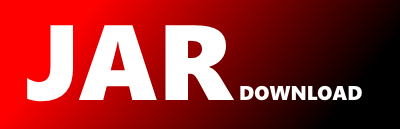
devutility.internal.util.CollectionUtils Maven / Gradle / Ivy
package devutility.internal.util;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Comparator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class CollectionUtils {
/**
* If collection does null or empty?
* @param collection: Elements collection.
* @return boolean
*/
public static boolean isNullOrEmpty(Collection collection) {
return collection == null || collection.size() == 0;
}
/**
* If collection does not empty?
* @param collection: Elements collection.
* @return boolean
*/
public static boolean isNotEmpty(Collection collection) {
return collection != null && collection.size() > 0;
}
/**
* Sort by comparator and return the minimum value.
* @param collection: Elements collection.
* @param comparator: Comparator for sort.
* @return {@code E}
*/
public static E min(Collection collection, Comparator super E> comparator) {
Optional optional = collection.stream().min(comparator);
if (optional.isPresent()) {
return optional.get();
}
return null;
}
/**
* Return the minimum integer value.
* @param collection: Elements collection.
* @return int
*/
public static int minInt(Collection collection) {
Integer value = min(collection, Comparator.comparingInt(i -> i));
if (value == null) {
return 0;
}
return value;
}
/**
* Sort by comparator and return the maximum value.
* @param collection: Elements collection.
* @param comparator: Comparator for sort.
* @return {@code E}
*/
public static E max(Collection collection, Comparator super E> comparator) {
Optional optional = collection.stream().max(comparator);
if (optional.isPresent()) {
return optional.get();
}
return null;
}
/**
* Return the maximum integer value.
* @param collection: Elements collection.
* @return int
*/
public static int maxInt(Collection collection) {
Integer value = max(collection, Comparator.comparingInt(i -> i));
if (value == null) {
return 0;
}
return value;
}
/**
* Calculate elements that meet predicate command.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @return long
*/
public static long count(Collection collection, Predicate predicate) {
return collection.stream().filter(predicate).count();
}
/**
* Execute predicate and check whether any object exist?
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @return boolean
*/
public static boolean exist(Collection collection, Predicate predicate) {
return count(collection, predicate) > 0;
}
/**
* Find element by predicate command and return optional object.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @return {@code Optional}
*/
public static Optional findOptional(Collection collection, Predicate predicate) {
return collection.stream().filter(predicate).findAny();
}
/**
* Find element by predicate command and return it.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @return {@code E}
*/
public static E find(Collection collection, Predicate predicate) {
Optional optional = findOptional(collection, predicate);
if (optional.isPresent()) {
return optional.get();
}
return null;
}
/**
* Parallel find element by predicate command and return optional object.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @return {@code Optional}
*/
public static Optional parallelFindOptional(Collection collection, Predicate predicate) {
return collection.stream().parallel().filter(predicate).findAny();
}
/**
* Parallel find element by predicate command and return it.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @return {@code E}
*/
public static E parallelFind(Collection collection, Predicate predicate) {
Optional optional = parallelFindOptional(collection, predicate);
if (optional.isPresent()) {
return optional.get();
}
return null;
}
/**
* Query elements by predicate command and return a stream.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @return {@code Stream}
*/
public static Stream query(Collection collection, Predicate predicate) {
return collection.stream().filter(predicate);
}
/**
* Parallel query elements by predicate command and return a stream.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @return {@code Stream}
*/
public static Stream parallelQuery(Collection collection, Predicate predicate) {
return collection.stream().parallel().filter(predicate);
}
/**
* Query elements by predicate command and return a list.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @return {@code List}
*/
public static List list(Collection collection, Predicate predicate) {
return query(collection, predicate).collect(Collectors.toList());
}
/**
* Parallel query elements by predicate command and return a list.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @return {@code List}
*/
public static List parallelList(Collection collection, Predicate predicate) {
return parallelQuery(collection, predicate).collect(Collectors.toList());
}
/**
* Map some fields and return a stream.
* @param collection: Elements collection.
* @param mapper: Fields mapper.
* @return {@code Stream}
*/
public static Stream map(Collection collection, Function super E, ? extends R> mapper) {
return collection.stream().map(mapper);
}
/**
* Map some fields and return a list.
* @param collection: Elements collection.
* @param mapper: Fields mapper.
* @return {@code List}
*/
public static List mapToList(Collection collection, Function super E, ? extends R> mapper) {
return map(collection, mapper).collect(Collectors.toList());
}
/**
* Query elements by predicate command, map some fields and return a stream.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @param mapper: Fields mapper.
* @return {@code Stream}
*/
public static Stream map(Collection collection, Predicate predicate, Function super E, ? extends R> mapper) {
return query(collection, predicate).map(mapper);
}
/**
* Query elements by predicate command, map some fields and return a list.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @param mapper: Fields mapper.
* @return {@code List}
*/
public static List mapToList(Collection collection, Predicate predicate, Function super E, ? extends R> mapper) {
return map(collection, predicate, mapper).collect(Collectors.toList());
}
/**
* Parallel map some fields and return a stream.
* @param collection: Elements collection.
* @param mapper: Fields mapper.
* @return {@code Stream}
*/
public static Stream parallelMap(Collection collection, Function super E, ? extends R> mapper) {
return collection.stream().parallel().map(mapper);
}
/**
* Parallel map some fields and return a list.
* @param collection: Elements collection.
* @param mapper: Fields mapper.
* @return {@code List}
*/
public static List parallelMapToList(Collection collection, Function super E, ? extends R> mapper) {
return parallelMap(collection, mapper).collect(Collectors.toList());
}
/**
* Parallel query elements by predicate command, map some fields and return a
* stream.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @param mapper: Fields mapper.
* @return {@code Stream}
*/
public static Stream parallelMap(Collection collection, Predicate predicate, Function super E, ? extends R> mapper) {
return collection.stream().parallel().filter(predicate).map(mapper);
}
/**
* Parallel query elements by predicate command, map some fields and return a
* list.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @param mapper: Fields mapper.
* @return {@code List}
*/
public static List parallelMapToList(Collection collection, Predicate predicate, Function super E, ? extends R> mapper) {
return parallelMap(collection, predicate, mapper).collect(Collectors.toList());
}
/**
* Map some fields, remove repeated items and return a stream.
* @param collection: Elements collection.
* @param mapper: Fields mapper.
* @return {@code Stream extends R>}
*/
public static Stream extends R> mapAndDistinct(Collection collection, Function super E, ? extends R> mapper) {
return map(collection, mapper).distinct();
}
/**
* Map some fields, remove repeated items and return a list.
* @param collection: Elements collection.
* @param mapper: Fields mapper.
* @return {@code List}
*/
public static List mapAndDistinctToList(Collection collection, Function super E, ? extends R> mapper) {
return mapAndDistinct(collection, mapper).collect(Collectors.toList());
}
/**
* Query elements by predicate command, map some fields, remove repeated items
* and return a stream.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @param mapper: Fields mapper.
* @return {@code Stream extends R>}
*/
public static Stream extends R> mapAndDistinct(Collection collection, Predicate predicate, Function super E, ? extends R> mapper) {
return map(collection, predicate, mapper).distinct();
}
/**
* Query elements by predicate command, map some fields, remove repeated items
* and return a list.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @param mapper: Fields mapper.
* @return {@code List}
*/
public static List mapAndDistinctToList(Collection collection, Predicate predicate, Function super E, ? extends R> mapper) {
return mapAndDistinct(collection, predicate, mapper).collect(Collectors.toList());
}
/**
* Map some fields (for each item, one field has an collection of values) and
* return a list.
* @param collection: Elements collection.
* @param mapper: Fields mapper.
* @return {@code List}
*/
public static List mapManyToList(Collection collection, Function super E, ? extends Stream extends R>> mapper) {
List list = new LinkedList<>();
map(collection, mapper).forEach(i -> {
list.addAll(i.collect(Collectors.toList()));
});
return list;
}
/**
* Group collection by classifier to map and return it.
* @param collection: Elements collection.
* @param classifier: Classifier for group.
* @return {@code Map>}
*/
public static Map> groupToMap(Collection collection, Function super E, ? extends K> classifier) {
return collection.stream().collect(Collectors.groupingBy(classifier));
}
/**
* Parallel group collection by classifier to map and return it.
* @param collection: Elements collection.
* @param classifier: Classifier for group.
* @return {@code Map>}
*/
public static Map> parallelGroupToMap(Collection collection, Function super E, ? extends K> classifier) {
return collection.stream().parallel().collect(Collectors.groupingBy(classifier));
}
/**
* Query elements by predicate command, group collection by classifier to map
* and return it.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @param classifier: Classifier for group.
* @return {@code Map>}
*/
public static Map> groupToMap(Collection collection, Predicate predicate, Function super E, ? extends K> classifier) {
return query(collection, predicate).collect(Collectors.groupingBy(classifier));
}
/**
* Parallel group elements by predicate command, group collection by classifier
* to map and return it.
* @param collection: Elements collection.
* @param predicate: Predicate command.
* @param classifier: Classifier for group.
* @return {@code Map>}
*/
public static Map> parallelGroupToMap(Collection collection, Predicate predicate, Function super E, ? extends K> classifier) {
return parallelQuery(collection, predicate).collect(Collectors.groupingBy(classifier));
}
/**
* Paging collection with specified page index and page size.
* @param collection: Elements collection.
* @param pageIndex: Page index.
* @param pageSize: Page size.
* @return {@code List}
*/
public static List paging(Collection collection, int pageIndex, int pageSize) {
if (isNullOrEmpty(collection) || pageIndex < 1) {
return new ArrayList<>();
}
int skip = (pageIndex - 1) * pageSize;
if (skip < 0 || skip >= collection.size()) {
return new ArrayList<>();
}
return collection.stream().skip(skip).limit(pageSize).collect(Collectors.toList());
}
/**
* Get the toppest count elements.
* @param collection: Elements collection.
* @param count: Count number.
* @return {@code List}
*/
public static List top(Collection collection, int count) {
if (isNullOrEmpty(collection) || count < 1) {
return new ArrayList<>();
}
return collection.stream().limit(count).collect(Collectors.toList());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy