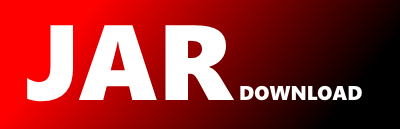
org.paukov.combinatorics3.SimpleCombinationIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of combinatoricslib3 Show documentation
Show all versions of combinatoricslib3 Show documentation
Simple java library to generate permutations, combinations and other combinatorial sequences
/**
* Combinatorics Library 3
* Copyright 2016 Dmytro Paukov [email protected]
*/
package org.paukov.combinatorics3;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/**
* Iterator for the simple combination generator
*
* @author Dmytro Paukov
* @version 3.0
* @see SimpleCombinationGenerator
* @param
* Type of the elements in the combinations
*/
class SimpleCombinationIterator implements
Iterator> {
final SimpleCombinationGenerator generator;
List currentSimpleCombination = null;
long currentIndex = 0;
final int lengthN;
final int lengthK;
// Internal array
int[] bitVector = null;
//Criteria to stop iterating
private int endIndex = 0;
SimpleCombinationIterator(SimpleCombinationGenerator generator) {
this.generator = generator;
lengthN = generator.originalVector.size();
lengthK = generator.combinationLength;
currentSimpleCombination = new ArrayList();
bitVector = new int[lengthK + 1];
for (int i = 0; i <= lengthK; i++) {
bitVector[i] = i;
}
if (lengthN > 0) {
endIndex = 1;
}
currentIndex = 0;
}
/**
* Returns true if all combinations were iterated, otherwise false
*/
@Override
public boolean hasNext() {
return !((endIndex == 0) || (lengthK > lengthN));
}
/**
* Moves to the next combination
*/
@Override
public List next() {
currentIndex++;
for (int i = 1; i <= lengthK; i++) {
int index = bitVector[i] - 1;
if (generator.originalVector.size() > 0) {
setValue(currentSimpleCombination, i - 1,
generator.originalVector.get(index));
}
}
endIndex = lengthK;
while (bitVector[endIndex] == lengthN - lengthK + endIndex) {
endIndex--;
if (endIndex == 0)
break;
}
bitVector[endIndex]++;
for (int i = endIndex + 1; i <= lengthK; i++) {
bitVector[i] = bitVector[i - 1] + 1;
}
// return the current combination
return new ArrayList(currentSimpleCombination);
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
@Override
public String toString() {
return "SimpleCombinationIterator=[#" + currentIndex + ", "
+ currentSimpleCombination + "]";
}
private static void setValue(List list, int index, T value) {
try {
list.set(index, value);
} catch (IndexOutOfBoundsException ex) {
list.add(index, value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy