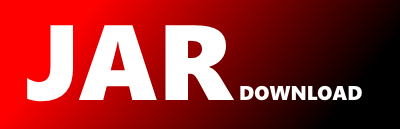
com.github.dynodao.processor.schema.SchemaContext Maven / Gradle / Ivy
package com.github.dynodao.processor.schema;
import com.github.dynodao.annotation.DynoDaoSchema;
import com.github.dynodao.processor.schema.attribute.DynamoAttribute;
import com.github.dynodao.processor.schema.parse.SchemaParsers;
import javax.lang.model.element.Element;
import javax.lang.model.type.TypeMirror;
/**
* Houses contextual data for parsing a {@link DynoDaoSchema} type and nested classes.
* Parsing entails serialization and deserialization of each attribute into an {@link com.amazonaws.services.dynamodbv2.model.AttributeValue},
* which then implies the attribute value types, and eventually the structure.
*/
public class SchemaContext {
private final SchemaParsers schemaParsers;
SchemaContext(SchemaParsers schemaParsers) {
this.schemaParsers = schemaParsers;
}
/**
* Returns true if this parser instance applies to the element provided.
* @param element the schema element to test
* @param typeMirror the specific type to test, may be element or nested within
* @return true if this applies to the element, false otherwise
*/
public boolean isApplicableTo(Element element, TypeMirror typeMirror) {
return schemaParsers.stream().anyMatch(schemaParser -> schemaParser.isApplicableTo(element, typeMirror, this));
}
/**
* Returns a new {@link DynamoAttribute} from the given contextual data.
* @param element the element to parse
* @param typeMirror the specific type to parse, may be element or nested within
* @param path the relative path to the attribute
* @return the attribute
*/
public DynamoAttribute parseAttribute(Element element, TypeMirror typeMirror, String path) {
return schemaParsers.stream()
.filter(schemaParser -> schemaParser.isApplicableTo(element, typeMirror, this))
.map(schemaParser -> schemaParser.parseAttribute(element, typeMirror, path, this))
.findFirst()
.orElseThrow(() -> new AssertionError("attribute is at least NullDynamoAttribute"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy