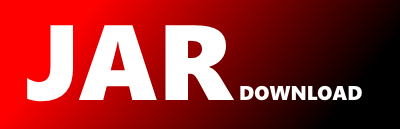
dz.jtsgen.processor.model.TSModuleInfoBuilder Maven / Gradle / Ivy
Show all versions of jtsgen-processor Show documentation
package dz.jtsgen.processor.model;
import dz.jtsgen.annotations.NameMappingStrategy;
import dz.jtsgen.annotations.NameSpaceMappingStrategy;
import dz.jtsgen.annotations.OutputType;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.regex.Pattern;
import javax.annotation.Generated;
/**
* Immutable implementation of {@link TSModuleInfo}.
*
* Use the builder to create immutable instances:
* {@code TSModuleInfoBuilder.builder()}.
*/
@SuppressWarnings({"all"})
@Generated({"Immutables.generator", "TSModuleInfo"})
public final class TSModuleInfoBuilder extends TSModuleInfo {
private final boolean getDefault;
private final String umdVariableName;
private final String moduleName;
private final String moduleDirectoryName;
private final String moduleVersion;
private final String moduleDescription;
private final String moduleAuthor;
private final String moduleLicense;
private final String moduleAuthorUrl;
private final Map customMappings;
private final String javaPackage;
private final List excludes;
private final List nameSpaceMappings;
private final OutputType outputType;
private final NameSpaceMappingStrategy nameSpaceMappingStrategy;
private final boolean generateTypeGuards;
private final List additionalTypes;
private final List getterPrefixes;
private final List setterPrefixes;
private final NameMappingStrategy nameMappingStrategy;
private TSModuleInfoBuilder(TSModuleInfoBuilder.Builder builder) {
this.umdVariableName = builder.umdVariableName;
this.javaPackage = builder.javaPackage;
if (builder.moduleName != null) {
initShim.moduleName(builder.moduleName);
}
if (builder.moduleVersion != null) {
initShim.moduleVersion(builder.moduleVersion);
}
if (builder.moduleDescription != null) {
initShim.moduleDescription(builder.moduleDescription);
}
if (builder.moduleAuthor != null) {
initShim.moduleAuthor(builder.moduleAuthor);
}
if (builder.moduleLicense != null) {
initShim.moduleLicense(builder.moduleLicense);
}
if (builder.moduleAuthorUrl != null) {
initShim.moduleAuthorUrl(builder.moduleAuthorUrl);
}
if (builder.customMappingsIsSet()) {
initShim.customMappings(createUnmodifiableMap(false, false, builder.customMappings));
}
if (builder.excludesIsSet()) {
initShim.excludes(createUnmodifiableList(true, builder.excludes));
}
if (builder.nameSpaceMappingsIsSet()) {
initShim.nameSpaceMappings(createUnmodifiableList(true, builder.nameSpaceMappings));
}
if (builder.outputType != null) {
initShim.outputType(builder.outputType);
}
if (builder.nameSpaceMappingStrategy != null) {
initShim.nameSpaceMappingStrategy(builder.nameSpaceMappingStrategy);
}
if (builder.generateTypeGuardsIsSet()) {
initShim.generateTypeGuards(builder.generateTypeGuards);
}
if (builder.additionalTypesIsSet()) {
initShim.additionalTypes(createUnmodifiableList(true, builder.additionalTypes));
}
if (builder.getterPrefixesIsSet()) {
initShim.getterPrefixes(createUnmodifiableList(true, builder.getterPrefixes));
}
if (builder.setterPrefixesIsSet()) {
initShim.setterPrefixes(createUnmodifiableList(true, builder.setterPrefixes));
}
if (builder.nameMappingStrategy != null) {
initShim.nameMappingStrategy(builder.nameMappingStrategy);
}
this.moduleName = initShim.getModuleName();
this.moduleVersion = initShim.getModuleVersion();
this.moduleDescription = initShim.getModuleDescription();
this.moduleAuthor = initShim.getModuleAuthor();
this.moduleLicense = initShim.getModuleLicense();
this.moduleAuthorUrl = initShim.getModuleAuthorUrl();
this.customMappings = initShim.getCustomMappings();
this.excludes = initShim.getExcludes();
this.nameSpaceMappings = initShim.getNameSpaceMappings();
this.outputType = initShim.getOutputType();
this.nameSpaceMappingStrategy = initShim.getNameSpaceMappingStrategy();
this.generateTypeGuards = initShim.generateTypeGuards();
this.additionalTypes = initShim.additionalTypes();
this.getterPrefixes = initShim.getterPrefixes();
this.setterPrefixes = initShim.setterPrefixes();
this.nameMappingStrategy = initShim.nameMappingStrategy();
this.getDefault = initShim.getDefault();
this.moduleDirectoryName = initShim.getModuleDirectoryName();
this.initShim = null;
}
private TSModuleInfoBuilder(
String umdVariableName,
String moduleName,
String moduleVersion,
String moduleDescription,
String moduleAuthor,
String moduleLicense,
String moduleAuthorUrl,
Map customMappings,
String javaPackage,
List excludes,
List nameSpaceMappings,
OutputType outputType,
NameSpaceMappingStrategy nameSpaceMappingStrategy,
boolean generateTypeGuards,
List additionalTypes,
List getterPrefixes,
List setterPrefixes,
NameMappingStrategy nameMappingStrategy) {
this.umdVariableName = umdVariableName;
this.moduleName = moduleName;
this.moduleVersion = moduleVersion;
this.moduleDescription = moduleDescription;
this.moduleAuthor = moduleAuthor;
this.moduleLicense = moduleLicense;
this.moduleAuthorUrl = moduleAuthorUrl;
this.customMappings = customMappings;
this.javaPackage = javaPackage;
this.excludes = excludes;
this.nameSpaceMappings = nameSpaceMappings;
this.outputType = outputType;
this.nameSpaceMappingStrategy = nameSpaceMappingStrategy;
this.generateTypeGuards = generateTypeGuards;
this.additionalTypes = additionalTypes;
this.getterPrefixes = getterPrefixes;
this.setterPrefixes = setterPrefixes;
this.nameMappingStrategy = nameMappingStrategy;
initShim.moduleName(this.moduleName);
initShim.moduleVersion(this.moduleVersion);
initShim.moduleDescription(this.moduleDescription);
initShim.moduleAuthor(this.moduleAuthor);
initShim.moduleLicense(this.moduleLicense);
initShim.moduleAuthorUrl(this.moduleAuthorUrl);
initShim.customMappings(this.customMappings);
initShim.excludes(this.excludes);
initShim.nameSpaceMappings(this.nameSpaceMappings);
initShim.outputType(this.outputType);
initShim.nameSpaceMappingStrategy(this.nameSpaceMappingStrategy);
initShim.generateTypeGuards(this.generateTypeGuards);
initShim.additionalTypes(this.additionalTypes);
initShim.getterPrefixes(this.getterPrefixes);
initShim.setterPrefixes(this.setterPrefixes);
initShim.nameMappingStrategy(this.nameMappingStrategy);
this.getDefault = initShim.getDefault();
this.moduleDirectoryName = initShim.getModuleDirectoryName();
this.initShim = null;
}
private static final int STAGE_INITIALIZING = -1;
private static final int STAGE_UNINITIALIZED = 0;
private static final int STAGE_INITIALIZED = 1;
private transient volatile InitShim initShim = new InitShim();
private final class InitShim {
private boolean getDefault;
private int getDefaultBuildStage;
boolean getDefault() {
if (getDefaultBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (getDefaultBuildStage == STAGE_UNINITIALIZED) {
getDefaultBuildStage = STAGE_INITIALIZING;
this.getDefault = TSModuleInfoBuilder.super.getDefault();
getDefaultBuildStage = STAGE_INITIALIZED;
}
return this.getDefault;
}
private String moduleName;
private int moduleNameBuildStage;
String getModuleName() {
if (moduleNameBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (moduleNameBuildStage == STAGE_UNINITIALIZED) {
moduleNameBuildStage = STAGE_INITIALIZING;
this.moduleName = Objects.requireNonNull(TSModuleInfoBuilder.super.getModuleName(), "moduleName");
moduleNameBuildStage = STAGE_INITIALIZED;
}
return this.moduleName;
}
void moduleName(String moduleName) {
this.moduleName = moduleName;
moduleNameBuildStage = STAGE_INITIALIZED;
}
private String moduleDirectoryName;
private int moduleDirectoryNameBuildStage;
String getModuleDirectoryName() {
if (moduleDirectoryNameBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (moduleDirectoryNameBuildStage == STAGE_UNINITIALIZED) {
moduleDirectoryNameBuildStage = STAGE_INITIALIZING;
this.moduleDirectoryName = Objects.requireNonNull(TSModuleInfoBuilder.super.getModuleDirectoryName(), "moduleDirectoryName");
moduleDirectoryNameBuildStage = STAGE_INITIALIZED;
}
return this.moduleDirectoryName;
}
private String moduleVersion;
private int moduleVersionBuildStage;
String getModuleVersion() {
if (moduleVersionBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (moduleVersionBuildStage == STAGE_UNINITIALIZED) {
moduleVersionBuildStage = STAGE_INITIALIZING;
this.moduleVersion = Objects.requireNonNull(TSModuleInfoBuilder.super.getModuleVersion(), "moduleVersion");
moduleVersionBuildStage = STAGE_INITIALIZED;
}
return this.moduleVersion;
}
void moduleVersion(String moduleVersion) {
this.moduleVersion = moduleVersion;
moduleVersionBuildStage = STAGE_INITIALIZED;
}
private String moduleDescription;
private int moduleDescriptionBuildStage;
String getModuleDescription() {
if (moduleDescriptionBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (moduleDescriptionBuildStage == STAGE_UNINITIALIZED) {
moduleDescriptionBuildStage = STAGE_INITIALIZING;
this.moduleDescription = Objects.requireNonNull(TSModuleInfoBuilder.super.getModuleDescription(), "moduleDescription");
moduleDescriptionBuildStage = STAGE_INITIALIZED;
}
return this.moduleDescription;
}
void moduleDescription(String moduleDescription) {
this.moduleDescription = moduleDescription;
moduleDescriptionBuildStage = STAGE_INITIALIZED;
}
private String moduleAuthor;
private int moduleAuthorBuildStage;
String getModuleAuthor() {
if (moduleAuthorBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (moduleAuthorBuildStage == STAGE_UNINITIALIZED) {
moduleAuthorBuildStage = STAGE_INITIALIZING;
this.moduleAuthor = Objects.requireNonNull(TSModuleInfoBuilder.super.getModuleAuthor(), "moduleAuthor");
moduleAuthorBuildStage = STAGE_INITIALIZED;
}
return this.moduleAuthor;
}
void moduleAuthor(String moduleAuthor) {
this.moduleAuthor = moduleAuthor;
moduleAuthorBuildStage = STAGE_INITIALIZED;
}
private String moduleLicense;
private int moduleLicenseBuildStage;
String getModuleLicense() {
if (moduleLicenseBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (moduleLicenseBuildStage == STAGE_UNINITIALIZED) {
moduleLicenseBuildStage = STAGE_INITIALIZING;
this.moduleLicense = Objects.requireNonNull(TSModuleInfoBuilder.super.getModuleLicense(), "moduleLicense");
moduleLicenseBuildStage = STAGE_INITIALIZED;
}
return this.moduleLicense;
}
void moduleLicense(String moduleLicense) {
this.moduleLicense = moduleLicense;
moduleLicenseBuildStage = STAGE_INITIALIZED;
}
private String moduleAuthorUrl;
private int moduleAuthorUrlBuildStage;
String getModuleAuthorUrl() {
if (moduleAuthorUrlBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (moduleAuthorUrlBuildStage == STAGE_UNINITIALIZED) {
moduleAuthorUrlBuildStage = STAGE_INITIALIZING;
this.moduleAuthorUrl = Objects.requireNonNull(TSModuleInfoBuilder.super.getModuleAuthorUrl(), "moduleAuthorUrl");
moduleAuthorUrlBuildStage = STAGE_INITIALIZED;
}
return this.moduleAuthorUrl;
}
void moduleAuthorUrl(String moduleAuthorUrl) {
this.moduleAuthorUrl = moduleAuthorUrl;
moduleAuthorUrlBuildStage = STAGE_INITIALIZED;
}
private Map customMappings;
private int customMappingsBuildStage;
Map getCustomMappings() {
if (customMappingsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (customMappingsBuildStage == STAGE_UNINITIALIZED) {
customMappingsBuildStage = STAGE_INITIALIZING;
this.customMappings = createUnmodifiableMap(true, false, TSModuleInfoBuilder.super.getCustomMappings());
customMappingsBuildStage = STAGE_INITIALIZED;
}
return this.customMappings;
}
void customMappings(Map customMappings) {
this.customMappings = customMappings;
customMappingsBuildStage = STAGE_INITIALIZED;
}
private List excludes;
private int excludesBuildStage;
List getExcludes() {
if (excludesBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (excludesBuildStage == STAGE_UNINITIALIZED) {
excludesBuildStage = STAGE_INITIALIZING;
this.excludes = createUnmodifiableList(false, createSafeList(TSModuleInfoBuilder.super.getExcludes(), true, false));
excludesBuildStage = STAGE_INITIALIZED;
}
return this.excludes;
}
void excludes(List excludes) {
this.excludes = excludes;
excludesBuildStage = STAGE_INITIALIZED;
}
private List nameSpaceMappings;
private int nameSpaceMappingsBuildStage;
List getNameSpaceMappings() {
if (nameSpaceMappingsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (nameSpaceMappingsBuildStage == STAGE_UNINITIALIZED) {
nameSpaceMappingsBuildStage = STAGE_INITIALIZING;
this.nameSpaceMappings = createUnmodifiableList(false, createSafeList(TSModuleInfoBuilder.super.getNameSpaceMappings(), true, false));
nameSpaceMappingsBuildStage = STAGE_INITIALIZED;
}
return this.nameSpaceMappings;
}
void nameSpaceMappings(List nameSpaceMappings) {
this.nameSpaceMappings = nameSpaceMappings;
nameSpaceMappingsBuildStage = STAGE_INITIALIZED;
}
private OutputType outputType;
private int outputTypeBuildStage;
OutputType getOutputType() {
if (outputTypeBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (outputTypeBuildStage == STAGE_UNINITIALIZED) {
outputTypeBuildStage = STAGE_INITIALIZING;
this.outputType = Objects.requireNonNull(TSModuleInfoBuilder.super.getOutputType(), "outputType");
outputTypeBuildStage = STAGE_INITIALIZED;
}
return this.outputType;
}
void outputType(OutputType outputType) {
this.outputType = outputType;
outputTypeBuildStage = STAGE_INITIALIZED;
}
private NameSpaceMappingStrategy nameSpaceMappingStrategy;
private int nameSpaceMappingStrategyBuildStage;
NameSpaceMappingStrategy getNameSpaceMappingStrategy() {
if (nameSpaceMappingStrategyBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (nameSpaceMappingStrategyBuildStage == STAGE_UNINITIALIZED) {
nameSpaceMappingStrategyBuildStage = STAGE_INITIALIZING;
this.nameSpaceMappingStrategy = Objects.requireNonNull(TSModuleInfoBuilder.super.getNameSpaceMappingStrategy(), "nameSpaceMappingStrategy");
nameSpaceMappingStrategyBuildStage = STAGE_INITIALIZED;
}
return this.nameSpaceMappingStrategy;
}
void nameSpaceMappingStrategy(NameSpaceMappingStrategy nameSpaceMappingStrategy) {
this.nameSpaceMappingStrategy = nameSpaceMappingStrategy;
nameSpaceMappingStrategyBuildStage = STAGE_INITIALIZED;
}
private boolean generateTypeGuards;
private int generateTypeGuardsBuildStage;
boolean generateTypeGuards() {
if (generateTypeGuardsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (generateTypeGuardsBuildStage == STAGE_UNINITIALIZED) {
generateTypeGuardsBuildStage = STAGE_INITIALIZING;
this.generateTypeGuards = TSModuleInfoBuilder.super.generateTypeGuards();
generateTypeGuardsBuildStage = STAGE_INITIALIZED;
}
return this.generateTypeGuards;
}
void generateTypeGuards(boolean generateTypeGuards) {
this.generateTypeGuards = generateTypeGuards;
generateTypeGuardsBuildStage = STAGE_INITIALIZED;
}
private List additionalTypes;
private int additionalTypesBuildStage;
List additionalTypes() {
if (additionalTypesBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (additionalTypesBuildStage == STAGE_UNINITIALIZED) {
additionalTypesBuildStage = STAGE_INITIALIZING;
this.additionalTypes = createUnmodifiableList(false, createSafeList(TSModuleInfoBuilder.super.additionalTypes(), true, false));
additionalTypesBuildStage = STAGE_INITIALIZED;
}
return this.additionalTypes;
}
void additionalTypes(List additionalTypes) {
this.additionalTypes = additionalTypes;
additionalTypesBuildStage = STAGE_INITIALIZED;
}
private List getterPrefixes;
private int getterPrefixesBuildStage;
List getterPrefixes() {
if (getterPrefixesBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (getterPrefixesBuildStage == STAGE_UNINITIALIZED) {
getterPrefixesBuildStage = STAGE_INITIALIZING;
this.getterPrefixes = createUnmodifiableList(false, createSafeList(TSModuleInfoBuilder.super.getterPrefixes(), true, false));
getterPrefixesBuildStage = STAGE_INITIALIZED;
}
return this.getterPrefixes;
}
void getterPrefixes(List getterPrefixes) {
this.getterPrefixes = getterPrefixes;
getterPrefixesBuildStage = STAGE_INITIALIZED;
}
private List setterPrefixes;
private int setterPrefixesBuildStage;
List setterPrefixes() {
if (setterPrefixesBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (setterPrefixesBuildStage == STAGE_UNINITIALIZED) {
setterPrefixesBuildStage = STAGE_INITIALIZING;
this.setterPrefixes = createUnmodifiableList(false, createSafeList(TSModuleInfoBuilder.super.setterPrefixes(), true, false));
setterPrefixesBuildStage = STAGE_INITIALIZED;
}
return this.setterPrefixes;
}
void setterPrefixes(List setterPrefixes) {
this.setterPrefixes = setterPrefixes;
setterPrefixesBuildStage = STAGE_INITIALIZED;
}
private NameMappingStrategy nameMappingStrategy;
private int nameMappingStrategyBuildStage;
NameMappingStrategy nameMappingStrategy() {
if (nameMappingStrategyBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (nameMappingStrategyBuildStage == STAGE_UNINITIALIZED) {
nameMappingStrategyBuildStage = STAGE_INITIALIZING;
this.nameMappingStrategy = Objects.requireNonNull(TSModuleInfoBuilder.super.nameMappingStrategy(), "nameMappingStrategy");
nameMappingStrategyBuildStage = STAGE_INITIALIZED;
}
return this.nameMappingStrategy;
}
void nameMappingStrategy(NameMappingStrategy nameMappingStrategy) {
this.nameMappingStrategy = nameMappingStrategy;
nameMappingStrategyBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
ArrayList attributes = new ArrayList();
if (getDefaultBuildStage == STAGE_INITIALIZING) attributes.add("getDefault");
if (moduleNameBuildStage == STAGE_INITIALIZING) attributes.add("moduleName");
if (moduleDirectoryNameBuildStage == STAGE_INITIALIZING) attributes.add("moduleDirectoryName");
if (moduleVersionBuildStage == STAGE_INITIALIZING) attributes.add("moduleVersion");
if (moduleDescriptionBuildStage == STAGE_INITIALIZING) attributes.add("moduleDescription");
if (moduleAuthorBuildStage == STAGE_INITIALIZING) attributes.add("moduleAuthor");
if (moduleLicenseBuildStage == STAGE_INITIALIZING) attributes.add("moduleLicense");
if (moduleAuthorUrlBuildStage == STAGE_INITIALIZING) attributes.add("moduleAuthorUrl");
if (customMappingsBuildStage == STAGE_INITIALIZING) attributes.add("customMappings");
if (excludesBuildStage == STAGE_INITIALIZING) attributes.add("excludes");
if (nameSpaceMappingsBuildStage == STAGE_INITIALIZING) attributes.add("nameSpaceMappings");
if (outputTypeBuildStage == STAGE_INITIALIZING) attributes.add("outputType");
if (nameSpaceMappingStrategyBuildStage == STAGE_INITIALIZING) attributes.add("nameSpaceMappingStrategy");
if (generateTypeGuardsBuildStage == STAGE_INITIALIZING) attributes.add("generateTypeGuards");
if (additionalTypesBuildStage == STAGE_INITIALIZING) attributes.add("additionalTypes");
if (getterPrefixesBuildStage == STAGE_INITIALIZING) attributes.add("getterPrefixes");
if (setterPrefixesBuildStage == STAGE_INITIALIZING) attributes.add("setterPrefixes");
if (nameMappingStrategyBuildStage == STAGE_INITIALIZING) attributes.add("nameMappingStrategy");
return "Cannot build TSModuleInfo, attribute initializers form cycle" + attributes;
}
}
/**
* @return is Module is default module
*/
@Override
public boolean getDefault() {
InitShim shim = this.initShim;
return shim != null
? shim.getDefault()
: this.getDefault;
}
/**
* @return The value of the {@code umdVariableName} attribute
*/
@Override
public Optional getUmdVariableName() {
return Optional.ofNullable(umdVariableName);
}
/**
* the module name, package name friendly, that appears also in the declared namespace
* @return the module name
*/
@Override
public String getModuleName() {
InitShim shim = this.initShim;
return shim != null
? shim.getModuleName()
: this.moduleName;
}
/**
* @return the module name for package.json, this must also be package name friendly, because the java compiler
* only accepts packages names for sub dirs (as ressource)
*/
@Override
public String getModuleDirectoryName() {
InitShim shim = this.initShim;
return shim != null
? shim.getModuleDirectoryName()
: this.moduleDirectoryName;
}
/**
* @return The value of the {@code moduleVersion} attribute
*/
@Override
public String getModuleVersion() {
InitShim shim = this.initShim;
return shim != null
? shim.getModuleVersion()
: this.moduleVersion;
}
/**
* @return The value of the {@code moduleDescription} attribute
*/
@Override
public String getModuleDescription() {
InitShim shim = this.initShim;
return shim != null
? shim.getModuleDescription()
: this.moduleDescription;
}
/**
* @return The value of the {@code moduleAuthor} attribute
*/
@Override
public String getModuleAuthor() {
InitShim shim = this.initShim;
return shim != null
? shim.getModuleAuthor()
: this.moduleAuthor;
}
/**
* @return The value of the {@code moduleLicense} attribute
*/
@Override
public String getModuleLicense() {
InitShim shim = this.initShim;
return shim != null
? shim.getModuleLicense()
: this.moduleLicense;
}
/**
* @return The value of the {@code moduleAuthorUrl} attribute
*/
@Override
public String getModuleAuthorUrl() {
InitShim shim = this.initShim;
return shim != null
? shim.getModuleAuthorUrl()
: this.moduleAuthorUrl;
}
/**
* @return The value of the {@code customMappings} attribute
*/
@Override
public Map getCustomMappings() {
InitShim shim = this.initShim;
return shim != null
? shim.getCustomMappings()
: this.customMappings;
}
/**
* @return The value of the {@code javaPackage} attribute
*/
@Override
public Optional getJavaPackage() {
return Optional.ofNullable(javaPackage);
}
/**
* @return The value of the {@code excludes} attribute
*/
@Override
public List getExcludes() {
InitShim shim = this.initShim;
return shim != null
? shim.getExcludes()
: this.excludes;
}
/**
* @return The value of the {@code nameSpaceMappings} attribute
*/
@Override
public List getNameSpaceMappings() {
InitShim shim = this.initShim;
return shim != null
? shim.getNameSpaceMappings()
: this.nameSpaceMappings;
}
/**
* @return The value of the {@code outputType} attribute
*/
@Override
public OutputType getOutputType() {
InitShim shim = this.initShim;
return shim != null
? shim.getOutputType()
: this.outputType;
}
/**
* @return The value of the {@code nameSpaceMappingStrategy} attribute
*/
@Override
public NameSpaceMappingStrategy getNameSpaceMappingStrategy() {
InitShim shim = this.initShim;
return shim != null
? shim.getNameSpaceMappingStrategy()
: this.nameSpaceMappingStrategy;
}
/**
* @return The value of the {@code generateTypeGuards} attribute
*/
@Override
public boolean generateTypeGuards() {
InitShim shim = this.initShim;
return shim != null
? shim.generateTypeGuards()
: this.generateTypeGuards;
}
/**
* @return The value of the {@code additionalTypes} attribute
*/
@Override
public List additionalTypes() {
InitShim shim = this.initShim;
return shim != null
? shim.additionalTypes()
: this.additionalTypes;
}
/**
* @return The value of the {@code getterPrefixes} attribute
*/
@Override
public List getterPrefixes() {
InitShim shim = this.initShim;
return shim != null
? shim.getterPrefixes()
: this.getterPrefixes;
}
/**
* @return The value of the {@code setterPrefixes} attribute
*/
@Override
public List setterPrefixes() {
InitShim shim = this.initShim;
return shim != null
? shim.setterPrefixes()
: this.setterPrefixes;
}
/**
* @return The value of the {@code nameMappingStrategy} attribute
*/
@Override
public NameMappingStrategy nameMappingStrategy() {
InitShim shim = this.initShim;
return shim != null
? shim.nameMappingStrategy()
: this.nameMappingStrategy;
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TSModuleInfo#getUmdVariableName() umdVariableName} attribute.
* @param value The value for umdVariableName
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withUmdVariableName(String value) {
String newValue = Objects.requireNonNull(value, "umdVariableName");
if (Objects.equals(this.umdVariableName, newValue)) return this;
return validate(new TSModuleInfoBuilder(
newValue,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting an optional value for the {@link TSModuleInfo#getUmdVariableName() umdVariableName} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for umdVariableName
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withUmdVariableName(Optional optional) {
String value = optional.orElse(null);
if (Objects.equals(this.umdVariableName, value)) return this;
return validate(new TSModuleInfoBuilder(
value,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting a value for the {@link TSModuleInfo#getModuleName() moduleName} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for moduleName
* @return A modified copy of the {@code this} object
*/
public final TSModuleInfoBuilder withModuleName(String value) {
if (this.moduleName.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "moduleName");
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
newValue,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting a value for the {@link TSModuleInfo#getModuleVersion() moduleVersion} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for moduleVersion
* @return A modified copy of the {@code this} object
*/
public final TSModuleInfoBuilder withModuleVersion(String value) {
if (this.moduleVersion.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "moduleVersion");
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
newValue,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting a value for the {@link TSModuleInfo#getModuleDescription() moduleDescription} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for moduleDescription
* @return A modified copy of the {@code this} object
*/
public final TSModuleInfoBuilder withModuleDescription(String value) {
if (this.moduleDescription.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "moduleDescription");
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
newValue,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting a value for the {@link TSModuleInfo#getModuleAuthor() moduleAuthor} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for moduleAuthor
* @return A modified copy of the {@code this} object
*/
public final TSModuleInfoBuilder withModuleAuthor(String value) {
if (this.moduleAuthor.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "moduleAuthor");
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
newValue,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting a value for the {@link TSModuleInfo#getModuleLicense() moduleLicense} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for moduleLicense
* @return A modified copy of the {@code this} object
*/
public final TSModuleInfoBuilder withModuleLicense(String value) {
if (this.moduleLicense.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "moduleLicense");
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
newValue,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting a value for the {@link TSModuleInfo#getModuleAuthorUrl() moduleAuthorUrl} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for moduleAuthorUrl
* @return A modified copy of the {@code this} object
*/
public final TSModuleInfoBuilder withModuleAuthorUrl(String value) {
if (this.moduleAuthorUrl.equals(value)) return this;
String newValue = Objects.requireNonNull(value, "moduleAuthorUrl");
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
newValue,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by replacing the {@link TSModuleInfo#getCustomMappings() customMappings} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the customMappings map
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withCustomMappings(Map entries) {
if (this.customMappings == entries) return this;
Map newValue = createUnmodifiableMap(true, false, entries);
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
newValue,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting a present value for the optional {@link TSModuleInfo#getJavaPackage() javaPackage} attribute.
* @param value The value for javaPackage
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withJavaPackage(String value) {
String newValue = Objects.requireNonNull(value, "javaPackage");
if (Objects.equals(this.javaPackage, newValue)) return this;
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
newValue,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting an optional value for the {@link TSModuleInfo#getJavaPackage() javaPackage} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for javaPackage
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withJavaPackage(Optional optional) {
String value = optional.orElse(null);
if (Objects.equals(this.javaPackage, value)) return this;
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
value,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSModuleInfo#getExcludes() excludes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withExcludes(Pattern... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
newValue,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSModuleInfo#getExcludes() excludes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of excludes elements to set
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withExcludes(Iterable extends Pattern> elements) {
if (this.excludes == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
newValue,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSModuleInfo#getNameSpaceMappings() nameSpaceMappings}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withNameSpaceMappings(NameSpaceMapping... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
newValue,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSModuleInfo#getNameSpaceMappings() nameSpaceMappings}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of nameSpaceMappings elements to set
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withNameSpaceMappings(Iterable extends NameSpaceMapping> elements) {
if (this.nameSpaceMappings == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
newValue,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting a value for the {@link TSModuleInfo#getOutputType() outputType} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for outputType
* @return A modified copy of the {@code this} object
*/
public final TSModuleInfoBuilder withOutputType(OutputType value) {
if (this.outputType == value) return this;
OutputType newValue = Objects.requireNonNull(value, "outputType");
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
newValue,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting a value for the {@link TSModuleInfo#getNameSpaceMappingStrategy() nameSpaceMappingStrategy} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for nameSpaceMappingStrategy
* @return A modified copy of the {@code this} object
*/
public final TSModuleInfoBuilder withNameSpaceMappingStrategy(NameSpaceMappingStrategy value) {
if (this.nameSpaceMappingStrategy == value) return this;
NameSpaceMappingStrategy newValue = Objects.requireNonNull(value, "nameSpaceMappingStrategy");
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
newValue,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting a value for the {@link TSModuleInfo#generateTypeGuards() generateTypeGuards} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for generateTypeGuards
* @return A modified copy of the {@code this} object
*/
public final TSModuleInfoBuilder withGenerateTypeGuards(boolean value) {
if (this.generateTypeGuards == value) return this;
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
value,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSModuleInfo#additionalTypes() additionalTypes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withAdditionalTypes(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
newValue,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSModuleInfo#additionalTypes() additionalTypes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of additionalTypes elements to set
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withAdditionalTypes(Iterable elements) {
if (this.additionalTypes == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
newValue,
this.getterPrefixes,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSModuleInfo#getterPrefixes() getterPrefixes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withGetterPrefixes(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
newValue,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSModuleInfo#getterPrefixes() getterPrefixes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of getterPrefixes elements to set
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withGetterPrefixes(Iterable elements) {
if (this.getterPrefixes == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
newValue,
this.setterPrefixes,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSModuleInfo#setterPrefixes() setterPrefixes}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withSetterPrefixes(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
newValue,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object with elements that replace the content of {@link TSModuleInfo#setterPrefixes() setterPrefixes}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of setterPrefixes elements to set
* @return A modified copy of {@code this} object
*/
public final TSModuleInfoBuilder withSetterPrefixes(Iterable elements) {
if (this.setterPrefixes == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
newValue,
this.nameMappingStrategy));
}
/**
* Copy the current immutable object by setting a value for the {@link TSModuleInfo#nameMappingStrategy() nameMappingStrategy} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for nameMappingStrategy
* @return A modified copy of the {@code this} object
*/
public final TSModuleInfoBuilder withNameMappingStrategy(NameMappingStrategy value) {
if (this.nameMappingStrategy == value) return this;
NameMappingStrategy newValue = Objects.requireNonNull(value, "nameMappingStrategy");
return validate(new TSModuleInfoBuilder(
this.umdVariableName,
this.moduleName,
this.moduleVersion,
this.moduleDescription,
this.moduleAuthor,
this.moduleLicense,
this.moduleAuthorUrl,
this.customMappings,
this.javaPackage,
this.excludes,
this.nameSpaceMappings,
this.outputType,
this.nameSpaceMappingStrategy,
this.generateTypeGuards,
this.additionalTypes,
this.getterPrefixes,
this.setterPrefixes,
newValue));
}
/**
* This instance is equal to all instances of {@code TSModuleInfoBuilder} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof TSModuleInfoBuilder
&& equalTo((TSModuleInfoBuilder) another);
}
private boolean equalTo(TSModuleInfoBuilder another) {
return getDefault == another.getDefault
&& Objects.equals(umdVariableName, another.umdVariableName)
&& moduleName.equals(another.moduleName)
&& moduleDirectoryName.equals(another.moduleDirectoryName)
&& moduleVersion.equals(another.moduleVersion)
&& moduleDescription.equals(another.moduleDescription)
&& moduleAuthor.equals(another.moduleAuthor)
&& moduleLicense.equals(another.moduleLicense)
&& moduleAuthorUrl.equals(another.moduleAuthorUrl)
&& customMappings.equals(another.customMappings)
&& Objects.equals(javaPackage, another.javaPackage)
&& excludes.equals(another.excludes)
&& nameSpaceMappings.equals(another.nameSpaceMappings)
&& outputType.equals(another.outputType)
&& nameSpaceMappingStrategy.equals(another.nameSpaceMappingStrategy)
&& generateTypeGuards == another.generateTypeGuards
&& additionalTypes.equals(another.additionalTypes)
&& getterPrefixes.equals(another.getterPrefixes)
&& setterPrefixes.equals(another.setterPrefixes)
&& nameMappingStrategy.equals(another.nameMappingStrategy);
}
/**
* Computes a hash code from attributes: {@code getDefault}, {@code umdVariableName}, {@code moduleName}, {@code moduleDirectoryName}, {@code moduleVersion}, {@code moduleDescription}, {@code moduleAuthor}, {@code moduleLicense}, {@code moduleAuthorUrl}, {@code customMappings}, {@code javaPackage}, {@code excludes}, {@code nameSpaceMappings}, {@code outputType}, {@code nameSpaceMappingStrategy}, {@code generateTypeGuards}, {@code additionalTypes}, {@code getterPrefixes}, {@code setterPrefixes}, {@code nameMappingStrategy}.
* @return hashCode value
*/
@Override
public int hashCode() {
int h = 5381;
h += (h << 5) + Boolean.hashCode(getDefault);
h += (h << 5) + Objects.hashCode(umdVariableName);
h += (h << 5) + moduleName.hashCode();
h += (h << 5) + moduleDirectoryName.hashCode();
h += (h << 5) + moduleVersion.hashCode();
h += (h << 5) + moduleDescription.hashCode();
h += (h << 5) + moduleAuthor.hashCode();
h += (h << 5) + moduleLicense.hashCode();
h += (h << 5) + moduleAuthorUrl.hashCode();
h += (h << 5) + customMappings.hashCode();
h += (h << 5) + Objects.hashCode(javaPackage);
h += (h << 5) + excludes.hashCode();
h += (h << 5) + nameSpaceMappings.hashCode();
h += (h << 5) + outputType.hashCode();
h += (h << 5) + nameSpaceMappingStrategy.hashCode();
h += (h << 5) + Boolean.hashCode(generateTypeGuards);
h += (h << 5) + additionalTypes.hashCode();
h += (h << 5) + getterPrefixes.hashCode();
h += (h << 5) + setterPrefixes.hashCode();
h += (h << 5) + nameMappingStrategy.hashCode();
return h;
}
/**
* Prints the immutable value {@code TSModuleInfo} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("TSModuleInfo{");
builder.append("default=").append(getDefault);
if (umdVariableName != null) {
builder.append(", ");
builder.append("umdVariableName=").append(umdVariableName);
}
builder.append(", ");
builder.append("moduleName=").append(moduleName);
builder.append(", ");
builder.append("moduleDirectoryName=").append(moduleDirectoryName);
builder.append(", ");
builder.append("moduleVersion=").append(moduleVersion);
builder.append(", ");
builder.append("moduleDescription=").append(moduleDescription);
builder.append(", ");
builder.append("moduleAuthor=").append(moduleAuthor);
builder.append(", ");
builder.append("moduleLicense=").append(moduleLicense);
builder.append(", ");
builder.append("moduleAuthorUrl=").append(moduleAuthorUrl);
builder.append(", ");
builder.append("customMappings=").append(customMappings);
if (javaPackage != null) {
builder.append(", ");
builder.append("javaPackage=").append(javaPackage);
}
builder.append(", ");
builder.append("excludes=").append(excludes);
builder.append(", ");
builder.append("nameSpaceMappings=").append(nameSpaceMappings);
builder.append(", ");
builder.append("outputType=").append(outputType);
builder.append(", ");
builder.append("nameSpaceMappingStrategy=").append(nameSpaceMappingStrategy);
builder.append(", ");
builder.append("generateTypeGuards=").append(generateTypeGuards);
builder.append(", ");
builder.append("additionalTypes=").append(additionalTypes);
builder.append(", ");
builder.append("getterPrefixes=").append(getterPrefixes);
builder.append(", ");
builder.append("setterPrefixes=").append(setterPrefixes);
builder.append(", ");
builder.append("nameMappingStrategy=").append(nameMappingStrategy);
return builder.append("}").toString();
}
private static TSModuleInfoBuilder validate(TSModuleInfoBuilder instance) {
instance.check();
return instance;
}
/**
* Creates an immutable copy of a {@link TSModuleInfo} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable TSModuleInfo instance
*/
public static TSModuleInfoBuilder copyOf(TSModuleInfo instance) {
if (instance instanceof TSModuleInfoBuilder) {
return (TSModuleInfoBuilder) instance;
}
return TSModuleInfoBuilder.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link TSModuleInfoBuilder TSModuleInfoBuilder}.
* @return A new TSModuleInfoBuilder builder
*/
public static TSModuleInfoBuilder.Builder builder() {
return new TSModuleInfoBuilder.Builder();
}
/**
* Builds instances of type {@link TSModuleInfoBuilder TSModuleInfoBuilder}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
public static final class Builder {
private static final long OPT_BIT_CUSTOM_MAPPINGS = 0x1L;
private static final long OPT_BIT_EXCLUDES = 0x2L;
private static final long OPT_BIT_NAME_SPACE_MAPPINGS = 0x4L;
private static final long OPT_BIT_GENERATE_TYPE_GUARDS = 0x8L;
private static final long OPT_BIT_ADDITIONAL_TYPES = 0x10L;
private static final long OPT_BIT_GETTER_PREFIXES = 0x20L;
private static final long OPT_BIT_SETTER_PREFIXES = 0x40L;
private long optBits;
private String umdVariableName;
private String moduleName;
private String moduleVersion;
private String moduleDescription;
private String moduleAuthor;
private String moduleLicense;
private String moduleAuthorUrl;
private Map customMappings = new LinkedHashMap();
private String javaPackage;
private List excludes = new ArrayList();
private List nameSpaceMappings = new ArrayList();
private OutputType outputType;
private NameSpaceMappingStrategy nameSpaceMappingStrategy;
private boolean generateTypeGuards;
private List additionalTypes = new ArrayList();
private List getterPrefixes = new ArrayList();
private List setterPrefixes = new ArrayList();
private NameMappingStrategy nameMappingStrategy;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code TSModuleInfo} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
public final Builder from(TSModuleInfo instance) {
Objects.requireNonNull(instance, "instance");
Optional umdVariableNameOptional = instance.getUmdVariableName();
if (umdVariableNameOptional.isPresent()) {
umdVariableName(umdVariableNameOptional);
}
moduleName(instance.getModuleName());
moduleVersion(instance.getModuleVersion());
moduleDescription(instance.getModuleDescription());
moduleAuthor(instance.getModuleAuthor());
moduleLicense(instance.getModuleLicense());
moduleAuthorUrl(instance.getModuleAuthorUrl());
putAllCustomMappings(instance.getCustomMappings());
Optional javaPackageOptional = instance.getJavaPackage();
if (javaPackageOptional.isPresent()) {
javaPackage(javaPackageOptional);
}
addAllExcludes(instance.getExcludes());
addAllNameSpaceMappings(instance.getNameSpaceMappings());
outputType(instance.getOutputType());
nameSpaceMappingStrategy(instance.getNameSpaceMappingStrategy());
generateTypeGuards(instance.generateTypeGuards());
addAllAdditionalTypes(instance.additionalTypes());
addAllGetterPrefixes(instance.getterPrefixes());
addAllSetterPrefixes(instance.setterPrefixes());
nameMappingStrategy(instance.nameMappingStrategy());
return this;
}
/**
* Initializes the optional value {@link TSModuleInfo#getUmdVariableName() umdVariableName} to umdVariableName.
* @param umdVariableName The value for umdVariableName
* @return {@code this} builder for chained invocation
*/
public final Builder umdVariableName(String umdVariableName) {
this.umdVariableName = Objects.requireNonNull(umdVariableName, "umdVariableName");
return this;
}
/**
* Initializes the optional value {@link TSModuleInfo#getUmdVariableName() umdVariableName} to umdVariableName.
* @param umdVariableName The value for umdVariableName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder umdVariableName(Optional umdVariableName) {
this.umdVariableName = umdVariableName.orElse(null);
return this;
}
/**
* Initializes the value for the {@link TSModuleInfo#getModuleName() moduleName} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link TSModuleInfo#getModuleName() moduleName}.
* @param moduleName The value for moduleName
* @return {@code this} builder for use in a chained invocation
*/
public final Builder moduleName(String moduleName) {
this.moduleName = Objects.requireNonNull(moduleName, "moduleName");
return this;
}
/**
* Initializes the value for the {@link TSModuleInfo#getModuleVersion() moduleVersion} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link TSModuleInfo#getModuleVersion() moduleVersion}.
* @param moduleVersion The value for moduleVersion
* @return {@code this} builder for use in a chained invocation
*/
public final Builder moduleVersion(String moduleVersion) {
this.moduleVersion = Objects.requireNonNull(moduleVersion, "moduleVersion");
return this;
}
/**
* Initializes the value for the {@link TSModuleInfo#getModuleDescription() moduleDescription} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link TSModuleInfo#getModuleDescription() moduleDescription}.
* @param moduleDescription The value for moduleDescription
* @return {@code this} builder for use in a chained invocation
*/
public final Builder moduleDescription(String moduleDescription) {
this.moduleDescription = Objects.requireNonNull(moduleDescription, "moduleDescription");
return this;
}
/**
* Initializes the value for the {@link TSModuleInfo#getModuleAuthor() moduleAuthor} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link TSModuleInfo#getModuleAuthor() moduleAuthor}.
* @param moduleAuthor The value for moduleAuthor
* @return {@code this} builder for use in a chained invocation
*/
public final Builder moduleAuthor(String moduleAuthor) {
this.moduleAuthor = Objects.requireNonNull(moduleAuthor, "moduleAuthor");
return this;
}
/**
* Initializes the value for the {@link TSModuleInfo#getModuleLicense() moduleLicense} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link TSModuleInfo#getModuleLicense() moduleLicense}.
* @param moduleLicense The value for moduleLicense
* @return {@code this} builder for use in a chained invocation
*/
public final Builder moduleLicense(String moduleLicense) {
this.moduleLicense = Objects.requireNonNull(moduleLicense, "moduleLicense");
return this;
}
/**
* Initializes the value for the {@link TSModuleInfo#getModuleAuthorUrl() moduleAuthorUrl} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link TSModuleInfo#getModuleAuthorUrl() moduleAuthorUrl}.
* @param moduleAuthorUrl The value for moduleAuthorUrl
* @return {@code this} builder for use in a chained invocation
*/
public final Builder moduleAuthorUrl(String moduleAuthorUrl) {
this.moduleAuthorUrl = Objects.requireNonNull(moduleAuthorUrl, "moduleAuthorUrl");
return this;
}
/**
* Put one entry to the {@link TSModuleInfo#getCustomMappings() customMappings} map.
* @param key The key in the customMappings map
* @param value The associated value in the customMappings map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putCustomMappings(String key, TSTargetType value) {
this.customMappings.put(
Objects.requireNonNull(key, "customMappings key"),
Objects.requireNonNull(value, "customMappings value"));
optBits |= OPT_BIT_CUSTOM_MAPPINGS;
return this;
}
/**
* Put one entry to the {@link TSModuleInfo#getCustomMappings() customMappings} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putCustomMappings(Map.Entry entry) {
String k = entry.getKey();
TSTargetType v = entry.getValue();
this.customMappings.put(
Objects.requireNonNull(k, "customMappings key"),
Objects.requireNonNull(v, "customMappings value"));
optBits |= OPT_BIT_CUSTOM_MAPPINGS;
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link TSModuleInfo#getCustomMappings() customMappings} map. Nulls are not permitted
* @param customMappings The entries that will be added to the customMappings map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder customMappings(Map customMappings) {
this.customMappings.clear();
optBits |= OPT_BIT_CUSTOM_MAPPINGS;
return putAllCustomMappings(customMappings);
}
/**
* Put all mappings from the specified map as entries to {@link TSModuleInfo#getCustomMappings() customMappings} map. Nulls are not permitted
* @param customMappings The entries that will be added to the customMappings map
* @return {@code this} builder for use in a chained invocation
*/
public final Builder putAllCustomMappings(Map customMappings) {
for (Map.Entry entry : customMappings.entrySet()) {
String k = entry.getKey();
TSTargetType v = entry.getValue();
this.customMappings.put(
Objects.requireNonNull(k, "customMappings key"),
Objects.requireNonNull(v, "customMappings value"));
}
optBits |= OPT_BIT_CUSTOM_MAPPINGS;
return this;
}
/**
* Initializes the optional value {@link TSModuleInfo#getJavaPackage() javaPackage} to javaPackage.
* @param javaPackage The value for javaPackage
* @return {@code this} builder for chained invocation
*/
public final Builder javaPackage(String javaPackage) {
this.javaPackage = Objects.requireNonNull(javaPackage, "javaPackage");
return this;
}
/**
* Initializes the optional value {@link TSModuleInfo#getJavaPackage() javaPackage} to javaPackage.
* @param javaPackage The value for javaPackage
* @return {@code this} builder for use in a chained invocation
*/
public final Builder javaPackage(Optional javaPackage) {
this.javaPackage = javaPackage.orElse(null);
return this;
}
/**
* Adds one element to {@link TSModuleInfo#getExcludes() excludes} list.
* @param element A excludes element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addExcludes(Pattern element) {
this.excludes.add(Objects.requireNonNull(element, "excludes element"));
optBits |= OPT_BIT_EXCLUDES;
return this;
}
/**
* Adds elements to {@link TSModuleInfo#getExcludes() excludes} list.
* @param elements An array of excludes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addExcludes(Pattern... elements) {
for (Pattern element : elements) {
this.excludes.add(Objects.requireNonNull(element, "excludes element"));
}
optBits |= OPT_BIT_EXCLUDES;
return this;
}
/**
* Sets or replaces all elements for {@link TSModuleInfo#getExcludes() excludes} list.
* @param elements An iterable of excludes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder excludes(Iterable extends Pattern> elements) {
this.excludes.clear();
return addAllExcludes(elements);
}
/**
* Adds elements to {@link TSModuleInfo#getExcludes() excludes} list.
* @param elements An iterable of excludes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllExcludes(Iterable extends Pattern> elements) {
for (Pattern element : elements) {
this.excludes.add(Objects.requireNonNull(element, "excludes element"));
}
optBits |= OPT_BIT_EXCLUDES;
return this;
}
/**
* Adds one element to {@link TSModuleInfo#getNameSpaceMappings() nameSpaceMappings} list.
* @param element A nameSpaceMappings element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addNameSpaceMappings(NameSpaceMapping element) {
this.nameSpaceMappings.add(Objects.requireNonNull(element, "nameSpaceMappings element"));
optBits |= OPT_BIT_NAME_SPACE_MAPPINGS;
return this;
}
/**
* Adds elements to {@link TSModuleInfo#getNameSpaceMappings() nameSpaceMappings} list.
* @param elements An array of nameSpaceMappings elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addNameSpaceMappings(NameSpaceMapping... elements) {
for (NameSpaceMapping element : elements) {
this.nameSpaceMappings.add(Objects.requireNonNull(element, "nameSpaceMappings element"));
}
optBits |= OPT_BIT_NAME_SPACE_MAPPINGS;
return this;
}
/**
* Sets or replaces all elements for {@link TSModuleInfo#getNameSpaceMappings() nameSpaceMappings} list.
* @param elements An iterable of nameSpaceMappings elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder nameSpaceMappings(Iterable extends NameSpaceMapping> elements) {
this.nameSpaceMappings.clear();
return addAllNameSpaceMappings(elements);
}
/**
* Adds elements to {@link TSModuleInfo#getNameSpaceMappings() nameSpaceMappings} list.
* @param elements An iterable of nameSpaceMappings elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllNameSpaceMappings(Iterable extends NameSpaceMapping> elements) {
for (NameSpaceMapping element : elements) {
this.nameSpaceMappings.add(Objects.requireNonNull(element, "nameSpaceMappings element"));
}
optBits |= OPT_BIT_NAME_SPACE_MAPPINGS;
return this;
}
/**
* Initializes the value for the {@link TSModuleInfo#getOutputType() outputType} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link TSModuleInfo#getOutputType() outputType}.
* @param outputType The value for outputType
* @return {@code this} builder for use in a chained invocation
*/
public final Builder outputType(OutputType outputType) {
this.outputType = Objects.requireNonNull(outputType, "outputType");
return this;
}
/**
* Initializes the value for the {@link TSModuleInfo#getNameSpaceMappingStrategy() nameSpaceMappingStrategy} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link TSModuleInfo#getNameSpaceMappingStrategy() nameSpaceMappingStrategy}.
* @param nameSpaceMappingStrategy The value for nameSpaceMappingStrategy
* @return {@code this} builder for use in a chained invocation
*/
public final Builder nameSpaceMappingStrategy(NameSpaceMappingStrategy nameSpaceMappingStrategy) {
this.nameSpaceMappingStrategy = Objects.requireNonNull(nameSpaceMappingStrategy, "nameSpaceMappingStrategy");
return this;
}
/**
* Initializes the value for the {@link TSModuleInfo#generateTypeGuards() generateTypeGuards} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link TSModuleInfo#generateTypeGuards() generateTypeGuards}.
* @param generateTypeGuards The value for generateTypeGuards
* @return {@code this} builder for use in a chained invocation
*/
public final Builder generateTypeGuards(boolean generateTypeGuards) {
this.generateTypeGuards = generateTypeGuards;
optBits |= OPT_BIT_GENERATE_TYPE_GUARDS;
return this;
}
/**
* Adds one element to {@link TSModuleInfo#additionalTypes() additionalTypes} list.
* @param element A additionalTypes element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAdditionalTypes(String element) {
this.additionalTypes.add(Objects.requireNonNull(element, "additionalTypes element"));
optBits |= OPT_BIT_ADDITIONAL_TYPES;
return this;
}
/**
* Adds elements to {@link TSModuleInfo#additionalTypes() additionalTypes} list.
* @param elements An array of additionalTypes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAdditionalTypes(String... elements) {
for (String element : elements) {
this.additionalTypes.add(Objects.requireNonNull(element, "additionalTypes element"));
}
optBits |= OPT_BIT_ADDITIONAL_TYPES;
return this;
}
/**
* Sets or replaces all elements for {@link TSModuleInfo#additionalTypes() additionalTypes} list.
* @param elements An iterable of additionalTypes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder additionalTypes(Iterable elements) {
this.additionalTypes.clear();
return addAllAdditionalTypes(elements);
}
/**
* Adds elements to {@link TSModuleInfo#additionalTypes() additionalTypes} list.
* @param elements An iterable of additionalTypes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllAdditionalTypes(Iterable elements) {
for (String element : elements) {
this.additionalTypes.add(Objects.requireNonNull(element, "additionalTypes element"));
}
optBits |= OPT_BIT_ADDITIONAL_TYPES;
return this;
}
/**
* Adds one element to {@link TSModuleInfo#getterPrefixes() getterPrefixes} list.
* @param element A getterPrefixes element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addGetterPrefixes(String element) {
this.getterPrefixes.add(Objects.requireNonNull(element, "getterPrefixes element"));
optBits |= OPT_BIT_GETTER_PREFIXES;
return this;
}
/**
* Adds elements to {@link TSModuleInfo#getterPrefixes() getterPrefixes} list.
* @param elements An array of getterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addGetterPrefixes(String... elements) {
for (String element : elements) {
this.getterPrefixes.add(Objects.requireNonNull(element, "getterPrefixes element"));
}
optBits |= OPT_BIT_GETTER_PREFIXES;
return this;
}
/**
* Sets or replaces all elements for {@link TSModuleInfo#getterPrefixes() getterPrefixes} list.
* @param elements An iterable of getterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder getterPrefixes(Iterable elements) {
this.getterPrefixes.clear();
return addAllGetterPrefixes(elements);
}
/**
* Adds elements to {@link TSModuleInfo#getterPrefixes() getterPrefixes} list.
* @param elements An iterable of getterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllGetterPrefixes(Iterable elements) {
for (String element : elements) {
this.getterPrefixes.add(Objects.requireNonNull(element, "getterPrefixes element"));
}
optBits |= OPT_BIT_GETTER_PREFIXES;
return this;
}
/**
* Adds one element to {@link TSModuleInfo#setterPrefixes() setterPrefixes} list.
* @param element A setterPrefixes element
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addSetterPrefixes(String element) {
this.setterPrefixes.add(Objects.requireNonNull(element, "setterPrefixes element"));
optBits |= OPT_BIT_SETTER_PREFIXES;
return this;
}
/**
* Adds elements to {@link TSModuleInfo#setterPrefixes() setterPrefixes} list.
* @param elements An array of setterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addSetterPrefixes(String... elements) {
for (String element : elements) {
this.setterPrefixes.add(Objects.requireNonNull(element, "setterPrefixes element"));
}
optBits |= OPT_BIT_SETTER_PREFIXES;
return this;
}
/**
* Sets or replaces all elements for {@link TSModuleInfo#setterPrefixes() setterPrefixes} list.
* @param elements An iterable of setterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder setterPrefixes(Iterable elements) {
this.setterPrefixes.clear();
return addAllSetterPrefixes(elements);
}
/**
* Adds elements to {@link TSModuleInfo#setterPrefixes() setterPrefixes} list.
* @param elements An iterable of setterPrefixes elements
* @return {@code this} builder for use in a chained invocation
*/
public final Builder addAllSetterPrefixes(Iterable elements) {
for (String element : elements) {
this.setterPrefixes.add(Objects.requireNonNull(element, "setterPrefixes element"));
}
optBits |= OPT_BIT_SETTER_PREFIXES;
return this;
}
/**
* Initializes the value for the {@link TSModuleInfo#nameMappingStrategy() nameMappingStrategy} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link TSModuleInfo#nameMappingStrategy() nameMappingStrategy}.
* @param nameMappingStrategy The value for nameMappingStrategy
* @return {@code this} builder for use in a chained invocation
*/
public final Builder nameMappingStrategy(NameMappingStrategy nameMappingStrategy) {
this.nameMappingStrategy = Objects.requireNonNull(nameMappingStrategy, "nameMappingStrategy");
return this;
}
/**
* Builds a new {@link TSModuleInfoBuilder TSModuleInfoBuilder}.
* @return An immutable instance of TSModuleInfo
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public TSModuleInfoBuilder build() {
return TSModuleInfoBuilder.validate(new TSModuleInfoBuilder(this));
}
private boolean customMappingsIsSet() {
return (optBits & OPT_BIT_CUSTOM_MAPPINGS) != 0;
}
private boolean excludesIsSet() {
return (optBits & OPT_BIT_EXCLUDES) != 0;
}
private boolean nameSpaceMappingsIsSet() {
return (optBits & OPT_BIT_NAME_SPACE_MAPPINGS) != 0;
}
private boolean generateTypeGuardsIsSet() {
return (optBits & OPT_BIT_GENERATE_TYPE_GUARDS) != 0;
}
private boolean additionalTypesIsSet() {
return (optBits & OPT_BIT_ADDITIONAL_TYPES) != 0;
}
private boolean getterPrefixesIsSet() {
return (optBits & OPT_BIT_GETTER_PREFIXES) != 0;
}
private boolean setterPrefixesIsSet() {
return (optBits & OPT_BIT_SETTER_PREFIXES) != 0;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList();
} else {
list = new ArrayList();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
private static Map createUnmodifiableMap(boolean checkNulls, boolean skipNulls, Map extends K, ? extends V> map) {
switch (map.size()) {
case 0: return Collections.emptyMap();
case 1: {
Map.Entry extends K, ? extends V> e = map.entrySet().iterator().next();
K k = e.getKey();
V v = e.getValue();
if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, "value");
}
if (skipNulls && (k == null || v == null)) {
return Collections.emptyMap();
}
return Collections.singletonMap(k, v);
}
default: {
Map linkedMap = new LinkedHashMap(map.size());
if (skipNulls || checkNulls) {
for (Map.Entry extends K, ? extends V> e : map.entrySet()) {
K k = e.getKey();
V v = e.getValue();
if (skipNulls) {
if (k == null || v == null) continue;
} else if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, "value");
}
linkedMap.put(k, v);
}
} else {
linkedMap.putAll(map);
}
return Collections.unmodifiableMap(linkedMap);
}
}
}
}