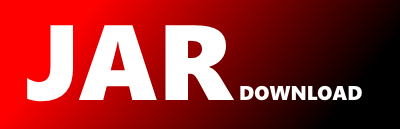
dz.jtsgen.processor.jtp.info.TSProcessingInfoBuilder Maven / Gradle / Ivy
Show all versions of jtsgen-processor Show documentation
package dz.jtsgen.processor.jtp.info;
import dz.jtsgen.processor.mapper.name.NameMapper;
import dz.jtsgen.processor.model.TSTargetType;
import dz.jtsgen.processor.model.TypeScriptModel;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import javax.annotation.Generated;
import javax.annotation.processing.ProcessingEnvironment;
import javax.lang.model.element.Element;
/**
* Immutable implementation of {@link TSProcessingInfo}.
*
* Use the builder to create immutable instances:
* {@code TSProcessingInfoBuilder.builder()}.
* Use the static factory method to create immutable instances:
* {@code TSProcessingInfoBuilder.of()}.
*/
@SuppressWarnings({"all"})
@Generated({"Immutables.generator", "TSProcessingInfo"})
public final class TSProcessingInfoBuilder extends TSProcessingInfo {
private final ProcessingEnvironment getpEnv;
private final TypeScriptModel tsModel;
private TSProcessingInfoBuilder(
ProcessingEnvironment getpEnv,
TypeScriptModel tsModel) {
this.getpEnv = Objects.requireNonNull(getpEnv, "getpEnv");
this.tsModel = Objects.requireNonNull(tsModel, "tsModel");
}
private TSProcessingInfoBuilder(
TSProcessingInfoBuilder original,
ProcessingEnvironment getpEnv,
TypeScriptModel tsModel) {
this.getpEnv = getpEnv;
this.tsModel = tsModel;
}
/**
* @return The value of the {@code getpEnv} attribute
*/
@Override
public ProcessingEnvironment getpEnv() {
return getpEnv;
}
/**
* @return The value of the {@code tsModel} attribute
*/
@Override
public TypeScriptModel getTsModel() {
return tsModel;
}
/**
* Copy the current immutable object by setting a value for the {@link TSProcessingInfo#getpEnv() getpEnv} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for getpEnv
* @return A modified copy of the {@code this} object
*/
public final TSProcessingInfoBuilder withGetpEnv(ProcessingEnvironment value) {
if (this.getpEnv == value) return this;
ProcessingEnvironment newValue = Objects.requireNonNull(value, "getpEnv");
return new TSProcessingInfoBuilder(this, newValue, this.tsModel);
}
/**
* Copy the current immutable object by setting a value for the {@link TSProcessingInfo#getTsModel() tsModel} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for tsModel
* @return A modified copy of the {@code this} object
*/
public final TSProcessingInfoBuilder withTsModel(TypeScriptModel value) {
if (this.tsModel == value) return this;
TypeScriptModel newValue = Objects.requireNonNull(value, "tsModel");
return new TSProcessingInfoBuilder(this, this.getpEnv, newValue);
}
/**
* This instance is equal to all instances of {@code TSProcessingInfoBuilder} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof TSProcessingInfoBuilder
&& equalTo((TSProcessingInfoBuilder) another);
}
private boolean equalTo(TSProcessingInfoBuilder another) {
return getpEnv.equals(another.getpEnv)
&& tsModel.equals(another.tsModel);
}
/**
* Computes a hash code from attributes: {@code getpEnv}, {@code tsModel}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + getpEnv.hashCode();
h += (h << 5) + tsModel.hashCode();
return h;
}
/**
* Prints the immutable value {@code TSProcessingInfo} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "TSProcessingInfo{"
+ "getpEnv=" + getpEnv
+ ", tsModel=" + tsModel
+ "}";
}
private volatile long lazyInitBitmap;
private static final long ELEMENT_CACHE_LAZY_INIT_BIT = 0x1L;
private TypeElementCache elementCache;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link TSProcessingInfo#elementCache() elementCache} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* @return A lazily initialized value of the {@code l.name} attribute
*/
@Override
public TypeElementCache elementCache() {
if ((lazyInitBitmap & ELEMENT_CACHE_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & ELEMENT_CACHE_LAZY_INIT_BIT) == 0) {
this.elementCache = Objects.requireNonNull(super.elementCache(), "elementCache");
lazyInitBitmap |= ELEMENT_CACHE_LAZY_INIT_BIT;
}
}
}
return elementCache;
}
private static final long ADDITIONAL_TYPES_TO_CONVERT_LAZY_INIT_BIT = 0x2L;
private Set additionalTypesToConvert;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link TSProcessingInfo#additionalTypesToConvert() additionalTypesToConvert} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* @return A lazily initialized value of the {@code l.name} attribute
*/
@Override
public Set additionalTypesToConvert() {
if ((lazyInitBitmap & ADDITIONAL_TYPES_TO_CONVERT_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & ADDITIONAL_TYPES_TO_CONVERT_LAZY_INIT_BIT) == 0) {
this.additionalTypesToConvert = Objects.requireNonNull(super.additionalTypesToConvert(), "additionalTypesToConvert");
lazyInitBitmap |= ADDITIONAL_TYPES_TO_CONVERT_LAZY_INIT_BIT;
}
}
}
return additionalTypesToConvert;
}
private static final long EXECUTABLE_HELPER_LAZY_INIT_BIT = 0x4L;
private ExecutableElementHelper executableHelper;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link TSProcessingInfo#executableHelper() executableHelper} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* @return A lazily initialized value of the {@code l.name} attribute
*/
@Override
public ExecutableElementHelper executableHelper() {
if ((lazyInitBitmap & EXECUTABLE_HELPER_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & EXECUTABLE_HELPER_LAZY_INIT_BIT) == 0) {
this.executableHelper = Objects.requireNonNull(super.executableHelper(), "executableHelper");
lazyInitBitmap |= EXECUTABLE_HELPER_LAZY_INIT_BIT;
}
}
}
return executableHelper;
}
private static final long NAME_MAPPER_LAZY_INIT_BIT = 0x8L;
private NameMapper nameMapper;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link TSProcessingInfo#nameMapper() nameMapper} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* @return A lazily initialized value of the {@code l.name} attribute
*/
@Override
public NameMapper nameMapper() {
if ((lazyInitBitmap & NAME_MAPPER_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & NAME_MAPPER_LAZY_INIT_BIT) == 0) {
this.nameMapper = Objects.requireNonNull(super.nameMapper(), "nameMapper");
lazyInitBitmap |= NAME_MAPPER_LAZY_INIT_BIT;
}
}
}
return nameMapper;
}
private static final long DECLARED_TYPE_CONVERSIONS_LAZY_INIT_BIT = 0x10L;
private Map declaredTypeConversions;
/**
* {@inheritDoc}
*
* Returns a lazily initialized value of the {@link TSProcessingInfo#declaredTypeConversions() declaredTypeConversions} attribute.
* Initialized once and only once and stored for subsequent access with proper synchronization.
* @return A lazily initialized value of the {@code l.name} attribute
*/
@Override
public Map declaredTypeConversions() {
if ((lazyInitBitmap & DECLARED_TYPE_CONVERSIONS_LAZY_INIT_BIT) == 0) {
synchronized (this) {
if ((lazyInitBitmap & DECLARED_TYPE_CONVERSIONS_LAZY_INIT_BIT) == 0) {
this.declaredTypeConversions = Objects.requireNonNull(super.declaredTypeConversions(), "declaredTypeConversions");
lazyInitBitmap |= DECLARED_TYPE_CONVERSIONS_LAZY_INIT_BIT;
}
}
}
return declaredTypeConversions;
}
/**
* Construct a new immutable {@code TSProcessingInfo} instance.
* @param getpEnv The value for the {@code getpEnv} attribute
* @param tsModel The value for the {@code tsModel} attribute
* @return An immutable TSProcessingInfo instance
*/
public static TSProcessingInfoBuilder of(ProcessingEnvironment getpEnv, TypeScriptModel tsModel) {
return new TSProcessingInfoBuilder(getpEnv, tsModel);
}
/**
* Creates an immutable copy of a {@link TSProcessingInfo} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable TSProcessingInfo instance
*/
public static TSProcessingInfoBuilder copyOf(TSProcessingInfo instance) {
if (instance instanceof TSProcessingInfoBuilder) {
return (TSProcessingInfoBuilder) instance;
}
return TSProcessingInfoBuilder.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link TSProcessingInfoBuilder TSProcessingInfoBuilder}.
* @return A new TSProcessingInfoBuilder builder
*/
public static TSProcessingInfoBuilder.Builder builder() {
return new TSProcessingInfoBuilder.Builder();
}
/**
* Builds instances of type {@link TSProcessingInfoBuilder TSProcessingInfoBuilder}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_GETP_ENV = 0x1L;
private static final long INIT_BIT_TS_MODEL = 0x2L;
private long initBits = 0x3L;
private ProcessingEnvironment getpEnv;
private TypeScriptModel tsModel;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code TSProcessingInfo} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(TSProcessingInfo instance) {
Objects.requireNonNull(instance, "instance");
getpEnv(instance.getpEnv());
tsModel(instance.getTsModel());
return this;
}
/**
* Initializes the value for the {@link TSProcessingInfo#getpEnv() getpEnv} attribute.
* @param getpEnv The value for getpEnv
* @return {@code this} builder for use in a chained invocation
*/
public final Builder getpEnv(ProcessingEnvironment getpEnv) {
this.getpEnv = Objects.requireNonNull(getpEnv, "getpEnv");
initBits &= ~INIT_BIT_GETP_ENV;
return this;
}
/**
* Initializes the value for the {@link TSProcessingInfo#getTsModel() tsModel} attribute.
* @param tsModel The value for tsModel
* @return {@code this} builder for use in a chained invocation
*/
public final Builder tsModel(TypeScriptModel tsModel) {
this.tsModel = Objects.requireNonNull(tsModel, "tsModel");
initBits &= ~INIT_BIT_TS_MODEL;
return this;
}
/**
* Builds a new {@link TSProcessingInfoBuilder TSProcessingInfoBuilder}.
* @return An immutable instance of TSProcessingInfo
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public TSProcessingInfoBuilder build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new TSProcessingInfoBuilder(null, getpEnv, tsModel);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList();
if ((initBits & INIT_BIT_GETP_ENV) != 0) attributes.add("getpEnv");
if ((initBits & INIT_BIT_TS_MODEL) != 0) attributes.add("tsModel");
return "Cannot build TSProcessingInfo, some of required attributes are not set " + attributes;
}
}
}