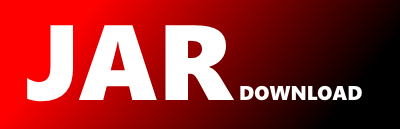
com.github.dnault.xmlpatch.batch.AssembledPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xml-patch Show documentation
Show all versions of xml-patch Show documentation
Java implementation of RFC 5261: An XML Patch Operations Framework Utilizing XPath Selectors
The newest version!
package com.github.dnault.xmlpatch.batch;
import static java.util.Objects.requireNonNull;
import java.io.File;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import org.jdom2.Element;
public class AssembledPatch {
private LinkedHashSet patchFiles = new LinkedHashSet<>();
private List diffs = new ArrayList<>();
private Set accessedPaths = new HashSet<>();
public AssembledPatch() {
}
public AssembledPatch(File patch) throws Exception {
new PatchAssembler().assembleRecursive(patch, this);
}
/**
* @return the set of files that comprise the patch
*/
public LinkedHashSet getPatchFiles() {
return patchFiles;
}
public boolean addPatchFile(File includedFile) {
return this.patchFiles.add(includedFile);
}
public List getDiffs() {
return diffs;
}
public List getDiffs(String sourcePath) {
requireNonNull(sourcePath);
List matchingDiffs = new ArrayList<>(0);
for (Element e : diffs) {
if (sourcePath.equals(e.getAttributeValue("file"))) {
matchingDiffs.add(e);
accessedPaths.add(sourcePath);
}
}
return matchingDiffs;
}
public Set getSourcePaths() {
Set sourcePaths = new HashSet<>();
for (Element e : diffs) {
sourcePaths.add(e.getAttributeValue("file"));
}
return sourcePaths;
}
public Set getAccessedPaths() {
return accessedPaths;
}
public void addDif(Element diff) {
this.diffs.add(diff);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy