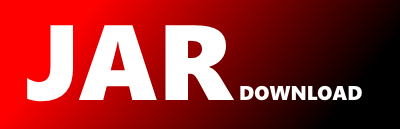
com.github.dekobon.domain.Instance Maven / Gradle / Ivy
package com.github.dekobon.domain;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.github.dekobon.CloudApiUtils;
import org.threeten.bp.Instant;
import java.net.InetAddress;
import java.util.*;
/**
* Domain object representing an instance of compute.
*
* @author Elijah Zupancic
* @since 1.0.0
*/
public class Instance implements Taggable, MetadataEnabled {
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private UUID id;
private String name;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private String brand;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private String state;
private UUID image;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private Set ips;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private long memory;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private long disk;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private Map metadata;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private Map tags;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private Instant created;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private Instant updated;
private Set networks;
@JsonProperty(access = JsonProperty.Access.WRITE_ONLY)
private InetAddress primaryIp;
@JsonProperty("firewall_enabled")
private boolean firewallEnabled;
@JsonProperty(value = "compute_node", access = JsonProperty.Access.WRITE_ONLY)
private UUID computeNode;
@JsonProperty(value = "package", access = JsonProperty.Access.WRITE_ONLY)
private String packageName;
@JsonProperty(value = "dns_names", access = JsonProperty.Access.WRITE_ONLY)
private Set dnsNames;
// --- Used in serialization only
@JsonProperty(access = JsonProperty.Access.READ_ONLY)
private Locality locality;
@JsonProperty(value = "package", access = JsonProperty.Access.READ_ONLY)
private UUID packageId;
public Instance() {
}
public Set privateIPs() {
if (getIps() == null) {
return null;
}
if (getIps().isEmpty()) {
return Collections.emptySet();
}
final Set privateIPs = new LinkedHashSet<>(getIps().size());
for (InetAddress ip : getIps()) {
if (ip.isAnyLocalAddress() || ip.isLoopbackAddress() ||
ip.isLinkLocalAddress() || ip.isSiteLocalAddress()) {
privateIPs.add(ip);
}
}
return Collections.unmodifiableSet(privateIPs);
}
public Map asMap() {
final Map attributes = new LinkedHashMap<>();
if (getBrand() != null) {
attributes.put("brand", getBrand());
}
if (getComputeNode() != null) {
attributes.put("compute_node", getComputeNode());
}
if (getCreated() != null) {
attributes.put("created", getCreated());
}
if (getDnsNames() != null) {
attributes.put("dns_name", getDnsNames());
}
if (getId() != null) {
attributes.put("id", getId());
}
if (getImage() != null) {
attributes.put("image", getImage());
}
if (getIps() != null) {
attributes.put("ips", getIps());
}
if (getLocality() != null) {
attributes.put("locality", getLocality());
}
if (getMetadata() != null) {
attributes.put("metadata", getMetadata());
}
if (getName() != null) {
attributes.put("name", getName());
}
if (getNetworks() != null) {
attributes.put("networks", getNetworks());
}
if (getPackageId() != null) {
attributes.put("package", getPackageId());
}
if (getPackageName() != null) {
attributes.put("package_name", getPackageName());
}
if (getPrimaryIp() != null) {
attributes.put("primary_ip", getPrimaryIp());
}
if (getState() != null) {
attributes.put("state", getState());
}
if (getTags() != null) {
attributes.put("tags", getTags());
}
if (getUpdated() != null) {
attributes.put("updated", getUpdated());
}
attributes.put("disk", getDisk());
attributes.put("memory", getMemory());
return Collections.unmodifiableMap(attributes);
}
public UUID getId() {
return id;
}
Instance setId(final UUID id) {
this.id = id;
return this;
}
public String getName() {
return name;
}
public Instance setName(String name) {
this.name = name;
return this;
}
public String getBrand() {
return brand;
}
Instance setBrand(String brand) {
this.brand = brand;
return this;
}
public String getState() {
return state;
}
Instance setState(String state) {
this.state = state;
return this;
}
public UUID getImage() {
return image;
}
public Instance setImage(UUID image) {
this.image = image;
return this;
}
public Set getIps() {
return ips;
}
Instance setIps(Set ips) {
this.ips = ips;
return this;
}
public long getMemory() {
return memory;
}
Instance setMemory(long memory) {
this.memory = memory;
return this;
}
public long getDisk() {
return disk;
}
Instance setDisk(long disk) {
this.disk = disk;
return this;
}
@Override
public Map getMetadata() {
return metadata;
}
@Override
public Instance setMetadata(Map metadata) {
this.metadata = metadata;
return this;
}
@Override
public Map getTags() {
return tags;
}
@Override
public Instance setTags(Map tags) {
this.tags = tags;
return this;
}
public Instant getCreated() {
return created;
}
Instance setCreated(Instant created) {
this.created = created;
return this;
}
public Instant getUpdated() {
return updated;
}
Instance setUpdated(Instant updated) {
this.updated = updated;
return this;
}
public Set getNetworks() {
return networks;
}
public Instance setNetworks(Set networks) {
this.networks = networks;
return this;
}
public InetAddress getPrimaryIp() {
return primaryIp;
}
Instance setPrimaryIp(InetAddress primaryIp) {
this.primaryIp = primaryIp;
return this;
}
public boolean isFirewallEnabled() {
return firewallEnabled;
}
public Instance setFirewallEnabled(boolean firewallEnabled) {
this.firewallEnabled = firewallEnabled;
return this;
}
public UUID getComputeNode() {
return computeNode;
}
Instance setComputeNode(UUID computeNode) {
this.computeNode = computeNode;
return this;
}
public String getPackageName() {
return packageName;
}
Instance setPackageName(String packageName) {
this.packageName = packageName;
return this;
}
public UUID getPackageId() {
return packageId;
}
public Instance setPackageId(UUID packageId) {
this.packageId = packageId;
return this;
}
public Set getDnsNames() {
return dnsNames;
}
Instance setDnsNames(Set dnsNames) {
this.dnsNames = dnsNames;
return this;
}
public Locality getLocality() {
return locality;
}
public Instance setLocality(Locality locality) {
this.locality = locality;
return this;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Instance instance = (Instance) o;
return memory == instance.memory &&
disk == instance.disk &&
firewallEnabled == instance.firewallEnabled &&
Objects.equals(id, instance.id) &&
Objects.equals(name, instance.name) &&
Objects.equals(brand, instance.brand) &&
Objects.equals(state, instance.state) &&
Objects.equals(image, instance.image) &&
Objects.equals(ips, instance.ips) &&
Objects.equals(metadata, instance.metadata) &&
Objects.equals(tags, instance.tags) &&
Objects.equals(created, instance.created) &&
Objects.equals(updated, instance.updated) &&
Objects.equals(networks, instance.networks) &&
Objects.equals(primaryIp, instance.primaryIp) &&
Objects.equals(computeNode, instance.computeNode) &&
Objects.equals(packageName, instance.packageName) &&
Objects.equals(dnsNames, instance.dnsNames) &&
Objects.equals(locality, instance.locality);
}
@Override
public int hashCode() {
return Objects.hash(id, name, brand, state, image, ips, memory,
disk, metadata, tags, created, updated, networks, primaryIp,
firewallEnabled, computeNode, packageName, dnsNames, locality);
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder("Machine{");
sb.append("id=").append(id);
sb.append(", name='").append(name).append('\'');
sb.append(", brand='").append(brand).append('\'');
sb.append(", state='").append(state).append('\'');
sb.append(", image=").append(image);
sb.append(", ips=").append(ips);
sb.append(", memory=").append(memory);
sb.append(", disk=").append(disk);
sb.append(", metadata=").append(metadata);
sb.append(", tags=").append(tags);
sb.append(", created=").append(created);
sb.append(", updated=").append(updated);
sb.append(", networks=").append(networks);
sb.append(", primaryIp=").append(primaryIp);
sb.append(", firewallEnabled=").append(firewallEnabled);
sb.append(", computeNode=").append(computeNode);
sb.append(", packageName='").append(packageName).append('\'');
sb.append(", dnsNames=").append(dnsNames);
sb.append(", locality=").append(locality);
sb.append('}');
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy