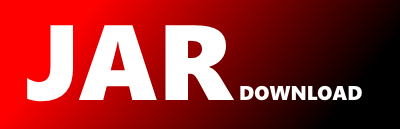
org.erc.coinbase.pro.rest.Signature Maven / Gradle / Ivy
/**
This file is part of coinbase-pro-client.
coinbase-pro-client is free software: you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
Foobar is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with coinbase-pro-client. If not, see .
*/
package org.erc.coinbase.pro.rest;
import java.util.Base64;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import org.erc.coinbase.pro.exceptions.SignatureException;
/**
* Signature
* The CB-ACCESS-SIGN header is generated by creating a sha256 HMAC.
*
* The base64-decoded secret key on the string:
* timestamp + method + requestPath + body and base64-encode the output.
*
* The timestamp value is the same as the CB-ACCESS-TIMESTAMP header.
*
*/
final class Signature {
/** The signature MAC Instance. */
private Mac sha256 = null;
/**
* Instantiates a new signature.
*
* @param secretKey the secret key
* @throws SignatureException the signature exception
*/
public Signature(String secretKey) throws SignatureException {
byte[] secretDecoded = Base64.getDecoder().decode(secretKey);
try {
sha256 = Mac.getInstance("HmacSHA256");
sha256.init(new SecretKeySpec(secretDecoded, sha256.getAlgorithm()));
} catch (Exception e) {
throw new SignatureException("Cannot set up authentication headers.",e);
}
}
/**
* Sign.
*
* @param requestPath the request path
* @param method the HTTP method
* @param body the body
* @param timestamp the timestamp (Execution TS)
* @return the result sing string
* @throws SignatureException the signature exception
*/
public String sign(String requestPath, String method, String body, Long timestamp) throws SignatureException {
try {
String prehash = timestamp + method.toUpperCase() + requestPath + body;
return Base64.getEncoder().encodeToString(sha256.doFinal(prehash.getBytes()));
} catch (Exception e) {
throw new SignatureException("Cannot set up authentication headers.",e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy