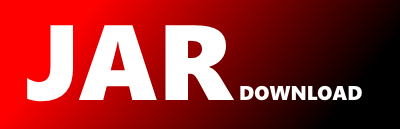
dz.jtsgen.processor.jtp.info.ExecutableElementHelperImplBuilder Maven / Gradle / Ivy
Show all versions of jtsgen-processor Show documentation
package dz.jtsgen.processor.jtp.info;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.regex.Pattern;
import javax.annotation.Generated;
/**
* Immutable implementation of {@link ExecutableElementHelperImpl}.
*
* Use the builder to create immutable instances:
* {@code ExecutableElementHelperImplBuilder.builder()}.
*/
@SuppressWarnings({"all"})
@Generated({"Immutables.generator", "ExecutableElementHelperImpl"})
final class ExecutableElementHelperImplBuilder
extends ExecutableElementHelperImpl {
private final List getterPrefixes;
private final List setterPrefixes;
private ExecutableElementHelperImplBuilder(ExecutableElementHelperImplBuilder.Builder builder) {
if (builder.getterPrefixesIsSet()) {
initShim.getterPrefixes(createUnmodifiableList(true, builder.getterPrefixes));
}
if (builder.setterPrefixesIsSet()) {
initShim.setterPrefixes(createUnmodifiableList(true, builder.setterPrefixes));
}
this.getterPrefixes = initShim.getterPrefixes();
this.setterPrefixes = initShim.setterPrefixes();
this.initShim = null;
}
private ExecutableElementHelperImplBuilder(List getterPrefixes, List setterPrefixes) {
this.getterPrefixes = getterPrefixes;
this.setterPrefixes = setterPrefixes;
this.initShim = null;
}
private static final int STAGE_INITIALIZING = -1;
private static final int STAGE_UNINITIALIZED = 0;
private static final int STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
private final class InitShim {
private List getterPrefixes;
private int getterPrefixesBuildStage;
List getterPrefixes() {
if (getterPrefixesBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (getterPrefixesBuildStage == STAGE_UNINITIALIZED) {
getterPrefixesBuildStage = STAGE_INITIALIZING;
this.getterPrefixes = createUnmodifiableList(false, createSafeList(ExecutableElementHelperImplBuilder.super.getterPrefixes(), true, false));
getterPrefixesBuildStage = STAGE_INITIALIZED;
}
return this.getterPrefixes;
}
void getterPrefixes(List getterPrefixes) {
this.getterPrefixes = getterPrefixes;
getterPrefixesBuildStage = STAGE_INITIALIZED;
}
private List setterPrefixes;
private int setterPrefixesBuildStage;
List setterPrefixes() {
if (setterPrefixesBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (setterPrefixesBuildStage == STAGE_UNINITIALIZED) {
setterPrefixesBuildStage = STAGE_INITIALIZING;
this.setterPrefixes = createUnmodifiableList(false, createSafeList(ExecutableElementHelperImplBuilder.super.setterPrefixes(), true, false));
setterPrefixesBuildStage = STAGE_INITIALIZED;
}
return this.setterPrefixes;
}
void setterPrefixes(List setterPrefixes) {
this.setterPrefixes = setterPrefixes;
setterPrefixesBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
ArrayList attributes = new ArrayList();
if (getterPrefixesBuildStage == STAGE_INITIALIZING) attributes.add("getterPrefixes");
if (setterPrefixesBuildStage == STAGE_INITIALIZING) attributes.add("setterPrefixes");
return "Cannot build ExecutableElementHelperImpl, attribute initializers form cycle" + attributes;
}
}
/**
* @return The value of the {@code getterPrefixes} attribute
*/
@Override
List getterPrefixes() {
InitShim shim = this.initShim;
return shim != null
? shim.getterPrefixes()
: this.getterPrefixes;
}
/**
* @return The value of the {@code setterPrefixes} attribute
*/
@Override
List setterPrefixes() {
InitShim shim = this.initShim;
return shim != null
? shim.setterPrefixes()
: this.setterPrefixes;
}
/**
* Copy the current immutable object with elements that replace the content of {@link ExecutableElementHelperImpl#getterPrefixes() getterPrefixes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ExecutableElementHelperImplBuilder withGetterPrefixes(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ExecutableElementHelperImplBuilder(newValue, this.setterPrefixes);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ExecutableElementHelperImpl#getterPrefixes() getterPrefixes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of getterPrefixes elements to set
