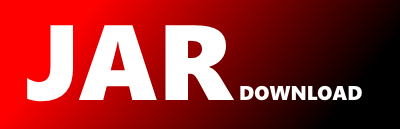
devutility.internal.text.format.DateFormatHelper Maven / Gradle / Ivy
package devutility.internal.text.format;
import java.text.SimpleDateFormat;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
public class DateFormatHelper {
/**
* Container for SimpleDateFormat
*/
private static volatile Map> container = new HashMap<>();
/**
* Standard DateFormat
*/
public final static String STANDARDDATEFORMAT = "yyyy-MM-dd";
/**
* Standard DateTime format
*/
public final static String STANDARDDATETIMEFORMAT = "yyyy-MM-dd HH:mm:ss";
/**
* Get standard date format
* @return SimpleDateFormat
*/
public static SimpleDateFormat getStandardDateFormat() {
return getSimpleDateFormat(STANDARDDATEFORMAT);
}
/**
* Get simple date format
* @param pattern: Date pattern.
* @return SimpleDateFormat
*/
public static SimpleDateFormat getSimpleDateFormat(String pattern) {
ThreadLocal threadLocal = container.get(pattern);
if (threadLocal != null) {
if (threadLocal.get() != null) {
return threadLocal.get();
}
container.put(pattern, null);
}
synchronized (DateFormatHelper.class) {
if (container.get(pattern) == null) {
SimpleDateFormat simpleDateFormat = new SimpleDateFormat(pattern);
threadLocal = new ThreadLocal();
threadLocal.set(simpleDateFormat);
container.put(pattern, threadLocal);
}
}
return threadLocal.get();
}
/**
* Get simple date format
* @param pattern: Date pattern.
* @param locale: Locale for date
* @return SimpleDateFormat
*/
public static SimpleDateFormat getSimpleDateFormat(String pattern, Locale locale) {
String key = String.format("%s-%s", pattern, locale.toString());
ThreadLocal threadLocal = container.get(key);
if (threadLocal != null) {
if (threadLocal.get() != null) {
return threadLocal.get();
}
container.put(key, null);
}
synchronized (DateFormatHelper.class) {
if (container.get(key) == null) {
SimpleDateFormat simpleDateFormat = new SimpleDateFormat(pattern, locale);
threadLocal = new ThreadLocal();
threadLocal.set(simpleDateFormat);
container.put(key, threadLocal);
}
}
return threadLocal.get();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy