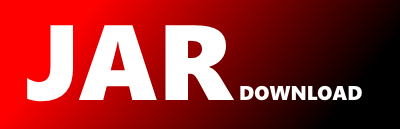
com.dxy.library.cache.Cache Maven / Gradle / Ivy
package com.dxy.library.cache;
import com.dxy.library.cache.constant.CacheType;
import com.dxy.library.cache.memory.IMemory;
import com.dxy.library.cache.memory.caffeine.CacheCaffeine;
import com.dxy.library.cache.memory.guava.CacheGuava;
import com.dxy.library.cache.redis.IRedis;
import com.dxy.library.cache.redis.cluster.CacheRedisCluster;
import com.dxy.library.cache.redis.sentinel.CacheRedisSentinel;
import com.dxy.library.cache.redis.shard.CacheRedisShard;
import com.dxy.library.cache.redis.single.CacheRedisSingle;
import com.dxy.library.json.gson.GsonUtil;
import com.dxy.library.util.common.config.ConfigUtils;
import com.google.gson.reflect.TypeToken;
import org.apache.commons.lang3.BooleanUtils;
import org.apache.commons.lang3.StringUtils;
import redis.clients.jedis.BitOP;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* 缓存操作类,部分缓存交由内存+Redis的模式进行,但仅限于不频繁变更的内容
* @author duanxinyuan
* 2018/8/9 15:27
*/
public class Cache {
private static boolean IS_MEMORY_ENABLE;
private volatile static IMemory memory;
private volatile static IRedis redis;
static {
IS_MEMORY_ENABLE = BooleanUtils.toBoolean(ConfigUtils.getConfig("cache.memory.enable", Boolean.class));
if (IS_MEMORY_ENABLE) {
String memoryCacheType = ConfigUtils.getConfig("cache.memory.type");
switch (memoryCacheType) {
case CacheType.Memory.caffeine:
memory = new CacheCaffeine();
break;
case CacheType.Memory.guava:
memory = new CacheGuava();
break;
default:
break;
}
}
String redisCacheType = ConfigUtils.getConfig("cache.redis.type");
switch (redisCacheType) {
case CacheType.Redis.single:
redis = new CacheRedisSingle();
break;
case CacheType.Redis.sentinel:
redis = new CacheRedisSentinel();
break;
case CacheType.Redis.shard:
redis = new CacheRedisShard();
break;
case CacheType.Redis.cluster:
redis = new CacheRedisCluster();
break;
default:
break;
}
}
public static String set(String key, T value) {
if (IS_MEMORY_ENABLE) {
if (value instanceof String) {
memory.set(key, value);
} else {
memory.set(key, GsonUtil.to(value));
}
}
return redis.set(key, value);
}
public static String set(String key, T value, int seconds) {
if (IS_MEMORY_ENABLE) {
if (value instanceof String) {
memory.set(key, value);
} else {
memory.set(key, GsonUtil.to(value));
}
}
return redis.set(key, value, seconds);
}
public static Long setnx(String key, T value) {
return redis.setnx(key, value);
}
public static Long setnx(String key, T value, int seconds) {
return redis.setnx(key, value, seconds);
}
public static String get(String key) {
if (IS_MEMORY_ENABLE) {
String value = memory.get(key);
if (StringUtils.isNotEmpty(value)) {
return value;
}
}
String value = redis.get(key);
if (IS_MEMORY_ENABLE && StringUtils.isNotEmpty(value)) {
memory.set(key, value);
}
return value;
}
public static T get(String key, Class c) {
if (IS_MEMORY_ENABLE) {
String value = memory.get(key);
if (StringUtils.isNotEmpty(value)) {
return GsonUtil.from(value, c);
}
}
String value = redis.get(key);
if (IS_MEMORY_ENABLE && StringUtils.isNotEmpty(value)) {
memory.set(key, value);
}
return GsonUtil.from(value, c);
}
public static T get(String key, TypeToken typeToken) {
if (IS_MEMORY_ENABLE) {
String value = memory.get(key);
if (StringUtils.isNotEmpty(value)) {
return GsonUtil.from(value, typeToken);
}
}
String value = redis.get(key);
if (IS_MEMORY_ENABLE && StringUtils.isNotEmpty(value)) {
memory.set(key, value);
}
return GsonUtil.from(value, typeToken);
}
public static Long incr(String key, Integer value, int seconds) {
if (IS_MEMORY_ENABLE) {//清除内存中的数据,防止脏读
memory.del(key);
}
return redis.incr(key, value, seconds);
}
public static Long incr(String key, Integer value) {
if (IS_MEMORY_ENABLE) {//清除内存中的数据,防止脏读
memory.del(key);
}
return redis.incr(key, value);
}
public static Long decr(String key, Integer value) {
if (IS_MEMORY_ENABLE) {//清除内存中的数据,防止脏读
memory.del(key);
}
return redis.decr(key, value);
}
public static Long decr(String key, Integer value, int seconds) {
if (IS_MEMORY_ENABLE) {//清除内存中的数据,防止脏读
memory.del(key);
}
return redis.decr(key, value, seconds);
}
public static Long expire(String key, int seconds) {
return redis.expire(key, seconds);
}
public static Long persist(String key) {
return redis.persist(key);
}
public static boolean exist(String key) {
//redis数据类型太多,判断是否存在之后无法回设内存
return redis.exist(key);
}
public static Long del(String key) {
if (IS_MEMORY_ENABLE) {
memory.del(key);
}
return redis.del(key);
}
public static void del(String... keys) {
if (IS_MEMORY_ENABLE) {
memory.del(keys);
}
redis.del(keys);
}
public static Long lpush(String key, T value) {
return redis.lpush(key, value);
}
public static Long lpush(String key, T value, int seconds) {
return redis.lpush(key, value, seconds);
}
public static Long lpush(String key, List values) {
return redis.lpush(key, values);
}
public static Long lpush(String key, List values, int seconds) {
return redis.lpush(key, values, seconds);
}
public static Long rpush(String key, T value) {
return redis.rpush(key, value);
}
public static Long rpush(String key, T value, int seconds) {
return redis.rpush(key, value, seconds);
}
public static Long rpush(String key, List values) {
return redis.rpush(key, values);
}
public static Long rpush(String key, List values, int seconds) {
return redis.rpush(key, values, seconds);
}
public static List lrange(String key) {
return redis.lrange(key);
}
public static List lrange(String key, Class c) {
return redis.lrange(key, c);
}
public static List lrange(String key, long end) {
return redis.lrange(key, end);
}
public static List lrange(String key, long end, Class c) {
return redis.lrange(key, end, c);
}
public static List lrange(String key, long start, long end) {
return redis.lrange(key, start, end);
}
public static List lrange(String key, long start, long end, Class c) {
return redis.lrange(key, start, end, c);
}
public static List lrangePage(String key, int pageNo, int pageSize) {
return redis.lrangePage(key, pageNo, pageSize);
}
public static List lrangePage(String key, int pageNo, int pageSize, Class c) {
return redis.lrangePage(key, pageNo, pageSize, c);
}
public static String lindex(String key, int index) {
return redis.lindex(key, index);
}
public static T lindex(String key, int index, Class c) {
return redis.lindex(key, index, c);
}
public static Long llen(String key) {
return redis.llen(key);
}
public static void lclear(String key) {
redis.lclear(key);
}
public static Long lrem(String key, String value) {
return redis.lrem(key, value);
}
public static Long lrem(String key, T value) {
return redis.lrem(key, value);
}
public static Long lrem(String key, long count, String value) {
return redis.lrem(key, count, value);
}
public static Long lrem(String key, long count, T value) {
return redis.lrem(key, count, value);
}
public static String ltrim(String key, long start, long end) {
return redis.ltrim(key, start, end);
}
public static String lpop(String key) {
return redis.lpop(key);
}
public static String rpop(String key) {
return redis.rpop(key);
}
public static Long sadd(String key, String... values) {
if (IS_MEMORY_ENABLE) {//清除内存中的数据,防止脏读
memory.del(key);
}
return redis.sadd(key, values);
}
public static Long sadd(String key, int seconds, String... values) {
if (IS_MEMORY_ENABLE) {//清除内存中的数据,防止脏读
memory.del(key);
}
return redis.sadd(key, seconds, values);
}
public static boolean sismember(String key, String value) {
return redis.sismember(key, value);
}
public static Set smembers(String key) {
if (IS_MEMORY_ENABLE) {
String value = memory.get(key);
if (StringUtils.isNotEmpty(value)) {
return GsonUtil.from(value, new TypeToken>() {});
}
}
Set set = redis.smembers(key);
if (IS_MEMORY_ENABLE && set != null && set.size() != 0) {
memory.set(key, GsonUtil.to(set));
}
return set;
}
public static Long hset(String key, String field, T value) {
if (IS_MEMORY_ENABLE) {//清除内存中的数据,防止脏读
memory.del(key);
}
return redis.hset(key, field, value);
}
public static String hmset(String key, String... values) {
if (IS_MEMORY_ENABLE) {//清除内存中的数据,防止脏读
memory.del(key);
}
return redis.hmset(key, values);
}
public static Long hset(String key, String field, T value, int seconds) {
if (IS_MEMORY_ENABLE) {//清除内存中的数据,防止脏读
memory.del(key);
}
return redis.hset(key, field, value, seconds);
}
public static String hmset(String key, int seconds, String... values) {
if (IS_MEMORY_ENABLE) {//清除内存中的数据,防止脏读
memory.del(key);
}
return redis.hmset(key, seconds, values);
}
public static String hget(String key, String field) {
if (IS_MEMORY_ENABLE) {
String value = memory.get(key);
if (StringUtils.isNotEmpty(value)) {
Map map = GsonUtil.from(value, new TypeToken
© 2015 - 2025 Weber Informatics LLC | Privacy Policy