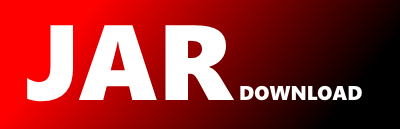
META-INF.templates.formulas.vm Maven / Gradle / Ivy
package $package;
import static com.github.eduardovalentim.easymath.Numbers.*;
import java.math.BigDecimal;
import java.math.MathContext;
import java.math.RoundingMode;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.github.eduardovalentim.easymath.FunctionCatalog;
import com.github.eduardovalentim.easymath.functions.CoreFunctionCatalog;
@Generated("$generator")
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class $classname extends $superclass {
private static Logger logger = LoggerFactory.getLogger(${classname}.class);
#foreach( $constant in ${constants} )
#declareConstant( $constant )
#end
private FunctionCatalog catalog = new CoreFunctionCatalog();
/**
* Public constructor with catalog customization
* @param catalogs
*/
public $classname(FunctionCatalog... catalogs) {
/*
* Method protection
*/
if (catalogs == null)
throw new IllegalArgumentException("Argument 'catalogs' cannot be null.");
/*
* Join the default catalog with all informed
*/
this.catalog = catalog.join(catalogs);
}
#foreach( $function in ${functions} )
/**
* The implementation of the formula: ${function.formula.value()}
* @param inputs The inputs for calculation
* @return the result of the calculation
*/
@Override
public ${function.type} ${utils.uncapitalize($function.name)}(Number... inputs) {
/*
* Method protection block
*/
if (inputs == null)
throw new IllegalArgumentException("Argument 'inputs' cannot be null.");
if (inputs.length != ${function.inputs.size()})
throw new IllegalArgumentException(
"Length mismatch for argument 'inputs'. Expected '${function.inputs.size()}' actual '" + inputs.length + "'");
/*
* Typecast block
*/
#typecast( ${function} )
return ${utils.uncapitalize($function.name)}( #arguments( ${function.inputs} ) );
}
/**
* The implementation of the formula: ${function.formula.value()}
#javadoc( ${function.inputs} )
* @return the result of the calculation
*/
public ${function.type} ${utils.uncapitalize($function.name)}( #typedArguments( ${function.type} ${function.inputs} )) {
#if( ${function.inputs.empty} )
logger.trace("Entering...");
#else
logger.trace("Entering with arguments: {}", new Object[] {#arguments( ${function.inputs} )});
#end
#check( $function )
/*
* Function precision and rounding mode definition
*/
MathContext mc = new MathContext(${function.precision}, RoundingMode.${function.roundingMode});
/*
* Function resolution
*/
#foreach( $operation in ${function.operations} )
#declareOperation( $function $operation )
#end
/*
* Result
*/
logger.trace("Exiting...");
return ${function.lastOperation.id};
}
#end
}
## ----------------------------------------------------------------------------
## javadoc
## ----------------------------------------------------------------------------
#macro( javadoc $inputs )
#foreach( $input in ${inputs} )
* @param ${input.id} The ${input.id} input
#end
#end
## ----------------------------------------------------------------------------
## arguments
## ----------------------------------------------------------------------------
#macro( arguments $inputs )
#foreach( $input in ${inputs} )
${input.id}#if( ${foreach.hasNext} ), #end
#end
#end
## ----------------------------------------------------------------------------
## typedArguments
## ----------------------------------------------------------------------------
#macro( typedArguments $type $inputs)
#foreach( $input in ${inputs} )
$type ${input.id}#if( ${foreach.hasNext} ), #end
#end
#end
## ----------------------------------------------------------------------------
## resolutionArguments
## ----------------------------------------------------------------------------
#macro( resolutionArguments $inputs )
#foreach( $input in ${inputs} )
${input}#if( ${foreach.hasNext} ), #end
#end
#end
## ----------------------------------------------------------------------------
## check
## ----------------------------------------------------------------------------
#macro( check $function )
#if( ${function.type} == "java.math.BigDecimal")
/*
* Method protection block
*/
#foreach( $input in ${function.inputs} )
if (${input.id} == null)
throw new IllegalArgumentException("Argument '${input.id}' cannot be null.");
#end
#end
#end
## ----------------------------------------------------------------------------
## typecast
## ----------------------------------------------------------------------------
#macro( typecast $function )
#foreach( $input in ${function.inputs} )
#if( ${function.type} == "double" || ${function.type} == "java.lang.Double")
${function.type} ${input.id} = toDouble(inputs[${foreach.index}], ${foreach.index});
#elseif( ${function.type} == "java.math.BigDecimal" )
${function.type} ${input.id} = toBigDecimal(inputs[${foreach.index}], ${foreach.index});
#else
Error declaring type: Type not found!!!!
#end
#end
#end
## ----------------------------------------------------------------------------
## declareConstant
## ----------------------------------------------------------------------------
#macro( declareConstant $constant )
#if( ${constant.type} == "double" || ${constant.type} == "java.lang.Double")
private static final ${constant.type} ${constant.id} = ${constant.value}D;
#elseif( ${constant.type} == "java.math.BigDecimal" )
private static final ${constant.type} ${constant.id} = new BigDecimal("${constant.value}");
#else
Error declaring constant: Type not found!!!!
#end
#end
## ----------------------------------------------------------------------------
## declareOperation
## ----------------------------------------------------------------------------
#macro( declareOperation $function $operation )
#if( ${operation.class.simpleName} == "UnaryOperation" )
#declareUnary( $operation )
#elseif( ${operation.class.simpleName} == "BinaryOperation" )
#declareBinary( $operation )
#elseif( ${operation.class.simpleName} == "FunctionOperation" )
#declareFunction( $operation )
#else
Operation not supported!!!!!
#end
#end
## ----------------------------------------------------------------------------
## declareUnary
## ----------------------------------------------------------------------------
#macro( declareUnary $operation )
#if( ${operation.type} == "double" || ${operation.type} == "java.lang.Double")
#if( ${operation.operator} == '!' )
${operation.type} ${operation.id} = toDouble(catalog.solve("fat", mc, ${operation.operand.id}), 0);
#else
${operation.type} ${operation.id} = ${operation.operand.id} * (${operation.operator}1);
#end
#else
#if( ${operation.operator} == '!' )
${operation.type} ${operation.id} = toBigDecimal(catalog.solve("fat", mc, ${operation.operand.id}), 0);
#elseif( ${operation.operator} == '-' )
${operation.type} ${operation.id} = ${operation.operand.id}.multiply(BigDecimal.ONE.negate(), mc);
#else
${operation.type} ${operation.id} = ${operation.operand.id};
#end
#end
#end
## ----------------------------------------------------------------------------
## declareBinary
## ----------------------------------------------------------------------------
#macro( declareBinary $operation )
#if( ${operation.type} == "double" || ${operation.type} == "java.lang.Double")
#if( ${operation.operator} == '^' )
${operation.type} ${operation.id} = toDouble(catalog.solve("pow", mc, ${operation.leftOperand.id}, ${operation.rightOperand.id}), 0);
#elseif( ${operation.operator} == '*' )
${operation.type} ${operation.id} = ${operation.leftOperand.id} * ${operation.rightOperand.id};
#elseif( ${operation.operator} == '/' )
${operation.type} ${operation.id} = ${operation.leftOperand.id} / ${operation.rightOperand.id};
#elseif( ${operation.operator} == '%' )
${operation.type} ${operation.id} = ${operation.leftOperand.id} % ${operation.rightOperand.id};
#elseif( ${operation.operator} == '+' )
${operation.type} ${operation.id} = ${operation.leftOperand.id} + ${operation.rightOperand.id};
#elseif( ${operation.operator} == '-' )
${operation.type} ${operation.id} = ${operation.leftOperand.id} - ${operation.rightOperand.id};
#else
Operator '${operation.operator}' not found!!!!!!
#end
#elseif( ${operation.type} == "java.math.BigDecimal" )
#if( ${operation.operator} == '^' )
${operation.type} ${operation.id} = toBigDecimal(catalog.solve("pow", mc, ${operation.leftOperand.id}, ${operation.rightOperand.id}), 0);
#elseif( ${operation.operator} == '*' )
${operation.type} ${operation.id} = ${operation.leftOperand.id}.multiply(${operation.rightOperand.id}, mc);
#elseif( ${operation.operator} == '/' )
${operation.type} ${operation.id} = ${operation.leftOperand.id}.divide(${operation.rightOperand.id}, mc);
#elseif( ${operation.operator} == '%' )
${operation.type} ${operation.id} = ${operation.leftOperand.id}.remainder(${operation.rightOperand.id}, mc);
#elseif( ${operation.operator} == '+' )
${operation.type} ${operation.id} = ${operation.leftOperand.id}.add(${operation.rightOperand.id}, mc);
#elseif( ${operation.operator} == '-' )
${operation.type} ${operation.id} = ${operation.leftOperand.id}.subtract(${operation.rightOperand.id}, mc);
#else
Operator '${operation.operator}' not found!!!!!!
#end
#end
#end
## ----------------------------------------------------------------------------
## declareFunction
## ----------------------------------------------------------------------------
#macro( declareFunction $operation )
#if( ${function.type} == "double" || ${function.type} == "java.lang.Double")
${operation.type} ${operation.id} = toDouble(catalog.solve("${operation.name}", mc, #arguments( ${operation.operands} )), 0);
#elseif( ${function.type} == "java.math.BigDecimal" )
${operation.type} ${operation.id} = toBigDecimal(catalog.solve("${operation.name}", mc, #arguments( ${operation.operands} )), 0);
#else
Error in operation type: Type not found!!!!
#end
#end
© 2015 - 2025 Weber Informatics LLC | Privacy Policy