number of open connections to the CloudAPI API.
*/
public static final String MAX_CONNS_KEY = "triton.max_connections";
/**
* Property key for looking up CloudAPI private key content.
*/
public static final String PRIVATE_KEY_CONTENT_KEY = "triton.key_content";
/**
* Property key for looking up CloudAPI password.
*/
public static final String PASSWORD_KEY = "triton.password";
/**
* Property key for setting HttpTransport implementation.
*/
public static final String HTTP_TRANSPORT_KEY = "triton.http_transport";
/**
* Property key for setting TLS protocols.
*/
public static final String HTTPS_PROTOCOLS_KEY = "https.protocols";
/**
* Property key for setting TLS ciphers.
*/
public static final String HTTPS_CIPHERS_KEY = "https.cipherSuites";
/**
* Property key for disabling HTTP signatures.
*/
public static final String NO_AUTH_KEY = "triton.no_auth";
/**
* Property key for disabling native code support for generating signatures.
*/
public static final String NO_NATIVE_SIGS_KEY = "triton.disable_native_sigs";
/**
* Property key for looking up the time in milliseconds to cache HTTP signature headers.
*/
public static final String SIGS_CACHE_TTL_KEY = "http.signature.cache.ttl";
// I know manually adding them all sucks, but it is the simplest operation
// for a shared library. We could do all sorts of complicated reflection
// or annotation processing, but they are error-prone.
/**
* List of all properties that we read from configuration.
*/
public static final String[] ALL_PROPERTIES = {
URL_KEY, USER_KEY, KEY_ID_KEY,
KEY_PATH_KEY, TIMEOUT_KEY, RETRIES_KEY,
MAX_CONNS_KEY, PRIVATE_KEY_CONTENT_KEY,
PASSWORD_KEY, HTTP_TRANSPORT_KEY,
HTTPS_PROTOCOLS_ENV_KEY, HTTPS_CIPHERS_KEY,
NO_AUTH_KEY, NO_NATIVE_SIGS_KEY,
SIGS_CACHE_TTL_KEY
};
/**
* Internal map used as the source of the configuration bean values.
*/
private final Map, ?> backingMap;
/**
* Creates a new instance using the passed {@link Map} implementation as
* a backing store.
*
* @param backingMap Map implementation used for the values of the configuration beans
*/
public MapConfigContext(final Map, ?> backingMap) {
this.backingMap = backingMap;
}
@Override
public String getCloudAPIURL() {
return normalizeEmptyAndNullAndDefaultToStringValue(
URL_KEY, TRITON_URL_ENV_KEY);
}
@Override
public String getUser() {
return normalizeEmptyAndNullAndDefaultToStringValue(
USER_KEY, TRITON_USER_ENV_KEY);
}
@Override
public String getKeyId() {
return normalizeEmptyAndNullAndDefaultToStringValue(
KEY_ID_KEY, TRITON_KEY_ID_ENV_KEY);
}
@Override
public String getKeyPath() {
return normalizeEmptyAndNullAndDefaultToStringValue(
KEY_PATH_KEY, TRITON_KEY_PATH_ENV_KEY);
}
@Override
public String getPrivateKeyContent() {
return normalizeEmptyAndNullAndDefaultToStringValue(
PRIVATE_KEY_CONTENT_KEY, PRIVATE_KEY_CONTENT_ENV_KEY);
}
@Override
public String getPassword() {
return normalizeEmptyAndNullAndDefaultToStringValue(
PASSWORD_KEY, PASSWORD_ENV_KEY);
}
@Override
public Integer getTimeout() {
Integer mapValue = CloudApiUtils.parseIntegerOrNull(backingMap.get(TIMEOUT_KEY));
if (mapValue != null) {
return mapValue;
}
return CloudApiUtils.parseIntegerOrNull(backingMap.get(TIMEOUT_ENV_KEY));
}
@Override
public Integer getRetries() {
Integer mapValue = CloudApiUtils.parseIntegerOrNull(backingMap.get(RETRIES_KEY));
if (mapValue != null) {
return mapValue;
}
return CloudApiUtils.parseIntegerOrNull(backingMap.get(RETRIES_ENV_KEY));
}
@Override
public String getHttpsProtocols() {
return normalizeEmptyAndNullAndDefaultToStringValue(
HTTPS_PROTOCOLS_KEY, HTTPS_PROTOCOLS_ENV_KEY);
}
@Override
public String getHttpsCipherSuites() {
return normalizeEmptyAndNullAndDefaultToStringValue(
HTTPS_CIPHERS_KEY, HTTPS_CIPHERS_ENV_KEY);
}
@Override
public Boolean disableNativeSignatures() {
Boolean mapValue = CloudApiUtils.parseBooleanOrNull(backingMap.get(NO_NATIVE_SIGS_KEY));
if (mapValue != null) {
return mapValue;
}
return CloudApiUtils.parseBooleanOrNull(backingMap.get(NO_NATIVE_SIGS_ENV_KEY));
}
/**
* Allows the caller to perform a put operation on the backing map of the
* context. This is typically used by other {@link ConfigContext}
* implementations that need to cobble together multiple map values.
*
* This method is scoped to default because no other packages should be
* using it.
*
* @param key configuration key
* @param value configuration value
* @return return value of the put() operation from the backing map
*/
Object put(final String key, final String value) {
if (key == null) {
throw new IllegalArgumentException("Config key can't be null");
}
if (key.isEmpty()) {
throw new IllegalArgumentException("Config key can't be blank");
}
// Java generics can be stupid
@SuppressWarnings("unchecked")
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy