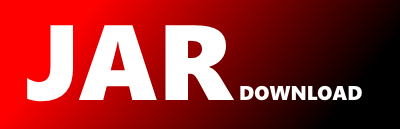
org.paukov.combinatorics3.PermutationWithRepetitionIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of combinatoricslib3 Show documentation
Show all versions of combinatoricslib3 Show documentation
Simple java library to generate permutations, combinations and other combinatorial sequences
package org.paukov.combinatorics3;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
class PermutationWithRepetitionIterator implements Iterator> {
final PermutationWithRepetitionGenerator _generator;
List _currentPermutation = null;
long _currentIndex = 0;
final int _n;
final int _k;
// Internal data
private int[] _bitVector = null;
public PermutationWithRepetitionIterator(
PermutationWithRepetitionGenerator generator) {
_generator = generator;
_n = generator._originalVector.size();
_k = generator._permutationLength;
List list = new ArrayList<>(_k);
T defaultValue = generator._originalVector.get(0);
for (int i = 0; i < _k; i++) {
list.add(defaultValue);
}
_currentPermutation = new ArrayList<>(list);
_bitVector = new int[_k + 2];
for (int j = 0; j <= _k; j++) {
_bitVector[j] = 0;
}
_currentIndex = 0;
}
@Override
public boolean hasNext() {
return (_bitVector[_k] != 1);
}
@Override
public List next() {
_currentIndex++;
for (int j = _k - 1; j >= 0; j--) {
_currentPermutation.set(j, _generator._originalVector.get(_bitVector[j]));
}
int i = 0;
while (_bitVector[i] == _n - 1) {
if (i < _k + 1)
_bitVector[i] = 0;
else {
_bitVector[_k] = 1;
return new ArrayList<>(_currentPermutation);
}
i++;
}
_bitVector[i]++;
return new ArrayList<>(_currentPermutation);
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
@Override
public String toString() {
return "PermutationWithRepetitionIterator=[#" + _currentIndex + ", "
+ _currentPermutation + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy