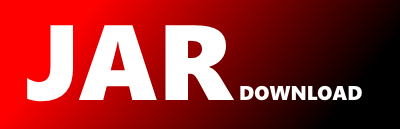
com.github.dylon.liblevenshtein.levenshtein.StateTransitionFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of liblevenshtein Show documentation
Show all versions of liblevenshtein Show documentation
A library for spelling-correction based on Levenshtein Automata.
The newest version!
// Generated by delombok at Sat Apr 30 14:43:05 PDT 2016
package com.github.dylon.liblevenshtein.levenshtein;
import java.io.Serializable;
import java.util.Comparator;
import com.github.dylon.liblevenshtein.levenshtein.factory.IPositionTransitionFactory;
import com.github.dylon.liblevenshtein.levenshtein.factory.IStateFactory;
/**
* Transitions one state to another, given a set of inputs. This function
* doesn't need to know about the Levenshtein algorithm, as the
* algorithm-specific components are injected via setters.
* @author Dylon Edwards
* @since 2.1.0
*/
public class StateTransitionFunction implements IStateTransitionFunction, Serializable {
private static final long serialVersionUID = 1L;
/**
* Sorts {@link IState} elements in an unsubsumption-friendly fashion.
*/
private Comparator comparator;
/**
* Builds and recycles {@link IState} instances.
*/
private IStateFactory stateFactory;
/**
* Builds position vector, transition functions according to the Levenshtein
* algorithm.
*/
private IPositionTransitionFactory transitionFactory;
/**
* Merges states together according to the Levenshtein algorithm.
*/
private IMergeFunction merge;
/**
* Removes positions from a state that are subsumed by other positions in that
* state.
*/
private IUnsubsumeFunction unsubsume;
/**
* Max number of errors tolerated in spelling candidates, from the query term.
*/
private int maxDistance;
/**
* {@inheritDoc}
*/
@Override
public IState of(final IState currState, final boolean[] characteristicVector) {
final IPositionTransitionFunction transition = transitionFactory.build();
final int offset = currState.getOuter(0)[0];
final IState nextState = stateFactory.build();
final int n = maxDistance;
for (int m = 0; m < currState.size(); ++m) {
final IState positions = transition.of(n, currState.getOuter(m), characteristicVector, offset);
if (null == positions) {
continue;
}
merge.into(nextState, positions);
}
unsubsume.at(nextState);
if (nextState.size() > 0) {
nextState.sort(comparator);
return nextState;
}
return null;
}
/**
* Sorts {@link IState} elements in an unsubsumption-friendly fashion.
* @param comparator Sorts {@link IState} elements in an unsubsumption-friendly fashion.
* @return This {@link StateTransitionFunction} for fluency.
*/
@java.lang.SuppressWarnings("all")
@edu.umd.cs.findbugs.annotations.SuppressFBWarnings(justification = "generated code")
@javax.annotation.Generated("lombok")
public StateTransitionFunction comparator(final Comparator comparator) {
this.comparator = comparator;
return this;
}
/**
* Builds and recycles {@link IState} instances.
* @param stateFactory Builds and recycles {@link IState} instances.
* @return This {@link StateTransitionFunction} for fluency.
*/
@java.lang.SuppressWarnings("all")
@edu.umd.cs.findbugs.annotations.SuppressFBWarnings(justification = "generated code")
@javax.annotation.Generated("lombok")
public StateTransitionFunction stateFactory(final IStateFactory stateFactory) {
this.stateFactory = stateFactory;
return this;
}
/**
* Builds position vector, transition functions according to the Levenshtein
* algorithm.
* @param transitionFactory Builds position vector, transition functions
* according to the Levenshtein algorithm.
* @return This {@link StateTransitionFunction} for fluency.
*/
@java.lang.SuppressWarnings("all")
@edu.umd.cs.findbugs.annotations.SuppressFBWarnings(justification = "generated code")
@javax.annotation.Generated("lombok")
public StateTransitionFunction transitionFactory(final IPositionTransitionFactory transitionFactory) {
this.transitionFactory = transitionFactory;
return this;
}
/**
* Merges states together according to the Levenshtein algorithm.
* @param merge Merges states together according to the Levenshtein algorithm.
* @return This {@link StateTransitionFunction} for fluency.
*/
@java.lang.SuppressWarnings("all")
@edu.umd.cs.findbugs.annotations.SuppressFBWarnings(justification = "generated code")
@javax.annotation.Generated("lombok")
public StateTransitionFunction merge(final IMergeFunction merge) {
this.merge = merge;
return this;
}
/**
* Removes positions from a state that are subsumed by other positions in that
* state.
* @param unsubsume Removes positions from a state that are subsumed by other
* positions in that state.
* @return This {@link StateTransitionFunction} for fluency.
*/
@java.lang.SuppressWarnings("all")
@edu.umd.cs.findbugs.annotations.SuppressFBWarnings(justification = "generated code")
@javax.annotation.Generated("lombok")
public StateTransitionFunction unsubsume(final IUnsubsumeFunction unsubsume) {
this.unsubsume = unsubsume;
return this;
}
/**
* Max number of errors tolerated in spelling candidates, from the query term.
* @param maxDistance Max number of errors tolerated in spelling candidates,
* from the query term.
* @return This {@link StateTransitionFunction} for fluency.
*/
@java.lang.SuppressWarnings("all")
@edu.umd.cs.findbugs.annotations.SuppressFBWarnings(justification = "generated code")
@javax.annotation.Generated("lombok")
public StateTransitionFunction maxDistance(final int maxDistance) {
this.maxDistance = maxDistance;
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy