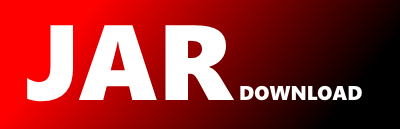
com.github.dennisit.vplus.tutorial.domain.Person Maven / Gradle / Ivy
The newest version!
/*--------------------------------------------------------------------------
* Copyright (c) 2010-2020, Elon.su All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are
* met:
*
* Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* Neither the name of the elon developer nor the names of its
* contributors may be used to endorse or promote products derived from
* this software without specific prior written permission.
* Author: Elon.su, you can also mail [email protected]
*--------------------------------------------------------------------------
*/
package com.github.dennisit.vplus.tutorial.domain;
import com.github.dennisit.vplus.core.anno.html.VHtml;
import com.github.dennisit.vplus.core.anno.table.VField;
import com.github.dennisit.vplus.core.anno.table.VTable;
import java.io.Serializable;
import java.util.Date;
import java.util.HashMap;
@VTable(tableName = "tb_person", comment = "用户表")
public class Person implements Serializable {
@VField(
order = 1, column = "_id", comment = "表主键", primary = true,
vHtml = @VHtml(inList = true, inForm = false, inQuery = true, type = VHtml.Type.TEXT)
)
private Long id;
@VField(
order = 2, column = "_account", comment = "账号",
vHtml = @VHtml(inList = true, inForm = true, inQuery = true, type = VHtml.Type.TEXT)
)
private String account;
@VField(
order = 3, column = "_pass", comment = "密码",
vHtml = @VHtml(inList = true, inForm = true, inQuery = true, type = VHtml.Type.PASSWORD, value = String.class)
)
private String pass;
@VField(
order = 4, column = "_pass", comment = "性别",
vHtml = @VHtml(inList = true, inForm = true, inQuery = true, type = VHtml.Type.RADIO, value = SexEnum.class)
)
private Integer sex;
@VField(
order = 5, column = "_level", comment = "级别",
vHtml = @VHtml(inList = true, inForm = true, inQuery = true, type = VHtml.Type.RADIO)
)
private Integer level;
@VField(
order = 6, column = "_depict", comment = "描述",
vHtml = @VHtml(inList = true, inForm = true, inQuery = false, type = VHtml.Type.TEXTAREA)
)
private String depict;
@VField(
order = 7, column = "create_time", comment = "记录创建时间",
vHtml = @VHtml(inList = true, inForm = false, inQuery = true, type = VHtml.Type.DATETIME)
)
private Date createTime;
@VField(vHtml = @VHtml, abolished = true)
private String extract;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getAccount() {
return account;
}
public void setAccount(String account) {
this.account = account;
}
public String getPass() {
return pass;
}
public void setPass(String pass) {
this.pass = pass;
}
public Integer getSex() {
return sex;
}
public void setSex(Integer sex) {
this.sex = sex;
}
public String getDepict() {
return depict;
}
public void setDepict(String depict) {
this.depict = depict;
}
public Date getCreateTime() {
return createTime;
}
public void setCreateTime(Date createTime) {
this.createTime = createTime;
}
public enum SexEnum {
BOY("男",1), GRIL("女", 2);
private String name;
private int value;
SexEnum(String name, int value) {
this.name = name;
this.value = value;
}
}
public class LevelMap extends HashMap{
private String name;
private int value;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getValue() {
return value;
}
public void setValue(int value) {
this.value = value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy