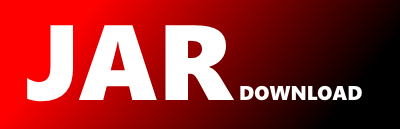
com.github.egatlovs.variablemanager.managers.RuntimeVariableManager Maven / Gradle / Ivy
package com.github.egatlovs.variablemanager.managers;
import org.camunda.bpm.engine.RuntimeService;
public interface RuntimeVariableManager {
/**
* Sets a Variable to the Execution.
*
* Note: the variable will be processed as follows:
*
* - The object will be validated using bean validation.
* - The given object will be processed using
* {@code ProcessingUnit.class}
*
* If you want more Information of how variables are processed watch out for
* {@code ProcessingUnit.class}.
* If you want to manipulate how your variables are processed look for
* {@code @ObjectValue}, {@code @FileValue}, {@code @FieldName} and {@code @Ignore}.
*
*
* @param value - The value to be set to the Execution
* @param executionId - The id of the execution to be used
*/
void setVariable(Object value, String executionId);
/**
* Sets a Variable Locally to the Execution.
*
* Note: the variable will be processed as follows:
*
* - The object will be validated using bean validation.
* - The given object will be processed using
* {@code ProcessingUnit.class}
*
* If you want more Information of how variables are processed watch out for
* {@code ProcessingUnit.class}.
* If you want to manipulate how your variables are processed look for
* {@code @ObjectValue}, {@code @FileValue}, {@code @FieldName} and {@code @Ignore}.
*
*
* @param value - The value to be set to the Execution
* @param executionId - The id of the execution to be used
*/
void setVariableLocal(Object value, String executionId);
/**
* Retrieves a Variable from the Execution.
*
* The variables will be read from the given class using
* {@code FieldNames.class}. After that each variable will be called out of the
* execution.
* Now that each variable is retrieved from the execution, the
* {@code ResultObject.class} will be used to build the requested object which
* is then returned.
*
* @param clazz - The Class which should be gathered
* @param executionId - The id of the execution to be used
* @return - The Object of the requested class
*/
T getVariable(Class clazz, String executionId);
/**
* Retrieves a Variable locally from the Execution.
*
* The variables will be read from the given class using
* {@code FieldNames.class}. After that each variable will be called out of the
* execution.
* Now that each variable is retrieved from the execution, the
* {@code ResultObject.class} will be used to build the requested object which
* is then returned.
*
* @param clazz - The Class which should be gathered
* @param executionId - The id of the execution to be used
* @return - The Object of the requested class
*/
T getVariableLocal(Class clazz, String executionId);
/**
* Removes a Variable from the Execution.
*
* The variables will be read from the given class using
* {@code FieldNames.class}. After that each variable will be removed from the
* execution.
*
* @param clazz - The Class which should be used to retrieve the fieldnames to be
* deleted
* @param executionId - The id of the execution to be used
*/
void removeVariables(Class clazz, String executionId);
/**
* Removes Variables locally from the Execution.
*
* The variables will be read from the given class using
* {@code FieldNames.class}. After that each variable will be removed from the
* execution.
*
* @param clazz - The Class which should be used to retrieve the fieldnames to be
* deleted
* @param executionId - The id of the execution to be used
*/
void removeVariablesLocal(Class clazz, String executionId);
/**
* Gives access to the wrapped runtimeService.
*
* @return Returns the wrapped runtimeService
*/
RuntimeService getExecutionService();
/**
* Manipulate the runtimeService used.
*
* @param runtimeService - The new runtimeService to be used
*/
void setExecutionService(RuntimeService runtimeService);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy