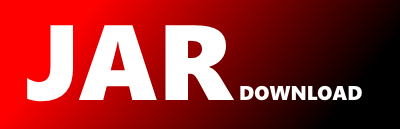
controllers.ApiHelpController.scala Maven / Gradle / Ivy
/**
* Copyright 2017 SmartBear Software, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package controllers
import io.swagger.core.filter.SpecFilter
import io.swagger.models.Swagger
import io.swagger.util.Json
import javax.inject.Inject
import play.api.Logger
import play.api.http.ContentTypes
import play.api.mvc._
import play.modules.swagger.SwaggerPlugin
import scala.jdk.CollectionConverters._
class ApiHelpController @Inject() (components: ControllerComponents, val swaggerPlugin: SwaggerPlugin)
extends AbstractController(components) with SwaggerBaseApiController {
def getResources: Action[AnyContent] = Action { implicit request =>
val host: String = swaggerPlugin.config.host
val resourceListing: Swagger = getResourceListing(host)
respond(resourceListing)
}
def getResource(path: String): Action[AnyContent] = Action { implicit request =>
val host: String = swaggerPlugin.config.host
val apiListing: Swagger = getApiListing(path, host)
respond(apiListing)
}
}
trait SwaggerBaseApiController {
def swaggerPlugin: SwaggerPlugin
protected val AccessControlAllowOrigin = ("Access-Control-Allow-Origin", "*")
/**
* Get a list of all top level resources
*/
protected def getResourceListing(host: String)(implicit requestHeader: RequestHeader): Swagger = {
Logger("swagger").debug("ApiHelpInventory.getRootResources")
val queryParams = (for((key, value) <- requestHeader.queryString) yield {
(key, value.toList.asJava)
}).asJava
val cookies = (for(cookie <- requestHeader.cookies) yield {
(cookie.name, cookie.value)
}).toMap.asJava
val headers = (for((key, value) <- requestHeader.headers.toMap) yield {
(key, value.toList.asJava)
}).asJava
val f = new SpecFilter
val specs = swaggerPlugin.apiListingCache.listing(host)
swaggerPlugin.swaggerSpecFilter match {
case Some(filter) => f.filter(specs, filter, queryParams, cookies, headers)
case None => specs
}
}
/**
* Get detailed API/models for a given resource
*/
protected def getApiListing(resourceName: String, host: String)(implicit requestHeader: RequestHeader): Swagger = {
Logger("swagger").debug("ApiHelpInventory.getResource(%s)".format(resourceName))
val f = new SpecFilter
val queryParams = requestHeader.queryString.map {case (key, value) => key -> value.toList.asJava}
val cookies = requestHeader.cookies.map {cookie => cookie.name -> cookie.value}.toMap.asJava
val headers = requestHeader.headers.toMap.map {case (key, value) => key -> value.toList.asJava}.asJava
val pathPart = resourceName
val specs = swaggerPlugin.apiListingCache.listing(host)
val clone = swaggerPlugin.swaggerSpecFilter match {
case Some(filter) => f.filter(specs, filter, queryParams.asJava, cookies, headers)
case None => specs
}
clone.setPaths(clone.getPaths.asScala.filter(_._1.startsWith(pathPart)).asJava)
clone
}
protected def respond(spec: Swagger): Result = {
Results.Ok(Json.pretty(spec))
.as(ContentTypes.JSON)
.withHeaders(AccessControlAllowOrigin)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy