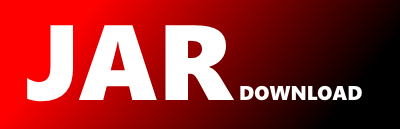
com.github.dreamhead.moco.runner.FileRunner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of moco-runner Show documentation
Show all versions of moco-runner Show documentation
Moco is an easy setup stub framework, mainly focusing on testing and integration.
package com.github.dreamhead.moco.runner;
import com.github.dreamhead.moco.MocoException;
import com.github.dreamhead.moco.bootstrap.arg.StartArgs;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
import java.util.stream.Collectors;
import java.util.stream.StreamSupport;
import static com.github.dreamhead.moco.runner.JsonRunner.newJsonRunnerWithStreams;
public abstract class FileRunner implements Runner {
private Logger logger = LoggerFactory.getLogger(FileRunner.class);
private Runner runner;
protected abstract Runner newRunner();
private FileRunner() {
this.runner = newRunner();
}
public synchronized void restart() {
this.runner.stop();
this.runner = refreshRunner(this.runner);
this.runner.run();
}
private Runner refreshRunner(final Runner current) {
try {
return newRunner();
} catch (Exception e) {
logger.warn("Fail to load new runner: " + e.getMessage());
return current;
}
}
public Runner getRunner() {
return runner;
}
@Override
public void run() {
this.runner.run();
}
@Override
public void stop() {
this.runner.stop();
}
public static FileRunner createConfigurationFileRunner(final Iterable files, final StartArgs startArgs) {
return new FileRunner() {
@Override
protected Runner newRunner() {
return newJsonRunnerWithStreams(toInputStreams(files), startArgs);
}
};
}
public static FileRunner createSettingFileRunner(final File settingsFile, final StartArgs startArgs) {
return new FileRunner() {
@Override
protected Runner newRunner() {
return new SettingRunner(toInputStream(settingsFile), startArgs);
}
};
}
private static FileInputStream toInputStream(final File file) {
try {
return new FileInputStream(file);
} catch (FileNotFoundException e) {
throw new MocoException(e);
}
}
private static Iterable toInputStreams(final Iterable files) {
return StreamSupport.stream(files.spliterator(), false)
.map(FileRunner::toInputStream)
.collect(Collectors.toList());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy