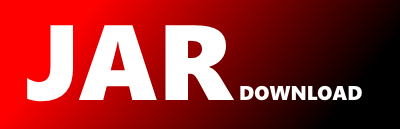
dz.jtsgen.processor.model.TSEnumBuilder Maven / Gradle / Ivy
Show all versions of jtsgen-processor Show documentation
package dz.jtsgen.processor.model;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Generated;
import javax.lang.model.element.Element;
/**
* Immutable implementation of {@link TSEnum}.
*
* Use the builder to create immutable instances:
* {@code TSEnumBuilder.builder()}.
* Use the static factory method to create immutable instances:
* {@code TSEnumBuilder.of()}.
*/
@SuppressWarnings({"all"})
@Generated({"Immutables.generator", "TSEnum"})
public final class TSEnumBuilder extends TSEnum {
private final String keyword;
private final String namespace;
private final String name;
private final List members;
private final String documentString;
private final List superTypes;
private final List typeParams;
private final Element element;
private TSEnumBuilder(Element element) {
this.element = Objects.requireNonNull(element, "element");
this.members = Collections.emptyList();
this.documentString = null;
this.superTypes = Collections.emptyList();
this.typeParams = Collections.emptyList();
this.keyword = initShim.getKeyword();
this.namespace = initShim.getNamespace();
this.name = initShim.getName();
this.initShim = null;
}
private TSEnumBuilder(TSEnumBuilder.Builder builder) {
this.members = createUnmodifiableList(true, builder.members);
this.documentString = builder.documentString;
this.superTypes = createUnmodifiableList(true, builder.superTypes);
this.typeParams = createUnmodifiableList(true, builder.typeParams);
this.element = builder.element;
if (builder.namespace != null) {
initShim.namespace(builder.namespace);
}
if (builder.name != null) {
initShim.name(builder.name);
}
this.namespace = initShim.getNamespace();
this.name = initShim.getName();
this.keyword = initShim.getKeyword();
this.initShim = null;
}
private TSEnumBuilder(
String namespace,
String name,
List members,
String documentString,
List superTypes,
List typeParams,
Element element) {
this.namespace = namespace;
this.name = name;
this.members = members;
this.documentString = documentString;
this.superTypes = superTypes;
this.typeParams = typeParams;
this.element = element;
initShim.namespace(this.namespace);
initShim.name(this.name);
this.keyword = initShim.getKeyword();
this.initShim = null;
}
private static final int STAGE_INITIALIZING = -1;
private static final int STAGE_UNINITIALIZED = 0;
private static final int STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
private final class InitShim {
private String keyword;
private int keywordBuildStage;
String getKeyword() {
if (keywordBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (keywordBuildStage == STAGE_UNINITIALIZED) {
keywordBuildStage = STAGE_INITIALIZING;
this.keyword = Objects.requireNonNull(TSEnumBuilder.super.getKeyword(), "keyword");
keywordBuildStage = STAGE_INITIALIZED;
}
return this.keyword;
}
private String namespace;
private int namespaceBuildStage;
String getNamespace() {
if (namespaceBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (namespaceBuildStage == STAGE_UNINITIALIZED) {
namespaceBuildStage = STAGE_INITIALIZING;
this.namespace = Objects.requireNonNull(TSEnumBuilder.super.getNamespace(), "namespace");
namespaceBuildStage = STAGE_INITIALIZED;
}
return this.namespace;
}
void namespace(String namespace) {
this.namespace = namespace;
namespaceBuildStage = STAGE_INITIALIZED;
}
private String name;
private int nameBuildStage;
String getName() {
if (nameBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (nameBuildStage == STAGE_UNINITIALIZED) {
nameBuildStage = STAGE_INITIALIZING;
this.name = Objects.requireNonNull(TSEnumBuilder.super.getName(), "name");
nameBuildStage = STAGE_INITIALIZED;
}
return this.name;
}
void name(String name) {
this.name = name;
nameBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
ArrayList attributes = new ArrayList();
if (keywordBuildStage == STAGE_INITIALIZING) attributes.add("keyword");
if (namespaceBuildStage == STAGE_INITIALIZING) attributes.add("namespace");
if (nameBuildStage == STAGE_INITIALIZING) attributes.add("name");
return "Cannot build TSEnum, attribute initializers form cycle" + attributes;
}
}
/**
* @return The computed-at-construction value of the {@code keyword} attribute
*/
@Override
public String getKeyword() {
InitShim shim = this.initShim;
return shim != null
? shim.getKeyword()
: this.keyword;
}
/**
* @return The value of the {@code namespace} attribute
*/
@Override
public String getNamespace() {
InitShim shim = this.initShim;
return shim != null
? shim.getNamespace()
: this.namespace;
}
/**
* @return The value of the {@code name} attribute
*/
@Override
public String getName() {
InitShim shim = this.initShim;
return shim != null
? shim.getName()
: this.name;
}
/**
* @return The value of the {@code members} attribute
*/
@Override
public List getMembers() {
return members;
}
/**
* @return The value of the {@code documentString} attribute
*/
@Override
public Optional getDocumentString() {
return Optional.ofNullable(documentString);
}
/**
* @return The value of the {@code superTypes} attribute
*/
@Override
public List getSuperTypes() {
return superTypes;
}
/**
* @return The value of the {@code typeParams} attribute
*/
@Override
public List getTypeParams() {
return typeParams;
}
/**
* @return The value of the {@code element} attribute
*/
@Override
public Element getElement() {
return element;
}
/**
* Copy the current immutable object by setting a value for the {@link TSEnum#getNamespace() namespace} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for namespace
* @return A modified copy of the {@code this} object
*/
public final TSEnumBuilder withNamespace(String value) {
if (this.namespace.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "namespace");
return new TSEnumBuilder(
newValue,
this.name,
this.members,
this.documentString,
this.superTypes,
this.typeParams,
this.element);
}
/**
* Copy the current immutable object by setting a value for the {@link TSEnum#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final TSEnumBuilder withName(String value) {
if (this.name.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "name");
return new TSEnumBuilder(
this.namespace,
newValue,
this.members,
this.documentString,
this.superTypes,
this.typeParams,
this.element);
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSEnum#getMembers() members}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final TSEnumBuilder withMembers(TSMember... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new TSEnumBuilder(
this.namespace,
this.name,
newValue,
this.documentString,
this.superTypes,
this.typeParams,
this.element);
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSEnum#getMembers() members}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of members elements to set
* @return A modified copy of {@code this} object
*/
public final TSEnumBuilder withMembers(Iterable extends TSMember> elements) {
if (this.members == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new TSEnumBuilder(
this.namespace,
this.name,
newValue,
this.documentString,
this.superTypes,
this.typeParams,
this.element);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TSEnum#getDocumentString() documentString} attribute.
* @param value The value for documentString
* @return A modified copy of {@code this} object
*/
public final TSEnumBuilder withDocumentString(String value) {
String newValue = Objects.requireNonNull(value, "documentString");
if (Objects.equals(this.documentString, newValue)) return this;
return new TSEnumBuilder(
this.namespace,
this.name,
this.members,
newValue,
this.superTypes,
this.typeParams,
this.element);
}
/**
* Copy the current immutable object by setting an optional value for the {@link TSEnum#getDocumentString() documentString} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for documentString
* @return A modified copy of {@code this} object
*/
public final TSEnumBuilder withDocumentString(Optional optional) {
String value = optional.orElse(null);
if (Objects.equals(this.documentString, value)) return this;
return new TSEnumBuilder(this.namespace, this.name, this.members, value, this.superTypes, this.typeParams, this.element);
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSEnum#getSuperTypes() superTypes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final TSEnumBuilder withSuperTypes(TSType... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new TSEnumBuilder(
this.namespace,
this.name,
this.members,
this.documentString,
newValue,
this.typeParams,
this.element);
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSEnum#getSuperTypes() superTypes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of superTypes elements to set
* @return A modified copy of {@code this} object
*/
public final TSEnumBuilder withSuperTypes(Iterable extends TSType> elements) {
if (this.superTypes == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new TSEnumBuilder(
this.namespace,
this.name,
this.members,
this.documentString,
newValue,
this.typeParams,
this.element);
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSEnum#getTypeParams() typeParams}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final TSEnumBuilder withTypeParams(TSTypeVariable... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new TSEnumBuilder(
this.namespace,
this.name,
this.members,
this.documentString,
this.superTypes,
newValue,
this.element);
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSEnum#getTypeParams() typeParams}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of typeParams elements to set
* @return A modified copy of {@code this} object
*/
public final TSEnumBuilder withTypeParams(Iterable extends TSTypeVariable> elements) {
if (this.typeParams == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new TSEnumBuilder(
this.namespace,
this.name,
this.members,
this.documentString,
this.superTypes,
newValue,
this.element);
}
/**
* Copy the current immutable object by setting a value for the {@link TSEnum#getElement() element} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for element
* @return A modified copy of the {@code this} object
*/
public final TSEnumBuilder withElement(Element value) {
if (this.element == value) return this;
Element newValue = Objects.requireNonNull(value, "element");
return new TSEnumBuilder(
this.namespace,
this.name,
this.members,
this.documentString,
this.superTypes,
this.typeParams,
newValue);
}
/**
* This instance is equal to all instances of {@code TSEnumBuilder} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof TSEnumBuilder
&& equalTo((TSEnumBuilder) another);
}
private boolean equalTo(TSEnumBuilder another) {
return keyword.equals(another.keyword)
&& namespace.equals(another.namespace)
&& name.equals(another.name)
&& members.equals(another.members)
&& Objects.equals(documentString, another.documentString)
&& superTypes.equals(another.superTypes)
&& typeParams.equals(another.typeParams)
&& element.equals(another.element);
}
/**
* Computes a hash code from attributes: {@code keyword}, {@code namespace}, {@code name}, {@code members}, {@code documentString}, {@code superTypes}, {@code typeParams}, {@code element}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + keyword.hashCode();
h += (h << 5) + namespace.hashCode();
h += (h << 5) + name.hashCode();
h += (h << 5) + members.hashCode();
h += (h << 5) + Objects.hashCode(documentString);
h += (h << 5) + superTypes.hashCode();
h += (h << 5) + typeParams.hashCode();
h += (h << 5) + element.hashCode();
return h;
}
/**
* Prints the immutable value {@code TSEnum} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("TSEnum{");
builder.append("keyword=").append(keyword);
builder.append(", ");
builder.append("namespace=").append(namespace);
builder.append(", ");
builder.append("name=").append(name);
builder.append(", ");
builder.append("members=").append(members);
if (documentString != null) {
builder.append(", ");
builder.append("documentString=").append(documentString);
}
builder.append(", ");
builder.append("superTypes=").append(superTypes);
builder.append(", ");
builder.append("typeParams=").append(typeParams);
builder.append(", ");
builder.append("element=").append(element);
return builder.append("}").toString();
}
/**
* Construct a new immutable {@code TSEnum} instance.
* @param element The value for the {@code element} attribute
* @return An immutable TSEnum instance
*/
public static TSEnumBuilder of(Element element) {
return new TSEnumBuilder(element);
}
/**
* Creates an immutable copy of a {@link TSEnum} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable TSEnum instance
*/
public static TSEnumBuilder copyOf(TSEnum instance) {
if (instance instanceof TSEnumBuilder) {
return (TSEnumBuilder) instance;
}
return TSEnumBuilder.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link TSEnumBuilder TSEnumBuilder}.
* @return A new TSEnumBuilder builder
*/
public static TSEnumBuilder.Builder builder() {
return new TSEnumBuilder.Builder();
}
/**
* Builds instances of type {@link TSEnumBuilder TSEnumBuilder}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long INIT_BIT_ELEMENT = 0x1L;
private long initBits = 0x1L;
private String namespace;
private String name;
private List members = new ArrayList();
private String documentString;
private List superTypes = new ArrayList();
private List typeParams = new ArrayList();
private Element element;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code dz.jtsgen.processor.model.TSType} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(TSType instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code dz.jtsgen.processor.model.TSEnum} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(TSEnum instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof TSType) {
TSType instance = (TSType) object;
addAllSuperTypes(instance.getSuperTypes());
Optional documentStringOptional = instance.getDocumentString();
if (documentStringOptional.isPresent()) {
documentString(documentStringOptional);
}
addAllMembers(instance.getMembers());
namespace(instance.getNamespace());
name(instance.getName());
addAllTypeParams(instance.getTypeParams());
element(instance.getElement());
}
}
/**
* Initializes the value for the {@link TSEnum#getNamespace() namespace} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link TSEnum#getNamespace() namespace}.
* @param namespace The value for namespace
* @return {@code this} builder for use in a chained invocation
*/
public final Builder namespace(String namespace) {
this.namespace = Objects.requireNonNull(namespace, "namespace");
return this;
}
/**
* Initializes the value for the {@link TSEnum#getName() name} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link TSEnum#getName() name}.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
return this;
}
/**
* Adds one element to {@link TSEnum#getMembers() members} list.
* @param element A members element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMembers(TSMember element) {
this.members.add(Objects.requireNonNull(element, "members element"));
return this;
}
/**
* Adds elements to {@link TSEnum#getMembers() members} list.
* @param elements An array of members elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addMembers(TSMember... elements) {
for (TSMember element : elements) {
this.members.add(Objects.requireNonNull(element, "members element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link TSEnum#getMembers() members} list.
* @param elements An iterable of members elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder members(Iterable extends TSMember> elements) {
this.members.clear();
return addAllMembers(elements);
}
/**
* Adds elements to {@link TSEnum#getMembers() members} list.
* @param elements An iterable of members elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllMembers(Iterable extends TSMember> elements) {
for (TSMember element : elements) {
this.members.add(Objects.requireNonNull(element, "members element"));
}
return this;
}
/**
* Initializes the optional value {@link TSEnum#getDocumentString() documentString} to documentString.
* @param documentString The value for documentString
* @return {@code this} builder for chained invocation
*/
public final Builder documentString(String documentString) {
this.documentString = Objects.requireNonNull(documentString, "documentString");
return this;
}
/**
* Initializes the optional value {@link TSEnum#getDocumentString() documentString} to documentString.
* @param documentString The value for documentString
* @return {@code this} builder for use in a chained invocation
*/
public final Builder documentString(Optional documentString) {
this.documentString = documentString.orElse(null);
return this;
}
/**
* Adds one element to {@link TSEnum#getSuperTypes() superTypes} list.
* @param element A superTypes element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addSuperTypes(TSType element) {
this.superTypes.add(Objects.requireNonNull(element, "superTypes element"));
return this;
}
/**
* Adds elements to {@link TSEnum#getSuperTypes() superTypes} list.
* @param elements An array of superTypes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addSuperTypes(TSType... elements) {
for (TSType element : elements) {
this.superTypes.add(Objects.requireNonNull(element, "superTypes element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link TSEnum#getSuperTypes() superTypes} list.
* @param elements An iterable of superTypes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder superTypes(Iterable extends TSType> elements) {
this.superTypes.clear();
return addAllSuperTypes(elements);
}
/**
* Adds elements to {@link TSEnum#getSuperTypes() superTypes} list.
* @param elements An iterable of superTypes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllSuperTypes(Iterable extends TSType> elements) {
for (TSType element : elements) {
this.superTypes.add(Objects.requireNonNull(element, "superTypes element"));
}
return this;
}
/**
* Adds one element to {@link TSEnum#getTypeParams() typeParams} list.
* @param element A typeParams element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addTypeParams(TSTypeVariable element) {
this.typeParams.add(Objects.requireNonNull(element, "typeParams element"));
return this;
}
/**
* Adds elements to {@link TSEnum#getTypeParams() typeParams} list.
* @param elements An array of typeParams elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addTypeParams(TSTypeVariable... elements) {
for (TSTypeVariable element : elements) {
this.typeParams.add(Objects.requireNonNull(element, "typeParams element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link TSEnum#getTypeParams() typeParams} list.
* @param elements An iterable of typeParams elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder typeParams(Iterable extends TSTypeVariable> elements) {
this.typeParams.clear();
return addAllTypeParams(elements);
}
/**
* Adds elements to {@link TSEnum#getTypeParams() typeParams} list.
* @param elements An iterable of typeParams elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllTypeParams(Iterable extends TSTypeVariable> elements) {
for (TSTypeVariable element : elements) {
this.typeParams.add(Objects.requireNonNull(element, "typeParams element"));
}
return this;
}
/**
* Initializes the value for the {@link TSEnum#getElement() element} attribute.
* @param element The value for element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder element(Element element) {
this.element = Objects.requireNonNull(element, "element");
initBits &= ~INIT_BIT_ELEMENT;
return this;
}
/**
* Builds a new {@link TSEnumBuilder TSEnumBuilder}.
* @return An immutable instance of TSEnum
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public TSEnumBuilder build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new TSEnumBuilder(this);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList();
if ((initBits & INIT_BIT_ELEMENT) != 0) attributes.add("element");
return "Cannot build TSEnum, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList();
} else {
list = new ArrayList();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}