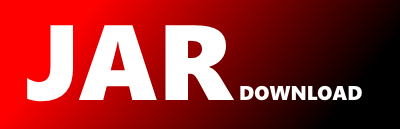
com.github.devnied.emvnfccard.parser.apdu.impl.AbstractByteBean Maven / Gradle / Ivy
package com.github.devnied.emvnfccard.parser.apdu.impl;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.github.devnied.emvnfccard.iso7816emv.ITag;
import com.github.devnied.emvnfccard.iso7816emv.TagAndLength;
import com.github.devnied.emvnfccard.model.AbstractData;
import com.github.devnied.emvnfccard.parser.apdu.IFile;
import com.github.devnied.emvnfccard.parser.apdu.annotation.AnnotationData;
import com.github.devnied.emvnfccard.parser.apdu.annotation.AnnotationUtils;
import fr.devnied.bitlib.BitUtils;
/**
* Abstract class for all object to parse
*
* @author MILLAU Julien
*/
public abstract class AbstractByteBean extends AbstractData implements IFile {
/**
* Generated serial UID
*/
private static final long serialVersionUID = -2016039522844322383L;
/**
* Logger of the class
*/
private static final Logger LOGGER = LoggerFactory.getLogger(AbstractByteBean.class.getName());
/**
* Method to get the annotation set from the current class
*
* @return An annotation set which contain all annotation data
*/
private Collection getAnnotationSet(final List pTags) {
Collection ret = null;
if (pTags != null) {
Map data = AnnotationUtils.getInstance().getMap().get(getClass().getName());
ret = new ArrayList(data.size());
for (TagAndLength tal : pTags) {
AnnotationData ann = data.get(tal.getTag());
if (ann != null) {
ann.setSize(tal.getLength() * BitUtils.BYTE_SIZE);
ret.add(ann);
}
}
} else {
ret = AnnotationUtils.getInstance().getMapSet().get(getClass().getName());
}
return ret;
}
/**
* Method to parse byte data
*
* @param pData
* byte to parse
* @param pTags
*/
@Override
public void parse(final byte[] pData, final List pTags) {
Collection set = getAnnotationSet(pTags);
BitUtils bit = new BitUtils(pData);
Iterator it = set.iterator();
while (it.hasNext()) {
AnnotationData data = it.next();
Object obj = DataFactory.getObject(data, bit);
setField(data.getField(), this, obj);
}
}
/**
* Method used to set the value of a field
*
* @param field
* the field to set
* @param pData
* Object containing the field
* @param pValue
* the value of the field
*/
protected void setField(final Field field, final IFile pData, final Object pValue) {
if (field != null) {
try {
field.set(pData, pValue);
} catch (IllegalArgumentException e) {
LOGGER.error("Parameters of fied.set are not valid", e);
} catch (IllegalAccessException e) {
LOGGER.error("Impossible to set the Field :" + field.getName(), e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy