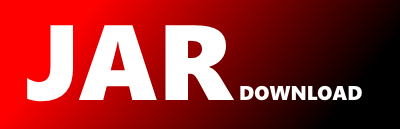
xy.reflect.ui.control.swing.customizer.CustomizationTools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of custom-ui Show documentation
Show all versions of custom-ui Show documentation
Customizations editor for ReflectionUI
The newest version!
package xy.reflect.ui.control.swing.customizer;
import java.awt.Color;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.Image;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.IOException;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import javax.imageio.ImageIO;
import javax.swing.AbstractAction;
import javax.swing.Action;
import javax.swing.JButton;
import javax.swing.JCheckBoxMenuItem;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JPopupMenu;
import xy.reflect.ui.ReflectionUI;
import xy.reflect.ui.control.DefaultFieldControlData;
import xy.reflect.ui.control.IFieldControlData;
import xy.reflect.ui.control.plugin.AbstractSimpleCustomizableFieldControlPlugin.AbstractConfiguration;
import xy.reflect.ui.control.plugin.ICustomizableFieldControlPlugin;
import xy.reflect.ui.control.plugin.IFieldControlPlugin;
import xy.reflect.ui.control.swing.ListControl;
import xy.reflect.ui.control.swing.builder.StandardEditorBuilder;
import xy.reflect.ui.control.swing.plugin.ImageViewPlugin;
import xy.reflect.ui.control.swing.renderer.FieldControlPlaceHolder;
import xy.reflect.ui.control.swing.renderer.Form;
import xy.reflect.ui.control.swing.renderer.MethodControlPlaceHolder;
import xy.reflect.ui.control.swing.util.SwingRendererUtils;
import xy.reflect.ui.info.IInfo;
import xy.reflect.ui.info.custom.InfoCustomizations;
import xy.reflect.ui.info.custom.InfoCustomizations.AbstractCustomization;
import xy.reflect.ui.info.custom.InfoCustomizations.ConversionMethodFinder;
import xy.reflect.ui.info.custom.InfoCustomizations.EnumerationCustomization;
import xy.reflect.ui.info.custom.InfoCustomizations.FieldCustomization;
import xy.reflect.ui.info.custom.InfoCustomizations.FieldTypeSpecificities;
import xy.reflect.ui.info.custom.InfoCustomizations.JavaClassBasedTypeInfoFinder;
import xy.reflect.ui.info.custom.InfoCustomizations.ListCustomization;
import xy.reflect.ui.info.custom.InfoCustomizations.Mapping;
import xy.reflect.ui.info.custom.InfoCustomizations.MethodCustomization;
import xy.reflect.ui.info.custom.InfoCustomizations.TextualStorage;
import xy.reflect.ui.info.custom.InfoCustomizations.TypeConversion;
import xy.reflect.ui.info.custom.InfoCustomizations.TypeCustomization;
import xy.reflect.ui.info.custom.InfoCustomizations.VirtualFieldDeclaration;
import xy.reflect.ui.info.field.CapsuleFieldInfo;
import xy.reflect.ui.info.field.IFieldInfo;
import xy.reflect.ui.info.method.DefaultConstructorInfo;
import xy.reflect.ui.info.method.DefaultMethodInfo;
import xy.reflect.ui.info.method.IMethodInfo;
import xy.reflect.ui.info.parameter.IParameterInfo;
import xy.reflect.ui.info.type.ITypeInfo;
import xy.reflect.ui.info.type.enumeration.IEnumerationItemInfo;
import xy.reflect.ui.info.type.enumeration.IEnumerationTypeInfo;
import xy.reflect.ui.info.type.factory.InfoCustomizationsFactory;
import xy.reflect.ui.info.type.iterable.IListTypeInfo;
import xy.reflect.ui.info.type.iterable.item.ItemPosition;
import xy.reflect.ui.info.type.iterable.structure.CustomizedListStructuralInfo.SubListGroupField;
import xy.reflect.ui.info.type.iterable.structure.IListStructuralInfo;
import xy.reflect.ui.info.type.iterable.structure.column.IColumnInfo;
import xy.reflect.ui.undo.ModificationStack;
import xy.reflect.ui.undo.UndoOrder;
import xy.reflect.ui.util.Accessor;
import xy.reflect.ui.util.IOUtils;
import xy.reflect.ui.util.ImageIcon;
import xy.reflect.ui.util.Listener;
import xy.reflect.ui.util.MiscUtils;
import xy.reflect.ui.util.MoreSystemProperties;
import xy.reflect.ui.util.ReflectionUIError;
import xy.reflect.ui.util.ReflectionUIUtils;
/**
* This class inserts controls (buttons, context menus, ...) in the generated
* UIs that allow to customize them in real-time.
*
* @author olitank
*
*/
public class CustomizationTools {
protected final SwingCustomizer swingCustomizer;
protected CustomizationToolsRenderer toolsRenderer;
protected CustomizationToolsUI toolsUI;
public CustomizationTools(SwingCustomizer swingCustomizer) {
this.swingCustomizer = swingCustomizer;
toolsUI = createToolsUI();
toolsRenderer = createToolsRenderer();
}
public CustomizationToolsRenderer getToolsRenderer() {
return toolsRenderer;
}
public CustomizationToolsUI getToolsUI() {
return toolsUI;
}
protected JButton makeButton() {
JButton result = new JButton(this.swingCustomizer.getCustomizationsIcon());
result.setForeground(toolsRenderer.getToolsForegroundColor());
result.setPreferredSize(new Dimension(result.getPreferredSize().height, result.getPreferredSize().height));
result.setFocusable(false);
return result;
}
protected CustomizationToolsRenderer createToolsRenderer() {
return new CustomizationToolsRenderer(toolsUI) {
@Override
public boolean isCustomizationsEditorEnabled() {
return isMetaCustomizationAllowed();
}
@Override
public CustomizationTools createCustomizationTools() {
if (!isMetaCustomizationAllowed()) {
return null;
}
return new CustomizationTools(this) {
@Override
protected boolean isMetaCustomizationAllowed() {
return false;
}
};
}
};
}
protected boolean isMetaCustomizationAllowed() {
return MoreSystemProperties.isInfoCustomizationToolsCustomizationAllowed();
}
protected CustomizationToolsUI createToolsUI() {
InfoCustomizations infoCustomizations = new InfoCustomizations();
try {
String customizationsFilePath = MoreSystemProperties.getInfoCustomizationToolsCustomizationsFilePath();
if (customizationsFilePath != null) {
infoCustomizations.loadFromFile(new File(customizationsFilePath),
ReflectionUIUtils.getDebugLogListener(swingCustomizer.getReflectionUI()));
} else {
infoCustomizations.loadFromStream(
ReflectionUI.class.getResourceAsStream("resource/customizations-tools.icu"),
ReflectionUIUtils.getDebugLogListener(swingCustomizer.getReflectionUI()));
}
} catch (IOException e) {
throw new ReflectionUIError(e);
}
return new CustomizationToolsUI(infoCustomizations, swingCustomizer);
}
public Component makeButtonForType(final Object object) {
final ITypeInfo customizedType = this.swingCustomizer.getReflectionUI()
.buildTypeInfo(this.swingCustomizer.getReflectionUI().getTypeInfoSource(object));
final JButton result = makeButton();
result.setToolTipText(toolsRenderer.prepareMessageToDisplay(getCustomizationTitle(customizedType.getName())));
result.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
final JPopupMenu popupMenu = new JPopupMenu();
popupMenu.add(createMenuItem(new AbstractAction(
CustomizationTools.this.toolsRenderer.prepareMessageToDisplay("Type Options (Shared)...")) {
private static final long serialVersionUID = 1L;
@Override
public void actionPerformed(ActionEvent e) {
openTypeCustomizationDialog(result, swingCustomizer.getInfoCustomizations(), customizedType);
}
}));
popupMenu.add(createMenuItem(new AbstractAction(
CustomizationTools.this.toolsRenderer.prepareMessageToDisplay("Add Virtual Text Field...")) {
private static final long serialVersionUID = 1L;
@Override
public void actionPerformed(ActionEvent e) {
try {
String title = "New Virtual Text Field";
String fieldName = toolsRenderer.openInputDialog(result, "", "Field Name", title);
if (fieldName == null) {
return;
}
checkNewFieldNameAvailability(fieldName, customizedType);
String text = toolsRenderer.openInputDialog(result, "", "Text", title);
if (text == null) {
return;
}
VirtualFieldDeclaration fieldDeclaration = new VirtualFieldDeclaration();
fieldDeclaration.setFieldName(fieldName);
JavaClassBasedTypeInfoFinder typeFinder = new InfoCustomizations.JavaClassBasedTypeInfoFinder();
typeFinder.setClassName(String.class.getName());
fieldDeclaration.setFieldTypeFinder(typeFinder);
final TypeCustomization tc = InfoCustomizations.getTypeCustomization(
swingCustomizer.getInfoCustomizations(), customizedType.getName(), true);
final List fieldDeclarations = new ArrayList(
tc.getVirtualFieldDeclarations());
fieldDeclarations.add(fieldDeclaration);
final FieldCustomization fc = InfoCustomizations.getFieldCustomization(tc, fieldName, true);
final TextualStorage nullReplacement = new TextualStorage();
nullReplacement.save(text);
ModificationStack modificationStack = swingCustomizer.getCustomizationController()
.getModificationStack();
modificationStack.insideComposite(title, UndoOrder.getNormal(), new Accessor() {
@Override
public Boolean get() {
changeCustomizationFieldValue(tc, "virtualFieldDeclarations", fieldDeclarations);
changeCustomizationFieldValue(fc, "nullReplacement", nullReplacement);
changeCustomizationFieldValue(fc, "getOnlyForced", true);
return true;
}
}, false);
} catch (Throwable t) {
toolsRenderer.handleObjectException(result, t);
}
}
}));
popupMenu.add(createMenuItem(new AbstractAction(
CustomizationTools.this.toolsRenderer.prepareMessageToDisplay("Add Virtual Image Field...")) {
private static final long serialVersionUID = 1L;
@Override
public void actionPerformed(ActionEvent e) {
try {
String title = "New Virtual Image Field";
String fieldName = toolsRenderer.openInputDialog(result, "", "Field Name", title);
if (fieldName == null) {
return;
}
checkNewFieldNameAvailability(fieldName, customizedType);
File imageFile = toolsRenderer.openInputDialog(result, new File(""), "Image File", title);
if (imageFile == null) {
return;
}
Image image = ImageIO.read(imageFile);
VirtualFieldDeclaration fieldDeclaration = new VirtualFieldDeclaration();
fieldDeclaration.setFieldName(fieldName);
JavaClassBasedTypeInfoFinder typeFinder = new InfoCustomizations.JavaClassBasedTypeInfoFinder();
typeFinder.setClassName(Image.class.getName());
fieldDeclaration.setFieldTypeFinder(typeFinder);
final TypeCustomization tc = InfoCustomizations.getTypeCustomization(
swingCustomizer.getInfoCustomizations(), customizedType.getName(), true);
final List fieldDeclarations = new ArrayList(
tc.getVirtualFieldDeclarations());
fieldDeclarations.add(fieldDeclaration);
final FieldCustomization fc = InfoCustomizations.getFieldCustomization(tc, fieldName, true);
final TextualStorage nullReplacement = new TextualStorage();
Mapping storageMapping = new Mapping();
{
ConversionMethodFinder conversionMethodFinder = new ConversionMethodFinder();
{
conversionMethodFinder.setConversionClassName(ImageIcon.class.getName());
conversionMethodFinder
.setConversionMethodSignature(ReflectionUIUtils.buildMethodSignature(
new DefaultConstructorInfo(ReflectionUIUtils.STANDARD_REFLECTION,
ImageIcon.class.getConstructor(Image.class))));
storageMapping.setConversionMethodFinder(conversionMethodFinder);
}
ConversionMethodFinder reverseConversionMethodFinder = new ConversionMethodFinder();
{
reverseConversionMethodFinder.setConversionClassName(ImageIcon.class.getName());
reverseConversionMethodFinder
.setConversionMethodSignature(ReflectionUIUtils.buildMethodSignature(
new DefaultMethodInfo(ReflectionUIUtils.STANDARD_REFLECTION,
ImageIcon.class.getMethod("getImage"))));
storageMapping.setReverseConversionMethodFinder(reverseConversionMethodFinder);
}
nullReplacement.setPreConversion(storageMapping);
}
nullReplacement.save(image);
final TypeCustomization ftc = InfoCustomizations.getTypeCustomization(
fc.getSpecificTypeCustomizations(), typeFinder.getClassName(), true);
final Map specificProperties = new HashMap(
ftc.getSpecificProperties());
IFieldControlPlugin imagePlugin = SwingRendererUtils.findFieldControlPlugin(swingCustomizer,
new ImageViewPlugin().getIdentifier());
SwingRendererUtils.setCurrentFieldControlPlugin(swingCustomizer, specificProperties,
imagePlugin);
ModificationStack modificationStack = swingCustomizer.getCustomizationController()
.getModificationStack();
modificationStack.insideComposite(title, UndoOrder.getNormal(), new Accessor() {
@Override
public Boolean get() {
changeCustomizationFieldValue(tc, "virtualFieldDeclarations", fieldDeclarations);
changeCustomizationFieldValue(fc, "nullReplacement", nullReplacement);
changeCustomizationFieldValue(fc, "getOnlyForced", true);
changeCustomizationFieldValue(ftc, "specificProperties", specificProperties);
return true;
}
}, false);
} catch (Throwable t) {
toolsRenderer.handleObjectException(result, t);
}
}
}));
popupMenu.add(createMenuItem(
new AbstractAction(CustomizationTools.this.toolsRenderer.prepareMessageToDisplay("Refresh")) {
private static final long serialVersionUID = 1L;
@Override
public void actionPerformed(ActionEvent e) {
Form form = SwingRendererUtils.findParentForm(result, swingCustomizer);
try {
form.refresh(true);
if (SwingRendererUtils.findAncestorForms(form, swingCustomizer).size() > 0) {
SwingRendererUtils.updateWindowMenu(form, swingCustomizer);
}
} catch (Throwable t) {
swingCustomizer.handleObjectException(form, t);
}
}
}));
showMenu(popupMenu, result);
}
});
return result;
}
protected void openTypeCustomizationDialog(JButton customizerButton, InfoCustomizations infoCustomizations,
ITypeInfo customizedType) {
TypeCustomization tc = InfoCustomizations.getTypeCustomization(infoCustomizations, customizedType.getName(),
true);
fillTypeCustomization(tc, customizedType);
openCustomizationEditor(customizerButton, tc);
}
protected void openCustomizationEditor(final JButton customizerButton, final Object customization) {
StandardEditorBuilder dialogBuilder = new StandardEditorBuilder(toolsRenderer, customizerButton,
customization) {
@Override
protected boolean isDialogCancellable() {
return true;
}
@Override
protected ModificationStack getParentModificationStack() {
return swingCustomizer.getCustomizationController().getModificationStack();
}
};
dialogBuilder.createAndShowDialog();
}
public ITypeInfo getContainingObjectCustomizedType(FieldControlPlaceHolder fieldControlPlaceHolder) {
return CustomizationTools.this.swingCustomizer.getReflectionUI()
.buildTypeInfo(CustomizationTools.this.swingCustomizer.getReflectionUI()
.getTypeInfoSource(fieldControlPlaceHolder.getObject()));
}
public ITypeInfo getContainingObjectCustomizedType(MethodControlPlaceHolder methodControlPlaceHolder) {
return CustomizationTools.this.swingCustomizer.getReflectionUI()
.buildTypeInfo(CustomizationTools.this.swingCustomizer.getReflectionUI()
.getTypeInfoSource(methodControlPlaceHolder.getObject()));
}
public ITypeInfo getFieldControlDataCustomizedType(FieldControlPlaceHolder fieldControlPlaceHolder) {
IFieldControlData controlData = fieldControlPlaceHolder.getControlData();
if (controlData == null) {
return null;
}
return controlData.getType();
}
public FieldCustomization getFieldCustomization(String typeName, String fieldName,
InfoCustomizations infoCustomizations) {
FieldCustomization fc = InfoCustomizations
.getFieldCustomization(getTypeCustomization(typeName, infoCustomizations), fieldName, true);
return fc;
}
public MethodCustomization getMethodCustomization(String typeName, String methodSignature,
InfoCustomizations infoCustomizations) {
MethodCustomization mc = InfoCustomizations
.getMethodCustomization(getTypeCustomization(typeName, infoCustomizations), methodSignature, true);
return mc;
}
public FieldCustomization getFieldCustomization(FieldControlPlaceHolder fieldControlPlaceHolder,
InfoCustomizations infoCustomizations) {
FieldCustomization fc = InfoCustomizations.getFieldCustomization(
getContaingTypeCustomization(fieldControlPlaceHolder, infoCustomizations),
fieldControlPlaceHolder.getField().getName(), true);
return fc;
}
public MethodCustomization getMethodCustomization(MethodControlPlaceHolder methodControlPlaceHolder,
InfoCustomizations infoCustomizations) {
MethodCustomization mc = InfoCustomizations.getMethodCustomization(
getContaingTypeCustomization(methodControlPlaceHolder, infoCustomizations),
methodControlPlaceHolder.getMethod().getSignature(), true);
return mc;
}
public TypeCustomization getContaingTypeCustomization(FieldControlPlaceHolder fieldControlPlaceHolder,
InfoCustomizations infoCustomizations) {
String containingTypeName = getContainingObjectCustomizedType(fieldControlPlaceHolder).getName();
return getTypeCustomization(containingTypeName, infoCustomizations);
}
public TypeCustomization getContaingTypeCustomization(MethodControlPlaceHolder methodControlPlaceHolder,
InfoCustomizations infoCustomizations) {
String containingTypeName = getContainingObjectCustomizedType(methodControlPlaceHolder).getName();
return getTypeCustomization(containingTypeName, infoCustomizations);
}
public TypeCustomization getTypeCustomization(String containingTypeName, InfoCustomizations infoCustomizations) {
TypeCustomization t = InfoCustomizations.getTypeCustomization(infoCustomizations, containingTypeName, true);
return t;
}
public Component makeButtonForField(final FieldControlPlaceHolder fieldControlPlaceHolder) {
final JButton result = makeButton();
SwingRendererUtils.setMultilineToolTipText(result, toolsRenderer
.prepareMessageToDisplay(getCustomizationTitle(fieldControlPlaceHolder.getField().getName())));
result.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
final JPopupMenu popupMenu = new JPopupMenu();
popupMenu.add(createMenuItem(
new AbstractAction(CustomizationTools.this.toolsRenderer.prepareMessageToDisplay("Hide")) {
private static final long serialVersionUID = 1L;
@Override
public void actionPerformed(ActionEvent e) {
try {
hideField(result, getContainingObjectCustomizedType(fieldControlPlaceHolder),
fieldControlPlaceHolder.getField().getName());
} catch (Throwable t) {
toolsRenderer.handleObjectException(result, t);
}
}
}));
for (JMenuItem menuItem : makeMenuItemsForFieldPosition(result, fieldControlPlaceHolder)) {
popupMenu.add(menuItem);
}
for (JMenuItem menuItem : makeMenuItemsForFieldEncapsulation(result, fieldControlPlaceHolder)) {
popupMenu.add(menuItem);
}
for (JMenuItem menuItem : makeMenuItemsForFieldType(result, fieldControlPlaceHolder, false,
getFieldControlDataCustomizedType(fieldControlPlaceHolder))) {
popupMenu.add(menuItem);
}
popupMenu.add(createMenuItem(new AbstractAction(
CustomizationTools.this.toolsRenderer.prepareMessageToDisplay("More Options...")) {
private static final long serialVersionUID = 1L;
@Override
public void actionPerformed(ActionEvent e) {
openFieldCutomizationDialog(result, swingCustomizer.getInfoCustomizations(),
getContainingObjectCustomizedType(fieldControlPlaceHolder),
fieldControlPlaceHolder.getField().getName());
}
}));
showMenu(popupMenu, result);
}
});
return result;
}
public Component makeButtonForTextualStorageDataField(
final FieldControlPlaceHolder textualStorageDatafieldControlPlaceHolder) {
final JButton result = makeButton();
SwingRendererUtils.setMultilineToolTipText(result, toolsRenderer.prepareMessageToDisplay("Editing Options"));
result.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
final JPopupMenu popupMenu = new JPopupMenu();
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy