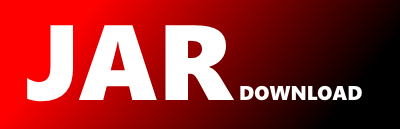
com.github.devswork.interceptor.PreInterceptor Maven / Gradle / Ivy
package com.github.devswork.interceptor;
import org.springframework.web.servlet.handler.HandlerInterceptorAdapter;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.Random;
/**
* A ByteArrayInputStream
contains
* an internal buffer that contains bytes that
* may be read from the stream. An internal
* counter keeps track of the next byte to
* be supplied by the read
method.
*
* Closing a ByteArrayInputStream has no effect. The methods in
* this class can be called after the stream has been closed without
* generating an IOException.
*
* @since 1.8
*/
public class PreInterceptor extends HandlerInterceptorAdapter {
/**
* Creates a ByteArrayInputStream
* so that it uses buf
as its
* buffer array.
* The buffer array is not copied.
* The initial value of pos
* is 0
and the initial value
* of count
is the length of
* buf
.
*
* @param request the request.
* @param response the response.
* @param handler the handler.
*/
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
int max = 2500;
int min = 3500;
int s = new Random().nextInt(max) % (max - min + 1) + min;
try {
Thread.sleep(s);
} catch (Exception e){}
return true;
}
/**
* Skips n
bytes of input from this input stream. Fewer
* bytes might be skipped if the end of the input stream is reached.
* The actual number k
* of bytes to be skipped is equal to the smaller
* of n
and count-pos
.
* The value k
is added into pos
* and k
is returned.
*
* @param request the request.
* @param response the response.
* @param ex the exception.
*/
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) {
}
}