* @return A modified copy of {@code this} object
*/
public final ExecutableElementHelperImplBuilder withGetterPrefixes(Iterable elements) {
if (this.getterPrefixes == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ExecutableElementHelperImplBuilder(newValue, this.setterPrefixes);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ExecutableElementHelperImpl#setterPrefixes() setterPrefixes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ExecutableElementHelperImplBuilder withSetterPrefixes(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ExecutableElementHelperImplBuilder(this.getterPrefixes, newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ExecutableElementHelperImpl#setterPrefixes() setterPrefixes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of setterPrefixes elements to set
* @return A modified copy of {@code this} object
*/
public final ExecutableElementHelperImplBuilder withSetterPrefixes(Iterable elements) {
if (this.setterPrefixes == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ExecutableElementHelperImplBuilder(this.getterPrefixes, newValue);
}
/**
* This instance is equal to all instances of {@code ExecutableElementHelperImplBuilder} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof ExecutableElementHelperImplBuilder
&& equalTo((ExecutableElementHelperImplBuilder) another);
}
private boolean equalTo(ExecutableElementHelperImplBuilder another) {
return getterPrefixes.equals(another.getterPrefixes)
&& setterPrefixes.equals(another.setterPrefixes);
}
/**
* Computes a hash code from attributes: {@code getterPrefixes}, {@code setterPrefixes}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + getterPrefixes.hashCode();
h += (h << 5) + setterPrefixes.hashCode();
return h;
}
/**
* Prints the immutable value {@code ExecutableElementHelperImpl} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "ExecutableElementHelperImpl{"
+ "getterPrefixes=" + getterPrefixes
+ ", setterPrefixes=" + setterPrefixes
+ "}";
}
private volatile long lazyInitBitmap;
private static final long COMPILED_SETTER_PREFIXES_LAZY_INIT_BIT = 0x1L;
private List compiledSetterPrefixes;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link ExecutableElementHelperImpl#compiledSetterPrefixes() compiledSetterPrefixes} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* @return A lazily initialized value of the {@code l.name} attribute
*/
@Override
public List compiledSetterPrefixes() {
if ((lazyInitBitmap & COMPILED_SETTER_PREFIXES_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & COMPILED_SETTER_PREFIXES_LAZY_INIT_BIT) == 0) {
this.compiledSetterPrefixes = Objects.requireNonNull(super.compiledSetterPrefixes(), "compiledSetterPrefixes");
lazyInitBitmap |= COMPILED_SETTER_PREFIXES_LAZY_INIT_BIT;
}
}
}
return compiledSetterPrefixes;
}
private static final long COMPILED_GETTER_PREFIXES_LAZY_INIT_BIT = 0x2L;
private List compiledGetterPrefixes;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link ExecutableElementHelperImpl#compiledGetterPrefixes() compiledGetterPrefixes} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* @return A lazily initialized value of the {@code l.name} attribute
*/
@Override
public List compiledGetterPrefixes() {
if ((lazyInitBitmap & COMPILED_GETTER_PREFIXES_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & COMPILED_GETTER_PREFIXES_LAZY_INIT_BIT) == 0) {
this.compiledGetterPrefixes = Objects.requireNonNull(super.compiledGetterPrefixes(), "compiledGetterPrefixes");
lazyInitBitmap |= COMPILED_GETTER_PREFIXES_LAZY_INIT_BIT;
}
}
}
return compiledGetterPrefixes;
}
/**
* Creates an immutable copy of a {@link ExecutableElementHelperImpl} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ExecutableElementHelperImpl instance
*/
public static ExecutableElementHelperImplBuilder copyOf(ExecutableElementHelperImpl instance) {
if (instance instanceof ExecutableElementHelperImplBuilder) {
return (ExecutableElementHelperImplBuilder) instance;
}
return ExecutableElementHelperImplBuilder.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ExecutableElementHelperImplBuilder ExecutableElementHelperImplBuilder}.
* @return A new ExecutableElementHelperImplBuilder builder
*/
public static ExecutableElementHelperImplBuilder.Builder builder() {
return new ExecutableElementHelperImplBuilder.Builder();
}
/**
* Builds instances of type {@link ExecutableElementHelperImplBuilder ExecutableElementHelperImplBuilder}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long OPT_BIT_GETTER_PREFIXES = 0x1L;
private static final long OPT_BIT_SETTER_PREFIXES = 0x2L;
private long optBits;
private List getterPrefixes = new ArrayList();
private List setterPrefixes = new ArrayList();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ExecutableElementHelperImpl} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(ExecutableElementHelperImpl instance) {
Objects.requireNonNull(instance, "instance");
addAllGetterPrefixes(instance.getterPrefixes());
addAllSetterPrefixes(instance.setterPrefixes());
return this;
}
/**
* Adds one element to {@link ExecutableElementHelperImpl#getterPrefixes() getterPrefixes} list.
* @param element A getterPrefixes element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addGetterPrefixes(String element) {
this.getterPrefixes.add(Objects.requireNonNull(element, "getterPrefixes element"));
optBits |= OPT_BIT_GETTER_PREFIXES;
return this;
}
/**
* Adds elements to {@link ExecutableElementHelperImpl#getterPrefixes() getterPrefixes} list.
* @param elements An array of getterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addGetterPrefixes(String... elements) {
for (String element : elements) {
this.getterPrefixes.add(Objects.requireNonNull(element, "getterPrefixes element"));
}
optBits |= OPT_BIT_GETTER_PREFIXES;
return this;
}
/**
* Sets or replaces all elements for {@link ExecutableElementHelperImpl#getterPrefixes() getterPrefixes} list.
* @param elements An iterable of getterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder getterPrefixes(Iterable elements) {
this.getterPrefixes.clear();
return addAllGetterPrefixes(elements);
}
/**
* Adds elements to {@link ExecutableElementHelperImpl#getterPrefixes() getterPrefixes} list.
* @param elements An iterable of getterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllGetterPrefixes(Iterable elements) {
for (String element : elements) {
this.getterPrefixes.add(Objects.requireNonNull(element, "getterPrefixes element"));
}
optBits |= OPT_BIT_GETTER_PREFIXES;
return this;
}
/**
* Adds one element to {@link ExecutableElementHelperImpl#setterPrefixes() setterPrefixes} list.
* @param element A setterPrefixes element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addSetterPrefixes(String element) {
this.setterPrefixes.add(Objects.requireNonNull(element, "setterPrefixes element"));
optBits |= OPT_BIT_SETTER_PREFIXES;
return this;
}
/**
* Adds elements to {@link ExecutableElementHelperImpl#setterPrefixes() setterPrefixes} list.
* @param elements An array of setterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addSetterPrefixes(String... elements) {
for (String element : elements) {
this.setterPrefixes.add(Objects.requireNonNull(element, "setterPrefixes element"));
}
optBits |= OPT_BIT_SETTER_PREFIXES;
return this;
}
/**
* Sets or replaces all elements for {@link ExecutableElementHelperImpl#setterPrefixes() setterPrefixes} list.
* @param elements An iterable of setterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setterPrefixes(Iterable elements) {
this.setterPrefixes.clear();
return addAllSetterPrefixes(elements);
}
/**
* Adds elements to {@link ExecutableElementHelperImpl#setterPrefixes() setterPrefixes} list.
* @param elements An iterable of setterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllSetterPrefixes(Iterable elements) {
for (String element : elements) {
this.setterPrefixes.add(Objects.requireNonNull(element, "setterPrefixes element"));
}
optBits |= OPT_BIT_SETTER_PREFIXES;
return this;
}
/**
* Builds a new {@link ExecutableElementHelperImplBuilder ExecutableElementHelperImplBuilder}.
* @return An immutable instance of ExecutableElementHelperImpl
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ExecutableElementHelperImplBuilder build() {
return new ExecutableElementHelperImplBuilder(this);
}
private boolean getterPrefixesIsSet() {
return (optBits & OPT_BIT_GETTER_PREFIXES) != 0;
}
private boolean setterPrefixesIsSet() {
return (optBits & OPT_BIT_SETTER_PREFIXES) != 0;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList();
} else {
list = new ArrayList();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}