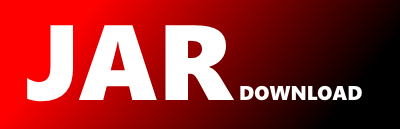
com.github.dockerjava.core.command.RemoveImageCmdImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of docker-java Show documentation
Show all versions of docker-java Show documentation
Java API Client for Docker
package com.github.dockerjava.core.command;
import static com.google.common.base.Preconditions.checkNotNull;
import com.github.dockerjava.api.command.RemoveImageCmd;
import com.github.dockerjava.api.exception.NotFoundException;
/**
*
* Remove an image, deleting any tags it might have.
*
*/
public class RemoveImageCmdImpl extends AbstrDockerCmd implements RemoveImageCmd {
private String imageId;
private Boolean force, noPrune;
public RemoveImageCmdImpl(RemoveImageCmd.Exec exec, String imageId) {
super(exec);
withImageId(imageId);
}
@Override
public String getImageId() {
return imageId;
}
@Override
public Boolean hasForceEnabled() {
return force;
}
@Override
public Boolean hasNoPruneEnabled() {
return noPrune;
}
@Override
public RemoveImageCmd withImageId(String imageId) {
checkNotNull(imageId, "imageId was not specified");
this.imageId = imageId;
return this;
}
@Override
public RemoveImageCmd withForce(Boolean force) {
this.force = force;
return this;
}
@Override
public RemoveImageCmd withNoPrune(Boolean noPrune) {
this.noPrune = noPrune;
return this;
}
/**
* @throws NotFoundException
* No such image
*/
@Override
public Void exec() throws NotFoundException {
return super.exec();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy