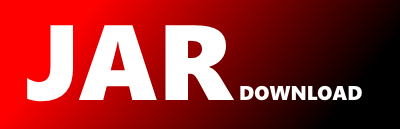
org.beigesoft.ajetty.BootEmbed Maven / Gradle / Ivy
Show all versions of a-jetty-base Show documentation
/*
BSD 2-Clause License
Copyright (c) 2019, Beigesoft™
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package org.beigesoft.ajetty;
import java.io.File;
import java.security.KeyStore;
import org.eclipse.jetty.server.Server;
import org.eclipse.jetty.server.Connector;
import org.eclipse.jetty.server.HttpConfiguration;
import org.eclipse.jetty.server.HttpConnectionFactory;
import org.eclipse.jetty.server.SecureRequestCustomizer;
import org.eclipse.jetty.server.ServerConnector;
import org.eclipse.jetty.webapp.WebAppContext;
import org.eclipse.jetty.server.SslConnectionFactory;
import org.eclipse.jetty.security.DataBaseLoginService;
import org.eclipse.jetty.util.ssl.SslContextFactory;
import org.beigesoft.afactory.IFactoryAppBeans;
/**
*
* BootEmbed for A-Jetty configured as minimum server
* with precompiled together WebApp with WEB-INF/web.xml, index.html, all other
* static files(css, js) that exists on given path, by default path is webapp.
* This is means that all classes A-Jetty
* and web application must be in same jar/dex file.
*
*
* @author Yury Demidenko
*/
public class BootEmbed {
/**
* Factory app-beans - only for WEB-app class loader.
**/
private IFactoryAppBeans factoryAppBeans;
/**
* Port.
**/
private Integer port = 8443;
/**
* Web.
**/
private String webAppPath = "webapp";
/**
* Jetty.
**/
private Server server;
/**
* Webapp context.
**/
private WebAppContext webAppContext;
/**
* Is started.
**/
private boolean isStarted = false;
/**
* A-Jetty start (keystore) password.
**/
private String password;
/**
* Keystore file.
**/
private File pkcs12File;
/**
* A-Jetty start HTTPS alias.
**/
private String httpsAlias;
/**
* A-Jetty start HTTPS alias.
**/
private String httpsPassword;
/**
* Crypto provider name.
**/
private String cryptoProviderName = "BC";
/**
* Key-store.
**/
private KeyStore keyStore;
/**
* A-Jetty instance number.
**/
private Integer ajettyIn;
/**
* Create and configure server.
* @throws Exception an Exception
**/
public final void createServer() throws Exception {
try {
File webappdir = new File(getWebAppPath());
if (!webappdir.exists() || !webappdir.isDirectory()) {
throw new Exception("Web app directory not found: " + getWebAppPath());
}
this.server = new Server();
SslContextFactory sslContextFactory = new SslContextFactory();
sslContextFactory.setKeyStorePath(this.pkcs12File.getAbsolutePath());
sslContextFactory.setKeyStorePassword(this.password);
sslContextFactory.setKeyStoreProvider(this.cryptoProviderName);
sslContextFactory.setKeyStoreType("PKCS12");
if (this.httpsPassword != null) {
sslContextFactory.setKeyManagerPassword(this.httpsPassword);
}
sslContextFactory.setCertAlias(this.httpsAlias);
HttpConfiguration httpsConf = new HttpConfiguration();
httpsConf.setSecureScheme("https");
httpsConf.setSecurePort(this.port);
httpsConf.setOutputBufferSize(32768);
httpsConf.addCustomizer(new SecureRequestCustomizer());
ServerConnector connector = new ServerConnector(server,
new SslConnectionFactory(sslContextFactory, "http/1.1"),
new HttpConnectionFactory(httpsConf));
connector.setHost("127.0.0.1");
connector.setPort(this.port);
connector.setIdleTimeout(500000);
server.setConnectors(new Connector[] {connector});
// without different application context path
// authentication works badly in case of two A-Jetty instance
// with the same web-app context path:
this.webAppContext = new WebAppContext(webappdir
.getAbsolutePath(), "/bsa" + this.port + "/");
DataBaseLoginService dataBaseLoginService =
new DataBaseLoginService("JDBCRealm");
this.webAppContext.getSecurityHandler()
.setLoginService(dataBaseLoginService);
this.webAppContext.setAttribute("JDBCRealm", dataBaseLoginService);
this.webAppContext.setFactoryAppBeans(getFactoryAppBeans());
this.webAppContext.setDefaultsDescriptor(webappdir
.getAbsolutePath() + File.separator + "webdefault.xml");
this.webAppContext.setAttribute("ajettyKeystore", this.keyStore);
this.webAppContext.setAttribute("ajettyIn", this.ajettyIn);
this.webAppContext.setAttribute("ksPassword", this.password);
this.server.setHandler(this.webAppContext);
} catch (Exception e) {
this.server = null;
this.webAppContext = null;
throw e;
}
}
/**
* Start server.
* @throws Exception an Exception
**/
public final void startServer() throws Exception {
try {
if (this.server == null) {
createServer();
}
this.server.start();
this.isStarted = true;
} catch (Exception e) {
this.server = null;
this.webAppContext = null;
}
}
/**
* Stop server.
* @throws Exception an Exception
**/
public final void stopServer() throws Exception {
try {
this.server.stop();
this.server.destroy();
} finally {
this.server = null;
this.webAppContext = null;
this.isStarted = false;
}
}
//Simple getters and setters:
/**
* Getter for port.
* @return Integer
**/
public final Integer getPort() {
return this.port;
}
/**
* Setter for port.
* @param pPort reference
**/
public final void setPort(final Integer pPort) {
this.port = pPort;
}
/**
* Getter for server.
* @return Server
**/
public final Server getServer() {
return this.server;
}
/**
* Getter for isStarted.
* @return boolean
**/
public final boolean getIsStarted() {
return this.isStarted;
}
/**
* Getter for factoryAppBeans.
* @return IFactoryAppBeans
**/
public final IFactoryAppBeans getFactoryAppBeans() {
return this.factoryAppBeans;
}
/**
* Setter for factoryAppBeans.
* @param pFactoryAppBeans reference
**/
public final void setFactoryAppBeans(
final IFactoryAppBeans pFactoryAppBeans) {
this.factoryAppBeans = pFactoryAppBeans;
}
/**
* Getter for webAppPath.
* @return String
**/
public final String getWebAppPath() {
return this.webAppPath;
}
/**
* Setter for webAppPath.
* @param pWebAppPath reference
**/
public final void setWebAppPath(final String pWebAppPath) {
this.webAppPath = pWebAppPath;
}
/**
* Getter for webAppContext.
* @return WebAppContext
**/
public final WebAppContext getWebAppContext() {
return this.webAppContext;
}
/**
* Getter for password.
* @return String
**/
public final String getPassword() {
return this.password;
}
/**
* Setter for password.
* @param pPassword reference
**/
public final void setPassword(final String pPassword) {
this.password = pPassword;
}
/**
* Getter for pkcs12File.
* @return File
**/
public final File getPkcs12File() {
return this.pkcs12File;
}
/**
* Setter for pkcs12File.
* @param pPkcs12File reference
**/
public final void setPkcs12File(final File pPkcs12File) {
this.pkcs12File = pPkcs12File;
}
/**
* Getter for httpsAlias.
* @return String
**/
public final String getHttpsAlias() {
return this.httpsAlias;
}
/**
* Setter for httpsAlias.
* @param pHttpsAlias reference
**/
public final void setHttpsAlias(final String pHttpsAlias) {
this.httpsAlias = pHttpsAlias;
}
/**
* Getter for httpsPassword.
* @return String
**/
public final String getHttpsPassword() {
return this.httpsPassword;
}
/**
* Setter for httpsPassword.
* @param pHttpsPassword reference
**/
public final void setHttpsPassword(final String pHttpsPassword) {
this.httpsPassword = pHttpsPassword;
}
/**
* Getter for cryptoProviderName.
* @return String
**/
public final String getCryptoProviderName() {
return this.cryptoProviderName;
}
/**
* Setter for cryptoProviderName.
* @param pCryptoProviderName reference
**/
public final void setCryptoProviderName(final String pCryptoProviderName) {
this.cryptoProviderName = pCryptoProviderName;
}
/**
* Getter for keyStore.
* @return KeyStore
**/
public final KeyStore getKeyStore() {
return this.keyStore;
}
/**
* Setter for keyStore.
* @param pKeyStore reference
**/
public final void setKeyStore(final KeyStore pKeyStore) {
this.keyStore = pKeyStore;
}
/**
* Getter for ajettyIn.
* @return Integer
**/
public final Integer getAjettyIn() {
return this.ajettyIn;
}
/**
* Setter for ajettyIn.
* @param pAjettyIn reference
**/
public final void setAjettyIn(final Integer pAjettyIn) {
this.ajettyIn = pAjettyIn;
}
}