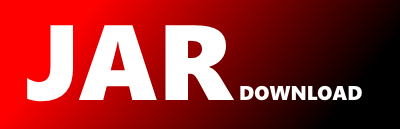
com.github.drstefanfriedrich.f2blib.antlr.FunctionsVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of f2blib Show documentation
Show all versions of f2blib Show documentation
Parse mathematical function expressions, convert them to Java bytecode, and evaluate them very quickly
// Generated from com/github/drstefanfriedrich/f2blib/antlr/Functions.g4 by ANTLR 4.7.1
package com.github.drstefanfriedrich.f2blib.antlr;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link FunctionsParser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface FunctionsVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link FunctionsParser#function_definition}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunction_definition(FunctionsParser.Function_definitionContext ctx);
/**
* Visit a parse tree produced by the {@code singleValuedFunctions}
* labeled alternative in {@link FunctionsParser#function_body}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSingleValuedFunctions(FunctionsParser.SingleValuedFunctionsContext ctx);
/**
* Visit a parse tree produced by {@link FunctionsParser#single_valued_functions}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSingle_valued_functions(FunctionsParser.Single_valued_functionsContext ctx);
/**
* Visit a parse tree produced by {@link FunctionsParser#single_valued_function}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSingle_valued_function(FunctionsParser.Single_valued_functionContext ctx);
/**
* Visit a parse tree produced by the {@code ln}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLn(FunctionsParser.LnContext ctx);
/**
* Visit a parse tree produced by the {@code arsinh}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArsinh(FunctionsParser.ArsinhContext ctx);
/**
* Visit a parse tree produced by the {@code const}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConst(FunctionsParser.ConstContext ctx);
/**
* Visit a parse tree produced by the {@code cos}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCos(FunctionsParser.CosContext ctx);
/**
* Visit a parse tree produced by the {@code arcosh}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArcosh(FunctionsParser.ArcoshContext ctx);
/**
* Visit a parse tree produced by the {@code subtraction}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSubtraction(FunctionsParser.SubtractionContext ctx);
/**
* Visit a parse tree produced by the {@code arccos}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArccos(FunctionsParser.ArccosContext ctx);
/**
* Visit a parse tree produced by the {@code faculty}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFaculty(FunctionsParser.FacultyContext ctx);
/**
* Visit a parse tree produced by the {@code division}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDivision(FunctionsParser.DivisionContext ctx);
/**
* Visit a parse tree produced by the {@code tanh}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTanh(FunctionsParser.TanhContext ctx);
/**
* Visit a parse tree produced by the {@code neg}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNeg(FunctionsParser.NegContext ctx);
/**
* Visit a parse tree produced by the {@code param}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParam(FunctionsParser.ParamContext ctx);
/**
* Visit a parse tree produced by the {@code sqrt}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSqrt(FunctionsParser.SqrtContext ctx);
/**
* Visit a parse tree produced by the {@code pos}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPos(FunctionsParser.PosContext ctx);
/**
* Visit a parse tree produced by the {@code sin}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSin(FunctionsParser.SinContext ctx);
/**
* Visit a parse tree produced by the {@code artanh}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArtanh(FunctionsParser.ArtanhContext ctx);
/**
* Visit a parse tree produced by the {@code power}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPower(FunctionsParser.PowerContext ctx);
/**
* Visit a parse tree produced by the {@code multiplication}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitMultiplication(FunctionsParser.MultiplicationContext ctx);
/**
* Visit a parse tree produced by the {@code exp}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExp(FunctionsParser.ExpContext ctx);
/**
* Visit a parse tree produced by the {@code addition}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAddition(FunctionsParser.AdditionContext ctx);
/**
* Visit a parse tree produced by the {@code tan}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTan(FunctionsParser.TanContext ctx);
/**
* Visit a parse tree produced by the {@code sinh}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSinh(FunctionsParser.SinhContext ctx);
/**
* Visit a parse tree produced by the {@code var}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVar(FunctionsParser.VarContext ctx);
/**
* Visit a parse tree produced by the {@code binomial}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitBinomial(FunctionsParser.BinomialContext ctx);
/**
* Visit a parse tree produced by the {@code parenthesis}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParenthesis(FunctionsParser.ParenthesisContext ctx);
/**
* Visit a parse tree produced by the {@code int}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInt(FunctionsParser.IntContext ctx);
/**
* Visit a parse tree produced by the {@code cosh}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCosh(FunctionsParser.CoshContext ctx);
/**
* Visit a parse tree produced by the {@code abs}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAbs(FunctionsParser.AbsContext ctx);
/**
* Visit a parse tree produced by the {@code round}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitRound(FunctionsParser.RoundContext ctx);
/**
* Visit a parse tree produced by the {@code arctan}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArctan(FunctionsParser.ArctanContext ctx);
/**
* Visit a parse tree produced by the {@code doub}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDoub(FunctionsParser.DoubContext ctx);
/**
* Visit a parse tree produced by the {@code arcsin}
* labeled alternative in {@link FunctionsParser#expression}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArcsin(FunctionsParser.ArcsinContext ctx);
/**
* Visit a parse tree produced by {@link FunctionsParser#constant}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitConstant(FunctionsParser.ConstantContext ctx);
/**
* Visit a parse tree produced by {@link FunctionsParser#class_name}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitClass_name(FunctionsParser.Class_nameContext ctx);
/**
* Visit a parse tree produced by {@link FunctionsParser#integer}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitInteger(FunctionsParser.IntegerContext ctx);
/**
* Visit a parse tree produced by {@link FunctionsParser#floatingPoint}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFloatingPoint(FunctionsParser.FloatingPointContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy